upload组件的参数属性
action | 必选参数,上传的地址 | string | — | — |
headers | 设置上传的请求头部 | object | — | — |
multiple | 是否支持多选文件 | boolean | — | — |
data | 上传时附带的额外参数 | object | — | — |
name | 上传的文件字段名 | string | — | file |
with-credentials | 支持发送 cookie 凭证信息 | boolean | — | false |
show-file-list | 是否显示已上传文件列表 | boolean | — | true |
drag | 是否启用拖拽上传 | boolean | — | false |
accept | 接受上传的文件类型(thumbnail-mode 模式下此参数无效) | string | — | — |
on-preview | 点击文件列表中已上传的文件时的钩子 | function(file) | — | — |
on-remove | 文件列表移除文件时的钩子 | function(file, fileList) | — | — |
on-success | 文件上传成功时的钩子 | function(response, file, fileList) | — | — |
on-error | 文件上传失败时的钩子 | function(err, file, fileList) | — | — |
on-progress | 文件上传时的钩子 | function(event, file, fileList) | — | — |
on-change | 文件状态改变时的钩子,添加文件、上传成功和上传失败时都会被调用 | function(file, fileList) | — | — |
before-upload | 上传文件之前的钩子,参数为上传的文件,若返回 false 或者返回 Promise 且被 reject,则停止上传。 | function(file) | — | — |
before-remove | 删除文件之前的钩子,参数为上传的文件和文件列表,若返回 false 或者返回 Promise 且被 reject,则停止删除。 | function(file, fileList) | — | — |
list-type | 文件列表的类型 | string | text/picture/picture-card | text |
auto-upload | 是否在选取文件后立即进行上传 | boolean | — | true |
file-list | 上传的文件列表, 例如: [{name: 'food.jpg', url: 'https://xxx.cdn.com/xxx.jpg'}] | array | — | [] |
http-request | 覆盖默认的上传行为,可以自定义上传的实现 | function | — | — |
disabled | 是否禁用 | boolean | — | false |
limit | 最大允许上传个数 | number | — | — |
on-exceed | 文件超出个数限制时的钩子 | function(files, fileList) | — | - |
常用的也只有下面这几个
multiple :是否支持多选文件,
show-file-list :是否显示已上传文件列表,
drag :是否启用拖拽上传,
accept :接受上传的文件类型(thumbnail-mode 模式下此参数无效),
on-preview :点击文件列表中已上传的文件时的钩子(查看文件),
on-remove :文件列表移除文件时的钩子(删除文件),
on-success :文件上传成功时的钩子,
on-progress :文件上传时的钩子,
before-upload :检查文件用的,
list-type :文件列表的类型,
auto-upload :是否选择了文件立即上传,一般默认立即上传,
扫描二维码关注公众号,回复:
16860039 查看本文章
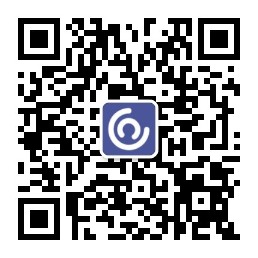
file-list:文件列表,用来展示上传的文件,后面用来回显文件或图片有用(有固定的格式),
http-request :自定义上传,会覆盖默认的action上传,最常用,
limit :允许上传的最大格式
upload组件的方法
方法名 | 说明 | 参数 |
---|---|---|
clearFiles | 清空已上传的文件列表(该方法不支持在 before-upload 中调用) | — |
abort | 取消上传请求 | ( file: fileList 中的 file 对象 ) |
submit | 手动上传文件列表 | — |
clearFiles :最常用
代码:(组件)
<template>
<div>
<el-upload ref="upload" :file-list="imgList" action="#" accept=".jpg,.jpeg,.png,.JPG,.JPEG" :http-request="handleFileUpload" list-type="picture-card" :on-preview="handlePictureCardPreview" :on-remove="handleRemove" :on-change="onChange" :before-upload="handleBeforeUpload">
<i class="el-icon-plus" />
</el-upload>
<!-- 点击图片查看大图 -->
<el-dialog :visible.sync="dialogVisible">
<img width="100%" :src="dialogImageUrl" alt="" />
</el-dialog>
</div>
</template>
<script>
//
import { videoApi } from '@/api/video.js'
// 上传文件的基路径前缀,上传文件的接口会返回一个路径,用来和https://yztest.zjrcq.cn/eyeValleyImg拼接
import { baseUrl } from '@/utils/pictureUrl'
export default {
name: '',
props: {
// 图片列表,用来实现回显,列表格式必须为[{ name: 'placard.jpeg', url: 'https://......' }]
imgList: {
default: '',
type: Array
}
},
data() {
return {
// 点击查看图片的路径
dialogImageUrl: '',
dialogVisible: false,
// 文件列表
fileList: [],
// 上传的数据
initForm: {
id: '',
picPath: '',
removed: false
},
showBtnDealImg: true,
noneBtnImg: false,
limitCountImg: 1 // 上传图片的最大数量
}
},
mounted() {},
methods: {
// 上传海报图
handleFileUpload(fileObject) {
videoApi.uploadImg(fileObject.file).then(res => {
const { msg, result } = res
if (result == 1) {
// 将图片地址拼接传给父组件
this.$emit('placardUpload', baseUrl + msg)
} else {
this.$message.warning(msg)
}
})
},
// 清空文件列表的方法,供父组件使用
clearFiles() {
this.$refs.upload.clearFiles()
},
// 移除
handleRemove(file, fileList) {
console.log(file, fileList)
this.$emit('placardUpload', fileList.join(''))
},
// 查看大图
handlePictureCardPreview(file) {
this.dialogImageUrl = file.url
this.dialogVisible = true
},
// 检查图片
handleBeforeUpload(file) {
const isLt2M = file.size / 1024 / 1024 < 2
if (!isLt2M) {
this.$message.warning('上传图片不能大于2M')
}
return true
},
onChange(file, fileList) {}
}
}
</script>
<style lang="scss">
.disUoloadSty .el-upload--picture-card {
display: none; /* 上传按钮隐藏 */
}
</style>
在使用的.vue文件中使用
<el-form-item label="海报图">
<placardUpload ref="placardUpload" :img-list="placardFileList" @placardUpload="placardUpload" />
</el-form-item>
placardFileList :为了实现图片回显的功能
placardUpload :接收子组件传过来的数据