1. return函数返回值要和定义的函数类型相同
2. 逗号表示式:返回逗号后面的表达式
例如:int func(){return ...} ...:中的数据类型必须是int型
3. 堆区:
利用关键字new来开辟堆区。
注意:利用new创建的数据会返回该数据对应的数据类型。
由程序员分配释放,用关键字delete + 堆名 来进行释放。若程序员没有释放,在程序结束后操作系统自动释放。
示例:
//开辟一个堆区,利用new关键字,类型为int型,存入20
int* b = new int(20);
4. 栈区:
扫描二维码关注公众号,回复:
17281633 查看本文章
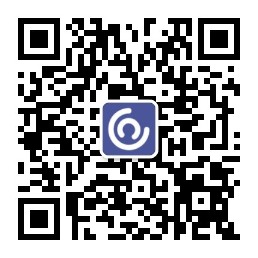
由编译器自行分配释放。
注意:不要返回局部变量的地址,栈区开辟的数据由编译器自行释放。
5. 局部变量:static -- 生命周期:整个程序运行过程
#include<iostream>
using namespace std;
//int& test01():代表该函数返回的是变量名
int& test01()
{
int a = 10;
return a;
}
int& test02()
{
//static局部变量:生命周期在整个程序运行期间
static int b = 20;
return b;
}
int& test03()
{
int c = 30;
return c;
}
int main()
{
int& a = test01();
//本质上,变量a仍为栈区的一个变量名,返回的是a的地址,编译器会自动释放
cout << "a = " << a << endl; //10
cout << "a = " << a << endl; //2076744072
int& b = test02();
cout << "b = " << b << endl; //20
cout << "b = " << b << endl; //2076744072
int& c = test03();
cout << "c = " << c << endl; //30
cout << "c = " << c << endl; //2076744072
//函数做左值,必须返回引用。(引用本质上就是返回变量本身,
即栈区。而栈内的变量名指向堆区的内存空间,即数值)
test03() = 40;
cout << "c = " << c << endl; //40
cout << "c = " << c << endl; //2076744072
test02() = 50;
cout << "b = " << b << endl; //50
cout << "b = " << b << endl; //50
return EXIT_SUCCESS;
}
6. 引用:
A)引用参数产生的效果同按地址传递是一样的。本质上是一个指针常量。
B)如果要防止形参改变实参,可以在函数的形参列表中,给引用加上const修饰
#include<iostream>
using namespace std;
int main0803()
{
int a = 10;
//int& b = a本质上就是int* const b = &a,即指针常量
int& b = a;
b = 20;
cout << "a = " << a << endl << "b = " << b << endl;
//int& c = 10; 报错,引用本身需要一个合法的内存空间,即栈
const int& c = 10; //加入const优化了代码,即:int temp = 10; const int& c = temp;
cout << "c = " << c << endl;
return EXIT_SUCCESS;
}
7. 函数默认的参数要放到参数列表的最后。
8. 函数重载:
条件:1.函数名相同 2.在同一个作用域下 3.函数参数类型不同或个数不同或顺序不同
#include<iostream>
using namespace std;
//1.引用作为重载条件
void func(int& a)
{
cout << "void func(int& a)" << endl;
}
void func(const int& a)
{
cout << "void func(const int& a)" << endl;
}
int main()
{
int a = 10;
func(10); //void func(const int& a)
func(a); //void func(int& a)
const int b = 11;
func(b);
return EXIT_SUCCESS;
}
9. 指针强化:
int a = 10;
int* p = &a;
int** q = &p;
cout << p << endl;//a的地址 00000020831FF854
cout << *p << endl;//a的值 10
cout << q << endl;//p的地址 00000020831FF878
cout << *q << endl;//*q就是p 00000020831FF854
cout << **q << endl;//**q就是a 10