To present its content onscreen, a collection view cooperates with many different objects. Some objects are custom and must be provided by your app. For example, your app must provide a data source object that tells the collection view how many items there are to display. Other objects are provided by UIKit and are part of the basic collection view design.
Like tables, collection views are data-oriented objects whose implementation involves a collaboration with your app’s objects. Understanding what you have to do in your code requires a little background information about how a collection view does what it does.
A Collection View Is a Collaboration of Objects(集合视图是对象之间的协作)
The design of collection views separates the data being presented from the way that data is arranged and presented onscreen. Although your app is strictly responsible for managing the data to be presented, its visual presentation is managed by many different objects. Table 1-1 lists the collection view classes in UIKit and organizes them by the roles they play in implementing a collection view interface. Most of the classes are designed to be used as is without any need for subclassing, so you can usually implement a collection view with very little code. And when you want to go beyond the provided behavior, you can subclass and provide that behavior
集合视图的设计分开了展示数据和屏幕上呈现的内容。尽管你的应用严格按照数据来展现内容,但是展示的内容由多个不同对象决定。表1.1列出了UIKit中的集合视图类以及他们是如何按照在集合视图界面中扮演的角色来组织他们的。这些类中的大部分被设计成不用子类化就可以直接使用的,所以你通常可以用很少的代码完成一个集合视图。当你想要做超过系统提供的行为的时候,你可以子类化,并且提供你的自定义行为。
Purpose
目的
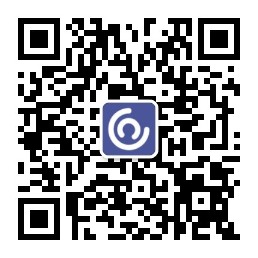
Classes/Protocols
类/协议
Description/ 描述
Table 1-1 The classes and protocols for implementing collection views 实现集合视图的类和协议 Top-level containment and management 最高级的包含和管理 |
A UICollectionView object defines the visible area for your collection view’s content. This class descends from UIScrollView and can contain a large scrollable area as needed. This class also facilitates the presentation of your data based on the layout information it receives from its layout object. UICollectionView为你的集合视图内容定义了可视区域。这个类继承自UIScrollView可以按照需要包含一片挺大的滚动区域。这个类很便于展示你的基于从布局对象获取的布局信息的数据。 A UICollectionViewController object provides view controller?level management support for a collection view. Its use is optional. UICollectionViewController 对象为集合视图提供了视图控制器级别的管理,他的使用是可选的。 |
|
Content management 内容管理 |
UICollectionViewDataSource protocol UICollectionViewDelegate protocol |
The data source object is the most important object associated with the collection view and is one that you must provide. The data source manages the content of the collection view and creates the views needed to present that content. To implement a data source object, you must create an object that conforms to the UICollectionViewDataSource protocol. 数据源对象是和集合视图有关的最重要的对象同事你必须完成他。数据源管理着集合视图的内容同时创建内容上的视图。为了实现数据源对象,你需要创建一个遵守数据源协议的对象。 The collection view delegate object lets you intercept interesting messages from the collection view and customize the view’s behavior. For example, you use a delegate object to track the selection and highlighting of items in the collection view. Unlike the data source object, the delegate object is optional. 集合视图协议对象让你可以拦截集合对象的有趣的信息,同时自定义视图行为。比如,你可以使用这个协议对象来跟踪选择,同时突出被选择的元素。和数据源对象不同的是,delegate的完成是可选的。 For information about how to implement the data source and delegate objects, see Designing Your Data Source and Delegate. 更多关于数据源和协议的资料,请见《设计你的数据源和协议》 |
Presentation 展示 |
All views displayed in a collection view must be instances of theUICollectionReusableView class. This class supports a recycling mechanism in use by collection views. Recycling views (instead of creating new ones) improves performance in general and especially improves it during scrolling. 所有集合视图中展示的视图都必须是UICollectionReusableView类的实例,这个类提供了集合视图中使用的回收机制。回收视图(替代创建新视图)一般可以提高新能,尤其是在滚动视图的时候 A UICollectionViewCell object is a specific type of reusable view that you use for your main data items. UICollectionViewCell对象是特定的一种重用视图,你用它作为你主要的数据项。 |
|
Layout 布局 |
Subclasses of UICollectionViewLayout are referred to as layout objects and are responsible for defining the location, size, and visual attributes of the cells and reusable views inside a collection view. UICollectionViewLayout的子类作为布局对象同时负责定义位置,大小,集合视图中的单元格与复用视图的视觉属性 During the layout process, a layout object creates layout attribute objects (instances of theUICollectionViewLayoutAttributes class) that tell the collection view where and how to display cells and reusable views. 布局的过程中,布局对象创建布局属性对象(UICollectionViewLayoutAttributes 的实例)告诉集合视图在什么位置,以什么形式展示单元格和复用视图。 The layout object receives instances of the UICollectionViewUpdateItem class whenever data items are inserted, deleted, or moved within the collection view. You never need to create instances of this class yourself. 不论何时集合视图中的数据项被插入,删除,移动,布局对象都会接受一个UICollectionViewUpdateItem类的实例。你不需要自己创建类的实例。 For more information about the layout object, see The Layout Object Controls the Visual Presentation. 更多关于布局对象的信息,请见《布局对象控制的视觉表现》 |
|
Flow layout 流动布局 |
The UICollectionViewFlowLayout class is a concrete layout object that you use to implement grids or other line-based layouts. You can use the class as-is or in conjunction with the flow delegate object, which allows you to customize the layout information dynamically.
UICollectionViewLayout类是一个实现网格和其他线性布局的布局对象。你可以直接使用这个类或者实现流动代理对象,这样你就可以实现自定义动态布局。
|
Figure 1-1 shows the relationship between the core objects associated with a collection view. The collection view gets information about the cells to display from its data source. The data source and delegate objects are custom objects provided by your app and used to manage the content, including the selection and highlighting of cells. The layout object is responsible for deciding where those cells belong and for sending that information to the collection view in the form of one or more layout attribute objects. The collection view then merges the layout information with the actual cells (and other views) to create the final visual presentation.
Figure 1-1 Merging content and layout to create the final presentation
图1-1展示了集合视图的相关核心类之间的关系。集合视图从数据源获取单元格展示信息。数据源和委托对象是由系统提供的自定义对象被用来管理内容,包括单元格的选中和强调状态。布局对象负责选择单元格的位置和用布局属性对象的形式给集合视图发出相关信息。然后集合视图会混合布局信息和实际的单元格(和其他视图)来创建最终的界面。
When creating a collection view interface, you first add a UICollectionView object to your storyboard or nib file. Think of the collection view as the central hub, from which all other objects emanate. After adding that object, you can begin to configure any related objects, such as the data source or delegate. All configurations are centered around the collection view itself. For example, you never create a layout object without also creating a collection view object.
Reusable Views Improve Performance(重用视图来提高性能)
Collection views employ a view recycling program to improve efficiency. As views move offscreen, they are removed from view and placed in a reuse queue instead of being deleted. As new content is scrolled onscreen, views are removed from the queue and repurposed with new content. To facilitate this recycling and reuse, all views displayed by the collection view must descend from theUICollectionReusableView class.
Collection views support three distinct types of reusable views, each of which has a specific intended usage:
Cells present the main content of your collection view. The job of a cell is to present the content for a single item from your data source object. Each cell must be an instance of theUICollectionViewCell class, which you may subclass as needed to present your content. Cell objects provide inherent support for managing their own selection and highlight state. To actually apply a highlight to a cell, you must write some custom code. For information on implementing cell highlighting/selecting, see Managing the Visual State for Selections and Highlights.
Supplementary views display information about a section. Like cells, supplementary views are data driven. Unlike cells, supplementary views are not mandatory, and their usage and placement is controlled by the layout object being used. For example, the flow layout supports headers and footers as optional supplementary views.
Decoration views are visual adornments that are wholly owned by the layout object and are not tied to any data in your data source object. For example, a layout object might use decoration views to implement a custom background appearance.
集合视图支持三种冥想不同的复用视图,每一种都有特定的用途。Unlike table views, collection views impose no specific style on the cells and supplementary views provided by your data source. Instead, the basic reusable view classes are blank canvases for you to modify. For example, you can use them to build small view hierarchies, to display images, or even to draw content dynamically.
Your data source object is responsible for providing the cells and supplementary views used by its associated collection view. However, the data source never creates views directly. When asked for a view, your data source dequeues a view of the desired type using the methods of the collection view. The dequeueing process always returns a valid view, either by retrieving one from a reuse queue or by using a class, nib file, or storyboard you provide to create a new view.
For information about how to create and configure views from your data source, see Configuring Cells and Supplementary Views.
The Layout Object Controls the Visual Presentation(布局对象控制视觉展示)
The layout object is solely responsible for determining the placement and visual styling of items within the collection view. Although your data source object provides the views and the actual content, the layout object determines the size, location, and other appearance-related attributes of those views. This separation of responsibilities makes it possible to change layouts dynamically without changing any of the data objects managed by your app.
The layout process used by collection views is related to, but distinct from, the layout process used by the rest of your app’s views. In other words, do not confuse what a layout object does with thelayoutSubviews method used to reposition child views inside a parent view. A layout object never touches the views it manages directly because it does not actually own any of those views. Instead, it generates attributes that describe the location, size, and visual appearance of the cells, supplementary views, and decoration views in the collection view. It is then the job of the collection view to apply those attributes to the actual view objects.
There are no limits to how a layout object can affect the views in a collection view. A layout object can move some views but not others. It can move views only a little bit, or it can move them randomly around the screen. It can even reposition views without any regard for the surrounding views. For example, a layout object can stack views on top of each other if it wants. The only real limitation is how the layout object affects the visual style you want your app to have.
Figure 1-2 shows how a vertically scrolling flow layout arranges its cells and supplementary views. In a vertically scrolling flow layout, the width of the content area remains fixed and the height grows to accommodate the content. To compute the area, the layout object places views and cells one at a time, choosing the most appropriate location for each. In the case of the flow layout, the size of the cells and supplementary views are specified as properties, either on the layout object or by using a delegate. Computing the layout is just a matter of using those properties to place each view.
布局对象的唯一职责就是决定集合视图中项的位置的视觉表现形式。尽管你的数据源支持视图和实际内容,布局对象决定了视图的大小,位置,和其他视图的布局相关的属性。这种责任的分离让你可以在不改变你应用的数据源的情况下动态的布局。
集合视图布局的过程和你的应用其余视图的布局过程相关,但又有冥想区别。换句话说,不要混用布局对象的layoutSubviews方法(这个方法在俯视图中布局子视图)。布局对象并不直接作用于视图,因为它并不实际拥有这些视图。相反的,它产程描述单元格,增补视图,装饰视图的位置,大小和视觉表现形式的属性。然后集合视图回把这些属性应用到真实的视图对象上
布局对象作用在集合视图上没有什么限制。布局对象可以移动一些视图,但是不能移动其他的。布局对象可以只挪动视图一点点,也可以把他们移动到屏幕上的随机位置。它甚至可以把视图放到和周围视图没有关系的位置。比如,只要你想,你可以让布局对象把视图们排成一排。真正能限制布局对象的是你的应用到底想要哪种展示方式?
图2-1展示了一个垂直滚动刘布局安排单元格和增补视图。在垂直滚动布局中,内容视图的宽是固定的,高根据内容视图来调整以容纳内容视图。布局对象通过计算区域来一次性布局所有的视图和单元格,并为所有的视图选好合适的位置。在这个流布局中,单元格和增补视图的大小被指定为属性,不管是在布局对象,或者是代理。计算布局就是使用这些属性来确定每个视图的位置。
Figure 1-2 The layout object provides layout metrics
Layout objects control more than just the size and position of their views. The layout object can specify other view-related attributes, such as its transparency, its transform in 3D space, and its visibility (if any) above or below other views. These attributes let you create more interesting layouts. For example, you might create stacks of cells by placing the views on top of one another and changing their z-ordering, or you might use a transform to rotate them on any axis.
For detailed information about how a layout object fulfills its responsibilities to the collection view, see Creating Custom Layouts.
Collection Views Initiate Animations Automatically (集合视图启动自动动画)
Collection views build in support for animations at a fundamental level. When you insert (or delete) items or sections, the collection view automatically animates any views impacted by the change. For example, when you insert an item, items after the insertion point are usually shifted to make room for the new item. The collection view can create these animations because it detects the current position of items and can calculate their final positions after the insertion takes place. Thus, it can animate each item from its initial position to its final position.
In addition to animating insertions, deletions, and move operations, you can invalidate the layout at any time and force it to recalculate its layout attributes. Invalidating the layout does not animate items directly; when you invalidate the layout, the collection view displays the items in their newly calculated positions without animating them. Instead in a custom layout, you might use this behavior to position cells at regular intervals and create an animated effect.
集合视图对动画有基本水平的支持。当你插入(或者删除)元素或者节的时候,集合根据视图的改变发生自动动画。比如呢,当你插入一个元素的时候,插入点后面的元素通常移动到新元素的位置。集合视图可以生成动画,因为可以通过查询现在元素的位置计算出插入后的最终位置。这样呢它就可以展示元素从初始位置到最终位置之间的动画了。