基本数据类型
1.数字
int(整型)
在32位机器上,整数的位数是32位,取值范围是-2**31~2--31-1
在64位系统上,整数的位数是64位,取值范围是-2**63~2**63-1

1 class int(object): 2 """ 3 int(x=0) -> integer 4 int(x, base=10) -> integer 5 6 Convert a number or string to an integer, or return 0 if no arguments 7 are given. If x is a number, return x.__int__(). For floating point 8 numbers, this truncates towards zero. 9 10 If x is not a number or if base is given, then x must be a string, 11 bytes, or bytearray instance representing an integer literal in the 12 given base. The literal can be preceded by '+' or '-' and be surrounded 13 by whitespace. The base defaults to 10. Valid bases are 0 and 2-36. 14 Base 0 means to interpret the base from the string as an integer literal. 15 >>> int('0b100', base=0) 16 4 17 """ 18 def bit_length(self): 19 """ 20 返回表示该数字的时候占用的最小位数 21 """ 22 return 0 23 24 def conjugate(self, *args, **kwargs): 25 """ 返回该复数的共轭复数 """ 26 pass 27 28 @classmethod # known case 29 def from_bytes(cls, bytes, byteorder, *args, **kwargs): # real signature unknown; NOTE: unreliably restored from __doc__ 30 pass 31 32 def to_bytes(self, length, byteorder, *args, **kwargs): # real signature unknown; NOTE: unreliably restored from __doc__ 33 pass 34 35 def __abs__(self, *args, **kwargs): # real signature unknown 36 """ 返回绝对值 """ 37 pass 38 39 def __add__(self, *args, **kwargs): # real signature unknown 40 """ Return self+value. """ 41 pass 42 43 def __and__(self, *args, **kwargs): # real signature unknown 44 """ Return self&value. """ 45 pass 46 47 def __bool__(self, *args, **kwargs): # real signature unknown 48 """ self != 0 """ 49 pass 50 51 def __ceil__(self, *args, **kwargs): # real signature unknown 52 """ Ceiling of an Integral returns itself. """ 53 pass 54 55 def __divmod__(self, *args, **kwargs): # real signature unknown 56 """ 相除,的得到商和余数组成的元组 """ 57 pass 58 59 def __eq__(self, *args, **kwargs): # real signature unknown 60 """ Return self==value. """ 61 pass 62 63 def __float__(self, *args, **kwargs): # real signature unknown 64 """ 转换位浮点数 """ 65 pass 66 67 def __floordiv__(self, *args, **kwargs): # real signature unknown 68 """ Return self//value. """ 69 pass 70 71 def __floor__(self, *args, **kwargs): # real signature unknown 72 """ Flooring an Integral returns itself. """ 73 pass 74 75 def __format__(self, *args, **kwargs): # real signature unknown 76 pass 77 78 def __getattribute__(self, *args, **kwargs): # real signature unknown 79 """ Return getattr(self, name). """ 80 pass 81 82 def __getnewargs__(self, *args, **kwargs): # real signature unknown 83 ''' 内部调用__new__方法或创建对象时传入参数使用 ''' 84 pass 85 86 def __ge__(self, *args, **kwargs): # real signature unknown 87 """ Return self>=value. """ 88 pass 89 90 def __gt__(self, *args, **kwargs): # real signature unknown 91 """ Return self>value. """ 92 pass 93 94 def __hash__(self, *args, **kwargs): # real signature unknown 95 """ 如果对象object位哈希表类型,返回对象object的哈希值。哈希值为整数。在字典查找中, 96 哈希值用于快速比较字典的键。两个数值相等,则哈希值也相等 97 """ 98 pass 99 100 def __index__(self, *args, **kwargs): # real signature unknown 101 """ 用于切片 """ 102 pass 103 104 def __init__(self, x, base=10): # known special case of int.__init__ 105 """ 106 int(x=0) -> integer 107 int(x, base=10) -> integer 108 109 Convert a number or string to an integer, or return 0 if no arguments 110 are given. If x is a number, return x.__int__(). For floating point 111 numbers, this truncates towards zero. 112 113 If x is not a number or if base is given, then x must be a string, 114 bytes, or bytearray instance representing an integer literal in the 115 given base. The literal can be preceded by '+' or '-' and be surrounded 116 by whitespace. The base defaults to 10. Valid bases are 0 and 2-36. 117 Base 0 means to interpret the base from the string as an integer literal. 118 >>> int('0b100', base=0) 119 4 120 # (copied from class doc) 121 """ 122 pass 123 124 def __int__(self, *args, **kwargs): # real signature unknown 125 """ 转化为整数 """ 126 pass 127 128 def __invert__(self, *args, **kwargs): # real signature unknown 129 """ ~self """ 130 pass 131 132 def __le__(self, *args, **kwargs): # real signature unknown 133 """ Return self<=value. """ 134 pass 135 136 def __lshift__(self, *args, **kwargs): # real signature unknown 137 """ Return self<<value. """ 138 pass 139 140 def __lt__(self, *args, **kwargs): # real signature unknown 141 """ Return self<value. """ 142 pass 143 144 def __mod__(self, *args, **kwargs): # real signature unknown 145 """ Return self%value. """ 146 pass 147 148 def __mul__(self, *args, **kwargs): # real signature unknown 149 """ Return self*value. """ 150 pass 151 152 def __neg__(self, *args, **kwargs): # real signature unknown 153 """ -self """ 154 pass 155 156 @staticmethod # known case of __new__ 157 def __new__(*args, **kwargs): # real signature unknown 158 """ Create and return a new object. See help(type) for accurate signature. """ 159 pass 160 161 def __ne__(self, *args, **kwargs): # real signature unknown 162 """ Return self!=value. """ 163 pass 164 165 def __or__(self, *args, **kwargs): # real signature unknown 166 """ Return self|value. """ 167 pass 168 169 def __pos__(self, *args, **kwargs): # real signature unknown 170 """ +self """ 171 pass 172 173 def __pow__(self, *args, **kwargs): # real signature unknown 174 """ 幂,次方 """ 175 pass 176 177 def __radd__(self, *args, **kwargs): # real signature unknown 178 """ Return value+self. """ 179 pass 180 181 def __rand__(self, *args, **kwargs): # real signature unknown 182 """ Return value&self. """ 183 pass 184 185 def __rdivmod__(self, *args, **kwargs): # real signature unknown 186 """ Return divmod(value, self). """ 187 pass 188 189 def __repr__(self, *args, **kwargs): # real signature unknown 190 """ 转化为解释器可读取的形式 """ 191 pass 192 193 def __rfloordiv__(self, *args, **kwargs): # real signature unknown 194 """ Return value//self. """ 195 pass 196 197 def __rlshift__(self, *args, **kwargs): # real signature unknown 198 """ Return value<<self. """ 199 pass 200 201 def __rmod__(self, *args, **kwargs): # real signature unknown 202 """ Return value%self. """ 203 pass 204 205 def __rmul__(self, *args, **kwargs): # real signature unknown 206 """ Return value*self. """ 207 pass 208 209 def __ror__(self, *args, **kwargs): # real signature unknown 210 """ Return value|self. """ 211 pass 212 213 def __round__(self, *args, **kwargs): # real signature unknown 214 """ 215 Rounding an Integral returns itself. 216 Rounding with an ndigits argument also returns an integer. 217 """ 218 pass 219 220 def __rpow__(self, *args, **kwargs): # real signature unknown 221 """ Return pow(value, self, mod). """ 222 pass 223 224 def __rrshift__(self, *args, **kwargs): # real signature unknown 225 """ Return value>>self. """ 226 pass 227 228 def __rshift__(self, *args, **kwargs): # real signature unknown 229 """ Return self>>value. """ 230 pass 231 232 def __rsub__(self, *args, **kwargs): # real signature unknown 233 """ Return value-self. """ 234 pass 235 236 def __rtruediv__(self, *args, **kwargs): # real signature unknown 237 """ Return value/self. """ 238 pass 239 240 def __rxor__(self, *args, **kwargs): # real signature unknown 241 """ Return value^self. """ 242 pass 243 244 def __sizeof__(self, *args, **kwargs): # real signature unknown 245 """ Returns size in memory, in bytes """ 246 pass 247 248 def __str__(self, *args, **kwargs): # real signature unknown 249 """ 转化为阅读的形式,如果没有适合于人阅读的形式则返回解释器阅读的形式 """ 250 pass 251 252 def __sub__(self, *args, **kwargs): # real signature unknown 253 """ Return self-value. """ 254 pass 255 256 def __truediv__(self, *args, **kwargs): # real signature unknown 257 """ Return self/value. """ 258 pass 259 260 def __trunc__(self, *args, **kwargs): # real signature unknown 261 """ 返回数值被截取为整形的值,在整数中无意义 """ 262 pass 263 264 def __xor__(self, *args, **kwargs): # real signature unknown 265 """ Return self^value. """ 266 pass 267 268 denominator = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 269 """分母 = 1""" 270 271 imag = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 272 """虚数,无意义""" 273 274 numerator = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 275 """分子 = 数字大小""" 276 277 real = property(lambda self: object(), lambda self, v: None, lambda self: None) # default 278 """实数,无意义"""
数值的类型:
2.布尔值
真或假
1 或 0
扫描二维码关注公众号,回复:
1654372 查看本文章
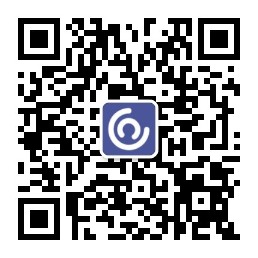
3.字符串
”hello python“ 一个标准的字符串
字符串的常用操作:
- 移除空白
- 分割
- 长度
- 索引
- 切片

4.列表
创建列表:
1 list1 = ['1','2',3] 2 3 list2 = list( ['1','2',3] )
基本操作:
- 索引
- 切片
- 追加
- 删除
- 长度
- 切片
- 循环
- 包含

1 class list(object): 2 """ 3 list() -> new empty list 4 list(iterable) -> new list initialized from iterable's items 5 """ 6 def append(self, p_object): 7 """ list添加数据的常用方法 """ 8 pass 9 10 def clear(self): # real signature unknown; restored from __doc__ 11 """ L.clear() -> None -- remove all items from L """ 12 pass 13 14 def copy(self): # real signature unknown; restored from __doc__ 15 """ L.copy() -> list -- a shallow copy of L """ 16 return [] 17 18 def count(self, value): # real signature unknown; restored from __doc__ 19 """ 计数 """ 20 return 0 21 22 def extend(self, iterable): # real signature unknown; restored from __doc__ 23 """ L.extend(iterable) -> None -- extend list by appending elements from the iterable """ 24 pass 25 26 def index(self, value, start=None, stop=None): # real signature unknown; restored from __doc__ 27 """ 28 L.index(value, [start, [stop]]) -> integer -- return first index of value. 29 Raises ValueError if the value is not present. 30 """ 31 return 0 32 33 def insert(self, index, p_object): # real signature unknown; restored from __doc__ 34 """ L.insert(index, object) -- insert object before index """ 35 pass 36 37 def pop(self, index=None): # real signature unknown; restored from __doc__ 38 """ 39 弹出 40 """ 41 pass 42 43 def remove(self, value): # real signature unknown; restored from __doc__ 44 """ 45 移除 46 """ 47 pass 48 49 def reverse(self): # real signature unknown; restored from __doc__ 50 """ L.reverse() -- reverse *IN PLACE* """ 51 pass 52 53 def sort(self, key=None, reverse=False): # real signature unknown; restored from __doc__ 54 """ L.sort(key=None, reverse=False) -> None -- stable sort *IN PLACE* """ 55 pass 56 57 def __add__(self, *args, **kwargs): # real signature unknown 58 """ Return self+value. """ 59 pass 60 61 def __contains__(self, *args, **kwargs): # real signature unknown 62 """ Return key in self. """ 63 pass 64 65 def __delitem__(self, *args, **kwargs): # real signature unknown 66 """ Delete self[key]. """ 67 pass 68 69 def __eq__(self, *args, **kwargs): # real signature unknown 70 """ Return self==value. """ 71 pass 72 73 def __getattribute__(self, *args, **kwargs): # real signature unknown 74 """ Return getattr(self, name). """ 75 pass 76 77 def __getitem__(self, y): # real signature unknown; restored from __doc__ 78 """ x.__getitem__(y) <==> x[y] """ 79 pass 80 81 def __ge__(self, *args, **kwargs): # real signature unknown 82 """ Return self>=value. """ 83 pass 84 85 def __gt__(self, *args, **kwargs): # real signature unknown 86 """ Return self>value. """ 87 pass 88 89 def __iadd__(self, *args, **kwargs): # real signature unknown 90 """ Implement self+=value. """ 91 pass 92 93 def __imul__(self, *args, **kwargs): # real signature unknown 94 """ Implement self*=value. """ 95 pass 96 97 def __init__(self, seq=()): # known special case of list.__init__ 98 """ 99 list() -> new empty list 100 list(iterable) -> new list initialized from iterable's items 101 # (copied from class doc) 102 """ 103 pass 104 105 def __iter__(self, *args, **kwargs): # real signature unknown 106 """ Implement iter(self). """ 107 pass 108 109 def __len__(self, *args, **kwargs): # real signature unknown 110 """ Return len(self). """ 111 pass 112 113 def __le__(self, *args, **kwargs): # real signature unknown 114 """ Return self<=value. """ 115 pass 116 117 def __lt__(self, *args, **kwargs): # real signature unknown 118 """ Return self<value. """ 119 pass 120 121 def __mul__(self, *args, **kwargs): # real signature unknown 122 """ Return self*value.n """ 123 pass 124 125 @staticmethod # known case of __new__ 126 def __new__(*args, **kwargs): # real signature unknown 127 """ Create and return a new object. See help(type) for accurate signature. """ 128 pass 129 130 def __ne__(self, *args, **kwargs): # real signature unknown 131 """ Return self!=value. """ 132 pass 133 134 def __repr__(self, *args, **kwargs): # real signature unknown 135 """ Return repr(self). """ 136 pass 137 138 def __reversed__(self): # real signature unknown; restored from __doc__ 139 """ L.__reversed__() -- return a reverse iterator over the list """ 140 pass 141 142 def __rmul__(self, *args, **kwargs): # real signature unknown 143 """ Return self*value. """ 144 pass 145 146 def __setitem__(self, *args, **kwargs): # real signature unknown 147 """ Set self[key] to value. """ 148 pass 149 150 def __sizeof__(self): # real signature unknown; restored from __doc__ 151 """ L.__sizeof__() -- size of L in memory, in bytes """ 152 pass 153 154 __hash__ = None
5.元组
创建元组:
1 set1 = (1,2,3,) 2 3 set2 = tuple((1,2,3,))
基本操作:
- 索引
- 切片
- 循环
- 长度
- 包含

1 class set(object): 2 """类似于list,set内部不允许有重复的元素""" 3 """ 4 set() -> new empty set object 5 set(iterable) -> new set object 6 7 Build an unordered collection of unique elements. 8 """ 9 def add(self, *args, **kwargs): # real signature unknown 10 """ 11 Add an element to a set. 12 13 This has no effect if the element is already present. 14 """ 15 pass 16 17 def clear(self, *args, **kwargs): # real signature unknown 18 """ Remove all elements from this set. """ 19 pass 20 21 def copy(self, *args, **kwargs): # real signature unknown 22 """ Return a shallow copy of a set. """ 23 pass 24 25 def difference(self, *args, **kwargs): # real signature unknown 26 """ 27 Return the difference of two or more sets as a new set. 28 29 (i.e. all elements that are in this set but not the others.) 30 """ 31 pass 32 33 def difference_update(self, *args, **kwargs): # real signature unknown 34 """ Remove all elements of another set from this set. """ 35 pass 36 37 def discard(self, *args, **kwargs): # real signature unknown 38 """ 39 Remove an element from a set if it is a member. 40 41 If the element is not a member, do nothing. 42 """ 43 pass 44 45 def intersection(self, *args, **kwargs): # real signature unknown 46 """ 47 Return the intersection of two sets as a new set. 48 49 (i.e. all elements that are in both sets.) 50 """ 51 pass 52 53 def intersection_update(self, *args, **kwargs): # real signature unknown 54 """ Update a set with the intersection of itself and another. """ 55 pass 56 57 def isdisjoint(self, *args, **kwargs): # real signature unknown 58 """ Return True if two sets have a null intersection. """ 59 pass 60 61 def issubset(self, *args, **kwargs): # real signature unknown 62 """ Report whether another set contains this set. """ 63 pass 64 65 def issuperset(self, *args, **kwargs): # real signature unknown 66 """ Report whether this set contains another set. """ 67 pass 68 69 def pop(self, *args, **kwargs): # real signature unknown 70 """ 71 Remove and return an arbitrary set element. 72 Raises KeyError if the set is empty. 73 """ 74 pass 75 76 def remove(self, *args, **kwargs): # real signature unknown 77 """ 78 Remove an element from a set; it must be a member. 79 80 If the element is not a member, raise a KeyError. 81 """ 82 pass 83 84 def symmetric_difference(self, *args, **kwargs): # real signature unknown 85 """ 86 Return the symmetric difference of two sets as a new set. 87 88 (i.e. all elements that are in exactly one of the sets.) 89 """ 90 pass 91 92 def symmetric_difference_update(self, *args, **kwargs): # real signature unknown 93 """ Update a set with the symmetric difference of itself and another. """ 94 pass 95 96 def union(self, *args, **kwargs): # real signature unknown 97 """ 98 Return the union of sets as a new set. 99 100 (i.e. all elements that are in either set.) 101 """ 102 pass 103 104 def update(self, *args, **kwargs): # real signature unknown 105 """ Update a set with the union of itself and others. """ 106 pass 107 108 def __and__(self, *args, **kwargs): # real signature unknown 109 """ Return self&value. """ 110 pass 111 112 def __contains__(self, y): # real signature unknown; restored from __doc__ 113 """ x.__contains__(y) <==> y in x. """ 114 pass 115 116 def __eq__(self, *args, **kwargs): # real signature unknown 117 """ Return self==value. """ 118 pass 119 120 def __getattribute__(self, *args, **kwargs): # real signature unknown 121 """ Return getattr(self, name). """ 122 pass 123 124 def __ge__(self, *args, **kwargs): # real signature unknown 125 """ Return self>=value. """ 126 pass 127 128 def __gt__(self, *args, **kwargs): # real signature unknown 129 """ Return self>value. """ 130 pass 131 132 def __iand__(self, *args, **kwargs): # real signature unknown 133 """ Return self&=value. """ 134 pass 135 136 def __init__(self, seq=()): # known special case of set.__init__ 137 """ 138 set() -> new empty set object 139 set(iterable) -> new set object 140 141 Build an unordered collection of unique elements. 142 # (copied from class doc) 143 """ 144 pass 145 146 def __ior__(self, *args, **kwargs): # real signature unknown 147 """ Return self|=value. """ 148 pass 149 150 def __isub__(self, *args, **kwargs): # real signature unknown 151 """ Return self-=value. """ 152 pass 153 154 def __iter__(self, *args, **kwargs): # real signature unknown 155 """ Implement iter(self). """ 156 pass 157 158 def __ixor__(self, *args, **kwargs): # real signature unknown 159 """ Return self^=value. """ 160 pass 161 162 def __len__(self, *args, **kwargs): # real signature unknown 163 """ Return len(self). """ 164 pass 165 166 def __le__(self, *args, **kwargs): # real signature unknown 167 """ Return self<=value. """ 168 pass 169 170 def __lt__(self, *args, **kwargs): # real signature unknown 171 """ Return self<value. """ 172 pass 173 174 @staticmethod # known case of __new__ 175 def __new__(*args, **kwargs): # real signature unknown 176 """ Create and return a new object. See help(type) for accurate signature. """ 177 pass 178 179 def __ne__(self, *args, **kwargs): # real signature unknown 180 """ Return self!=value. """ 181 pass 182 183 def __or__(self, *args, **kwargs): # real signature unknown 184 """ Return self|value. """ 185 pass 186 187 def __rand__(self, *args, **kwargs): # real signature unknown 188 """ Return value&self. """ 189 pass 190 191 def __reduce__(self, *args, **kwargs): # real signature unknown 192 """ Return state information for pickling. """ 193 pass 194 195 def __repr__(self, *args, **kwargs): # real signature unknown 196 """ Return repr(self). """ 197 pass 198 199 def __ror__(self, *args, **kwargs): # real signature unknown 200 """ Return value|self. """ 201 pass 202 203 def __rsub__(self, *args, **kwargs): # real signature unknown 204 """ Return value-self. """ 205 pass 206 207 def __rxor__(self, *args, **kwargs): # real signature unknown 208 """ Return value^self. """ 209 pass 210 211 def __sizeof__(self): # real signature unknown; restored from __doc__ 212 """ S.__sizeof__() -> size of S in memory, in bytes """ 213 pass 214 215 def __sub__(self, *args, **kwargs): # real signature unknown 216 """ Return self-value. """ 217 pass 218 219 def __xor__(self, *args, **kwargs): # real signature unknown 220 """ Return self^value. """ 221 pass 222 223 __hash__ = None
6.字典
创建字典:
1 dict1 = {'k1':'v1','k2':'v2'} 2 3 dict2 =dict({'k1':'v1','k2':'v2'})
常用操作:
- 索引
- 新增
- 删除
- 键、值、键值对的操作
- 循环
- 长度