1. 输入日期查询年龄
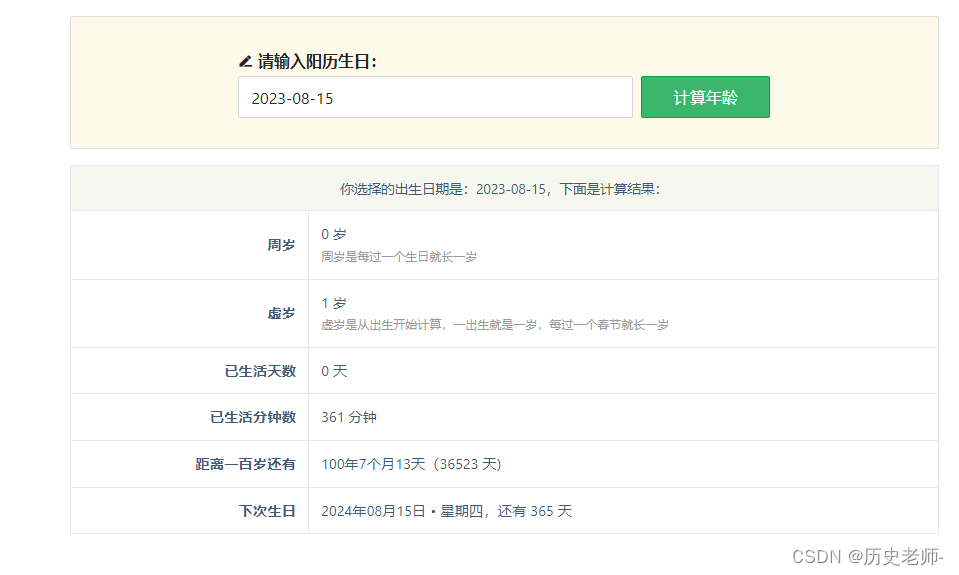
2. php laravel框架实现 代码
/**
* 在线年龄计算器
*/
public function ageDateCal()
{
// 输入的生日时间
$birthday = $this->request('birthday');
// 当前时间
$currentDate = date('Y-m-d');
// 计算周岁
$age = date_diff(date_create($birthday), date_create($currentDate))->y;
// 计算虚岁
$nominalAge = $age + 1;
// 计算已生活天数
$days = date_diff(date_create($birthday), date_create($currentDate))->days;
// 计算已生活分钟数
// 获取当前时间戳
$currentTimestamp = time();
// 转换日期字符串为时间戳
$inputTimestamp = strtotime($birthday);
// 计算时间差(以分钟为单位)
$minutes = round(abs($currentTimestamp - $inputTimestamp) / 60);
// 计算距离一百岁还有多少年多少月多少日合计多少天
$hundredYears = date("Y-m-d H:i:s", strtotime("$birthday+100year"));
$diffDate = $this->diffDate($currentDate, $hundredYears);
$remainingYears = $diffDate['y'];
$remainingMonths = $diffDate['m'];
$remainingDays = $diffDate['d'];
$remainingTotalDays = $diffDate['a'];
// 计算下次生日的日期和天数
$nextBirthday = date('Y-m-d', strtotime($birthday . ' + ' . ($age + 1) . ' years'));
$daysToNextBirthday = date_diff(date_create($currentDate), date_create($nextBirthday))->days;
$week = $this->getWeekDay($nextBirthday);
$data = [
'age' => $age,
'nominalAge' => $nominalAge,
'days' => $days,
'minutes' => $minutes,
'remainingYears' => $remainingYears,
'remainingMonths' => $remainingMonths,
'remainingDays' => $remainingDays,
'remainingTotalDays' => $remainingTotalDays,
'nextBirthday' => $nextBirthday,
'week' => $week,
'daysToNextBirthday' => $daysToNextBirthday,
];
return $this->jsonSuc(['result' => $data]);
}
/**
* 判断星期几
* @param $time
* @return string
*/
public function getWeekDay($time)
{
$week_array = ['日', '一', '二', '三', '四', '五', '六'];
$week = date("w", strtotime($time));
return '星期' . $week_array[$week];
}
/**
* function:计算两个日期相隔多少年,多少月,多少天
* param string $date1[格式如:2020-11-5]
* param string $date2[格式如:2023-12-01]
* return array array('年','月','日');
*/
function diffDate($date1, $date2)
{
$datetime1 = new \DateTime($date1);
$datetime2 = new \DateTime($date2);
$interval = $datetime1->diff($datetime2);
$time['y'] = $interval->format('%Y');
$time['m'] = $interval->format('%m');
$time['d'] = $interval->format('%d');
$time['h'] = $interval->format('%H');
$time['i'] = $interval->format('%i');
$time['s'] = $interval->format('%s');
$time['a'] = $interval->format('%a'); // 两个时间相差总天数
return $time;
}