一、前言
除了上一章C++变量与蓝图通信讲的变量能与蓝图通信外,还有函数和枚举也可以和蓝图通信。函数的关键字为”UFUNCTION“、枚举的关键字为”UENUM“。
二、实现
2.1、BlueprintCallable蓝图中调用
该函数时带执行的,带入如下。编译成功后在蓝图中输入后可以找到,并点击使用如图2.1.1所示
/// <summary>
/// 暴露该函数可在蓝图中调用
/// </summary>
UFUNCTION(BlueprintCallable, Category = "MyFunction")
void BlueprintCallable1();
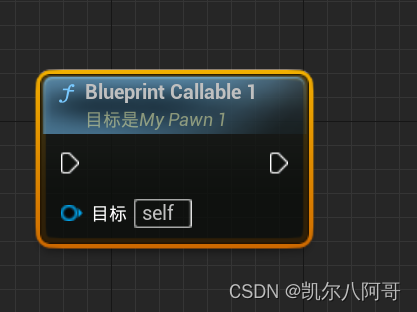
2.2、BlueprintPure蓝图中的纯函数
代码如下,其在蓝图中的形式如图2.2.1所示,它是一个纯函数和图2.1.1不同的是它没有左右
/// <summary>
/// 可在蓝图中调用的纯函数的定义
/// </summary>
UFUNCTION(BlueprintPure, Category = "MyFunction")
bool BlueprintPure2();
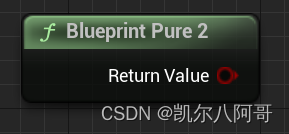
两边的执行引脚。
2.3、BlueprintImplementableEvent
在C++中声明蓝图中实现,在蓝图中可重载,可以有参数和返回值,无返回值的是事件,有返回值的是函数。
1、没有返回值和参数
定义的代码如下所示,
UFUNCTION(BlueprintImplementableEvent)
void ImplementableEvent1();
然后在蓝图中它会作为事件添加到蓝图中使用,如图2.3.1所示:
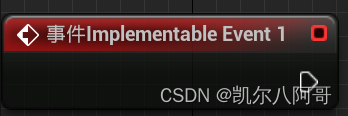
该事件可以在C++代码中调用
void AMyPawn1::BeginPlay()
{
Super::BeginPlay();
ImplementableEvent1();
}
2、只有返回值
只有返回值的在蓝图中会作为函数,如图2.3.2所示,同时这个函数可以在蓝图中被重写
UFUNCTION(BlueprintImplementableEvent)
int32 ImplementableEvent2();
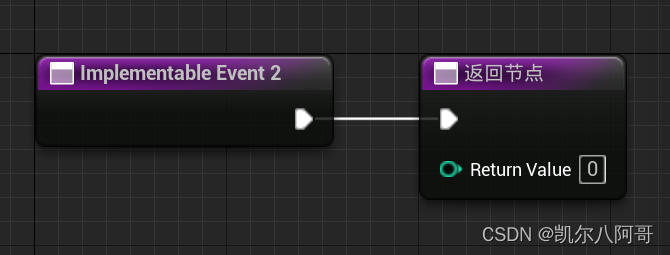
但是在蓝图还无法调用它,需要添加”Blueprint Callable“关键字,如下代码,注意两个关键字的顺

UFUNCTION(BlueprintCallable, BlueprintImplementableEvent)
int32 ImplementableEvent2();
序,如果把Blueprint Callable“关键字放在后面编译不通过。增加关键字后的蓝图中可以调用,如图2.3.4所示:
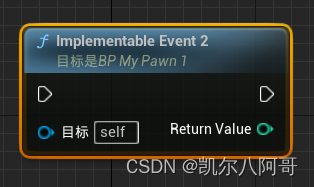
3、只有参数
代码如下所示,添加一个带参数的,这个效果和图2.3.1中的类似,不同之处是这里会带参数,如图2.3.4所示:
UFUNCTION(BlueprintImplementableEvent)
void ImplementableEvent3(const FString& myStr);
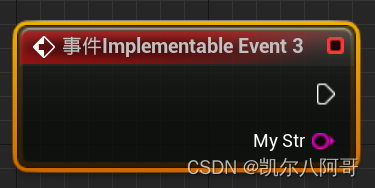
4、参数和返回值都有
代码如下所示,其效果和图2.3.2所示类似,区别之处在于此处是带输入参数的。
UFUNCTION(BlueprintImplementableEvent)
int32 ImplementableEvent4(const FString& myStr);
2.4、BlueprintNativeEvent
在C++中声明和实现,蓝图可重载,函数实现的后面要加Implementation,否则会编译出错,但是在调用的时候还是用声明时的名字。无返回值的是事件,有返回值的是函数。代码如下
UFUNCTION(BlueprintNativeEvent)
void BlueprintNativeEvent1();
UFUNCTION(BlueprintNativeEvent)
int32 BlueprintNativeEvent2();
UFUNCTION(BlueprintNativeEvent)
void BlueprintNativeEvent3(const FString& myStr);
UFUNCTION(BlueprintNativeEvent)
int32 BlueprintNativeEvent4(const FString& myStr);
实现的代码如下,又返回值的必须返回一个值。
void AMyPawn1::BlueprintNativeEvent1_Implementation()
{
GEngine->AddOnScreenDebugMessage(-1, 5.0f, FColor::Red, TEXT("这是一个C++代码写的BlueprintNativeEvent事件"));
}
int32 AMyPawn1::BlueprintNativeEvent2_Implementation()
{
return 0;
}
void AMyPawn1::BlueprintNativeEvent3_Implementation(const FString& myStr)
{
}
int32 AMyPawn1::BlueprintNativeEvent4_Implementation(const FString& myStr)
{
return 0;
}
2.5、Meta元素说明符
该函数定义如下,而且必须实现,通过meta关键字实现其在蓝图中的名称改为“MyPrintTest”
//元数据说明符,也即别名,在蓝图中会显示DisplayName定义的名字
UFUNCTION(BlueprintCallable, Category = "MyFunction", meta = (DisplayName = "MyPrintTest"))
void PrintMeta();
2.6、枚举
首先定义一个宏,代码如下,生成枚举的反射数据,通过反射将枚举暴露给蓝图,实现C++和蓝图的通信,BlueprintType的作用是可以在蓝图创建变量的时候也可以作为选项。如图2.6.1所示
UENUM(BlueprintType)//生成枚举的反射数据,通过反射将枚举暴露给蓝图,实现C++和蓝图的通信,BlueprintType的作用是可以在蓝图创建变量的时候也可以作为选项
namespace MyEnumType
{
enum MyCustomEnum
{
Type1,
Type2,
Type3,
};
}
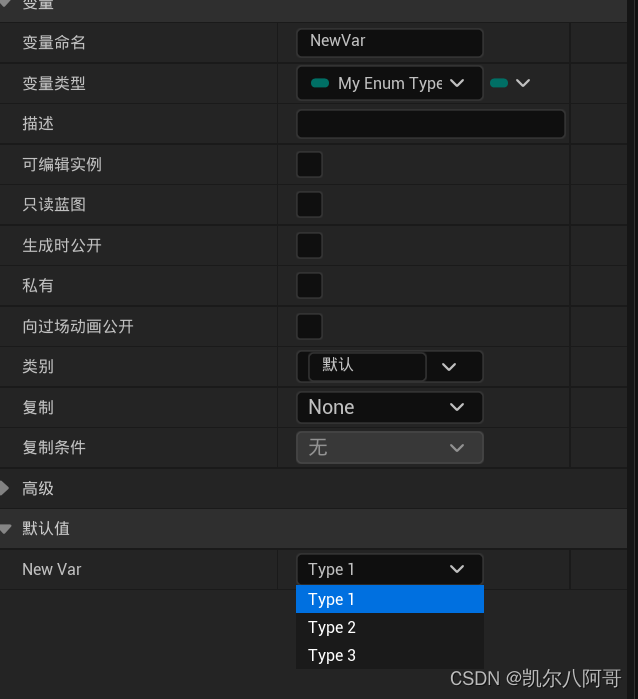
在蓝图中创建一个新的变量,变量的类型中可以选中C++代码中创建的这个枚举。同时,在蓝图中也可以调用该枚举,如图2.6.2所示:
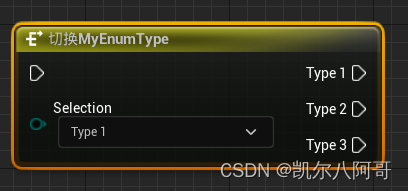
通过以下代码可以实现在各类面板和蓝图中使用该变量
//枚举
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "MyEnum")
TEnumAsByte<MyEnumType::MyCustomEnum> MyCustomEnum;
同时也可以在蓝图中调用和编辑该变量,如图2.6.3所示
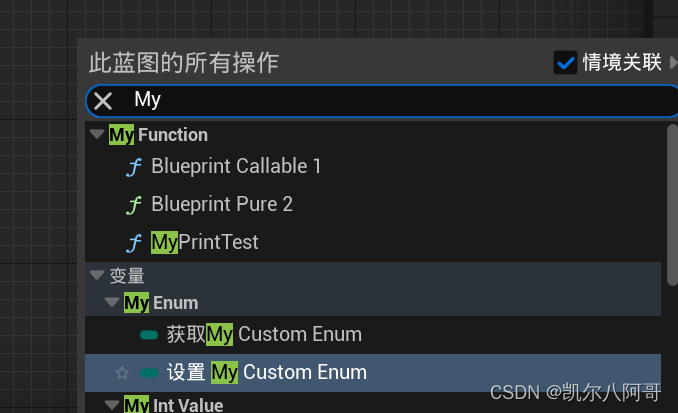
通常一个定义里只能有一个枚举,如下代码中会编译不通过,在MyEnumType中只能定义一个。
UENUM(BlueprintType)
namespace MyEnumType
{
enum MyCustomEnum
{
Type1,
Type2,
Type3,
};
enum MyEnum2
{
One, Two, Three,
};
}
三、总结
3.1、BlueprintImplementableEvent的函数在C++代码中只需要声明不需要实现。
3.2、BlueprintNativeEvent的函数在C++代码中声明了还必须实现,不实现会编译报错。实现中的函数名称后总有加上”_Implementation“,但是在调用的时候又要去掉”_Implementation“,这样才能在蓝图中被调用,使用带后缀”_Implementation“的只会在C++中调用,而蓝图中不会被调用。
3.3、”Blueprint Callable“关键字通常是放在前面的,如果放在后面可能会编译不通过。
3.4、BlueprintType的作用是可以在蓝图创建变量的时候也可以作为选项。
3.5、枚举的定义中只能定义一个枚举,多个会编译不通过。