简单记录一下vue中组件之间的几种通信方式
1、父传子,子组件中定义“props”来接收;
props传值的限定条件以及规则可参考官网:Props | Vue.js
父:
<template>
<div>
<div>我是父组件</div>
<children :father="father"></children>
</div>
</template>
<script>
import children from './children.vue'
export default {
data() {
return {
father: '这是父组件传递过来的值'
}
},
components: {
children
},
methods: {
}
}
</script>
<style>
</style>
子:
<template>
<div>我是子组件:<span style="color: blue;">{
{father}}</span>
</div>
</template>
<script>
export default {
data() {
return {
}
},
props: ['father'],
}
</script>
<style>
</style>
2、子传父:子组件通过自定义事件,然后父组件通过监听子组件的事件获取传递过来的值
子组件定义this.$emit('myFun',value)父组件通过@myFun获取传递的值,代码如下
父:
<template>
<div>
<div>我是父组件:
<span style="color:blue">{
{value}}</span>
</div>
<children @myFun="receive"></children>
</div>
</template>
<script>
import children from './children.vue'
export default {
data() {
return {
value: '',
}
},
components: {
children
},
methods: {
receive(val) {
this.value = val
},
}
}
</script>
<style>
</style>
子:
<template>
<div>
<div>我是子组件</div>
<button @click="btn">传递</button>
</div>
</template>
<script>
export default {
data() {
return {
}
},
methods: {
btn() {
this.$emit('myFun', '子组件传递过来的值')
},
}
}
</script>
<style>
</style>
3、兄弟组件之间的数据传递:运用定义事件emit的触发和监听能力,定义一个公共的事件总线bus,通过它作为中间桥梁,可以实现组件之间的通信
首先创建bus.js文件
扫描二维码关注公众号,回复:
17261367 查看本文章
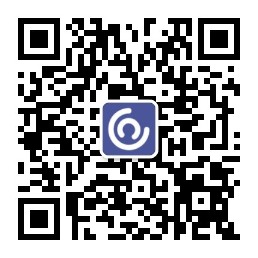
import Vue from 'vue'
export default new Vue()
父:
<template>
<div>
<div>我是父组件</div>
<children></children>
<children2></children2>
</div>
</template>
<script>
import children from './children.vue'
import children2 from './children2.vue'
export default {
data() {
return {}
},
components: {
children,
children2
},
methods: {
}
}
</script>
<style>
</style>
子1:
<template>
<div>
<div>我是子组件1:</div>
<button @click="btn">传递</button>
</div>
</template>
<script>
import bus from './bus.js'
export default {
data() {
return {
}
},
methods: {
btn() {
bus.$emit('myFun', '子组件1传递是值')
},
}
}
</script>
<style>
</style>
子2:
<template>
<div>我是子组件2:<span style="color: blue;">{
{value}}</span></div>
</template>
<script>
import bus from './bus.js'
export default {
data() {
return {
value: '',
}
},
mounted() {
bus.$on('myFun', (val) => {
this.value = val
})
}
}
</script>
<style>
</style>
以上是最为常用的组件之间数据传递的方法,以下还有存储传递,vuex传递,路由传递、$refs等后续在进行补充