1.安装 mysql
npm install mysql
2.引入MySql
import mysql from 'mysql'
3.连接MySql
const connection = mysql.createConnection({
host: 'yourServerip',
user: 'yourUsername',
password: 'yourPassword',
database: 'yourDatabase'
})
connection.connect(err => {
if (err) {
console.error('数据库连接失败: ' + err.stack)
return
}
console.log('数据库连接成功', err)
})
执行Mysql 增删改查
const sqlSentence = 'yourSqlStatementOrAddOrDeleteOrPutOrSelect'
connection.query(sqlSentence , (error, results, fields) => {
if (error) {
console.error('失败: ' + error)
return
}
console.log('成功:',results)
})
MySql 增删改查 常见语句
查询
扫描二维码关注公众号,回复:
17244515 查看本文章
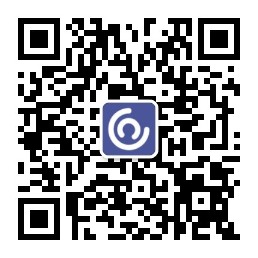
select * from 表名; //查询表内所有数据 select * from 表名1 union select * from 表名2 union select * from 表名3; //查询多个表内所有数据 select * from 表名 where id = 4; //查询表内单行数据
增加
1.增加列值 insert into 表名 (列名) values (列值); //增加列值 insert into 表名 (列名1, 列名2, 列名3, 列名3) values (列值1, "列值2", 列值3, "列值4"); //增加多个列值 2.增加列名 alter table 表名 add 列名 类型 //增加列名
修改
1.修改列值 update 表名 set 列名1 = "列值1" where id = 4; //修改单个 update 表名 set 列名1 = "列值1", 列名2 = "列值2" , 列名3 = "列值3" where id = 4; //修改多个 2.修改列名 alter table 表名 change 旧列名 新列名 新类型; alter table 表名 change 旧列名1 新列名1 新类型1 , change 旧列名2 新列名2 新类型2;//修改多个列名
删除
1.删除列值 delete from 表名; //删除表内所有值 delete from 表名1, 表名2, 表名3; //删除多个表内所有值 delete from 表名 where id = 5; //删除表内单行值 2.删除列名 alter table 表名 drop column 列名; alter table 表名 drop column 列名1 , alter table 表名 drop column 列名2; //删除多个列名 3.删除列名和列值 truncate table 表名 //删除表内所有列名和列值