目录
7、删除所有元组(表中所有行的数据)(delete from)
扫描二维码关注公众号,回复:
17370433 查看本文章
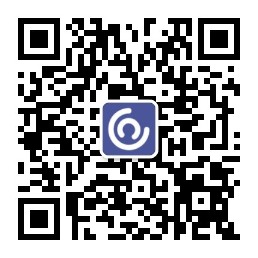
注:本文仅写了一些常用的SQL代码,一些不常用的并未列出。其次为了使代码框中的文字更加清晰,代码框中的文字并没有使用注释符进行注释。
1、基本数据类型
char(n):固定长度为n的字符串;
varchar(n):可变长度的字符串,最大为n;
int:整数类型;
smallint:小整数类型;
numeric(m,n):定点数,共m位,有n位处于小数点右边;
例如 numeric(3,1),符合条件的数据:31.4
float(n):精度为n的浮点数
real,double precision:浮点数与双精度浮点数
2、创建数据库关系(create)
这里以创建一个学生关系为例使用
create table 关系名();
进行创建,()里面包含:属性+数据类型
create table Student
(
Sno char(5),
Sname varchar(20),
Sage smallint,
Ssex char(2),
Sdept char(2));
3、主键声明(primary key)
使用
primary key(属性)
进行主键声明
例如,对以下创建声明Sno为Student的主键
create table Student
(
Sno char(5),
Sname varchar(20),
Sage smallint,
Ssex char(2),
Sdept char(2)),
primary key(Sno));
4、外键声明(foreign key)
声明外键的代码为:
foreign key 属性 references 关系(属性);
声明外键关系表示,两个属性的取值必须对应。
具体声明代码如下示例
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint,
Ssex char(2),
Sdept char(2),
primary key(Sno)); --主键可以在最后设置
create table Course
(
Cno char(2) primary key, --主键也可以采用这种方法
Cname char(20),
Cpno char(2),
Ccredit smallint,
create table SC
(
Sno char(5),
Cno char(2),
Grade smallint,
foreign key (Sno) references Student(Sno),
这里声明了SC关系中的Sno作为外键,
被参考对象为Student关系中的Sno属性,
其中Student(Sno)也可写成Student
foreign key (Cno) references Course);
这里声明了SC关系中的Cno作为外键,
被参考对象为Course关系中的Cno属性,
两个关系中都有Cno,可省略括号
5、约束语句之不允许为空值约束(not null)
不为空约束通常就在创建关系,定义属性的同时进行约束
采用 not null,示例如下
create table student
(
st_id varchar(9) not null,
st_nm varchar(8) not null,
st_sex varchar(2),
st_birth varchar(4),
primary key(st_id));
6、关系中添加(插入)数据(insert into)
在关系中添加一个元组采用
insert into 关系名 values(属性对应的值)
具体示例如下
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint check(Sage>=15 and Sage<=45),
Ssex char(2),
Sdept char(2),
primary key(Sno));
insert into Student values('17001','钱横',18,'男','CS');
insert into Student values('17002','王林',19,'女','CS');
insert into Student values('17003','李民',20,'男','IS');
insert into Student values('17004','赵三',16,'女','MA');
这里添加了四条student关系表的元组信息
7、删除所有元组(表中所有行的数据)(delete from)
如果要删除一个关系表中的所有信息,则可以采用delete语句
delete from student;
表示删除student关系表中的所有信息,
但会保留关系student,
即还可以往里面添加信息。
8、删除一个关系(数据表)(drop table)
如果要删除整个关系表里的所有信息以及对应关系表本身
则采用drop语句,例如
drop table student;
9、为已有关系增加属性(alter)
为一个关系表添加属性:
alter table 关系表名 add 属性 对应的域
具体示例如下
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint,
Sdept char(2),
primary key(Sno)); --主键可以在最后设置
我们要为student关系添加一个Ssex属性为char(2)类型
alter table student add Ssex char(2);
10、从关系中删除属性(alter)
为一个关系表删除属性:
alter table 关系表名 drop 属性
具体示例如下
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint,
Sdept char(2),
primary key(Sno)); --主键可以在最后设置
我们要删除student关系中的Sage属性
alter table student drop Sage;
要注意,对于删除属性这个操作,
在很多数据库系统里面是不支持的
11、查询语句之去重(默认不去重)(distinct)
最简单的查询语句
select 需要查询的属性名
from 关系表名
有时候我们想要强行删除重复的项,
则在select后面加上distinct去重,例如
select distinct name
from student
表示查询student关系下,name属性集,且去除重复项
12、查询语句之自然连接(natural join)
有时候,我们需要根据某些属性,
将两个表联系起来进行查询,例如以下
select name,course_id
from instructer,teacher
where instructer.id = teacher.id;
则该查询可以用更简洁的自然连接表示
select name,course_id
from instructer natural join teacher;
以此类推,也可以进行多个表的自然连接
select name,title
from student natural join course natural join teacher natural join instructer;
13、更名运算(as)
重命名的原因一个是把长的关系名替换成短的,
另一个原因则是为了比较同一个关系中的元组。
重命名采用as语句,例如
select course_id as '课程号' --将course_id属性重命名为课程号
from course
select T.name
from instructer as T,instructer as S
where T.salary > S.salary and S.detp_name = 'math'
该语句的作用是找出那些工资至少比数学系某一个工资要高的教师姓名。
14、字符串运算之 %
百分号一般常用于查询语句中
where子句进行字符串匹配,直接举例说明
Intro%
表示匹配任何以Intro打头的字符串
15、字符串运算之__
下划线匹配固定数目字符串
例如
_ _ _ 三个下划线表示匹配只含三个字符的字符串
_ _ _ % 表示匹配至少含三个字符的字符串
16、字符串运算之escape
escape 常用于声明转义字符
直接上例子
like 'ab\%cd%' escape '\';
匹配所有以'ab%cd'开头的字符串
上述代码利用escape语句声明了\为转义字符,
则字符串中的\后面的%不再具有作用,只是普通的%
17、字符串运算之like比较运算
通常14、15、16、17会运用在一个查询语句中
通常匹配字符串的where语句为固定搭配
where……like……
例如
select dept_name
from department
where building like '%Wat\%son% escape '\';
表示匹配所有包含子串'Wat%som'的建筑名称
18、查询语句之排列顺序(order by)
order by 语句就是以某个属性进行排序,
通常有两种排序,升序(默认):asc,降序:desc
放在where语句的后面,例如:
select name
from student
where course = 'math'
order by name desc;
对名字进行降序排序
19、where子句之between
between……and……表示位于两者之间,通常用于where子句
例如
select name
from instructor
where salary between 9000 and 10000;
表示找出工资位于9000到10000之间的员工姓名;
20、集合运算交并差
并集采用union
交集采用intersect
差集采用except
(select course_id
from section
where smseter = 'Fall' and year = 2009)
union
(select course_id
from section
where smseter = 'Spring' and year = 2010)
表示找出2009年秋季或者在2010春季开课的所有课程号,交集和差集就不再例举
21、聚集函数之平均值(avg)
平均值计算聚集函数,格式为avg(属性)
例如
select avg(salary) as avg_salary
from instructor
where dept_name = 'math';
计算数学系的平均工资
22、聚集函数之最大值(max)
最大值输出聚集函数,格式为max(属性)
例如
select max(salary) as max_salary
from instructor
where dept_name = 'math';
找出数学系的最大工资
23、聚集函数之最小值(min)
最小值输出聚集函数,格式为min(属性)
例如
select min(salary) as min_salary
from instructor
where dept_name = 'math';
找出数学系的最低工资
24、聚集函数之总和(sum)
求和聚集函数,格式为sum(属性)
例如
select sum(salary) as sum_salary
from instructor
where dept_name = 'math';
计算数学系的所有员工总工资
25、聚集函数之计数(count)
统计计数聚集函数,格式为count(属性)
例如
select count(ID)
from instructor
where dept_name = 'math';
统计数学系的总人数
26、聚集函数之分组(group by)
group by语句就是以某个属性进行分组,
通常放在where语句的后面,例如:
select dept_name,avg(salary)
from instructor
group by dept_name;
按dept_name进行分组,然后计算每个系的平均工资
27、聚集函数之having语句
通过情况下,不仅用group by语句对某个属性进行分组,
而且需要对分组限定条件,常常在group后面运用having进行限定
例如
select dept_name,avg(salary)
from instructor
group by dept_name
having avg(salary) < 7000
找出员工平均工资低于7000的系的平均工资
28、查询语句之where嵌套
select distinct course_id
from section
where semster = 'Fall' and year = 2009 and
course_id not in (select course_id
from section
where semster = 'Spring' and year = 2010);
上述语句实现了找出在2009年秋季开课但不在2010年春季开课的课程号
上述语句就是一个嵌套语句,利用了 not in 将两个集合进行判断连接
29、where子句之“至少比某一个”(some)
至少比某一个大:> some
至少比某一个大于等于:>= some
类似的还有 < some , <= some , <>some 注意最后一个并不等价于not in
select name
from instructor
where salary > some (select salary
from instructor
where dept_name = 'math');
找出满足工资至少比数学系某一个教师工资要高的教师姓名
30、where子句之“比所有都”(all)
至少比某一个大:> all
至少比某一个大于等于:>= all
类似的还有 < all , <= all , <>all 注意最后一个等价于not in
select name
from instructor
where salary > all (select salary
from instructor
where dept_name = 'math');
找出满足工资比数学系所有教师工资要高的教师姓名
31、空关系测试(exists)
exists在作为参数的子查询非空时返回true值
例子
select course_id
from section as S
where semster = 'Fall' and year = 2009 and
exists (select *
from section as T
where semster = 'Spring' and year = 2010 and S.course_id = T.course_id);
表示找出2009年秋季开课和2010年春季同时开课的所有课程号
32、是否存在重复子查询(unique)
unique用法同exists类似,直接上例子
select T.course_id
from course as T
where unique (select R.course_id
from section as R
where T.course_id = R.course_id and R.year = 2009);
表示查找所有在2009年最多开设一次的课程
33、lateral语句
用处不大,具体用法可参考百度
34、with语句
with子句提供了定义临时关系的方法,
这个定义只对包含with子句的查询有效
例如
with max_budget(value) as(select max(budget) from department)
select budget
from department,max_budget
where department.budget = max_budget.value;
with 子句定义了临时关系max_budget,此关系在随后的查询中马上被使用了。
35、删除满足条件的属性或元组
此处还是运用delete语句只是将其与where语句搭配
例如
delete from student
where score between 60 and 80
表示删除score在60到80之间的学生
36、更新语句(update…set)
更新某个属性的值通常采用update……set……语句
具体示例如下:
update instructor
set salary = salary * 1.05
where salary < 7000;
表示给那些工资小于7000的人涨5%的工资
37、左外连接(left outer)
select *
from student natural left outer join takes;
38、右外连接(right outer)
select *
from student natural right outer join takes;
39、全外连接(full outer)
select *
from student natural full outer join takes;
40、内连接(inner)
内连接等价于自然连接
即 natural join 等价于 natural inner join
41、视图创建(view)
视图的创建语句为
create view 视图名 as <查询表达式>
例如以下语句
create view ST as
select id,name,dept_name
from Student
从student关系中选出id、name、dept_name属性创建一个名为ST的视图
42、约束语句之check
check语句为属性添加域即范围
check(条件)
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint check(Sage>=15 and Sage<=45),
Sage属性必须大于15,小于45
Ssex char(2),
primary key(Sno));
create table Course
(
Cno char(2) primary key,
Cname char(20),
semester char(20)
Cpno char(2),
Ccredit smallint,
foreign key (Cpno) references Course(Cno)
check(semester in ('Fall','Spring','Summer','Winter')));
check也可放在最后,这里表示semester属性只能是以上括号内的四个字符串
43、约束之默认值设置(default)
1、可以直接在创建关系时,直接添加默认值
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint defalut 20, --默认Sage为20
Ssex char(2),
Sdept char(2),
primary key(Sno));
2、可以在创建完后再添加默认值
create table Student
(
Sno char(5) ,
Sname char(20),
Sage smallint,
Ssex char(2),
Sdept char(2),
primary key(Sno));
alter table Student add default 20 for Sage;
添加Student关系中的Sage属性默认值为20
44、授予权限语句(grant)
授予权限代码如下
grant <权限列表>
on <关系名或视图名>
to <用户>
例如
grant select
on department
to Amit
45、收回权限语句(remoke)
收回权限语句如下
revoke <权限列表>
on <关系名或视图>
from <用户>
例如
revoke select
on department
from Aimt
家人们,考试前一天万字总结,ddl,求求了点个赞吧。