hashCode()和equals()都是java.lang.Object类自带的函数,为了更好的测试这两个函数,我们先写一个Student类
public class Student {
String name;
int age;
public Student(String name, int age){
this.name = name;
this.age = age;
}
}
一、hashCode()方法
我们接着写一个Student测试类,即StudentDemo类
public class StudentDemo{
public static void main(String[] args){
Student stu1 = new Student("lhy",18);
Student stu2 = new Student("lhy",18);
System.out.println(stu1.hashCode()); //725223732
System.out.println(stu2.hashCode()); //1865566999
}
}
hashCode()返回对象的哈希值,这个哈希值是通过哈希算法,将对象的地址值转换成一个整数得到的。也就是说一般一个地址值对应于一个哈希值,我们可以理解为hashCode()取出了对象的地址。
二、”==”的用法
同样在StudentDemo类中进行测试
public class StudentDemo{
public static void main(String[] args){
int a = 3;
int b = 4
int c = 3;
Student stu1 = new Student("lhy",18);
Student stu2 = new Student("lhy",18);
System.out.println(stu1 == stu2); // false
System.out.println(a == b); // false
System.out.println(a == c); // true
}
}
“==”的作用:
1、当比较基本类型时,比较的是值是否相同
2、当比较引用类型时,比较的是对象的地址值是否相同
三、equals()方法
equals()方法继承于Object类,源码为
public boolean equals(Object obj) {
return (this == obj);
}
我们可以看到,equals()方法使用“==”对两个对象进行比较的,而刚刚我们分析了“==”对于引用类型比较的是其地址值是否相同,所以,equals()方法默认比较的是两个对象的地址值是否相同,测试如下:
public class StudentDemo{
public static void main(String[] args){
Student stu1 = new Student("lhy",18);
Student stu2 = new Student("lhy",18);
Student stu3 = stu1;
System.out.println(stu1.equals(stu2)); //false
System.out.println(stu1.equals(stu3)); //true
}
}
因为stu1和stu2都是new出来的,他们存在于不同的内存地址中,所以地址是不同的,返回false;而stu3和stu1指向内存中的同一地址,所以返回true。
重写equals()方法
一般我们不会比较两个对象的地址是否相同,我们需要比较的是成员变量是否相同,所以我们需要重写equals()方法,在Student类中重写如下:
public class Student {
String name;
int age;
public Student(String name, int age){
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
Student s = (Student) obj; //向下转型
if(this.name.equals(s.name) && this.age == s.age){
return true;
}else {
return false;
}
}
}
是否有人问,为什么重写equals()方法时,还使用了equals()方法??因为this.name返回的是一个String对象,我们调用的是String类中的equals()方法,而String类中重写了equals()方法(比较两个字符串的值是否相同),所以我们可以借助String类的equals()方法来进行比较String类型(引用类型)的name是否相同,而age是基本类型,直接通过“==”进行比较就ok了。
测试如下:
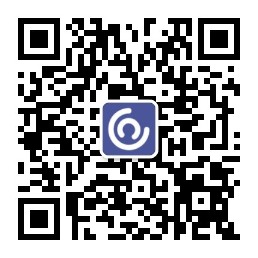
public class StudentDemo{
public static void main(String[] args){
Student stu1 = new Student("lhy",18);
Student stu2 = new Student("lhy",18);
Student stu3 = stu1;
Student stu4 = new Student("xs",24);
System.out.println(stu1.equals(stu2)); //true
System.out.println(stu1.equals(stu3)); //true
System.out.println(stu1.equals(stu4)); //false
}
}