以下是一个基于Spring Boot的简单后台管理网站的示例代码,包括用户管理、角色管理、登录退出等功能。
- 创建Spring Boot项目
首先,创建一个新的Spring Boot项目。可以使用Spring Initializer(https://start.spring.io/)来生成项目的初始结构。确保选择适当的依赖项,如Spring Web和Spring Security。
- 配置数据库
在application.properties文件中配置数据库连接信息,例如:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb spring.datasource.username=root spring.datasource.password=123456 spring.datasource.driver-class-name=com.mysql.jdbc.Driver
- 创建实体类和数据库表
创建User和Role实体类,用于表示用户和角色。同时,在数据库中创建相应的表。
User.java:
@Entity @Table(name = "users") public class User implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
// getters and setters
}
Role.java:
@Entity @Table(name = "roles") public class Role implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String name;
// getters and setters
}
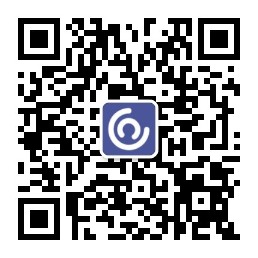
- 创建数据访问层
创建UserRepository和RoleRepository接口,用于访问数据库中的用户和角色数据。
UserRepository.java:
@Repository public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
RoleRepository.java:
@Repository public interface RoleRepository extends JpaRepository<Role, Long> {
Role findByName(String name);
}
- 创建服务层
创建UserService和RoleService接口以及其实现类,用于处理用户和角色的业务逻辑。
UserService.java:
public interface UserService {
User findByUsername(String username);
}
UserServiceImpl.java:
@Service public class UserServiceImpl implements UserService {
@Autowired
private UserRepository userRepository;
@Override
public User findByUsername(String username) {
return userRepository.findByUsername(username);
}
}
RoleService.java:
public interface RoleService {
Role findByName(String name);
}
RoleServiceImpl.java:
@Service public class RoleServiceImpl implements RoleService {
@Autowired
private RoleRepository roleRepository;
@Override
public Role findByName(String name) {
return roleRepository.findByName(name);
}
}
- 创建控制器
创建UserController和RoleController类,用于处理用户和角色的HTTP请求。
UserController.java:
@RestController @RequestMapping("/users") public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{username}")
public User getUserByUsername(@PathVariable String username) {
return userService.findByUsername(username);
}
}
RoleController.java:
@RestController @RequestMapping("/roles") public class RoleController {
@Autowired
private RoleService roleService;
@GetMapping("/{name}")
public Role getRoleByName(@PathVariable String name) {
return roleService.findByName(name);
}
}
- 创建安全配置
创建一个SecurityConfig类,用于配置Spring Security,包括登录和退出功能。
SecurityConfig.java:
@Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserService userService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login", "/logout").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/home")
.permitAll()
.and()
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/login")
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userService);
}
}
- 创建页面
创建login.html和home.html等页面,用于用户登录和后台管理功能。这些页面可以使用Thymeleaf或其他前端技术来渲染。
login.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title></head>
<body><h1>Login</h1>
<form action="/login" method="post"><label for="username">Username:</label> <input type="text" id="username"
name="username" required><br> <label
for="password">Password:</label> <input type="password" id="password" name="password" required><br> <input
type="submit" value="Login"></form>
</body>
</html>
home.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Home</title></head>
<body><h1>Welcome, [[${username}]]!</h1> <a href="/logout">Logout</a></body>
</html>
- 启动应用程序
运行应用程序,访问http://localhost:8080/login进行登录,然后访问http://localhost:8080/home进行后台管理。
这只是一个简单的示例,可以根据具体需求进行扩展和定制。例如,可以添加更多的功能、权限控制和页面样式等。