文章目录
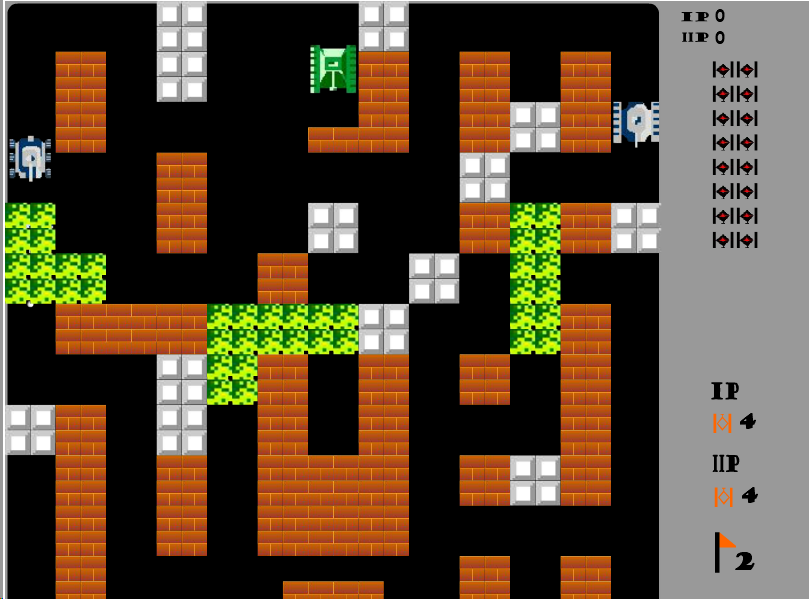
前言:
坦克大战90版本是一款2D射击游戏,玩家扮演一辆坦克,通过操纵坦克进行战斗。游戏场景通常是由迷宫状的地图构成,在地图中有各种不同类型的障碍物,如墙壁、水域、草地等,这些障碍物会对玩家的行动和战斗产生影响。
玩家的目标是摧毁敌方坦克并保护自己的基地。游戏中会有敌方坦克和敌方基地,玩家需要躲避敌方坦克的攻击并利用自己的技巧和策略来摧毁敌方坦克和基地。同时,玩家还可以尝试收集道具,如增强装甲、提高射速等,以增加自己的战斗能力。
这款游戏具有简单直观的操作方式,通过方向键控制坦克移动,空格键发射子弹。游戏节奏紧凑,战斗刺激,让玩家体验到了紧张的战争氛围。此外,游戏还支持多人模式,玩家可以与朋友一起组队或对战,增加了游戏的趣味性和竞争性。
坦克大战90版本不仅在当时取得了巨大成功,在后来的几十年里也不断被翻新和改进,衍生出了更多精彩的版本和新的玩法。无论是当年的经典版还是现代的重制版,坦克大战都是一款具有吸引力和娱乐性的经典游戏,让玩家流连忘返。
第一步:安装Pygame库
确保你已经安装了Python:在安装Pygame之前,确保你已经安装了Python。你可以从官方网站下载Python的最新版本并进行安装。Pygame兼容Python 2.x和Python 3.x版本。
打开终端:在Windows操作系统中,你可以按下Win+R键,在运行窗口中输入"cmd"并按下回车键打开命令提示符。在macOS和Linux系统中,你可以打开终端应用程序。
使用pip安装Pygame:在终端中输入以下命令来使用pip包管理器安装Pygame库:
pip install pygame
如果你使用的是Python 3,则使用以下命令:
pip3 install pygame
等待安装完成:一旦执行了安装命令,pip将会自动下载和安装Pygame库及其依赖项。这个过程可能需要一些时间,取决于你的网络速度和系统环境。
验证安装:安装完成后,你可以通过在终端中输入以下命令来验证Pygame是否成功安装:
python -m pygame.examples.aliens
或者
python3 -m pygame.examples.aliens
如果一切顺利,你将会看到一个外星人射击游戏的窗口弹出。
第二步:实现思路:
场景实现:
游戏场景,场景的组成主要包括:石头墙、钢墙、地面、坦克、子弹地图。
石头墙:
石头墙是一种阻挡玩家和敌人行进路径的障碍物。可以使用矩形或图片来表示石头墙,并将其绘制在游戏界面的指定位置上。
# 石头墙
class Brick(pygame.sprite.Sprite):
def __init__(self, position):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.image.load('images/scene/brick.png').convert_alpha()
self.rect = self.image.get_rect()
self.rect.topleft = position
self.is_destroyed = False
def destroy(self):
self.is_destroyed = True
def draw(self, surface):
if not self.is_destroyed:
surface.blit(self.image, self.rect)
position 参数:用于指定石头墙在游戏界面上的位置。
is_destroyed 属性:用于标记石头墙是否被摧毁。
destroy() 方法:用于将石头墙标记为被摧毁。
draw() 方法:用于将石头墙绘制在游戏界面上。
钢墙:
钢墙是一种更坚固的墙体,通常比石头墙更难被破坏。与石头墙类似,可以使用矩形或图片来表示钢墙,并将其绘制在游戏界面上。
# 钢墙
class Iron(pygame.sprite.Sprite):
def __init__(self):
pygame.sprite.Sprite.__init__(self)
self.iron = pygame.image.load('images/scene/iron.png')
self.rect = self.iron.get_rect()
self.being = False
地面类(Grass)
草地是游戏中的一种地形,玩家可以在草地上自由行动。以下是一个简单的草地类定义:
import pygame
class Grass(pygame.sprite.Sprite):
def __init__(self, x, y, width=32, height=32):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.image.load('images/grass.gif').convert_alpha()
self.rect = self.image.get_rect()
self.rect.left, self.rect.top = x, y
self.width, self.height = width, height
def draw(self, surface):
surface.blit(self.image, self.rect)
在这个示例中,我们定义了一个 Grass 类,用于表示游戏中的草地。x 和 y 参数用于指定草地的位置,width 和 height 参数用于指定草地的宽度和高度。
draw() 方法用于将草地绘制在游戏界面上。
地图:
地图:地图是一个整体的场景,它包含了所有的游戏元素,并定义了玩家和敌人的移动范围。地图可以通过一个矩阵或类似的数据结构来表示,其中不同的值代表不同的场景元素(如石头墙、钢墙、地板等)。根据地图的定义,可以将相应的场景元素绘制在游戏界面上。
# 地图
class Map():
def __init__(self, stage):
self.brickGroup = pygame.sprite.Group()
self.ironGroup = pygame.sprite.Group()
self.iceGroup = pygame.sprite.Group()
self.riverGroup = pygame.sprite.Group()
self.treeGroup = pygame.sprite.Group()
if stage == 1:
self.stage1()
elif stage == 2:
self.stage2()
# 关卡
def stage1(self):
for x in [2, 3, 6, 7, 18, 19, 22, 23]:
for y in [2, 3, 4, 5, 6, 7, 8, 9, 10, 17, 18, 19, 20, 21, 22, 23]:
self.brick = Brick()
self.brick.rect.left, self.brick.rect.top = 3 + x * 24, 3 + y * 24
self.brick.being = True
self.brickGroup.add(self.brick)
for x in [10, 11, 14, 15]:
for y in [2, 3, 4, 5, 6, 7, 8, 11, 12, 15, 16, 17, 18, 19, 20]:
self.brick = Brick()
self.brick.rect.left, self.brick.rect.top = 3 + x * 24, 3 + y * 24
self.brick.being = True
self.brickGroup.add(self.brick)
for x in [4, 5, 6, 7, 18, 19, 20, 21]:
for y in [13, 14]:
self.brick = Brick()
self.brick.rect.left, self.brick.rect.top = 3 + x * 24, 3 + y * 24
self.brick.being = True
self.brickGroup.add(self.brick)
for x in [12, 13]:
for y in [16, 17]:
self.brick = Brick()
self.brick.rect.left, self.brick.rect.top = 3 + x * 24, 3 + y * 24
self.brick.being = True
self.brickGroup.add(self.brick)
for x, y in [(11, 23), (12, 23), (13, 23), (14, 23), (11, 24), (14, 24), (11, 25), (14, 25)]:
self.brick = Brick()
self.brick.rect.left, self.brick.rect.top = 3 + x * 24, 3 + y * 24
self.brick.being = True
self.brickGroup.add(self.brick)
for x, y in [(0, 14), (1, 14), (12, 6), (13, 6), (12, 7), (13, 7), (24, 14), (25, 14)]:
self.iron = Iron()
self.iron.rect.left, self.iron.rect.top = 3 + x * 24, 3 + y * 24
self.iron.being = True
self.ironGroup.add(self.iron)
第三步:坦克类的详细实现:
坦克类(Tank)
坦克是游戏中最主要的角色之一,玩家可以操控它来击败敌人。以下是一个简单的坦克类定义:
import pygame
class Tank(pygame.sprite.Sprite):
def __init__(self, x, y, speed=5):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.image.load('images/tank/player1.png').convert_alpha()
self.rect = self.image.get_rect()
self.rect.left, self.rect.top = x, y
self.speed = speed
self.direction = 'up'
self.is_alive = True
def move(self):
if self.direction == 'up':
self.rect.top -= self.speed
elif self.direction == 'down':
self.rect.top += self.speed
elif self.direction == 'left':
self.rect.left -= self.speed
elif self.direction == 'right':
self.rect.left += self.speed
def draw(self, surface):
surface.blit(self.image, self.rect)
在这个示例中,我们定义了一个 Tank 类,用于表示游戏中的坦克。x 和 y 参数用于指定坦克的初始位置,speed 参数用于指定坦克的移动速度。direction 属性表示坦克当前的朝向,is_alive 属性表示坦克是否存活。
move() 方法用于根据坦克的当前朝向,改变坦克的位置。draw() 方法用于将坦克绘制在游戏界面上。
子弹类(Bullet)
子弹是坦克和敌人所使用的武器。
子弹的主要属性包括:方向、速度、是否存活、是否为加强版等,代码实现如下:
# 子弹类
class Bullet(pygame.sprite.Sprite):
def __init__(self):
pygame.sprite.Sprite.__init__(self)
# 子弹四个方向(上下左右)
self.bullets = ['images/bullet/bullet_up.png', 'images/bullet/bullet_down.png', 'images/bullet/bullet_left.png', 'images/bullet/bullet_right.png']
# 子弹方向(默认向上)
self.direction_x, self.direction_y = 0, -1
self.bullet = pygame.image.load(self.bullets[0])
self.rect = self.bullet.get_rect()
# 在坦克类中再赋实际值
self.rect.left, self.rect.right = 0, 0
# 速度
self.speed = 6
# 是否存活
self.being = False
# 是否为加强版子弹(可碎钢板)
self.stronger = False
# 改变子弹方向
def turn(self, direction_x, direction_y):
self.direction_x, self.direction_y = direction_x, direction_y
if self.direction_x == 0 and self.direction_y == -1:
self.bullet = pygame.image.load(self.bullets[0])
elif self.direction_x == 0 and self.direction_y == 1:
self.bullet = pygame.image.load(self.bullets[1])
elif self.direction_x == -1 and self.direction_y == 0:
self.bullet = pygame.image.load(self.bullets[2])
elif self.direction_x == 1 and self.direction_y == 0:
self.bullet = pygame.image.load(self.bullets[3])
else:
raise ValueError('Bullet class -> direction value error.')
# 移动
def move(self):
self.rect = self.rect.move(self.speed*self.direction_x, self.speed*self.direction_y)
# 到地图边缘后消失
if (self.rect.top < 3) or (self.rect.bottom > 630 - 3) or (self.rect.left < 3) or (self.rect.right > 630 - 3):
self.being = False
爆炸类(Explosion)
爆炸是游戏中的一种特效,当坦克被击中或者墙壁被摧毁时会产生爆炸效果。以下是一个简单的爆炸类定义:
import pygame
class Explosion(pygame.sprite.Sprite):
def __init__(self, x, y):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.image.load('images/explosion.png').convert_alpha()
self.rect = self.image.get_rect()
self.rect.centerx, self.rect.centery = x, y
self.frame = 0
self.last_update = pygame.time.get_ticks()
self.frame_rate = 50
def update(self):
now = pygame.time.get_ticks()
if now - self.last_update > self.frame_rate:
self.frame += 1
if self.frame == 6:
self.kill()
else:
self.last_update = now
def draw(self, surface):
surface.blit(self.image, self.rect, pygame.Rect(self.frame * 64, 0, 64, 64))
在这个示例中,我们定义了一个 Explosion 类,用于表示游戏中的爆炸效果。x 和 y 参数用于指定爆炸的位置。
update() 方法用于更新爆炸动画的帧数。draw() 方法用于将当前帧的爆炸效果绘制在游戏界面上。
坦克类详细代码以及实现:
坦克包括我方坦克和敌方坦克,我方坦克由玩家自己控制移动、射击等操作,敌方坦克实现自动移动、射击等操作,代码实现如下:
# 我方坦克类
class myTank(pygame.sprite.Sprite):
def __init__(self, player):
pygame.sprite.Sprite.__init__(self)
# 玩家编号(1/2)
self.player = player
# 不同玩家用不同的坦克(不同等级对应不同的图)
if player == 1:
self.tanks = ['images/myTank/tank_T1_0.png', 'images/myTank/tank_T1_1.png', 'images/myTank/tank_T1_2.png']
elif player == 2:
self.tanks = ['images/myTank/tank_T2_0.png', 'images/myTank/tank_T2_1.png', 'images/myTank/tank_T2_2.png']
else:
raise ValueError('myTank class -> player value error.')
# 坦克等级(初始0)
self.level = 0
# 载入(两个tank是为了轮子特效)
self.tank = pygame.image.load(self.tanks[self.level]).convert_alpha()
self.tank_0 = self.tank.subsurface((0, 0), (48, 48))
self.tank_1 = self.tank.subsurface((48, 0), (48, 48))
self.rect = self.tank_0.get_rect()
# 保护罩
self.protected_mask = pygame.image.load('images/others/protect.png').convert_alpha()
self.protected_mask1 = self.protected_mask.subsurface((0, 0), (48, 48))
self.protected_mask2 = self.protected_mask.subsurface((48, 0), (48, 48))
# 坦克方向
self.direction_x, self.direction_y = 0, -1
# 不同玩家的出生位置不同
if player == 1:
self.rect.left, self.rect.top = 3 + 24 * 8, 3 + 24 * 24
elif player == 2:
self.rect.left, self.rect.top = 3 + 24 * 16, 3 + 24 * 24
else:
raise ValueError('myTank class -> player value error.')
# 坦克速度
self.speed = 3
# 是否存活
self.being = True
# 有几条命
self.life = 3
# 是否处于保护状态
self.protected = False
# 子弹
self.bullet = Bullet()
# 射击
def shoot(self):
self.bullet.being = True
self.bullet.turn(self.direction_x, self.direction_y)
if self.direction_x == 0 and self.direction_y == -1:
self.bullet.rect.left = self.rect.left + 20
self.bullet.rect.bottom = self.rect.top - 1
elif self.direction_x == 0 and self.direction_y == 1:
self.bullet.rect.left = self.rect.left + 20
self.bullet.rect.top = self.rect.bottom + 1
elif self.direction_x == -1 and self.direction_y == 0:
self.bullet.rect.right = self.rect.left - 1
self.bullet.rect.top = self.rect.top + 20
elif self.direction_x == 1 and self.direction_y == 0:
self.bullet.rect.left = self.rect.right + 1
self.bullet.rect.top = self.rect.top + 20
else:
raise ValueError('myTank class -> direction value error.')
if self.level == 0:
self.bullet.speed = 8
self.bullet.stronger = False
elif self.level == 1:
self.bullet.speed = 12
self.bullet.stronger = False
elif self.level == 2:
self.bullet.speed = 12
self.bullet.stronger = True
elif self.level == 3:
self.bullet.speed = 16
self.bullet.stronger = True
else:
raise ValueError('myTank class -> level value error.')
# 等级提升
def up_level(self):
if self.level < 3:
self.level += 1
try:
self.tank = pygame.image.load(self.tanks[self.level]).convert_alpha()
except:
self.tank = pygame.image.load(self.tanks[-1]).convert_alpha()
# 等级降低
def down_level(self):
if self.level > 0:
self.level -= 1
self.tank = pygame.image.load(self.tanks[self.level]).convert_alpha()
# 向上
def move_up(self, tankGroup, brickGroup, ironGroup, myhome):
self.direction_x, self.direction_y = 0, -1
# 先移动后判断
self.rect = self.rect.move(self.speed*self.direction_x, self.speed*self.direction_y)
self.tank_0 = self.tank.subsurface((0, 0), (48, 48))
self.tank_1 = self.tank.subsurface((48, 0), (48, 48))
# 是否可以移动
is_move = True
# 地图顶端
if self.rect.top < 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞石头/钢墙
if pygame.sprite.spritecollide(self, brickGroup, False, None) or \
pygame.sprite.spritecollide(self, ironGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞其他坦克
if pygame.sprite.spritecollide(self, tankGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 大本营
if pygame.sprite.collide_rect(self, myhome):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
return is_move
# 向下
def move_down(self, tankGroup, brickGroup, ironGroup, myhome):
self.direction_x, self.direction_y = 0, 1
# 先移动后判断
self.rect = self.rect.move(self.speed*self.direction_x, self.speed*self.direction_y)
self.tank_0 = self.tank.subsurface((0, 48), (48, 48))
self.tank_1 = self.tank.subsurface((48, 48), (48, 48))
# 是否可以移动
is_move = True
# 地图底端
if self.rect.bottom > 630 - 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞石头/钢墙
if pygame.sprite.spritecollide(self, brickGroup, False, None) or \
pygame.sprite.spritecollide(self, ironGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞其他坦克
if pygame.sprite.spritecollide(self, tankGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 大本营
if pygame.sprite.collide_rect(self, myhome):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
return is_move
# 向左
def move_left(self, tankGroup, brickGroup, ironGroup, myhome):
self.direction_x, self.direction_y = -1, 0
# 先移动后判断
self.rect = self.rect.move(self.speed*self.direction_x, self.speed*self.direction_y)
self.tank_0 = self.tank.subsurface((0, 96), (48, 48))
self.tank_1 = self.tank.subsurface((48, 96), (48, 48))
# 是否可以移动
is_move = True
# 地图左端
if self.rect.left < 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞石头/钢墙
if pygame.sprite.spritecollide(self, brickGroup, False, None) or \
pygame.sprite.spritecollide(self, ironGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞其他坦克
if pygame.sprite.spritecollide(self, tankGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 大本营
if pygame.sprite.collide_rect(self, myhome):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
return is_move
# 向右
def move_right(self, tankGroup, brickGroup, ironGroup, myhome):
self.direction_x, self.direction_y = 1, 0
# 先移动后判断
self.rect = self.rect.move(self.speed*self.direction_x, self.speed*self.direction_y)
self.tank_0 = self.tank.subsurface((0, 144), (48, 48))
self.tank_1 = self.tank.subsurface((48, 144), (48, 48))
# 是否可以移动
is_move = True
# 地图右端
if self.rect.right > 630 - 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞石头/钢墙
if pygame.sprite.spritecollide(self, brickGroup, False, None) or \
pygame.sprite.spritecollide(self, ironGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 撞其他坦克
if pygame.sprite.spritecollide(self, tankGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
# 大本营
if pygame.sprite.collide_rect(self, myhome):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
is_move = False
return is_move
# 死后重置
def reset(self):
self.level = 0
self.protected = False
self.tank = pygame.image.load(self.tanks[self.level]).convert_alpha()
self.tank_0 = self.tank.subsurface((0, 0), (48, 48))
self.tank_1 = self.tank.subsurface((48, 0), (48, 48))
self.rect = self.tank_0.get_rect()
self.direction_x, self.direction_y = 0, -1
if self.player == 1:
self.rect.left, self.rect.top = 3 + 24 * 8, 3 + 24 * 24
elif self.player == 2:
self.rect.left, self.rect.top = 3 + 24 * 16, 3 + 24 * 24
else:
raise ValueError('myTank class -> player value error.')
self.speed = 3
# 敌方坦克类
class enemyTank(pygame.sprite.Sprite):
def __init__(self, x=None, kind=None, is_red=None):
pygame.sprite.Sprite.__init__(self)
# 用于给刚生成的坦克播放出生特效
self.born = True
self.times = 90
# 坦克的种类编号
if kind is None:
self.kind = random.randint(0, 3)
else:
self.kind = kind
# 所有坦克
self.tanks1 = ['images/enemyTank/enemy_1_0.png', 'images/enemyTank/enemy_1_1.png', 'images/enemyTank/enemy_1_2.png', 'images/enemyTank/enemy_1_3.png']
self.tanks2 = ['images/enemyTank/enemy_2_0.png', 'images/enemyTank/enemy_2_1.png', 'images/enemyTank/enemy_2_2.png', 'images/enemyTank/enemy_2_3.png']
self.tanks3 = ['images/enemyTank/enemy_3_0.png', 'images/enemyTank/enemy_3_1.png', 'images/enemyTank/enemy_3_2.png', 'images/enemyTank/enemy_3_3.png']
self.tanks4 = ['images/enemyTank/enemy_4_0.png', 'images/enemyTank/enemy_4_1.png', 'images/enemyTank/enemy_4_2.png', 'images/enemyTank/enemy_4_3.png']
self.tanks = [self.tanks1, self.tanks2, self.tanks3, self.tanks4]
# 是否携带食物(红色的坦克携带食物)
if is_red is None:
self.is_red = random.choice((True, False, False, False, False))
else:
self.is_red = is_red
# 同一种类的坦克具有不同的颜色, 红色的坦克比同类坦克多一点血量
if self.is_red:
self.color = 3
else:
self.color = random.randint(0, 2)
# 血量
self.blood = self.color
# 载入(两个tank是为了轮子特效)
self.tank = pygame.image.load(self.tanks[self.kind][self.color]).convert_alpha()
self.tank_0 = self.tank.subsurface((0, 48), (48, 48))
self.tank_1 = self.tank.subsurface((48, 48), (48, 48))
self.rect = self.tank_0.get_rect()
# 坦克位置
if x is None:
self.x = random.randint(0, 2)
else:
self.x = x
self.rect.left, self.rect.top = 3 + self.x * 12 * 24, 3
# 坦克是否可以行动
self.can_move = True
# 坦克速度
self.speed = max(3 - self.kind, 1)
# 方向
self.direction_x, self.direction_y = 0, 1
# 是否存活
self.being = True
# 子弹
self.bullet = Bullet()
# 射击
def shoot(self):
self.bullet.being = True
self.bullet.turn(self.direction_x, self.direction_y)
if self.direction_x == 0 and self.direction_y == -1:
self.bullet.rect.left = self.rect.left + 20
self.bullet.rect.bottom = self.rect.top - 1
elif self.direction_x == 0 and self.direction_y == 1:
self.bullet.rect.left = self.rect.left + 20
self.bullet.rect.top = self.rect.bottom + 1
elif self.direction_x == -1 and self.direction_y == 0:
self.bullet.rect.right = self.rect.left - 1
self.bullet.rect.top = self.rect.top + 20
elif self.direction_x == 1 and self.direction_y == 0:
self.bullet.rect.left = self.rect.right + 1
self.bullet.rect.top = self.rect.top + 20
else:
raise ValueError('enemyTank class -> direction value error.')
# 随机移动
def move(self, tankGroup, brickGroup, ironGroup, myhome):
self.rect = self.rect.move(self.speed*self.direction_x, self.speed*self.direction_y)
is_move = True
if self.direction_x == 0 and self.direction_y == -1:
self.tank_0 = self.tank.subsurface((0, 0), (48, 48))
self.tank_1 = self.tank.subsurface((48, 0), (48, 48))
if self.rect.top < 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
self.direction_x, self.direction_y = random.choice(([0, 1], [0, -1], [1, 0], [-1, 0]))
is_move = False
elif self.direction_x == 0 and self.direction_y == 1:
self.tank_0 = self.tank.subsurface((0, 48), (48, 48))
self.tank_1 = self.tank.subsurface((48, 48), (48, 48))
if self.rect.bottom > 630 - 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
self.direction_x, self.direction_y = random.choice(([0, 1], [0, -1], [1, 0], [-1, 0]))
is_move = False
elif self.direction_x == -1 and self.direction_y == 0:
self.tank_0 = self.tank.subsurface((0, 96), (48, 48))
self.tank_1 = self.tank.subsurface((48, 96), (48, 48))
if self.rect.left < 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
self.direction_x, self.direction_y = random.choice(([0, 1], [0, -1], [1, 0], [-1, 0]))
is_move = False
elif self.direction_x == 1 and self.direction_y == 0:
self.tank_0 = self.tank.subsurface((0, 144), (48, 48))
self.tank_1 = self.tank.subsurface((48, 144), (48, 48))
if self.rect.right > 630 - 3:
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
self.direction_x, self.direction_y = random.choice(([0, 1], [0, -1], [1, 0], [-1, 0]))
is_move = False
else:
raise ValueError('enemyTank class -> direction value error.')
if pygame.sprite.spritecollide(self, brickGroup, False, None) \
or pygame.sprite.spritecollide(self, ironGroup, False, None) \
or pygame.sprite.spritecollide(self, tankGroup, False, None):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
self.direction_x, self.direction_y = random.choice(([0, 1], [0, -1], [1, 0], [-1, 0]))
is_move = False
if pygame.sprite.collide_rect(self, myhome):
self.rect = self.rect.move(self.speed*-self.direction_x, self.speed*-self.direction_y)
self.direction_x, self.direction_y = random.choice(([0, 1], [0, -1], [1, 0], [-1, 0]))
is_move = False
return is_move
# 重新载入坦克
def reload(self):
self.tank = pygame.image.load(self.tanks[self.kind][self.color]).convert_alpha()
self.tank_0 = self.tank.subsurface((0, 48), (48, 48))
self.tank_1 = self.tank.subsurface((48, 48), (48, 48))
完整代码压缩包:
由于代码内容过多就不一一展示放在文章当中了,如需完整项目请私信…