1. 及时体验,自动生成简单demo 链接
2. 注解理解
2.1 @RestController
Spring4之后新加入的注解,原来返回json需要@ResponseBody和@Controller配合。
即@RestController是@ResponseBody和@Controller的组合注解。
2.2 体验 HelloAPI
运行效果:
3. 项目属性配置
运行效果:
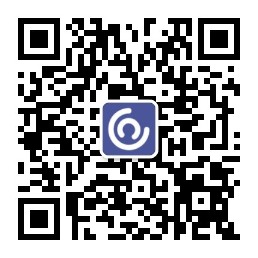
配置文件,代码访问值
注意:yml文件功能与properties文件功能异曲同工
在这里面声明了两个配置
代码访问语法 @Value("${xxx}")
运行效果:
配置文件中引用上下文配置
代码中访问其值:
运行效果如下:
单个配置量 工作量很大,有时候需要配置一个对象,比如人,学生...
使用@Component 和@ConfigurationProperties注解 标注这类的实例化来自配置文件
@ConfigurationProperties注解的语法,可以参考源码,可以设置一个前缀,如同配置文件
运行效果:
有时候 我们希望多多环境配置,比如dev用一套,prod生成环境用一套,这个时候怎么做呢?
在这里我生成了两套配置文件:
在application.properties配置文件中配置我们需要引用那个文件
语法:
spring: profiles: active:dev/prod/xxx
运行效果如下:
Controller的使用
@Controller 处理http请求
@RestController Spring4之后新加的注解,原来返回json需要@ResponseBody配合@Controller
@RequestMapping 配置url映射
对于RequestMapping,查看源码发现,基本操作都是支持多个,比如多个地址请求执行逻辑都是这个方法,多种请求方式都执行这个方法:
// // Source code recreated from a .class file by IntelliJ IDEA // (powered by Fernflower decompiler) // package org.springframework.web.bind.annotation; import java.lang.annotation.Documented; import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; import org.springframework.core.annotation.AliasFor; @Target({ElementType.METHOD, ElementType.TYPE}) @Retention(RetentionPolicy.RUNTIME) @Documented @Mapping public @interface RequestMapping { String name() default ""; @AliasFor("path") String[] value() default {}; @AliasFor("value") String[] path() default {}; RequestMethod[] method() default {}; String[] params() default {}; String[] headers() default {}; String[] consumes() default {}; String[] produces() default {}; }
如:
@RequestMapping(value = {"hello","hi"}, method = RequestMethod.GET) public String sayHello() { return desc; }
执行结果:
@PathVariable 获取url中的数据
@RequestParam 获取请求参数的值
@GetMapping 组合注解
pathvariable 例子
@RequestMapping(value = {"hello/{id}"}, method = RequestMethod.GET) public String sayHello3(@PathVariable("id") int id) { return desc + " _id: "+id; }运行效果:
@RequestParams 是获取请求中的参数,与@PathVariable不同的是,@PathVariable是获取路径中的参数
@RequestMapping(value = {"hellowithid"}, method = RequestMethod.GET) public String sayHello4(@RequestParam("id") int id) { return desc + " _id: " + id; }
运行效果:
@GetMapping 等价与@RequestMapping(value="xxx",method=RequestMethod.GET) 等价get请求,可以简化代码
同样的类似:@PostMapping ....
springboot 全局处理异常
package com.asange.demo.handler; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.bind.annotation.RestControllerAdvice; import javax.servlet.http.HttpServletRequest; import java.util.HashMap; import java.util.Map; /** * com.asange.demo.handler * icourt * author:asange * email:[email protected] **/ @RestControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(value = Exception.class) @ResponseBody private Map<String, Object> exceptionHandler(HttpServletRequest req, Exception e) { Map<String, Object> modelMap = new HashMap<>(); modelMap.put("errorMsg", e.getMessage()); modelMap.put("success", false); return modelMap; } }