Dima is a beginner programmer. During his working process, he regularly has to repeat the following operation again and again: to remove every second element from the array. One day he has been bored with easy solutions of this problem, and he has come up with the following extravagant algorithm.
Let's consider that initially array contains n numbers from 1 to n and the number i is located in the cell with the index 2i - 1 (Indices are numbered starting from one) and other cells of the array are empty. Each step Dima selects a non-empty array cell with the maximum index and moves the number written in it to the nearest empty cell to the left of the selected one. The process continues until all nnumbers will appear in the first n cells of the array. For example if n = 4, the array is changing as follows:
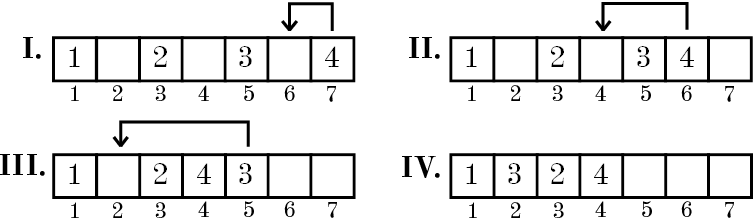
You have to write a program that allows you to determine what number will be in the cell with index x (1 ≤ x ≤ n) after Dima's algorithm finishes.
The first line contains two integers n and q (1 ≤ n ≤ 1018, 1 ≤ q ≤ 200 000), the number of elements in the array and the number of queries for which it is needed to find the answer.
Next q lines contain integers xi (1 ≤ xi ≤ n), the indices of cells for which it is necessary to output their content after Dima's algorithm finishes.
For each of q queries output one integer number, the value that will appear in the corresponding array cell after Dima's algorithm finishes.
4 3 2 3 4
3 2 4
13 4 10 5 4 8
13 3 8 9
The first example is shown in the picture.
In the second example the final array is [1, 12, 2, 8, 3, 11, 4, 9, 5, 13, 6, 10, 7].
题意:现在给你一个n,表示有2*n-1个方格,第奇数方格上会有一个数字 1-n按顺序放。第偶数个方格上是没有数字的。变动规则是排在最后一个位置的数字,移动到它前边最近的空位 。 直到数字之间没有空位。最终的数列是由n已经确定的。给你q,表示q次查询,每次查询输入一个x,问数列第x位上的数字是多少?
思路:由于数列是固定的,由n确定,并且n非常大,所以肯定是个规律题。所以就打表找规律。(1<=n<=20)表格如下:
#include<cstdio>//打表代码 #include<cstring> using namespace std; int a[100000]; int main() { for(int n=1; n<=20; n++) { printf("%2d: ",n); memset(a,0,sizeof a); for(int i=1; i<=n; i++) a[2*i-1]=i; int w=2*n-2,num=2*n-1; while(w) { while(!a[num]) num--; a[w]=a[num--]; w-=2; } for(int i=1; i<=n; i++) printf(" %d",a[i]); printf("\n"); } }
1: 1
2: 1 2
3: 1 3 2
4: 1 3 2 4
5: 1 5 2 4 3
6: 1 4 2 6 3 5
7: 1 6 2 5 3 7 4
8: 1 5 2 7 3 6 4 8
9: 1 9 2 6 3 8 4 7 5
10: 1 6 2 10 3 7 4 9 5 8
11: 1 9 2 7 3 11 4 8 5 10 6
12: 1 7 2 10 3 8 4 12 5 9 6 11
13: 1 12 2 8 3 11 4 9 5 13 6 10 7
14: 1 8 2 13 3 9 4 12 5 10 6 14 7 11
15: 1 12 2 9 3 14 4 10 5 13 6 11 7 15 8
16: 1 9 2 13 3 10 4 15 5 11 6 14 7 12 8 16
17: 1 17 2 10 3 14 4 11 5 16 6 12 7 15 8 13 9
18: 1 10 2 18 3 11 4 15 5 12 6 17 7 13 8 16 9 14
19: 1 15 2 11 3 19 4 12 5 16 6 13 7 18 8 14 9 17 10
20: 1 11 2 16 3 12 4 20 5 13 6 17 7 14 8 19 9 15 10 18
规律1:细心观察会发现,上面的12,13行标记的数字下面是上面+1的结果。并且12行的11,加一之后变成12在13行是在第二个。以上规律是所有相邻行共有的。
规律2:所有行的第奇数个位置的数字是确定的。对应关系是x — 2*x-1;
问题是现在给你一个位置x,让你计算出这个位置上的数字ans;现在由第n行开始向上反推:
如果x为奇数,那么答案很明显ans=x/2+1;
如果x为偶数,n行的第x个数字是ans,那么请你思考一下 ans-1这个数字在n-1行的哪个位置,很明显是在x-2这个位置。但是有种特殊情况:如果x是等于2的话,x-2就为零了,现在的问题是n行的第2个数字是ans,那么请你思考一下 ans-1这个数字在n-1行的哪个位置, 不难看出ans-1是在n-1行的第n-1个位置。如果这样一步步把层数向上推,那么这个位置是会不断地变化的,由规律2可以知道,奇数位置的数字是确定的,如果我们向上推了m层,并且第n-m层这个数字是在奇数位置s,那么我们就能知道这个奇数位置s的数字是多少,每次向上推一层,数字减小1, ans=(s/2+1)+m,(s表示奇数位置,m表示n层向上推了m层)
举个栗子:13层的第4个位置是多少? 开始向上推:13层的第4个位置-->12层的第2个位置-->11层的第11个位置,因为11为奇数,所以能算出是数字6,然后反推回去就是+1+1,及13层是8;
再举个栗子:13层的第10个位置是多少? 开始向上推:13层的第10个位置-->12层的第8个位置-->11层的第6个位置-->10层的第4个位置-->9层的第2个位置-->8层的第8个位置-->7层的第6个位置-->6层的第4个位置-->5层的第2个位置-->4层的第4个位置-->3层的第2个位置-->2层的第2个位置-->1层的第1个位置, 位置为奇数,确定数字为1/2+1,反推回去到第13层,及+12,所以第13层为1+12=13;
大家应该看明白了,就是向上推,位置为2时向上推的话,位置和层数是一样的。并且每次减2,偶数位置还会是偶数位置,干脆一次性把偶数减完,: 13层的第10个位置-->8层的第8个位置-->4层的第4个位置-->2层的第2个位置-->1层的第1个位置
8=13-10/2;4=8-8/2;2=4-4/2;
a层的第b个位置 -->c 层的第d个位置 (d为奇数然后反推即可)
d=c=a-b/2. (b为偶数),到这里整道题目就水落石出了。
#include<cstdio> #include<cstring> #define LL long long using namespace std; int main() { int p; LL n,m,a; scanf("%lld%d",&n,&p); while(p--) { scanf("%lld",&a); if(a%2==1) { printf("%lld\n",a/2+1); continue; } m=n; while(a%2==0) { a=m-a/2; m=a; } printf("%lld\n",n-a+(a+1)/2); } }