在Android中,很多应用都需要存储一些参数,例如在天气app中,在某一次使用时用户添加了若干个城市,当用户下一次点开时也希望之前设置的城市会保存在手机中,以方便直接获取信息。这个时候就需要用到 SharedPreference 类的辅助,利用它存储一些键值对(Key-Value)参数。SharedPreference类时Android提供的一个轻量级的存储类,特别适用于存储软件的各项参数。
具体步骤:
1.获取SharedPreference的对象,通过getSharedPreference(String,int)方法。第一个参数用于指定该存储文件的名称,不用加后缀,第二个参数指定文件的操作模式。一般用MODE_PRIVATE 私有方式存储,其他应用无法访问。
2.设置参数,必须通过一个SharedPreference.Editor对象。存储键值对。只能存放Boolean,Float,Int,Long,String 五种类型。editor.putXxx("key","value")。
3.通过editor.commit()提交数据。也可以通过clean(),remove()清除。
4.数据存储在Android系统的 /data/data/"app package name"/shared_prefs 目录下的一个.xml文件。
简单例子实现SharedPreference存储用户信息的代码:
package com.engineer.shizhibin.sharedprefs;
import android.content.SharedPreferences;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private SharedPreferences mSpf;
private EditText in;
private Button write;
private Button read;
private TextView out;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
in = (EditText) this.findViewById(R.id.intext);
write = (Button) this.findViewById(R.id.input);
read = (Button) this.findViewById(R.id.output);
out = (TextView) this.findViewById(R.id.showtext);
mSpf = super.getSharedPreferences("test",MODE_PRIVATE);
}
public void writeInfo(View view) {
SharedPreferences.Editor editor = mSpf.edit();
editor.putString("name",in.getText().toString());
editor.commit();
}
public void readInfo(View view) {
String info = mSpf.getString("name","");
out.setText(info);
}
}
布局文件:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="com.engineer.shizhibin.sharedprefs.MainActivity">
<EditText
android:layout_marginTop="20dp"
android:id="@+id/intext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:layout_marginTop="20dp">
<Button
android:id="@+id/input"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="存储"
android:onClick="writeInfo"/>
<Button
android:id="@+id/output"
android:layout_marginLeft="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读取"
android:onClick="readInfo"/>
</LinearLayout>
<TextView
android:id="@+id/showtext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_marginLeft="15dp"
android:text="存储的信息"
android:textSize="25dp"/>
</LinearLayout>
运行结果:
1.输入信息:
扫描二维码关注公众号,回复:
1817202 查看本文章
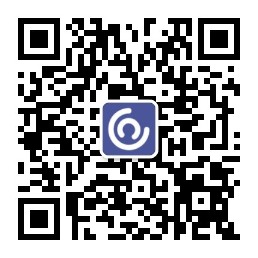
2.读取信息: