Given a string, find the length of the longest substring without repeating characters.
Examples:
Given "abcabcbb"
, the answer is "abc"
, which the length is 3.
Given "bbbbb"
, the answer is "b"
, with the length of 1.
Given "pwwkew"
, the answer is "wke"
, with the length of 3. Note that the answer must be a substring, "pwke"
is a subsequence and not a substring.
题意:找到字符串中的一个子串,该子串是无重复的最长子串。
思路:最大滑窗算法
左指针指向不重复子串的第一个字符,右指针指向要检测的字符。
如果要检测的字符在【左指针,右指针),滑窗左指针应该移动到和检测字符重复字符的右边一位。
不在那个范围,证明左指针不需要更新,因为检测字符和左侧的字符都没有重复
扫描二维码关注公众号,回复:
1854881 查看本文章
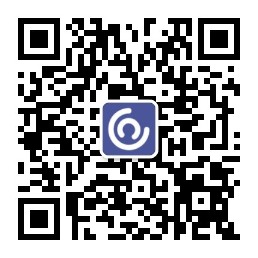
此时,滑窗不存在重复字符,所有右指针右移,继续指向下一个要检测的字符
说明:每次检测完一个字符后,才能将这个字符对应到哈希表。
class Solution {
public:
int lengthOfLongestSubstring(string s) {
int len = s.length();
if( len == 0 )
return 0;
if( len == 1 )
return 1;
int ret = 1;
int left = 0, right = 1;
map<char, int> m;
m[s[left]] = 0;
while( right < len )
{
if( m.find(s[right]) != m.end() && (m[s[right]] >= left) )
{
left = m[s[right]] + 1;
}
m[s[right]] = right++;
ret = (ret > (right-left)) ? ret : (right-left);
}
return ret;
}
};