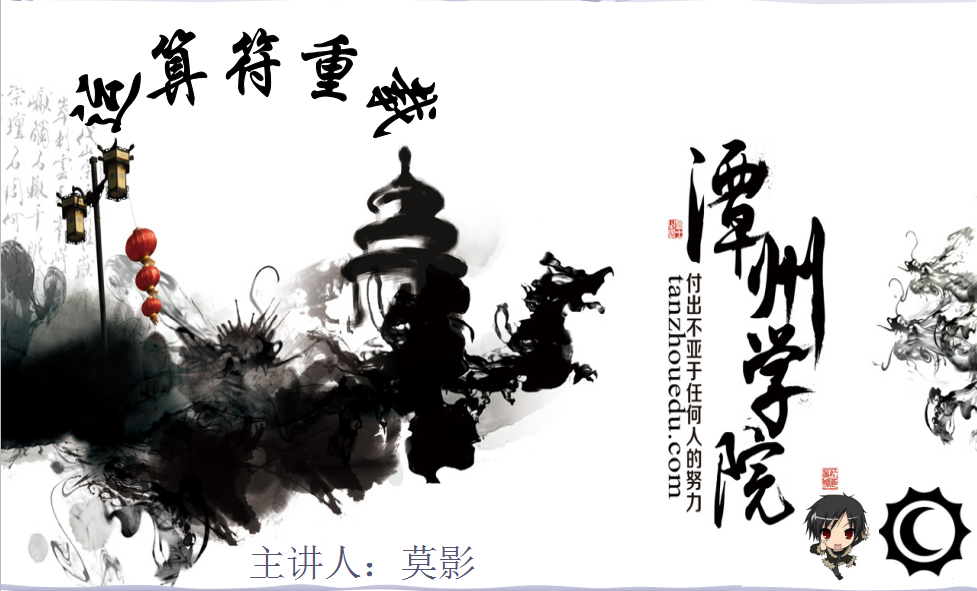
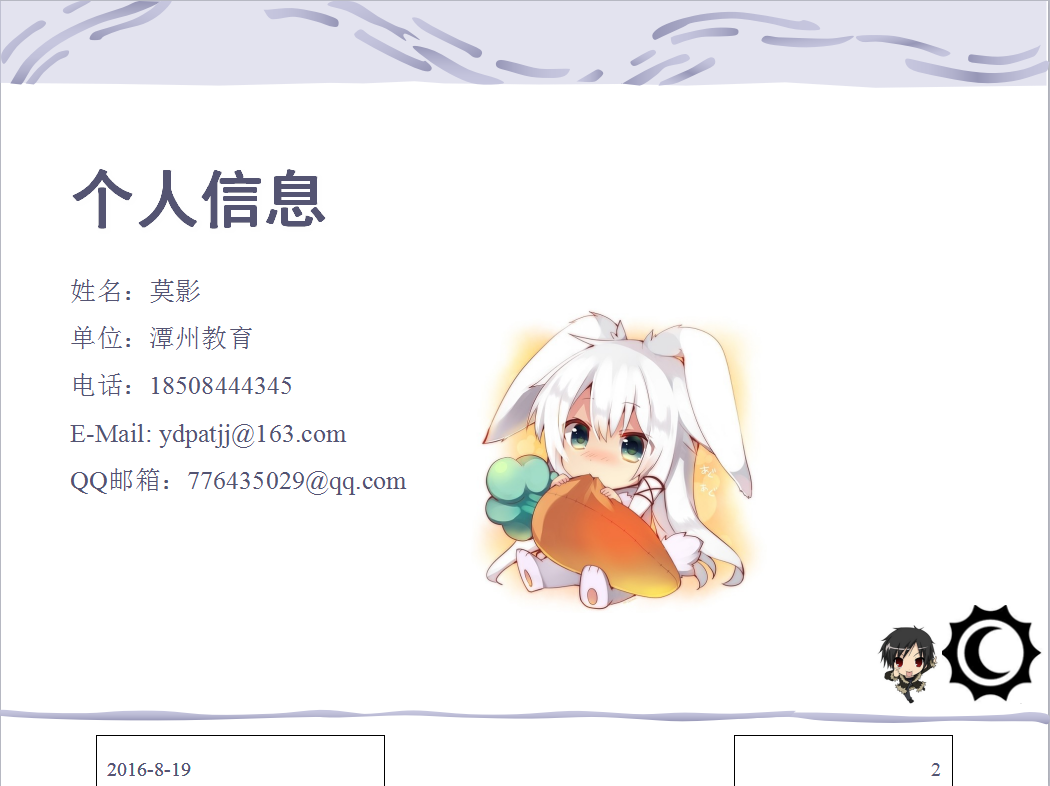
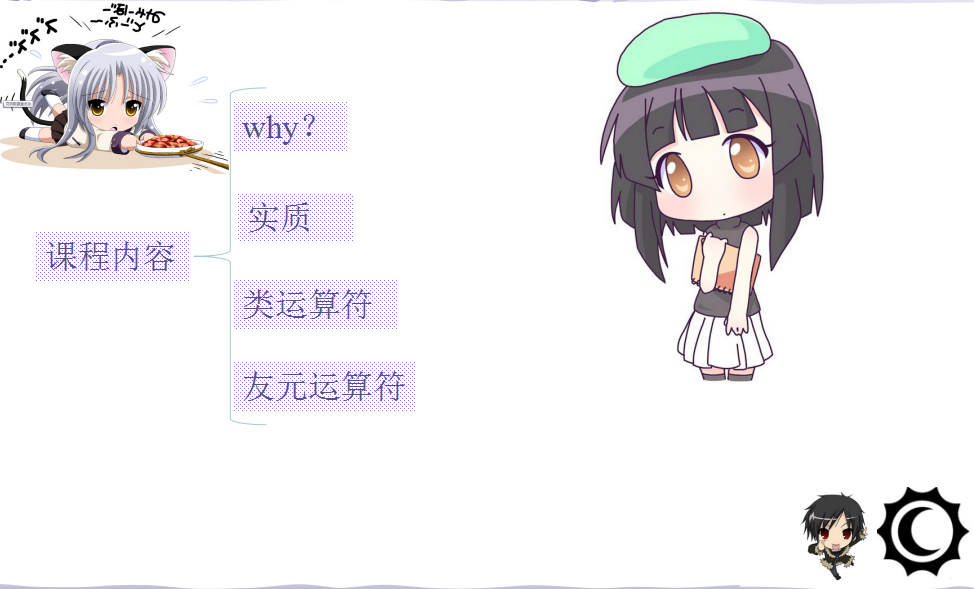
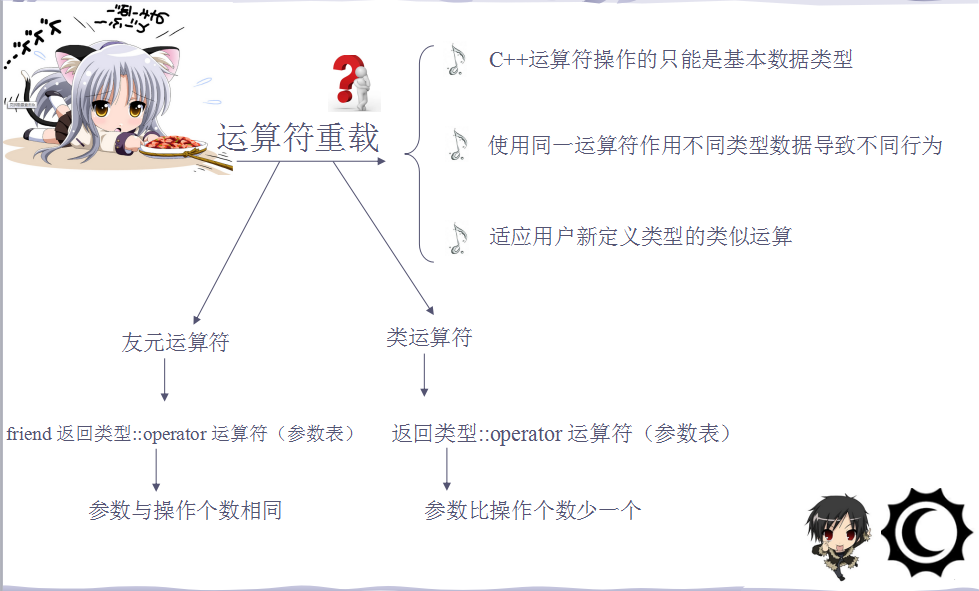
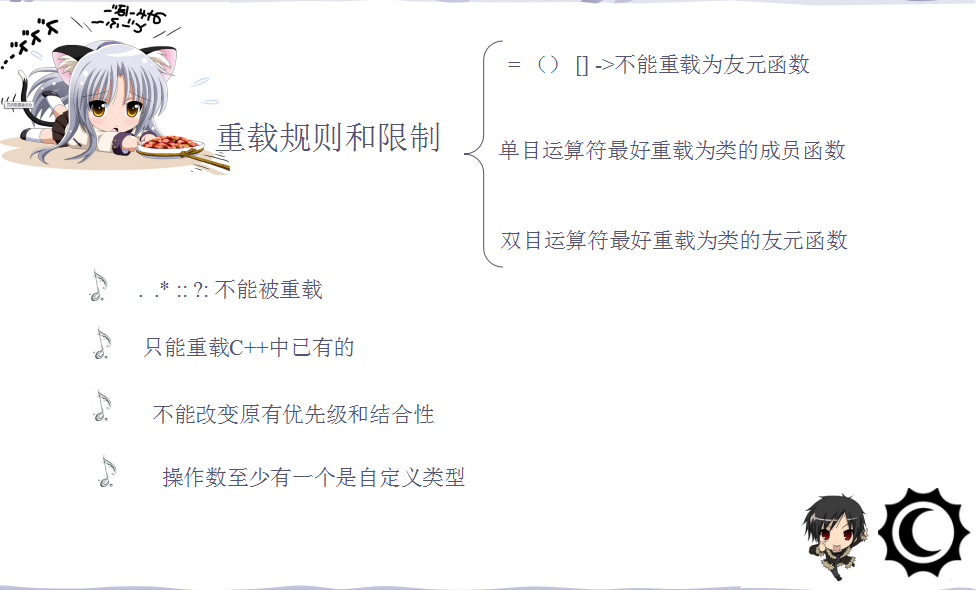
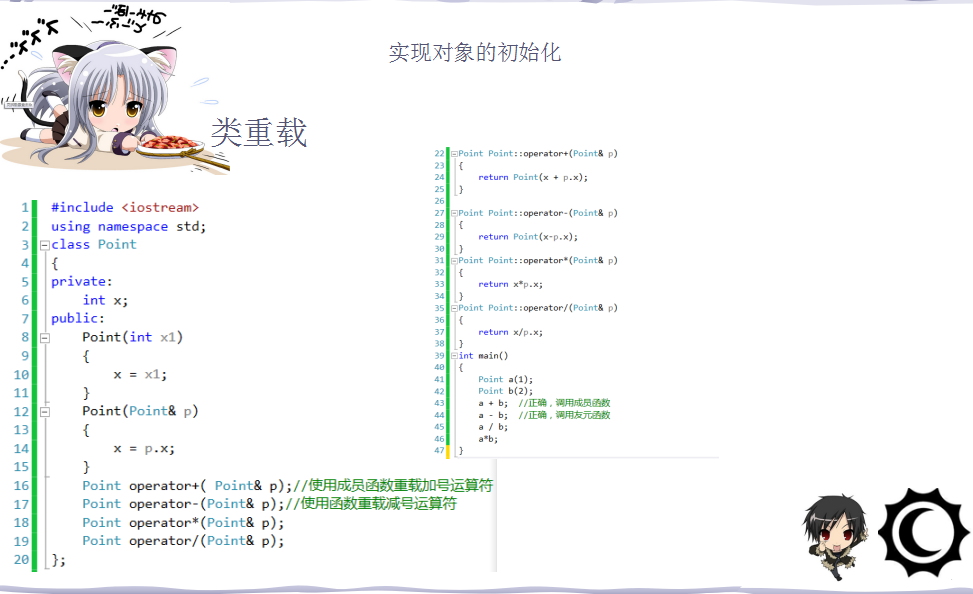
#include<iostream>
using namespace std;
//重载函数是类的成员函数 ,形参个数=操作数-1;
class Point
{
public:
Point(int x=0, int y=0):x(x), y(y){}
//x1.show(); //x 和y 1.2
void show()
{
cout << "x=" << x << endl << "y=" << y << endl;
}
//重载的过程就是调用函数的的过程
//object1+object2;
//object1.operator+(object2);
// this->x a.x
//函数返回值 operator+ :函数名 (参数)
Point operator+(const Point &a)
{
Point object;
//x1.operator+(x2);
//x+a.x
object.x = this->x+a.x;
object.y = this->y+a.y;
return object;
}
Point operator-(const Point &a){}
protected:
int x;
int y;
};
int main()
{
Point x1(1, 2);
Point x2(3, 4);
Point x3 = x1 + x2;
//对象.函数名(实参)
//x1.operator+(x2); x???
x1.show(); //x 和y 1.2
return 0;
}
//基本数据类型不需要重载运算
//对类来说,重载:实现对象与对象之间的运算
#include<iostream>
using namespace std;
class Age
{
public:
//构造函数+初始化参数列表
Age(int num = 0, int age = 0) :age(age), num(num){}
//友元重载函数的声明: friend 函数返回值 函数名(参数)
// 函数名: operator加上运算符组成函数名
//友元重载的参数个数=操作数
//双目运算符
friend Age operator*(const Age &a,const Age &b);
friend Age operator-(const Age &a, const Age &b);
//有一些运算符必须使用成员函数的方式
friend Age operator-(const Age& a);
void show()
{
cout << "num=:" << num << endl;
cout << "age=:" << age << endl;
}
protected:
int num;
int age;
};
Age operator*(const Age &a, const Age &b)
{
//重载函数的函数体,就是用打包,复杂运算符实现
Age c;
c.num = a.num*b.num;
c.age = a.age*b.age;
return c;
}
//友元重载操作数等于参数个数
//单目运算符
Age operator-(const Age &a)
{
Age b;
b.num = -a.num;
b.age = -a.age;
return b;
}
//重载可以实现对象和对象之间的运算
//对象加对象有什么用???
//复数 1+2i
int main()
{
Age a;
Age b(1, 2);
Age c(3, 4);
a = b*c; //a.num=b.num+c.num a.age=b.age+c.age
a.show();
Age d = -a;
d.show();
system("pause");
return 0;
}
#include<iostream>
#include <string>
using namespace std;
class student
{
public:
student(int age = 0, int num = 0) :age(age), num(num){}
// cout ----ostream 输出流对象
// cin ----istream 输入流对象
// operator<< 函数名()
friend ostream& operator<<(ostream& out, const student& mystudent);
friend istream& operator>>(istream& put,student& mystudent);
protected:
int age;
int num;
};
//返回值一定是返回引用
//<< 连续操作
//cout<<a<<b<<endl
ostream& operator<<(ostream& out, const student& mystudent)
{
//out
out <<"\tnum=:"<< mystudent.num <<"\tage=:"<< mystudent.age << endl;
return out;
}
istream& operator>>(istream &put, student& mystudent)
{
cout << "请输入编号和年龄" << endl;
put >> mystudent.num >> mystudent.age;
return put;
}
int main()
{
student mystudent(16, 1);
//cout对象<<自定义对象
cout << mystudent;
//请输入学生年龄和姓名
student mystudent2;
cin >> mystudent2;
cout << mystudent2;
string str("Anonymous");
cout << str << endl;
system("pause");
return 0;
}
#include<iostream>
#include <string>
using namespace std;
class student
{
public:
student(int age = 0, int num = 0) :age(age), num(num){}
// cout ----ostream 输出流对象
// cin ----istream 输入流对象
// operator<< 函数名()
void outPut(ostream &out) //注意函数类型
{
out << "\tnum=:" << num << "\tage=:" <<age << endl;
}
void Input(istream &put)
{
cout << "请输入编号和年龄" << endl;
put >> num >> age;
}
friend ostream& operator<<(ostream& out, student& mystudent);
friend istream& operator>>(istream& put, student& mystudent);
protected:
int age;
int num;
};
//返回值一定是返回引用
//<< 连续操作
//cout<<a<<b<<endl
ostream& operator<<(ostream& out, student& mystudent)
{
//out
mystudent.outPut(out);
return out;
}
istream& operator>>(istream &put, student& mystudent)
{
mystudent.Input(put);
return put;
}
int main()
{
student mystudent(16, 1);
//cout对象<<自定义对象
cout << mystudent;
//请输入学生年龄和姓名
student mystudent2;
cin >> mystudent2;
cout << mystudent2;
string str("Anonymous");
cout << str << endl;
system("pause");
return 0;
}
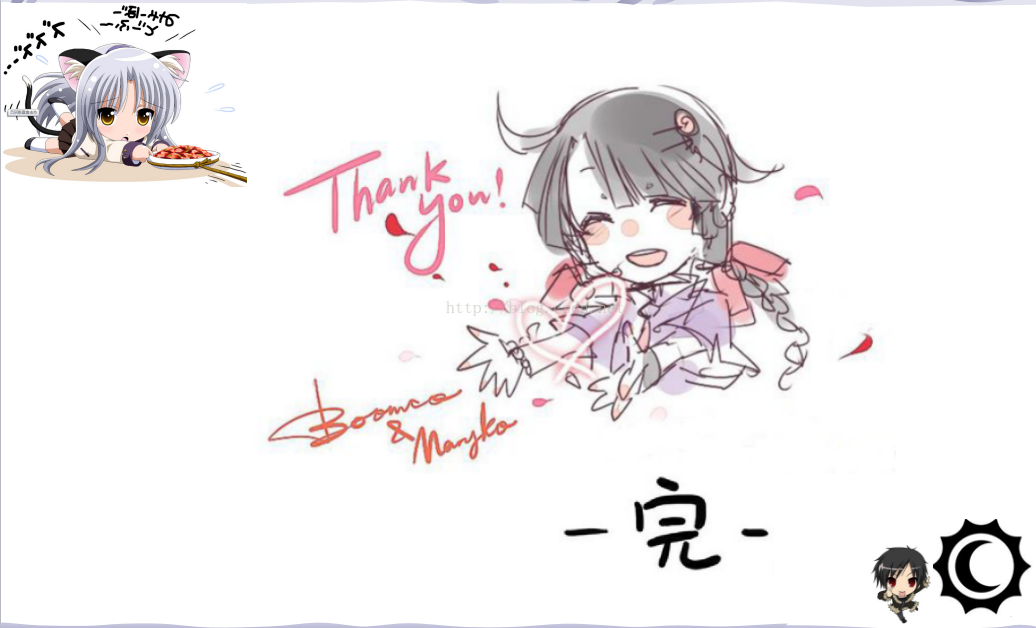