一、帧动画:
第一步:drawable中添加要做帧动画的图片,并新建一个frame.xml文件
<?xml version="1.0" encoding="utf-8"?>
<animation-list xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 添加图片和显示的时间-->
<item android:drawable="@drawable/img1" android:duration="220"/>
<item android:drawable="@drawable/img2" android:duration="220"/>
<item android:drawable="@drawable/img3" android:duration="220"/>
</animation-list>
第二步:MainActivity的xml文件中引用 frame :
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/frame"
android:id="@+id/ctl"
tools:context=".MainActivity">
</androidx.constraintlayout.widget.ConstraintLayout>
第三步:点击启动和停止动画
private boolean flag=true;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ConstraintLayout mCtl = findViewById(R.id.ctl);
AnimationDrawable animation= (AnimationDrawable) mCtl.getBackground();
mCtl.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 启动动画
if (flag){
animation.start(); //启动动画
flag=false;
}else {
animation.stop();
flag=true;
}
}
});
}
二、补间动画:
第一、alpha 透明度
1、res中新见一个alpha.xml文件
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!--
fromAlpha:0 完全透明
toAlpha:1 完全不透明
duration: 显示周期
-->
<alpha
android:fromAlpha="0"
android:toAlpha="1"
android:duration="2000"
/>
</set>
2、activity的xml文件中添加ImageView
<androidx.appcompat.widget.AppCompatImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/img1"
android:adjustViewBounds="true"
android:maxWidth="500dp"
android:maxHeight="500dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
/>
3、activity中使用:
ImageView img=findViewById(R.id.image);
img.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 通过加载xml动画设置文件,来创建一个Animation对象
Animation animation=AnimationUtils.loadAnimation(TweenAnimActivity.this,R.anim.alpha);
img.startAnimation(animation);
}
});
第二、rotate 旋转
1、res中新建一个rotate.xml文件:
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!--
fromDegrees:开始的位置
toDegrees:旋转多少度
pivotX:旋转的中心点
pivotY:
-->
<rotate
android:fromDegrees="0"
android:toDegrees="360"
android:pivotX="50%"
android:pivotY="50%"
android:duration="2000"
/>
</set>
2、activity中使用:
ImageView img=findViewById(R.id.image);
img.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 通过加载xml动画设置文件,来创建一个Animation对象
Animation animation=AnimationUtils.loadAnimation(TweenAnimActivity.this,R.anim.rotate);
img.startAnimation(animation);
}
});
第三、scale 缩放
1、res中新建scale,xml文件:
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!--
fromXScale:x的原始大小
fromYScale:Y的原始大小
pivotY:缩放到的y的大小
pivotX:缩放到的x的大小
toXScale:缩放的中心点
toYScale:
duration:动画执行的周期时间
-->
<scale android:fromXScale="1"
android:fromYScale="1"
android:pivotY="0.5"
android:pivotX="0.5"
android:toXScale="50%"
android:toYScale="50%"
android:duration="2000"/>
</set>
2、activity中使用:
ImageView img=findViewById(R.id.image);
img.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 通过加载xml动画设置文件,来创建一个Animation对象
Animation animation=AnimationUtils.loadAnimation(TweenAnimActivity.this,R.anim.scale);
img.startAnimation(animation);
}
});
第四、translate 平移
1、在res中新建translate.xml文件
扫描二维码关注公众号,回复:
17319465 查看本文章
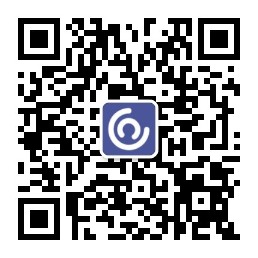
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<translate android:fromXDelta="0"
android:fromYDelta="0"
android:toXDelta="200"
android:toYDelta="200"
android:duration="2000"/>
</set>
2、在activity中使用
ImageView img=findViewById(R.id.image);
img.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 通过加载xml动画设置文件,来创建一个Animation对象
Animation animation=AnimationUtils.loadAnimation(TweenAnimActivity.this,R.anim.translate);
img.startAnimation(animation);
}
});
三、属性动画:
1第一、ValueAnimator:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tween_anim);
ValueAnimator animator=ValueAnimator.ofFloat(0f,1f);
animator.setDuration(2000);
animator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(@NonNull ValueAnimator animation) {
Object value = animation.getAnimatedValue();
Log.d(TAG, "onAnimationUpdate: "+value);
}
});
animator.start();
}
第二、ObjectAnimator:给某个空间设置动画就家那个控件
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tween_anim);
TextView textView=findViewById(R.id.tv);
// 第二个参数只要是textview中有set的属性都可以设置
ObjectAnimator objectAnimator = ObjectAnimator.ofFloat(textView, "alpha", 0f,1f);
objectAnimator.setDuration(2000);
objectAnimator.start();
}
第三、监听器:
1、全部监听,重写所有
objectAnimator.addListener(new Animator.AnimatorListener() {
@Override
public void onAnimationStart(@NonNull Animator animation) {
// 开始调用
}
@Override
public void onAnimationEnd(@NonNull Animator animation) {
// 结束调用
}
@Override
public void onAnimationCancel(@NonNull Animator animation) {
// 取消调用
}
@Override
public void onAnimationRepeat(@NonNull Animator animation) {
// 重复执行调用
}
});
2、使用哪个监听,重写哪个
objectAnimator.addListener(new AnimatorListenerAdapter() {
@Override
public void onAnimationStart(Animator animation) {
super.onAnimationStart(animation);
}
});