异常一:Error creating bean with name 'multipartResolver': Failed to introspect bean class
解决办法:在pom.xml添加依赖
<dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.3.1</version> </dependency>
异常二:MissingServletRequestParameterException:Required: CommonsMultipartFile parameter 'file' is not present
在SpringMVC-Servlet.xml里面增加一个bean字段
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <!-- 设置上传文件的最大尺寸为10MB 10*1024*1024 --> <property name="maxUploadSize"> <value>10485760</value> </property> </bean>
控制器的方法里要加上@RequestParam(Value="")
public String upload(@RequestParam(value = "file",required = false) CommonsMultipartFile file, HttpSession session)
接着提供七牛云的工具类:
package com.zy.ninepalaceappapi.util; import com.google.gson.Gson; import com.qiniu.common.QiniuException; import com.qiniu.common.Zone; import com.qiniu.http.Response; import com.qiniu.storage.BucketManager; import com.qiniu.storage.Configuration; import com.qiniu.storage.UploadManager; import com.qiniu.storage.model.DefaultPutRet; import com.qiniu.util.Auth; import java.io.File; public class QiniuUploadImageUtil { //accessKey private static String accessKey = "████████████████████████████████████";//用钥匙屏幕得字符串 //secretKey private static String secretKey ="██████████████████████████████████████████"; //储存空间名 private static String bucket ="██████████████████████████████████████████"; //外链URL前缀 private static String fronturl = "████████████████████████████████████"; /** * 图片上传 * @param file 图片路径 * @return 链接 * @throws QiniuException */ public static String fileUpload(String file)throws QiniuException { Auth auth = Auth.create(accessKey,secretKey); String upToken = auth.uploadToken(bucket); //上传凭证 Configuration cfg = new Configuration(Zone.autoZone()); UploadManager uploadManager = new UploadManager(cfg); String key = null; try { Response response = uploadManager.put(file, key, upToken); DefaultPutRet putRet = new Gson().fromJson(response.bodyString(),DefaultPutRet.class); return fronturl+putRet.key; }catch (QiniuException ex){ Response r = ex.response; System.err.println(r.toString()); try { System.err.println(r.bodyString()); } catch (QiniuException ex2) { // ignore } } return null; } /** *删除图片 * @param key * @return * @throws QiniuException */ public static String delete(String key)throws QiniuException{ Auth auth = Auth.create(accessKey,secretKey); Configuration cfg = new Configuration(Zone.zone2()); BucketManager bucketMgr = new BucketManager(auth, cfg); try { bucketMgr.delete(bucket,key); }catch (QiniuException Q){ return Q.toString(); } System.out.println("success"); return null; } }
接着控制器的方法:
步骤:
扫描二维码关注公众号,回复:
1914931 查看本文章
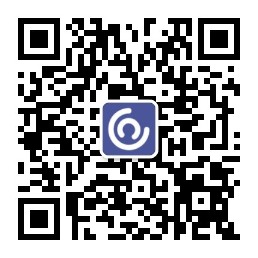
1.由于七牛云工具类的方法是获取图片的本地路径,所以需要一个中转,因此请求参数是MultipartFile类型
2.从前端拿到file对象后先临时储存到项目本地再获取路径
3.调用七牛工具类的上传图片方法传入图片路径
4.最后上传完了就删除本地的那张图片
@ResponseBody @RequestMapping("uploadImage") public String upload(@RequestParam(value = "file",required = false) CommonsMultipartFile file, HttpSession session)throws QiniuException{ try { String path = session.getServletContext().getRealPath("/")+file.getOriginalFilename(); System.out.println(path); file.transferTo(new File(path)); String url =QiniuUploadImageUtil.fileUpload(path); File f = new File(path); f.delete(); return url; }catch (Exception e){ return e.toString(); } }
最后写 一个前端html来上传图片:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<input type="file" onchange="aaa()" name="">
<img src="https://afp.alicdn.com/afp-creative/creative/u124884735/55308755880f8b637dde1f88b84ea9da.png" onclick="ButtonClick(this)">
<script type="text/javascript">
function aaa(e) {
let a = document.getElementsByTagName('input')[0].files[0]
let b = document.getElementsByTagName('input')[0].value
let c = new FormData()
c.append('file',a)
let ajax = new XMLHttpRequest()
ajax.open('POST','http://192.168.0.119:8080/shop/uploadImage',true)
ajax.send(c)
}
function ButtonClick(div)
{
// var div = document.getElementById('divId');
div.contentEditable = 'true';
var controlRange;
if (document.body.createControlRange) {
controlRange = document.body.createControlRange();
controlRange.addElement(div);
controlRange.execCommand('Copy');
}
div.contentEditable = 'false';
}
</script>
</body>
</html>
最后测试。
上传图片,返回了一个URL
打开链接:
最后附上七牛云的依赖(我也是拷过来的):
<dependency> <groupId>com.qiniu</groupId> <artifactId>qiniu-java-sdk</artifactId> <version>7.2.6</version> <scope>compile</scope> </dependency> <dependency> <groupId>com.squareup.okhttp3</groupId> <artifactId>okhttp</artifactId> <version>3.3.1</version> <scope>compile</scope> </dependency> <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.6.2</version> <scope>compile</scope> </dependency> <dependency> <groupId>com.qiniu</groupId> <artifactId>happy-dns-java</artifactId> <version>0.1.4</version> <scope>compile</scope> </dependency>
异常部分参考教程文章 :点击打开链接