参考string,实现mystring
#include <iostream>
#include <cstring>
using namespace std;
class myString
{
private:
char *str; //记录c风格的字符串
int size; //字符串实际长度
public:
//无参构造
myString():size(10) {
cout << "无参构造 this = " << this <<endl;
str = new char [size]; //申请空间
strcpy(str, ""); //赋值为空串
}
//有参构造
myString(const char *s){
cout << "有参构造 this = " << this <<endl;
size = strlen(s); //计算大小,不算\0
str = new char [size+1]; //申请空间,含\0
strcpy(str,s); //传进来的参数拷贝给str
}
//拷贝构造,初始化,参数:同类实例对象的引用,深
myString(const myString & other){
size = other.size;
str= new char[other.size+1];
strcpy(str,other.str);
cout << "拷贝构造" << endl;
}
//析构函数
~myString() {
delete str; //释放堆区空间
cout << "析构函数 this = " << this<< endl;
}
//拷贝赋值函数,赋值,参数:同类实例对象,深拷贝,返回自身的引用,本质:赋值运算符
myString &operator=(const myString &other){
if(this != &other){
this->str = new char[other.size +1] {*(other.str)};
this->size = other.size;
strcpy(str,other.str);
}
cout << "拷贝赋值函数" << endl;
return *this;
}
//判空函数
bool my_empty(const myString &other){
return other.size;
}
//size函数
size_t my_size(myString &other){
return strlen(other.str);
}
//c_str函数
char* my_str(myString &dest,const myString &src){
dest.size = src.size;
dest.str = strcpy(dest.str, src.str);
return dest.str;
}
//at函数 char &at(int pos)
char &at(int pos){
return this->str[pos];
}
//加号运算符重载
const myString operator+(const myString &sec)const{
myString temp;
temp.size = this->size+ sec.size;
temp.str = strcat(this->str,sec.str);
return temp.str;
}
//加等于运算符重载
myString &operator+= (const myString &R){
// char *temp = this->str;
// delete []str;
// this->size += sec.size;
// this->str = new char[this->size + 1];
// strcpy(this->str, temp);
// temp[sizeof (temp) -1] = 'A';
// strcat(this->str,sec.str);
// return *this;
int s_size = size + R.size;
char *s_str = new char[s_size + 1];
strcpy(s_str,str);
strcat(s_str,R.str);
delete[] str;
str = s_str;
size = s_size;
return *this;
}
//关系运算符重载 (>)
bool operator> (const myString &sec)const {
return strcmp(this->str,sec.str);
}
//遍历
void show(){
cout << "str = " << str << " size = " << size << endl;
}
};
int main()
{
myString s1; //无参构造
myString s2("hello world"); //有参构造
myString s3(s2); //拷贝构造
myString s4;
s4 = s2; //加号
myString s5("long time no see ");
s5 += s2; //加等
cout << "s1 >>> ";
s1.show();
cout << "s2 >>> ";
s2.show();
cout << "s3 >>> ";
s3.show();
cout << "s4 >>> ";
s4.show();
cout << "s5 >>> ";
s5.show();
if(s2>s4){
cout << "yes" << endl;
}else {
cout << "no" << endl;
}
return 0;
}
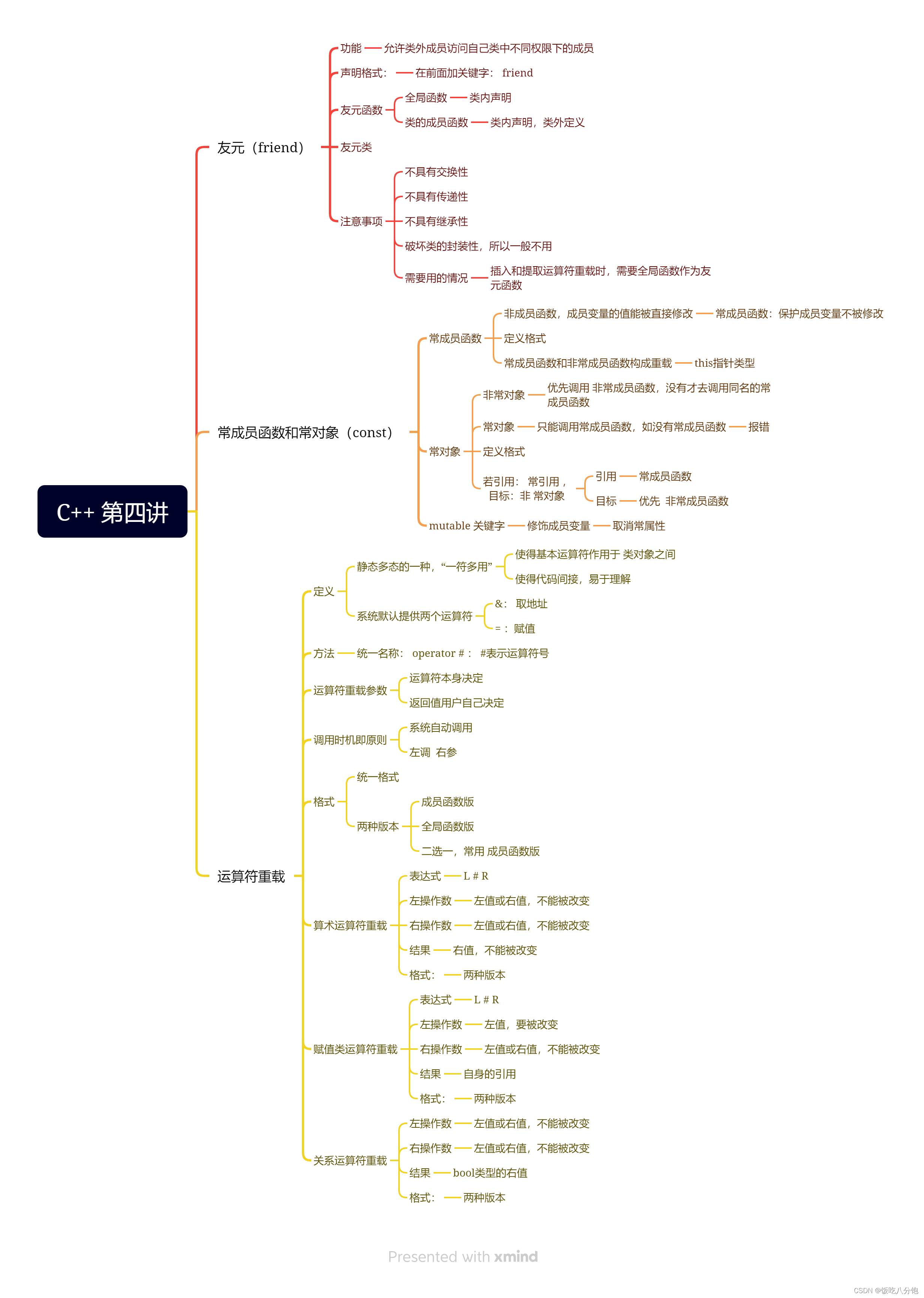