本专栏记录C++学习过程包括C++基础以及数据结构和算法,其中第一部分计划时间一个月,主要跟着黑马视频教程,学习路线如下,不定时更新,欢迎关注。
当前章节处于:
====>第1阶段-C++基础入门
---------第2阶段实战-通讯录管理系统,
---------第3阶段-C++核心编程,
---------第4阶段实战-基于多态的企业职工系统
---------第5阶段-C++提高编程
---------第6阶段实战-基于STL泛化编程的演讲比赛
---------第7阶段-C++实战项目机房预约管理系统
1.C++初识
编写一个C++程序的4个步骤
- 创建项目 (使用Visual C++进行创建)
- 创建文件 (在源文件中进行添加)
- 编写代码(最核心最耗时的部分)
- 运行程序(F5运行代码)
所有代码使用Visual C++进行编写,在编写时,需要先创建项目,再在源文件中添加cpp文件,在cpp文件中进行编写代码。
1.1 第一个C++程序
学习一门语言第一步是配置环境,第二步该是HelloWorld的,使用C++输出HelloWorld的代码如下:
#include <iostream>
using namespace std;
int main() {
cout << "HelloWorld" << endl;
system("pause");
return 0;
}
1.2 C++注释
- 单行注释: //
- 多行注释: /* */
1.3 变量
给一段指定的内存空间起名,方便操作这段内存。
定义语法:
数据类型 变量名=变量初始值
int a =0;
1.4 常量
用于记录程序中不可改变的数据
两种定义的方式:
- #define 宏常量 注意不能在后面添加分号
定义格式
: #define 变量名 变量值 - const 修饰的变量
定义格式
: const 变量名=变量值;
#include <iostream>
using namespace std;
// 定义一个宏常量
#define Day 7
int main() {
//Day = 8;会报错,宏常量不能更改
cout << "Day值为:"<< Day <<endl;
system("pause");
return 0;
}
#include <iostream>
using namespace std;
int main() {
const int a = 7; // 注意需要添加分号
//a = 8; // 会报错,const常量不能更改
cout << "a值为:" << a << endl;
system("pause");
return 0;
}
1.5 关键字(标识符)
起变量名时,不可以关键字作为变量名。
1.6 标识符起名规则
作用:给C++规定的标识符(变量、常量)命名时,有一套自己的规则。给标识符命名时,争取左到见名知意的效果,方便自己和其他人阅读。
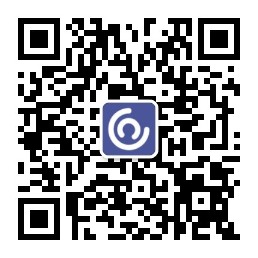
- 标识符不能是关键字(见1.5)
- 标识符只能由字母、数字、下划线组成
- 第一个字符必须是字母或者下划线
- 标识符中字母区别大小写
2. 数据类型
给变量分配一个合适的内存空间,
2.1 整型
int 变量名=变量值
长长整型long long
中间有空格,短整型short
取值范围为(-32768~32767),最常用的为int
2.2 sizeof
可以统计数据类型所占内存大小
sizeof(数据类型/变量名)
#include <iostream>
using namespace std;
int main() {
short a = 1;
int b = 2;
long c = 3;
long long d = 4;
cout << "size of short " << sizeof(short) << endl;
cout << "size of short " << sizeof(a) << endl;
cout << "size of int " << sizeof(int) << endl;
cout << "size of int " << sizeof(b) << endl;
cout << "size of long " << sizeof(c) << endl;
cout << "size of long " << sizeof(c) << endl;
cout << "size of long long " << sizeof(d) << endl;
cout << "size of long long " << sizeof(d) << endl;
system("pause");
return 0;
}
short<int<=long<=long long (long在linux32位为4字节,在linux64位为8字节)
2.3 浮点型
根据可以表示的数字范围不同可以分为单精度和双精度
- 单精度 float
- 双精度 double
定义格式
float 变量名=变量值f f是因为编译器默认数字为double类型,如果不加f,会多做一步转换
double 变量名=变量值
默认情况,输出一个小数,会输出6位有效数字,不是小数点后6位,而是六位有效数字,示例代码如下
#include <iostream>
using namespace std;
int main() {
float a = 3.1415926f;
cout << a*100 << endl;
system("pause");
return 0;
}
科学计数法:
#include <iostream>
using namespace std;
int main() {
//float a = 3.1415926f;
float a = 1e-3;
cout << a << endl;
system("pause");
return 0;
}
如果位数过高那么会以e的形式打印:
2.4 字符型
占用一个字符
char 变量名=字符值
‘a’
- 注意1 :要用单引号括起来,不能用多引号
- 注意2 :单引号里只能有一个字符,不可以是字符串
- 注意3:字符型变量并不是把字符本身放到内存中存储,而是将对应的ASCII编码存到存储单元中去,用强制转换可以查看字符串对应的ASCII码
#include <iostream>
using namespace std;
int main() {
char a = 'a';
cout << a << endl;
/*
a:97
A:65
*/
cout <<"a对应的ASCII码为:" << (int)a << endl; // 强制转换
system("pause");
return 0;
}
a
a对应的ASCII码为:97
请按任意键继续. . .
2.5 转义字符
用于表示一些不能显示出来的ASCII字符
水平制表位可以让输出更加整齐,有八个空格,下面演示水平制表符的效果:
#include <iostream>
using namespace std;
int main() {
cout << "aa\tHello World" << endl;
cout << "aaa\tHello World" << endl;
cout << "aaaa\tHello World" << endl;
system("pause");
return 0;
}
aa Hello World
aaa Hello World
aaaa Hello World
请按任意键继续. . .
2.6 字符串型
字符串在C++中有两种风格,一种是沿用C风格、一种是C++风格:
- C风格:
char 变量名[ ] = “字符串值”
- C++风格:
string 变量名=“字符串值”
C风格的字符串和C++风格的字符串演示如下:
#include <iostream>
using namespace std;
int main() {
/*
// C语言风格定义字符串
char a[] = "Hello World";
cout << a << endl;
system("pause");
return 0;
*/
// C++风格定义字符串
string b = "Hello World";
cout << b << endl;
system("pause");
return 0;
}
输出都为Hello World。visual studio2022中不需要再引入#include <string>,但是还是建议引入。
2.7 布尔类型
占用1个字节,取值为0和1,0代表假(false),1代表真(true)。
2.8 数据输入
之前写的代码只包括输出,为cout,通过键盘输入数据为cin,通过cin可以接收不同数据类型的值,实例代码如下:
#include <iostream>
#include <string>
using namespace std;
int main() {
//1.整型
int a; // 不定义初始值
cout << "请输入一个整数:" << endl;
cin >> a;
//2.浮点型
float b;
cout << "请输入一个浮点数:" << endl;
cin >> b;
//3.字符型
char c;
cout << "请输入一个字符:" << endl;
cin >> c;
//4.字符串
string d;
cout << "请输入一个字符串:" << endl;
cin >> d;
//5.布尔型
bool e;
cout << "请输入一个布尔值:" << endl;
cin >> e;
cout << "整数a的值为:" << a << endl;
cout << "浮点数b的值为:" << b << endl;
cout << "字符c的值为:" << c << endl;
cout << "字符串d的值为:" << d << endl;
cout << "布尔e的值为:" << e << endl;
system("pause");
return 0;
}
请输入一个整数:
1
请输入一个浮点数:
1.1
请输入一个字符:
a
请输入一个字符串:
about
请输入一个布尔值:
1
整数a的值为:1
浮点数b的值为:1.1
字符c的值为:a
字符串d的值为:about
布尔e的值为:1
ctrl+K+C是整段注释
3. 运算符
3.1 算术运算符
- 两个整数相除,结果仍然是整数,除数不能为0
10/20=0
10/0报错
- 两个小数不能做取模运算
递增和递减都分为前置和后置,区别在于是先加还是先运算。举例前置和后置递增代码如下:
#include <iostream>
using namespace std;
int main() {
int a = 2;
int b;
//前置++
//b = ++a; // 结果为a=3,b=3
// 后置++
b = a++; // 结果为a=3,b=2
cout << "a: " << a << " b: " << b<<endl;
system("pause");
return 0;
}
前置++是先+再运算,后置++是先运算再+
3.2 赋值运算符
3.3 比较运算符
比较运算符返回结果为bool值,0或1,相等是两个=,不等于是 !=
3.4 逻辑运算符
4. 程序流程结构
流程结构分为三种:顺序结构、选择结构、循环结构
- 顺序结构:程序按顺序执行,不发生跳转
- 选择结构:依据条件是否满足,有选择的执行相应功能
- 循环结构:依据条件是否满足,循环多次执行某段代码
4.1 选择结构
4.1.1 if 语句判断
if-else
#include <iostream>
using namespace std;
int main() {
// 定义一个分数
int score = 0;
cout << "请输入你的分数:";
cin >> score;
cout << "你输入的分数为:" << score << endl;
if (score > 600) {
cout << "恭喜你,你已被录取!" << endl;
}
else
{
cout << "很遗憾,你没有被录取" << endl;
}
system("pause");
return 0;
}
if=else if -else
#include <iostream>
using namespace std;
int main() {
// 定义钱数
int money = 0;
cout << "请输入你的钱数:";
cin >> money;
cout << "你的钱数为:" <<money<< endl;
// 语句判断
if (money >= 1000) {
cout << "坐飞机回家!" << endl;
}
else if (money >= 500) {
cout << "坐高铁回家!" << endl;
}
else {
cout << "坐火车回家!" << endl;
}
system("pause");
return 0;
}
嵌套if语句
目的是更精准的判断
#include <iostream>
using namespace std;
int main() {
// 定义钱数
int money = 0;
cout << "请输入你的钱数:";
cin >> money;
cout << "你的钱数为:" << money << endl;
// 语句判断
if (money >= 1000) {
if (money >= 2000) {
cout << "坐飞机商务舱回家!" << endl;
}
else {
cout << "坐飞机经济舱回家!" << endl;
}
}
else if (money >= 500) {
cout << "坐高铁回家!" << endl;
}
else {
cout << "坐火车回家!" << endl;
}
system("pause");
return 0;
}
三只小猪比体重:
#include <iostream>
using namespace std;
int main() {
// 初始化体重
int weightA = 0;
int weightB = 0;
int weightC = 0;
// 接收体重
cout << "请输入小猪A的体重:";
cin >> weightA;
cout << "请输入小猪B的体重:";
cin >> weightB;
cout << "请输入小猪C的体重:";
cin >> weightC;
// 打印体重
cout << "小猪A的体重为:" << weightA << endl;
cout << "小猪B的体重为:" << weightB << endl;
cout << "小猪C的体重为:" << weightC << endl;
// 进行比较
if ((weightA > weightB) && (weightA > weightC)) {
cout<<"三只小猪中体重最重的为小猪A,体重为:"<<weightA<<endl;
}
else if ((weightB > weightA) && (weightB > weightC)) {
cout << "三只小猪中体重最重的为小猪B,体重为:" << weightB << endl;
}
else
{
cout << "三只小猪中体重最重的为小猪C,体重为" << weightC << endl;
}
system("pause");
return 0;
}
请输入小猪A的体重:100
请输入小猪B的体重:200
请输入小猪C的体重:300
小猪A的体重为:100
小猪B的体重为:200
小猪C的体重为:300
三只小猪中体重最重的为小猪C,体重为300
请按任意键继续. . .
4.1.2 三目运算符
语法:表达式1?表达式2:表达式3
- 如果表达式1为真,执行表达式2,并返回表达式2的结果;
- 如果表达式1为假,执行表达式3,并返回表达式3的结果;
三目运算符返回的是变量,可以继续赋值
int a =10;
int b =10 ;
(a>b? a:b) =100 ======> b==100
4.1.3 switch语句
if判断适合有区间判断的情况,switch语句适用于选择项比较明确的情况,下面进行代码举例,特别说明,需要在每一个case后加上break,否则代码将从第一个符合条件的case一直往下执行。最后加上default捕获没有case列举的情况:
#include <iostream>
using namespace std;
int main() {
int day = 0;
cout << "请输入星期:";
cin >> day;
switch (day)
{
case 1:
cout << "星期一" << endl;
break;
case 2:
cout << "星期二" << endl;
break;
case 3:
cout << "星期三" << endl;
break;
case 4:
cout << "星期四" << endl;
break;
case 5:
cout << "星期五" << endl;
break;
default:
cout << "星期放假!" << endl;
break;
}
system("pause");
return 0;
}
4.2 循环结构
满足循环条件,执行循环语句
4.2.1 while循环
while (循环条件){循环语句}
需要注意的是,需要明确while语句退出的条件,否则会陷入循环
代码举例:
#include <iostream>
using namespace std;
int main() {
int num = 10;
int count = 0;
while (count < num) {
cout << count << endl;
count++;
}
system("pause");
return 0;
}
0
1
2
3
4
5
6
7
8
9
猜数字实战
案例描述: 系统随机生成一个1到100之间的数字,玩家进行猜测,如果猜错,提示玩家数字过大或过小,如果猜对恭喜玩家胜利,并且退出游戏。
#include <iostream>
using namespace std;
#include <ctime>
int main() {
// 添加随机数种子,防止每一次目标数字都一样
srand((unsigned int)time(NULL));
int target = rand()%100 +1; // 目标数字
int num = 0;
while (true)
{
// 键盘接受数字
cout << "请输入一个数字:";
cin >> num;
if (num == target) {
cout << "猜中了!" << endl;
break;
}
else if (num > target) {
cout << "大了!" << endl;
}
else
{
cout << "小了!" << endl;
}
}
system("pause");
return 0;
}
请输入一个数字:45
大了!
请输入一个数字:34
小了!
请输入一个数字:42
猜中了!
请按任意键继续. . .
4.2.2 do-while循环
与while的区别是do-while会先执行一次循环语句,再判断循环条件,同样以输出0-9数字为例
#include <iostream>
using namespace std;
int main() {
int num = 0;
do {
cout << num << endl;
num++;
} while (num < 10);
system("pause");
return 0;
}
0
1
2
3
4
5
6
7
8
9
请按任意键继续. . .
4.2.3 for循环
语法:for(起始表达式;条件表达式;末尾循环体){循环语句}
同样,以打印数字0-9为例:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
cout << i << endl;
}
system("pause");
return 0;
}
0
1
2
3
4
5
6
7
8
9
请按任意键继续. . .
可以看到,for循环比while结构性更强,可读性也更好,while和for在大多数情况下可以互换,while和for最大区别就是for知道循环次数,while不知道循环次数。
4.2.4 嵌套循环
在循环体中再嵌套一层循环,解决一些实际问题,以9*9乘法表为例:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i < 10; i++) {
for (int j = 1; j <= i; j++) {
cout << j << "x" << i <<"=" << i * j<<" ";
}
cout << endl;
}
system("pause");
return 0;
}
1x1=1
1x2=2 2x2=4
1x3=3 2x3=6 3x3=9
1x4=4 2x4=8 3x4=12 4x4=16
1x5=5 2x5=10 3x5=15 4x5=20 5x5=25
1x6=6 2x6=12 3x6=18 4x6=24 5x6=30 6x6=36
1x7=7 2x7=14 3x7=21 4x7=28 5x7=35 6x7=42 7x7=49
1x8=8 2x8=16 3x8=24 4x8=32 5x8=40 6x8=48 7x8=56 8x8=64
1x9=9 2x9=18 3x9=27 4x9=36 5x9=45 6x9=54 7x9=63 8x9=72 9x9=81
请按任意键继续. . .
4.3 跳转语句
4.3.1 break
break用于跳出选择结构或者循环结构,使用情况如下:
- switch语句中,case后使用
- 循环语句中,跳出循环
- 嵌套循环,跳出当前最近的内层循环
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
cout << i << endl;
if (i == 5) {
break;
}
}
system("pause");
return 0;
}
0
1
2
3
4
5
请按任意键继续. . .
4.3.2 continue语句
跳过本次循环中余下尚未执行的语句,继续执行下一次循环,同样以上面的代码为例:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue;
}
cout << i << endl;
}
system("pause");
return 0;
}
0
1
2
3
4
6
7
8
9
请按任意键继续. . .
可以看到,跳过了5,并且继续向后执行后面的循环。
4.3.3 goto语句
无条件跳转语句,跳转到标记的位置
注意第二个FLAG之后是一个冒号,说明是一个标记,不建议使用
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
goto FLAG;
}
cout << i << endl;
}
FLAG:
cout << "跳转成功!" << endl;
system("pause");
return 0;
}
0
1
2
3
4
跳转成功!
请按任意键继续. . .