1.Service的基本作用
Service在代码中的的作用是调用Mapper、被Controller调用。是后端项目中非常重要的组件。
用于设计业务流程、业务逻辑,以保障数据的完整性、有效性、安全性。
2. Service使用举例——“添加相册”
在项目的根包下创建pojo.dto.AlbumAddNewDTO
类,用于封装业务方法所需的参数:
@Data
public class AlbumAddNewDTO implements Serializable {
private String name;
private String description;
private Integer sort;
}
再在项目的根包下创建service.IAlbumService
接口,并在接口添加“添加相册”的抽象方法:
public interface IAlbumService {
void addNew(AlbumAddNewDTO albumAddNewDTO);
}
再在项目的根包下创建service.impl.AlbumServiceImpl
类,实现以上接口,重写接口中声明的抽象方法,具体的实现,应该是:
package com.luoyang.small.service.impl;
import com.luoyang.small.mapper.AlbumMapper;
import com.luoyang.small.pojo.dto.AlbumAddNewDTO;
import com.luoyang.small.pojo.entity.Album;
import com.luoyang.small.service.IAlbumService;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* 接口实现
*
* @author luoyang
* @Date 2023/12/12
*/
// 添加在类上,标记当前类是业务逻辑组件类,用法同@Component
@Service
public class IAlbumServiceImpl implements IAlbumService {
/**
* 添加在属性上,使得Spring自动装配此属性的值
* 添加在构造方法上,使得Spring自动调用此构造方法
* 添加在Setter方法上,使得Spring自动调用此方法
*/
@Autowired
private AlbumMapper albumMapper;
@Override
public void addNew(AlbumAddNewDTO albumAddNewDTO) {
//检查相册名称是否占用
String name = albumAddNewDTO.getName();
int countByName = albumMapper.countByName(name);
//如果数据已存在还继续插入,我们这边直接报异常,不添加。
if (countByName > 0) {
throw new RuntimeException();
}
//创建Album对象
Album album = new Album();
//复制属性到album
BeanUtils.copyProperties(albumAddNewDTO, album);
//执行插入数据
albumMapper.insert(album);
}
}
以上实现中 不要忘记两个注解@Service @Autowired
@Service添加在类上,标记当前类是业务逻辑组件类
@Autowired 添加在属性上,使得Spring自动装配此属性的值; 添加在构造方法上,使得Spring自动调用此构造方法; 添加在Setter方法上,使得Spring自动调用此方法
以上实现中 countByName为计数——新增数据前检查是否数据已存在
在实现以上业务之前,需要在Mapper中补充功能,用于检查相册名称是否已经被占用,
这边以查看数据库中相册名是否存在为检查方式,需要执行的SQL语句大致是:
#查看当前相册名数量
select count(*) from pms_album where name=?
在AlbumMapper.java
接口中添加抽象方法:
/**
* 根据相册名称统计相册数据的数量
* @param name 相册名称
* @return 匹配相册名称的相册数据的数量
*/
int countByName(String name);
并在AlbumMapper.xml
中配置SQL语句:
<!-- int countByName(String name); -->
<select id="countByName" resultType="int">
SELECT count(*) FROM pms_album WHERE name=#{name}
</select>
完成后,在AlbumMapperTests
中编写并执行测试:
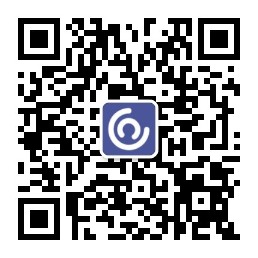
package com.luoyang.small;
import com.luoyang.small.mapper.AlbumMapper;
import com.luoyang.small.pojo.entity.Album;
import lombok.extern.slf4j.Slf4j;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
/**
* @author luoyang
* @date 2023/11/28
*/
@Slf4j
@SpringBootTest
public class AlbumMapperTests {
@Autowired
AlbumMapper mapper;
@Test
void insert() {
Album album = new Album();
album.setName("测试名称001");
album.setDescription("测试简介001l啦啦啦啦啦");
album.setSort(100); // 注意:由于MySQL中表设计的限制,此值只能是[0,255]区间内的
int rows = mapper.insert(album);
System.out.println("插入数据完成,受影响的行数:" + rows);
}
@Test
void countByName(){
String name = "测试名称001";
int count = mapper.countByName(name);
log.debug("根据名称【{}】统计梳理完成,结果:{}",name,count);
}
}
测试检查数据库已有名称的数量:1,表示数据已存在;
如果数据已存在还继续插入,我们这边直接报异常,不添加。
3.Service调用效果展示——编写测试类
完成后,在src/test/java
的根包下创建service.AlbumServiceTests
测试类*(其中新建service文件夹,相对于src/main/java
就不需要导包了)*
在此类中编写并执行测试,如下:
package com.luoyang.small.service;
import com.luoyang.small.pojo.dto.AlbumAddNewDTO;
import lombok.extern.slf4j.Slf4j;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
/**
* @author luoyang
* @Date 2023/12/12
*/
@Slf4j
@SpringBootTest
public class AlbumServiceTests {
//不建议声明为实现类型
@Autowired
IAlbumService iAlbumService;
@Test
void addNew() {
AlbumAddNewDTO albumAddNewDTO = new AlbumAddNewDTO();
albumAddNewDTO.setName("测试名称004");
albumAddNewDTO.setDescription("测试简介004啦啦啦啦啦");
albumAddNewDTO.setSort(100); // 注意:由于MySQL中表设计的限制,此值只能是[0,255]区间内的
try {
iAlbumService.addNew(albumAddNewDTO);
log.debug("添加相册成功!");
} catch (Exception e) {
log.debug("添加相册失败,{}", e.getMessage());
}
}
}
声明:部分代码来自有网络,文章只供学习参考
创造价值,乐哉分享!