序言
在Android开发中,merge
、include
和ViewStub
都是用于布局的标签。
merge标签:
merge标签用于优化布局层级,可以减少不必要的视图层次,提高布局的性能。
它会将标记的子视图合并到其父视图中,而不会创建新的视图层次结构。这意味着merge标签本身不会生成视图,仅用于组织布局结构。
merge通常与其他布局标签(如LinearLayout、RelativeLayout等)一起使用,以组成复杂的布局。
示例
<!-- parent_layout.xml -->
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<merge>
<!-- 子视图 -->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, world!" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click me" />
</merge>
</LinearLayout>
parent_layout.xml使用了merge标签将TextView和Button合并到LinearLayout中,而无需创建额外的视图层次结构。
include标签:
include标签用于在布局中重用其他布局文件。
通过include标签,可以将其他布局文件中定义的视图结构嵌入到当前布局中,实现布局的模块化和复用。
include标签需要指定要包含的布局文件的名称,并可以通过属性进行配置。
示例
<!-- parent_layout.xml -->
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/child_layout" />
</LinearLayout>
<!-- child_layout.xml -->
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, world!" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click me" />
</LinearLayout>
parent_layout.xml使用include标签引用了名为child_layout.xml的布局文件,从而实现了布局的重用和模块化。
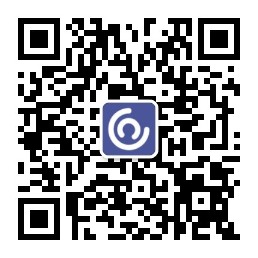
ViewStub类:
ViewStub类允许您在布局中延迟加载或懒加载视图,以优化布局的性能和内存占用。
在初始加载时,ViewStub只会占用非常小的内存空间,只有在需要显示时才会被实例化。
ViewStub通常用作一个占位符,占据布局中的位置,但最初不会显示任何内容。当需要加载显示视图时,可以通过ViewStub的inflate()方法将其实例化为真正的视图,并替代占位符。
示例
<!-- parent_layout.xml -->
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<Button
android:id="@+id/show_view_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show View" />
<ViewStub
android:id="@+id/view_stub"
android:inflatedId="@+id/inflated_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout="@layout/inflatable_view" />
</LinearLayout>
<!-- inflatable_view.xml -->
<TextView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/inflated_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="This is the inflated view" />
parent_layout.xml包含了一个ViewStub标签,该标签引用了一个名为inflatable_view.xml的布局文件。初始加载时,ViewStub只会占用很小的内存空间。当点击"Show View"按钮后,可以通过以下代码将ViewStub实例化为真正的视图:
Button showViewButton = findViewById(R.id.show_view_button);
ViewStub viewStub = findViewById(R.id.view_stub);
showViewButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
View inflatedView = viewStub.inflate(); // 实例化ViewStub为真正的视图
// 进行对视图的操作
TextView textView = inflatedView.findViewById(R.id.inflated_view);
textView.setText("This is the inflated view");
}
});
通过点击按钮触发的事件,可以将ViewStub动态地实例化为真正的视图,并在其中进行相应的操作。