目录
一.介绍顺序表
顺序表是用一段物理地址连续的存储单元依次存储数据元素的线性结构,一般情况下采用数组存储。
顺序表与通讯录类似,可以完成增删查改等功能。在此基础上,还可以实现头插、头删、尾插、尾删以及某位置的插入和删除
二.实现顺序表
1.创建多文件
用多文件的好处在通讯录一文中已经说明过了,所以这里直接进入正题:
SeqList.h——函数和类型的声明
SeqList.c——函数的实现
Test.c——测试顺序表
注:为了方便测试,所以没有Test.c没有菜单,直接进行测试
2.顺序表的存储方式
顺序表可以采用两种存储方式:静态存储和动态存储
本文使用的是动态存储,因为静态存储只适用于确定知道需要存多少数据的场景,空间开多了浪费,开少了不够用。现实中基本都是使用动态顺序表,根据需要动态的分配空间大小。
typedef int SLDataType;//重定义方便类型的修改
typedef struct SeqList
{
SLDataType* a;//指向动态开辟的数组
int size;//数据的个数
int capacity;//容量的大小
}SL;
3.函数的声明
该顺序表要实现的函数有:
1.初始化顺序表
2.清理顺序表
3.打印顺序表
4.扩容
5.尾插
6.尾删
7.头插
8.头删
9.查找
10.修改
11.在pos位置插入
12.在pos位置删除
//初始化
void SLInit(SL* ps);
//清理
void SLDestroy(SL* ps);
//打印
void SLPrint(SL* ps);
//扩容
void SLCapacity(SL* ps);
//尾插
void SLPushBack(SL* ps, SLDataType x);
//尾删
void SLPopBack(SL* ps);
//头插
void SLPushFront(SL* ps, SLDataType x);
//头删
void SLPopFront(SL* ps);
//查找
void SLFind(SL* ps, SLDataType x);
//修改
void SLModify(SL* ps, int pos, SLDataType x);
//在pos位置插入
void SLInsert(SL* ps, int pos, SLDataType x);
//在pos位置删除
void SLErase(SL* ps, int pos);
4.初始化顺序表
刚开始要对传过来的指针进行断言,防止为空(后面的也是)
使用malloc函数为数组开辟一块空间(容量大小自己定),数据个数初始化为0
void SLInit(SL* ps)
{
assert(ps);
ps->a = (SLDataType*)malloc(sizeof(SLDataType) * 4);
if (ps->a == NULL)
{
perror("malloc fail");
exit(-1);
}
ps->size = 0;
ps->capacity = 4;
}
5.清理顺序表
程序结束前,要对内存进行清理,因为使用了动态开辟函数,所以必须对使用的空间进行释放,防止内存泄漏
void SLDestroy(SL* ps)
{
assert(ps);
free(ps->a);
ps->a = NULL;
ps->capacity = 0;
ps->size = 0;
}
6.打印顺序表
因为顺序表是连续的空间,所以打印顺序表的数据用for循环遍历出来就可以了
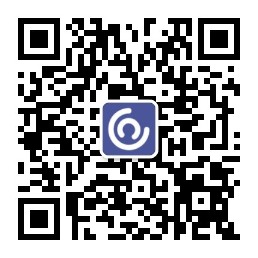
void SLPrint(SL* ps)
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
printf("%d ", ps->a[i]);
}
printf("\n");
}
7.扩容
当顺序表的数据满了(等于刚开始开辟的空间大小),就要进行扩容。使用realloc函数,可以对容量进行修改。
void SLCapacity(SL* ps)
{
assert(ps);
if (ps->size == ps->capacity)
{
SLDataType* ptr = (SLDataType*)realloc(ps->a, 2 * ps->capacity * sizeof(SLDataType));
if (ptr == NULL)
{
perror("realloc fail");
exit(-1);
}
else
{
ps->a = ptr;
ps->capacity *= 2;
}
}
}
8.尾插
进入尾插这个函数,首先要对检查容量是否已满,满了就扩容。
size是数据个数,数组a[ps->size]是下一个数据的下标,尾插一个数把这个数赋给a[ps->size]就行了,然后size++
void SLPushBack(SL* ps, SLDataType x)
{
assert(ps);
SLCapacity(ps);
ps->a[ps->size] = x;
ps->size++;
}
8.尾删
将最后一个元素置为0,然后size减1
注意:当size为0时就不能再减了,所以对size的范围要断言
void SLPopBack(SL* ps)
{
assert(ps);
assert(ps->size > 0);
ps->a[ps->size - 1] = 0;
ps->size--;
}
9.头插
头插数据,先要检查容量;定义一个变量end,指向的是最后一个元素的下一个位置,然后利用while循环,end的范围>=0(把原来的首元素挪动才能头插),将最后一个元素放进它的下个位置,循环一次end减1,依次将前一个元素置到后一个元素的地址去,直到将首元素的位置变成空的状态,然后头插,size加1
void SLPushFront(SL* ps, SLDataType x)
{
assert(ps);
SLCapacity(ps);
int end = ps->size;
while (end >= 0)
{
ps->a[end] = ps->a[end - 1];
end--;
}
ps->a[0] = x;
ps->size++;
}
10.头删
定义一个变量begin等于0,指向首元素。用while循环,将后一个元素覆盖前一个元素,每次循环begin加1,直到最后一个元素向前覆盖完就结束,为头的元素就删除了,然后size减1
注意:当size为0时就不能再减了,所以对size的范围要断言
void SLPopFront(SL* ps)
{
assert(ps);
assert(ps->size > 0);
int begin = 0;
while (begin < ps->size)
{
ps->a[begin] = ps->a[begin + 1];
begin++;
}
ps->size--;
}
11.查找
用for循环遍历顺序表,有与x相同的数就找到了,否则没找到
void SLFind(SL* ps, SLDataType x)
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
if (ps->a[i] == x)
{
printf("找到了\n");
return;
}
}
printf("没找到\n");
}
12.修改
pos的值经过断言如果是在范围内就直接将pos位置的值修改为x,否则报错
void SLModify(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
ps->a[pos] = x;
}
13.在pos位置插入
首先对pos的值进行断言,确定其是否在范围内。插入数值,要考虑容量是否已满,所以要检查容量。接下来与头插类似,把pos位置的数据和后面的数据往后挪动,然后在pos位置插入x,size加1
void SLInsert(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
SLCapacity(ps);
int end = ps->size;
while (end >= pos)
{
ps->a[end] = ps->a[end - 1];
end--;
}
ps->a[pos] = x;
ps->size++;
}
13.在pos位置删除
与头删类似,直到最后一个元素向前覆盖完就结束
void SLErase(SL* ps, int pos)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
int begin = pos;
while (begin < ps->size)
{
ps->a[begin] = ps->a[begin + 1];
begin++;
}
ps->size--;
}
三.全部代码
1.SeqList.h
#pragma once
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
typedef int SLDataType;//重定义方便类型的修改
typedef struct SeqList
{
SLDataType* a;//指向动态开辟的数组
int size;//数据的个数
int capacity;//容量的大小
}SL;
//初始化
void SLInit(SL* ps);
//清理
void SLDestroy(SL* ps);
//打印
void SLPrint(SL* ps);
//扩容
void SLCapacity(SL* ps);
//尾插
void SLPushBack(SL* ps, SLDataType x);
//尾删
void SLPopBack(SL* ps);
//头插
void SLPushFront(SL* ps, SLDataType x);
//头删
void SLPopFront(SL* ps);
//查找
void SLFind(SL* ps, SLDataType x);
//修改
void SLModify(SL* ps, int pos, SLDataType x);
//在pos位置插入
void SLInsert(SL* ps, int pos, SLDataType x);
//在pos位置删除
void SLErase(SL* ps, int pos);
2.SeqList.c
#include "SeqList.h"
//初始化
void SLInit(SL* ps)
{
assert(ps);
ps->a = (SLDataType*)malloc(sizeof(SLDataType) * 4);
if (ps->a == NULL)
{
perror("malloc fail");
exit(-1);
}
ps->size = 0;
ps->capacity = 4;
}
//清理
void SLDestroy(SL* ps)
{
assert(ps);
free(ps->a);
ps->a = NULL;
ps->capacity = 0;
ps->size = 0;
}
//打印
void SLPrint(SL* ps)
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
printf("%d ", ps->a[i]);
}
printf("\n");
}
//扩容
void SLCapacity(SL* ps)
{
assert(ps);
if (ps->size == ps->capacity)
{
SLDataType* ptr = (SLDataType*)realloc(ps->a, 2 * ps->capacity * sizeof(SLDataType));
if (ptr == NULL)
{
perror("realloc fail");
exit(-1);
}
else
{
ps->a = ptr;
ps->capacity *= 2;
}
}
}
//尾插
void SLPushBack(SL* ps, SLDataType x)
{
assert(ps);
SLCapacity(ps);
ps->a[ps->size] = x;
ps->size++;
}
//尾删
void SLPopBack(SL* ps)
{
assert(ps);
assert(ps->size > 0);
ps->a[ps->size - 1] = 0;
ps->size--;
}
//头插
void SLPushFront(SL* ps, SLDataType x)
{
assert(ps);
SLCapacity(ps);
int end = ps->size;
while (end >= 0)
{
ps->a[end] = ps->a[end - 1];
end--;
}
ps->a[0] = x;
ps->size++;
}
//头删
void SLPopFront(SL* ps)
{
assert(ps);
assert(ps->size > 0);
int begin = 0;
while (begin < ps->size)
{
ps->a[begin] = ps->a[begin + 1];
begin++;
}
ps->size--;
}
//查找
void SLFind(SL* ps, SLDataType x)
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
if (ps->a[i] == x)
{
printf("找到了\n");
return;
}
}
printf("没找到\n");
}
//修改
void SLModify(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
ps->a[pos] = x;
}
//在pos位置插入
void SLInsert(SL* ps, int pos, SLDataType x)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
SLCapacity(ps);
int end = ps->size;
while (end >= pos)
{
ps->a[end] = ps->a[end - 1];
end--;
}
ps->a[pos] = x;
ps->size++;
}
//在pos位置删除
void SLErase(SL* ps, int pos)
{
assert(ps);
assert(pos >= 0 && pos < ps->size);
int begin = pos;
while (begin < ps->size)
{
ps->a[begin] = ps->a[begin + 1];
begin++;
}
ps->size--;
}
3.Test.c
#include "SeqList.h"
void test()
{
SL s1;
SLInit(&s1);//初始化
SLPushBack(&s1, 1);
SLPushBack(&s1, 2);
SLPushBack(&s1, 3);
SLPushBack(&s1, 4);
SLPushBack(&s1, 5);//测试尾插
SLPrint(&s1);
SLPopBack(&s1);
SLPopBack(&s1);//测试尾删
SLPrint(&s1);
SLPushFront(&s1, 10);
SLPushFront(&s1, 20);
SLPushFront(&s1, 30);
SLPushFront(&s1, 40);//测试头插
SLPrint(&s1);
SLPopFront(&s1);
SLPopFront(&s1);//测试头删
SLPrint(&s1);
SLFind(&s1, 100);//测试查找
SLModify(&s1, 2, 99);//测试修改
SLPrint(&s1);
SLInsert(&s1, 3, 77);//测试pos位置插入
SLPrint(&s1);
SLErase(&s1, 1);//测试pos位置删除
SLPrint(&s1);
SLDestroy(&s1);
}
int main()
{
test();
return 0;
}
~ ~
感谢观看