原题
HRY and Abbas Problem Description
In a parallel universe, HRY is the president of the Kassel Academy Student Union. Once he and the Lionheart Association President Abdullah Abbas performed a secret mission: to investigate the mixed blood drug trafficking group in country X.
However, an accident happened and they were caught together. They were forced to play Russian gambling roulette games!
The terrorists brought a revolver which works as follows: a revolver has n places 1,2,…,n to place bullet, and these n places forms a circle. The revolver has a position it is currently pointing at, and when pulling the trigger, the revolver will shoot out the bullet (if it has) at the current position and turn to the next position. The next position of1 ≤ i ≤ n-1
is n+1, and for n the next position is 1. Now the terrorist puts m bullets in the revolver, and turned the wheel of the revolver, which means the starting position is randomly chosen. He orders HRY and Abbas shoot at their heads in turn using the same revolver. If someone is hit by bullet, then the game is over.
HRY and Abbas are both not ordinary mixed blood, so the revolver can do no harm to them. However, they still decided to play the game. Now they want to know the probability of the game ending exactly after the ith shoot.input
The first line is an integer T, indicating the number of test cases.
For each test case there are two lines :
The first line contains two integers n,m, and the second line contains m different positive integers ai, indicating the initial positions of bullets.
1 ≤ T ≤ 1000, 1 ≤ m ≤ n ≤ 105, 1 ≤ ai ≤ n
The sum of n does not exceed 106ouput
For each test case output n lines, the ith line indicates the probability of game ending exactly after the ith shoot. The probability is in the form of irreducible fraction p/q, which means the greatest common divisor of p and q is 1. If the answer is 0, output 0 directly.
Sample Input
2
10 2
1 3
10 4
1 2 6 10Sample Output
1/5
1/5
1/10
1/10
1/10
1/10
1/10
1/10
0
0
2/5
1/5
1/5
1/5
0
0
0
0
0
0
题意分析:
题目的意思是,有一把类似于左轮的枪(弹夹是环形的),最多有n个位置(位置编号从1开始)可以装子弹,其中有m个位置是有子弹的,其余位置是没有子弹的,现在随机选一个位置开始,不断地让大家轮流开枪,直到第一个人死亡时,游戏结束。输入第一行是样例个数,以下一行是n m,接下来是m个数,代表m个有子弹的位置。要求输出n行,分别表示第一枪就结束游戏的概率,第二枪结束的概率。。。第n枪结束的概率。
ps:当概率为1时,输出1/1
总体思路:
仔细分析,问题其实十分简单。
现在用第一个样例输入说明思路:
现在有子弹的分布如下图所示:(第一行代表位置标号,第二行代表弹夹,黄色代表有子弹,绿色无子弹)
首先我们定义一个数组a,数组表示从第i个位置开始,要打多少枪结束游戏。
观察上图,我们发现,从第一个位置开始只要打一枪就结束游戏;从第二个位置开始要经历第2,3个位置结束游戏;从第三个位置开始则打一枪就结束游戏;从第四个位置开始则要经历4,5,6,7,8,9,10,1一共8枪才结束游戏。。。
因此我们的数组a就确认了。
最后我们再定义一个数组cnt,用来统计第一枪结束的情况个数,第二枪结束的情况个数。。。。第n枪结束的情况个数。最后,根据a数组,可以导出cnt数组。
到此,答案已经出来了,即分别将cnt数组每一项除以n输出后就是答案。
仔细想想,到这里其实还有一个小问题,就是如何快速确认我们的a数组,如何可以快速知道从当前位置开始要打多少枪结束游戏?其实我们可以建立一个next数组,记录离第i个位置最近的右边(因为可以循环,所以并不是严格的右边,而是逻辑的右边)的位置,这样就可以轻松算出当前位置需要打多少枪结束。
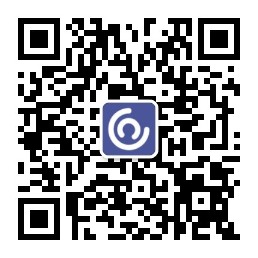
AC代码:
ps:题目没有说给出的m个位置是有序的,因此在建立next数组时,要先sort。
#include <iostream>
#include <algorithm>
#include <cstdio>
#define MAXN 100005
using namespace std;
typedef long long ll;
int Next[MAXN] = {
0};
ll cnt[MAXN] = {
0};
int n, m;
int f(int a, int b){
if (a > b){
return b+n-a+1;
}else{
return b-a+1;
}
}
ll gcd (ll x, ll y){
return y == 0 ? x : gcd(y, x%y);
}
void output(ll a, ll b){
if (a == 0){
printf("0\n");
return ;
}
ll g = gcd(a, b);
a /= g;
b /= g;
printf("%lld/%lld\n", a, b);
}
int main(){
int i, j, x, T;
scanf ("%d", &T);
while (T--){
for (i = 0; i < MAXN; i++){
Next[i] = cnt[i] = 0;
}
scanf ("%d%d", &n, &m);
ll temp[MAXN] = {
0};
for (j = 0; j < m; j++){
scanf ("%d", &x);
temp[j] = x;
}
sort(temp, temp+m);
if (m == 1){
for (i = 1; i <= n; i++){
output(1, n);
}
continue;
}else{
for (j = 0; j < m; j++){
ll left = temp[j], right = temp[(j+1)%m];
ll len = f(left, right);
Next[left] = left;
Next[right] = right;
for (i = 0; i < len-2; i++){
if (!Next[(i+left)%n+1]){
Next[(i+left)%n+1] = right;
}
}
}
}
for (i = 1; i <= n; i++){
cnt[f(i, Next[i])]++;
}
ll sum = 0;
for (i = 1; i <= n; i++){
sum += cnt[i];
}
for (i = 1; i <= n; i++){
output(cnt[i], sum);
}
}
return 0;
}