winform+Oracle数据库
通过数据库查询出所有列名、数据类型、描述等信息进行动态生成winform表单文件,再通过发射对类进行取值赋值.........适用于表结构单一,需要快速开发的项目
经理预估期1个星期的工作时间,我也不知道如果真的一个星期做完我会不会挂掉,而实际情况是数据库建立都花了一个星期虽然表名称、数据类型都不需要自己想,从建表模型设计,框架寻找。
1.需要用的类

public class FiledInfo { /// <summary> /// 字段名 /// </summary> public string FiledName { get; set; } /// <summary> /// 字段描述 /// </summary> public string FiledDesc { get; set; } /// <summary> /// 在界面中的排序 /// </summary> public double Index { get; set; } /// <summary> /// 是否只读 /// </summary> [DefaultValue(false)] public bool Readonly { get; set; } /// <summary> /// 是否隐藏 /// </summary> [DefaultValue(false)] public bool IsHide { get; set; } /// <summary> /// 是否占全行 /// </summary> [DefaultValue(false)] public bool AllLine { get; set; } /// <summary> /// 是否为空 /// </summary> public bool IsRequired { get; set; } public string Placeholder { get; set; } /// <summary> /// 验证类型 /// </summary> public VerificationStr VerificationStrType { get; set; } /// <summary> /// 提示信息 /// </summary> public string errormessage { get; set; } /// <summary> /// 设置长度最大 /// </summary> public int length { get; set; } /// <summary> /// 设置最小长度 /// </summary> public int minlen { get; set; } /// <summary> /// 设置必须填写多少位 /// </summary> public int eqlen { get; set; } }

/// <summary> /// 验证 /// </summary> public enum VerificationStr { /// <summary> /// 固定长度 /// </summary> length, /// <summary> /// 是否为空 /// </summary> isnull, /// <summary> /// 电话号码验证 /// </summary> phone, /// <summary> /// 密码验证 /// </summary> password, /// <summary> /// 邮箱验证 /// </summary> email, /// <summary> /// 字母和数字 /// </summary> Lettersandnumbers, /// <summary> /// 字母 /// </summary> Letters, /// <summary> /// 数字 /// </summary> intnumbers, /// <summary> /// 浮点数 /// </summary> decmilnumber, /// <summary> /// 时间验证2007-06-05 10:57:10 /// </summary> checkFullTime, /// <summary> /// 时间验证 10:57:10 /// </summary> checkTime, /// <summary> /// 时间验证 2007-06-05 /// </summary> checkDate, /// <summary> /// 身份证号码验证 /// </summary> card, /// <summary> /// 整型数据验证 /// </summary> checkInteger, /// <summary> /// 固定电话号码 验证 /// </summary> checkTelephone, /// <summary> /// QQ号码验证 /// </summary> checkQQ, /// <summary> /// 外部链接地址验证 /// </summary> checkURL, /// <summary> /// 金额类型 /// </summary> isMoney }

public class EditPageControl {/// <summary> /// 字段名 /// </summary> public string FiledName { get; set; } /// <summary> /// 控件类型 /// </summary> public ContorlType CType { get; set; } /// <summary> /// 取值 /// </summary> public object Value { get; set; } public bool isRequery { get; set; } }

public enum ContorlType { select, uedit, fileUpload }

/// <summary> /// 数据访问基础类(基于OleDb) Copyright (C) Maticsoft /// 可以用户可以修改满足自己项目的需要。 /// </summary> public abstract class DbHelperOleDb { //数据库连接字符串(web.config来配置),可以动态更改connectionString支持多数据库. public static string connectionString = ConfigurationManager.ConnectionStrings["database"].ToString(); // "Data Source=182.148.123.214/orcl;User ID=DEV_LSYJ;Password=DEV_LSYJ;Unicode=true"; public DbHelperOleDb() { } #region 执行简单SQL语句 /// <summary> /// 执行SQL语句,返回影响的记录数 /// </summary> /// <param name="SQLString">SQL语句</param> /// <returns>影响的记录数</returns> public static int ExecuteSql(string SQLString) { using (OracleConnection connection = new OracleConnection(connectionString)) { using (OracleCommand cmd = new OracleCommand(SQLString, connection)) { try { connection.Open(); int rows = cmd.ExecuteNonQuery(); return rows; } catch (System.Data.OleDb.OleDbException E) { connection.Close(); throw new Exception(E.Message); } } } } /// <summary> /// 执行多条SQL语句,实现数据库事务。 /// </summary> /// <param name="SQLStringList">多条SQL语句</param> public static void ExecuteSqlTran(ArrayList SQLStringList) { using (OracleConnection conn = new OracleConnection(connectionString)) { conn.Open(); OracleCommand cmd = new OracleCommand(); cmd.Connection = conn; OracleTransaction tx = conn.BeginTransaction(); cmd.Transaction = tx; try { for (int n = 0; n < SQLStringList.Count; n++) { string strsql = SQLStringList[n].ToString(); if (strsql.Trim().Length > 1) { cmd.CommandText = strsql; cmd.ExecuteNonQuery(); } } tx.Commit(); } catch (System.Data.OleDb.OleDbException E) { tx.Rollback(); throw new Exception(E.Message); } } } /// <summary> /// 执行带一个存储过程参数的的SQL语句。 /// </summary> /// <param name="SQLString">SQL语句</param> /// <param name="content">参数内容,比如一个字段是格式复杂的文章,有特殊符号,可以通过这个方式添加</param> /// <returns>影响的记录数</returns> public static int ExecuteSql(string SQLString, string content) { using (OracleConnection connection = new OracleConnection(connectionString)) { OracleCommand cmd = new OracleCommand(SQLString, connection); System.Data.OleDb.OleDbParameter myParameter = new System.Data.OleDb.OleDbParameter("@content", OleDbType.VarChar); myParameter.Value = content; cmd.Parameters.Add(myParameter); try { connection.Open(); int rows = cmd.ExecuteNonQuery(); return rows; } catch (System.Data.OleDb.OleDbException E) { throw new Exception(E.Message); } finally { cmd.Dispose(); connection.Close(); } } } /// <summary> /// 向数据库里插入图像格式的字段(和上面情况类似的另一种实例) /// </summary> /// <param name="strSQL">SQL语句</param> /// <param name="fs">图像字节,数据库的字段类型为image的情况</param> /// <returns>影响的记录数</returns> public static int ExecuteSqlInsertImg(string strSQL, byte[] fs) { using (OracleConnection connection = new OracleConnection(connectionString)) { OracleCommand cmd = new OracleCommand(strSQL, connection); System.Data.OleDb.OleDbParameter myParameter = new System.Data.OleDb.OleDbParameter("@fs", OleDbType.Binary); myParameter.Value = fs; cmd.Parameters.Add(myParameter); try { connection.Open(); int rows = cmd.ExecuteNonQuery(); return rows; } catch (System.Data.OleDb.OleDbException E) { throw new Exception(E.Message); } finally { cmd.Dispose(); connection.Close(); } } } /// <summary> /// 执行一条计算查询结果语句,返回查询结果(object)。 /// </summary> /// <param name="SQLString">计算查询结果语句</param> /// <returns>查询结果(object)</returns> public static object GetSingle(string SQLString) { using (OracleConnection connection = new OracleConnection(connectionString)) { using (OracleCommand cmd = new OracleCommand(SQLString, connection)) { try { connection.Open(); object obj = cmd.ExecuteScalar(); if ((Object.Equals(obj, null)) || (Object.Equals(obj, System.DBNull.Value))) { return null; } else { return obj; } } catch (System.Data.OleDb.OleDbException e) { connection.Close(); throw new Exception(e.Message); } } } } /// <summary> /// 执行查询语句,返回OleDbDataReader /// </summary> /// <param name="strSQL">查询语句</param> /// <returns>OleDbDataReader</returns> public static OracleDataReader ExecuteReader(string strSQL) { OracleConnection connection = new OracleConnection(connectionString); OracleCommand cmd = new OracleCommand(strSQL, connection); try { connection.Open(); OracleDataReader myReader = cmd.ExecuteReader(); return myReader; } catch (System.Data.OleDb.OleDbException e) { throw new Exception(e.Message); } } public static DataSet GetListBySql(string strSql) {//// (OracleConnection)CreateConnection() using (OracleConnection connection = new OracleConnection(connectionString)) { DataSet ds = new DataSet(); try { connection.Open(); OracleDataAdapter command = new OracleDataAdapter(strSql, connection); command.Fill(ds, "ds"); } catch (DbException ex) { throw new Exception(ex.Message); } return ds; } } /// <summary> /// 执行查询语句,返回DataSet /// </summary> /// <param name="SQLString">查询语句</param> /// <returns>DataSet</returns> public static DataSet Query(string SQLString) { using (OracleConnection connection = new OracleConnection(connectionString)) { DataSet ds = new DataSet(); try { connection.Open(); OracleDataAdapter command = new OracleDataAdapter(SQLString, connection); command.Fill(ds, "ds"); } catch (System.Data.OleDb.OleDbException ex) { throw new Exception(ex.Message); } return ds; } } #endregion #region 执行带参数的SQL语句 /// <summary> /// 执行SQL语句,返回影响的记录数 /// </summary> /// <param name="SQLString">SQL语句</param> /// <returns>影响的记录数</returns> public static int ExecuteSql(string SQLString, params OleDbParameter[] cmdParms) { using (OracleConnection connection = new OracleConnection(connectionString)) { using (OracleCommand cmd = new OracleCommand()) { try { PrepareCommand(cmd, connection, null, SQLString, cmdParms); int rows = cmd.ExecuteNonQuery(); cmd.Parameters.Clear(); return rows; } catch (System.Data.OracleClient.OracleException E) { throw new Exception(E.Message); } } } } /// <summary> /// 执行多条SQL语句,实现数据库事务。 /// </summary> /// <param name="SQLStringList">SQL语句的哈希表(key为sql语句,value是该语句的OleDbParameter[])</param> public static void ExecuteSqlTran(Hashtable SQLStringList) { using (OracleConnection conn = new OracleConnection(connectionString)) { conn.Open(); using (OracleTransaction trans = conn.BeginTransaction()) { OracleCommand cmd = new OracleCommand(); try { //循环 foreach (DictionaryEntry myDE in SQLStringList) { string cmdText = myDE.Key.ToString(); OleDbParameter[] cmdParms = (OleDbParameter[])myDE.Value; PrepareCommand(cmd, conn, trans, cmdText, cmdParms); int val = cmd.ExecuteNonQuery(); cmd.Parameters.Clear(); trans.Commit(); } } catch { trans.Rollback(); throw; } } } } /// <summary> /// 执行一条计算查询结果语句,返回查询结果(object)。 /// </summary> /// <param name="SQLString">计算查询结果语句</param> /// <returns>查询结果(object)</returns> public static object GetSingle(string SQLString, params OleDbParameter[] cmdParms) { using (OracleConnection connection = new OracleConnection(connectionString)) { using (OracleCommand cmd = new OracleCommand()) { try { PrepareCommand(cmd, connection, null, SQLString, cmdParms); object obj = cmd.ExecuteScalar(); cmd.Parameters.Clear(); if ((Object.Equals(obj, null)) || (Object.Equals(obj, System.DBNull.Value))) { return null; } else { return obj; } } catch (System.Data.OracleClient.OracleException e) { throw new Exception(e.Message); } } } } /// <summary> /// 执行查询语句,返回OleDbDataReader /// </summary> /// <param name="strSQL">查询语句</param> /// <returns>OleDbDataReader</returns> public static OracleDataReader ExecuteReader(string SQLString, params OleDbParameter[] cmdParms) { OracleConnection connection = new OracleConnection(connectionString); OracleCommand cmd = new OracleCommand(); try { PrepareCommand(cmd, connection, null, SQLString, cmdParms); OracleDataReader myReader = cmd.ExecuteReader(); cmd.Parameters.Clear(); return myReader; } catch (System.Data.OleDb.OleDbException e) { throw new Exception(e.Message); } } /// <summary> /// 执行查询语句,返回DataSet /// </summary> /// <param name="SQLString">查询语句</param> /// <returns>DataSet</returns> public static DataSet Query(string SQLString, params OleDbParameter[] cmdParms) { using (OracleConnection connection = new OracleConnection(connectionString)) { OracleCommand cmd = new OracleCommand(); PrepareCommand(cmd, connection, null, SQLString, cmdParms); using (OracleDataAdapter da = new OracleDataAdapter(cmd)) { DataSet ds = new DataSet(); try { da.Fill(ds, "ds"); cmd.Parameters.Clear(); } catch (System.Data.OleDb.OleDbException ex) { throw new Exception(ex.Message); } return ds; } } } private static void PrepareCommand(OracleCommand cmd, OracleConnection conn, OracleTransaction trans, string cmdText, OleDbParameter[] cmdParms) { if (conn.State != ConnectionState.Open) conn.Open(); cmd.Connection = conn; cmd.CommandText = cmdText; if (trans != null) cmd.Transaction = trans; cmd.CommandType = CommandType.Text;//cmdType; if (cmdParms != null) { foreach (OleDbParameter parm in cmdParms) cmd.Parameters.Add(parm); } } #endregion }
2.创建一个公用的类CreateModel 由于winform页面需要用到这个类 就直接继承了这个类,而这个类继承了System.Web.UI.Page 可能这样有点不妥 但是对于从来没有这么大工作量的我来说也就简简单单的写了个
/// <summary> /// 编辑页面处理公用类 /// </summary> public class CreateModel : System.Web.UI.Page {
/// <summary> /// 定义一个XTYPE公用方法 /// </summary> public object XTYPE; /// <summary> /// 省平台的apikey 上报数据的时候有用 /// </summary> public string APIKEY = ConfigurationManager.AppSettings["APIKEY"]; /// <summary> /// 设置 /// </summary> public List<FiledInfo> fileds = new List<FiledInfo>(); //非必填字段 public List<string> nonMFileds = new List<string>();
//设置特殊字段 public List<EditPageControl> SpecialControls = new List<EditPageControl>();/// <summary> /// 设置是否只读 /// </summary> public bool isReadonly = false;

/// <summary> /// 创建表单 /// </summary> /// <param name="type"></param> public void CreateData1(Type type) { //创建外层div HtmlGenericControl htmlDD = new HtmlGenericControl("div"); if (Cache["" + type.Name + ""] == null) { //查询所有列名 DataSet ds = DbHelperOleDb.GetListBySql("select table_name,column_Name,Comments from user_col_comments where table_name='" + type.Name + "'"); //查询该表的列类型及可允许最大长度 DataSet datalenth = DbHelperOleDb.GetListBySql("select column_name as Names, data_type as types, CHAR_COL_DECL_LENGTH as lengths from user_tab_columns where table_name= '" + type.Name + "'"); Cache["" + type.Name + ""] = ds; Cache["data" + type.Name + ""] = datalenth; } if (Cache["" + type.Name + ""] != null) { int index = 0; #region 设置字段 foreach (DataRow item in (Cache["" + type.Name + ""] as DataSet).Tables[0].Rows) { //没有预置的字段 if (fileds.Count == 0 || fileds.Count(p => p.FiledName.ToUpper() == item["column_Name"].ToString().ToUpper()) == 0) { fileds.Add(new FiledInfo() { FiledName = item["column_Name"].ToString(), FiledDesc = item["Comments"].ToString(), Index = index }); } else { var f = fileds.FirstOrDefault(p => p.FiledName.ToUpper() == item["column_Name"].ToString()); if (f == null) { fileds.FirstOrDefault(p => p.FiledName.ToString() == item["column_Name"].ToString()); } if (f != null) { f.FiledDesc = item["Comments"].ToString(); if (f.Index == 0) f.Index = index; } } index++; } #endregion fileds = fileds.OrderBy(p => p.Index).ToList(); foreach (var filed in fileds) { //隐藏字段不处理 if (filed.IsHide) continue; if (Cache["data" + type.Name + ""] != null) { //设置最大允许值 var so = (Cache["data" + type.Name + ""] as DataSet).Tables[0].Select("Names='" + filed.FiledName + "'"); if (so.Length > 0) { var text = so[0]["Names"]; var ty = so[0]["types"] + ""; var lenth = so[0]["lengths"] + ""; if (!string.IsNullOrEmpty(lenth)) { //if (ty == "NVARCHAR2") //{ // filed.length = Convert.ToInt32(lenth) / 2; //} if (ty == "VARCHAR2" || ty == "CHAR" || ty == "NVARCHAR2") { filed.length = Convert.ToInt32(lenth); } } } } #region MyRegion HtmlGenericControl DIV = new HtmlGenericControl("DIV"); DIV.Attributes.Add("STYLE", "WIDTH:50%;height:53px; FLOAT:LEFT"); HtmlGenericControl htmlpdiv = new HtmlGenericControl("p"); #region 设置lable及样式 HtmlGenericControl label = new HtmlGenericControl("label"); if (ConfigurationManager.AppSettings["IsShowIndex"] == "1") label.InnerText = filed.FiledDesc + filed.Index.ToString(); else label.InnerText = filed.FiledDesc; //Label labelControl = new Label(); //labelControl.CssClass = "";//设置样式 //labelControl.Text = item["Comments"].ToString();//设置文本 htmlpdiv.Controls.Add(label); #endregion HtmlGenericControl spanDiv = new HtmlGenericControl("div"); spanDiv.Attributes.Add("class", "field"); PropertyInfo cons = type.GetProperty(filed.FiledName); #endregion //获取特殊控件 可能存在大小写不一致导致查询出错的问题 var specialContorl = SpecialControls.FirstOrDefault(p => p.FiledName.ToLower() == filed.FiledName.ToLower()); if (specialContorl == null) { specialContorl = SpecialControls.FirstOrDefault(p => p.FiledName.ToUpper() == filed.FiledName.ToUpper()); } if (specialContorl == null) { specialContorl = SpecialControls.FirstOrDefault(p => p.FiledName == filed.FiledName); } if (specialContorl != null) { #region 处理特殊控件 switch (specialContorl.CType) { case ContorlType.select://下拉列表 spanDiv.Attributes.Add("class", "field"); if (filed.AllLine) DIV.Attributes.Add("style", "width:100%;clear: both;"); DropDownList ddl = new DropDownList(); ddl.ID = "S_" + filed.FiledName; ddl.Enabled = !isReadonly; if (specialContorl.Value != null) { var items = specialContorl.Value as List<DictionaryEntry>; ddl.DataSource = items; ddl.DataValueField = "value"; ddl.DataTextField = "key"; ddl.DataBind(); ddl.SelectedIndex = ddl.Items.IndexOf(ddl.Items.FindByValue((cons.GetValue(XTYPE) + "").ToString().Trim())); } ddl.Attributes.Add("style", "width:98%;"); spanDiv.Controls.Add(ddl); break; case ContorlType.uedit://文本编辑器 spanDiv.Attributes.Add("class", "field"); DIV.Attributes.Add("style", "width:90%;clear: both;"); HtmlTextArea txtTextArea1 = new HtmlTextArea(); txtTextArea1.ID = "S_" + filed.FiledName; txtTextArea1.Attributes.Add("class", "show"); txtTextArea1.InnerText = cons.GetValue(XTYPE) + ""; txtTextArea1.Disabled = isReadonly; spanDiv.Controls.Add(txtTextArea1); break; case ContorlType.fileUpload://附件 DIV.Attributes.Add("STYLE", "WIDTH:50%;height:Auto; FLOAT:LEFT"); spanDiv.Attributes.Add("class", "field"); if (cons.GetValue(XTYPE) != null) { spanDiv.Attributes.Add("style", "border: 1px solid #ccc;padding: 5px;"); string[] fileNames = cons.GetValue(XTYPE).ToString().Split(new string[] { ";" }, StringSplitOptions.RemoveEmptyEntries); if (fileNames.Length > 0) { //显示已上传的文件 HtmlGenericControl table = new HtmlGenericControl("table"); table.Attributes.Add("name", "files"); foreach (string fileName in fileNames) { HtmlGenericControl tr = new HtmlGenericControl("tr"); HtmlGenericControl td1 = new HtmlGenericControl("td"); string fileSortName = fileName.Substring(fileName.LastIndexOf("/") + 1, fileName.Length - fileName.LastIndexOf("/") - 1); fileSortName = fileSortName.Substring(0, fileSortName.LastIndexOf("_")) + fileSortName.Substring(fileSortName.LastIndexOf("."), fileSortName.Length - fileSortName.LastIndexOf(".")); td1.InnerHtml = string.Format("<a href='{0}' target='_blank'>{1}</a>", fileName, fileSortName); HtmlGenericControl td2 = new HtmlGenericControl("td"); td2.InnerHtml = "<a href='JavaScript:;' name='delFile'>删除</a>"; tr.Controls.Add(td1); tr.Controls.Add(td2); table.Controls.Add(tr); } spanDiv.Controls.Add(table); HiddenField hid = new HiddenField(); hid.ID = "S_HID_" + filed.FiledName; hid.Value = cons.GetValue(XTYPE).ToString(); spanDiv.Controls.Add(hid); } } FileUpload file = new FileUpload(); file.ID = "S_" + filed.FiledName; file.AllowMultiple = true; //设置附件是否必填 if (specialContorl.isRequery) { filed.IsRequired = false; } else { filed.IsRequired = true; } //filed.IsRequired = true; file.Enabled = !isReadonly; spanDiv.Controls.Add(file); if (filed.AllLine) DIV.Attributes.Add("style", "width:100%;clear: both;"); break; default: break; } if (filed.AllLine) { DIV.Attributes.Add("style", "width:100%;clear: both;"); spanDiv.Attributes.Add("style", "margin-left: 12.5%;"); label.Attributes.Add("style", "width: 12.5%"); } if (!filed.IsRequired) { HtmlGenericControl error = new HtmlGenericControl("span"); error.Attributes.Add("title", filed.errormessage);//添加提示信息 error.Attributes.Add("type", filed.VerificationStrType + "");//添加提示信息 if (filed.length > 0) { error.Attributes.Add("length", filed.length + "");//添加限制长度 } if (filed.minlen > 0) { error.Attributes.Add("minlen", filed.minlen + ""); } if (filed.eqlen > 0) { error.Attributes.Add("eqlen", filed.eqlen + ""); } error.InnerText = "*"; error.Attributes.Add("style", "color:red;position: absolute;left: 0;folat:left"); spanDiv.Controls.Add(error); } #endregion } else { #region 处理一般控件 PropertyInfo con = type.GetProperty(filed.FiledName); var valtype = con.PropertyType; if (valtype.IsGenericType && valtype.GetGenericTypeDefinition().Equals(typeof(Nullable<>)))//判断convertsionType是否为nullable泛型类 { //如果type为nullable类,声明一个NullableConverter类,该类提供从Nullable类到基础基元类型的转换 System.ComponentModel.NullableConverter nullableConverter = new System.ComponentModel.NullableConverter(valtype); //将type转换为nullable对的基础基元类型 valtype = nullableConverter.UnderlyingType; } TextBox txtControl = new TextBox(); if (valtype.Name == "Decimal" || valtype.Name == "Single" || valtype.Name == "Int16" || valtype.Name == "Int32" || valtype.Name == "Double" || valtype.Name == "Float") { filed.VerificationStrType = VerificationStr.decmilnumber; if (filed.Placeholder == null) { filed.Placeholder = "请输入数字"; } //txtControl.Attributes.Add("type", "number"); txtControl.CssClass = "mediuminput"; txtControl.Text = "0";//设置初始值 } else if (valtype.Name == "DateTime") { filed.VerificationStrType = VerificationStr.checkFullTime; filed.Placeholder = "请选择时间"; txtControl.CssClass = "laydate-icon"; if (!isReadonly) { txtControl.Text = "";//设置初始值 txtControl.Attributes.Add("onclick", "laydate({istime: true, format: 'YYYY-MM-DD hh:mm:ss'})"); } if (filed.Readonly) { txtControl.Text = "";//设置初始值 txtControl.Attributes.Add("onclick", ""); } txtControl.Attributes.Add("style", "width:91%"); } else { txtControl.CssClass = "mediuminput"; } if (filed.AllLine) { DIV.Attributes.Add("style", "width:100%;clear: both;"); spanDiv.Attributes.Add("style", "margin-left: 12.5%;"); label.Attributes.Add("style", "width: 12.5%"); txtControl.Attributes.Add("style", "width:98%"); } txtControl.ReadOnly = isReadonly; //设置id txtControl.ID = "S_" + filed.FiledName.ToString(); //赋值 txtControl.Text = cons.GetValue(XTYPE) + ""; if (filed.Readonly) { txtControl.ReadOnly = true; } //txtControl.TextMode = TextBoxMode.Password;//设置密码框 //txtControl.TextMode = TextBoxMode.MultiLine;//设置多行文本框 txtControl.Attributes.Add("tipmsg", "提示信息");//设置属性 txtControl.Attributes.Add("Placeholder", filed.Placeholder);//水印提示 spanDiv.Controls.Add(txtControl); #endregion } //获取数据库长度 if (!filed.IsRequired) { HtmlGenericControl error = new HtmlGenericControl("span"); error.Attributes.Add("title", filed.errormessage);//添加提示信息 error.Attributes.Add("type", filed.VerificationStrType + "");//添加提示信息 if (filed.length > 0) { error.Attributes.Add("length", filed.length + "");//添加限制长度 } if (filed.minlen > 0) { error.Attributes.Add("minlen", filed.minlen + ""); } if (filed.eqlen > 0) { error.Attributes.Add("eqlen", filed.eqlen + ""); } error.InnerText = "*"; error.Attributes.Add("style", "color:red;position: absolute;left: 0;folat:left"); spanDiv.Controls.Add(error); } htmlpdiv.Controls.Add(spanDiv); DIV.Controls.Add(htmlpdiv); htmlDD.Controls.Add(DIV); } } Cache[type.Name + "htmlDD"] = htmlDD; }

/// <summary> /// 保存的时候验证是否必填 /// </summary> /// <param name="field_tab_content">包裹文本框编辑框下拉框附件的页面控件</param> /// <param name="type">类类型</param> /// <param name="XTYPE">数据</param> /// <param name="ok">是否必填</param> /// <returns></returns> public bool Save1(HtmlGenericControl field_tab_content, Type type, object XTYPE, string ok) { #region 赋值 var flog = false; var cheurl = false; if (ok == "ok") { cheurl = true; flog = true; } foreach (var filed in fileds) { //取到属性 PropertyInfo property = type.GetProperty(filed.FiledName.ToUpper()); if (property == null) property = type.GetProperty(filed.FiledName); var valtype = property.PropertyType; var va = valtype.Name; if (valtype.IsGenericType && valtype.GetGenericTypeDefinition().Equals(typeof(Nullable<>)))//判断convertsionType是否为nullable泛型类 { //如果type为nullable类,声明一个NullableConverter类,该类提供从Nullable类到基础基元类型的转换 System.ComponentModel.NullableConverter nullableConverter = new System.ComponentModel.NullableConverter(valtype); //将type转换为nullable对的基础基元类型 valtype = nullableConverter.UnderlyingType; } //获取特殊控件 var specialContorl = SpecialControls.FirstOrDefault(p => p.FiledName.ToLower() == filed.FiledName.ToLower()); if (specialContorl != null) { #region 特殊控件取值 switch (specialContorl.CType) { case ContorlType.select://下拉列表 DropDownList ddl = field_tab_content.FindControl("S_" + filed.FiledName) as DropDownList; if (ddl != null) { var ddlVal = ddl.SelectedItem.Value.Trim(); if (ddlVal == "") { flog = false; } if (ddlVal != "") property.SetValue(XTYPE, Convert.ChangeType(ddlVal, valtype), null); else ddl.Attributes.Add("style", "border-color: red;"); } break; case ContorlType.fileUpload://附件 FileUpload file = field_tab_content.FindControl("S_" + filed.FiledName) as FileUpload; IList<System.Web.HttpPostedFile> lis = file.PostedFiles;//使用fileupload控件获取上传文件的文件名 string fileNames = ""; foreach (System.Web.HttpPostedFile item in lis) { string strName = item.FileName; if (strName != "")//如果文件名存在 { bool fileOK = false; int i = strName.LastIndexOf(".");//获取.的索引顺序号 string kzm = strName.Substring(i);//获取文件扩展名的另一种方法 string fileExtension = System.IO.Path.GetExtension( string newName = item.FileName.Substring(0, i) + "_" + DateTime.Now.ToString("HHmmssfff");//生成新的文件名,保证唯一性 string fullname = GetUpLoadPath(); string juedui = Server.MapPath(fullname);//设置文件保存的本地目录绝对路径,对于路径中的字符“\”在字符串中必须以“\\”表示,因为“\”为特殊字符。或者可以使用上一行的给路径前面加上@ string newFileName = juedui + newName + kzm; fileOK = CheckFileExt(kzm); if (fileOK) { try { // 判定该路径是否存在 if (!Directory.Exists(juedui)) Directory.CreateDirectory(juedui); string file1 = file.PostedFile.FileName; string file2 = file.PostedFile.ContentLength + ""; string file3 = file.PostedFile.ContentType; string filename = newName + kzm; file.PostedFile.SaveAs(newFileName);//将文件存储到服务器上 fileNames += fullname + newName + kzm + ";"; } catch (Exception) { // } } else { // } } } HiddenField hidFileNames = field_tab_content.FindControl("S_HID_" + filed.FiledName) as HiddenField; if (hidFileNames != null) property.SetValue(XTYPE, Convert.ChangeType(hidFileNames.Value + fileNames, valtype), null); else property.SetValue(XTYPE, Convert.ChangeType(fileNames, valtype), null); break; case ContorlType.uedit://文本编辑器 HtmlTextArea txtControls = field_tab_content.FindControl("S_" + filed.FiledName) as HtmlTextArea; var ueditVal = txtControls.InnerText; if (ueditVal != "") { property.SetValue(XTYPE, Convert.ChangeType(ueditVal, valtype), null); } break; } #endregion } else { //一般控件取值 TextBox txtControl = field_tab_content.FindControl("S_" + filed.FiledName) as TextBox; if (txtControl != null) { var val = txtControl.Text.Trim();//txtControl.Text.Trim(); decimal dl = 0; DateTime dt; txtControl.Attributes.Add("style", "border-color: #CCC;"); if (valtype.Name == "Decimal" || valtype.Name == "Single" || valtype.Name == "Double" || valtype.Name == "Int32") { if (!decimal.TryParse(val, out dl)) { flog = false; val = 0 + ""; } val = dl.ToString(); } else if (valtype.Name == "DateTime") { if (!DateTime.TryParse(val, out dt)) { flog = false; val = DateTime.Now + ""; } val = dt.ToString(); } if (string.IsNullOrEmpty(val) && valtype.Name == "DateTime") { property.SetValue(XTYPE, Convert.ChangeType(DateTime.MinValue, valtype), null); } else if (string.IsNullOrEmpty(val) && (valtype.Name == "Int32" || valtype.Name == "Int16" || valtype.Name == "Decimal" || valtype.Name == "Single" || valtype.Name == "Double" || valtype.Name == "Float")) { property.SetValue(XTYPE, Convert.ChangeType(0, valtype), null); } else { property.SetValue(XTYPE, Convert.ChangeType(val, valtype), null); } //if (val != "") //{ // //其他验证 // property.SetValue(XTYPE, Convert.ChangeType(val.Trim(), valtype), null); //} //else //{ // flog = false; //} //如果是非必填字段,则不用判断 if (nonMFileds.Contains(filed.FiledName)) flog = true; //验证是否为空 如果为空则返回true if (filed.IsRequired) { flog = true; } //验证连接地址是否是死链接 if (filed.VerificationStrType == VerificationStr.checkURL) { //检测死链接 if (!CheckUrlVisit(val)) { cheurl = false; } if (!cheurl) { //如果是死链接 将文本框设置为红色 txtControl.Attributes.Add("style", "border-color: red;"); } } if (!flog) { //如果必须填写的数据没有填写则将文本框设置为红色 txtControl.Attributes.Add("style", "border-color: red;"); } } } } return flog == cheurl; #endregion }

/// <summary> /// 直接保存页面信息 不验证是否填写完毕 /// </summary> /// <param name="field_tab_content"></param> /// <param name="type"></param> /// <param name="XTYPE"></param> public void Save1(HtmlGenericControl field_tab_content, Type type, object XTYPE) { #region 赋值 foreach (var filed in fileds) { //取到属性 PropertyInfo property = type.GetProperty(filed.FiledName.ToUpper()); if (property == null) property = type.GetProperty(filed.FiledName); var valtype = property.PropertyType; if (valtype.IsGenericType && valtype.GetGenericTypeDefinition().Equals(typeof(Nullable<>)))//判断convertsionType是否为nullable泛型类 { //如果type为nullable类,声明一个NullableConverter类,该类提供从Nullable类到基础基元类型的转换 System.ComponentModel.NullableConverter nullableConverter = new System.ComponentModel.NullableConverter(valtype); //将type转换为nullable对的基础基元类型 valtype = nullableConverter.UnderlyingType; } var specialContorl = SpecialControls.FirstOrDefault(p => p.FiledName.ToLower() == filed.FiledName.ToLower()); if (specialContorl != null) { #region 特殊控件取值 switch (specialContorl.CType) { case ContorlType.select: DropDownList ddl = field_tab_content.FindControl("S_" + filed.FiledName) as DropDownList; if (ddl != null) { var ddlVal = ddl.SelectedItem.Value.Trim(); if (ddlVal != "") property.SetValue(XTYPE, Convert.ChangeType(ddlVal, valtype), null); } break; case ContorlType.fileUpload: FileUpload file = field_tab_content.FindControl("S_" + filed.FiledName) as FileUpload; IList<System.Web.HttpPostedFile> lis = file.PostedFiles;//使用fileupload控件获取上传文件的文件名 string fileNames = ""; foreach (System.Web.HttpPostedFile item in lis) { string strName = item.FileName; if (strName != "")//如果文件名存在 { bool fileOK = false; int i = strName.LastIndexOf(".");//获取.的索引顺序号 string kzm = strName.Substring(i);//获取文件扩展名的另一种方法 string fileExtension = System.IO.Path.GetExtension( string newName = item.FileName.Substring(0, i) + "_" + DateTime.Now.ToString("HHmmssfff");//生成新的文件名,保证唯一性 string fullname = GetUpLoadPath(); string juedui = Server.MapPath(fullname);//设置文件保存的本地目录绝对路径,对于路径中的字符“\”在字符串中必须以“\\”表示,因为“\”为特殊字符。或者可以使用上一行的给路径前面加上@ string newFileName = juedui + newName + kzm; fileOK = CheckFileExt(kzm); if (fileOK) { try { // 判定该路径是否存在 if (!Directory.Exists(juedui)) Directory.CreateDirectory(juedui); string file1 = file.PostedFile.FileName; string file2 = file.PostedFile.ContentLength + ""; string file3 = file.PostedFile.ContentType; string filename = newName + kzm; file.PostedFile.SaveAs(newFileName);//将文件存储到服务器上 fileNames += fullname + newName + kzm + ";"; } catch (Exception) { // } } else { // } } } HiddenField hidFileNames = field_tab_content.FindControl("S_HID_" + filed.FiledName) as HiddenField; if (hidFileNames != null) property.SetValue(XTYPE, Convert.ChangeType(hidFileNames.Value + fileNames, valtype), null); else property.SetValue(XTYPE, Convert.ChangeType(fileNames, valtype), null); break; case ContorlType.uedit: HtmlTextArea txtControls = field_tab_content.FindControl("S_" + filed.FiledName) as HtmlTextArea; var ueditVal = txtControls.InnerText; property.SetValue(XTYPE, Convert.ChangeType(ueditVal, valtype), null); break; } #endregion } else { //一般控件取值 TextBox txtControl = field_tab_content.FindControl("S_" + filed.FiledName) as TextBox; if (txtControl != null) { var val = txtControl.Text.Trim();//txtControl.Text.Trim(); decimal dl = 0; DateTime dt; if (valtype.Name == "Decimal" || valtype.Name == "Single" || valtype.Name == "Double" || valtype.Name == "Int32") { if (decimal.TryParse(val, out dl)) val = dl.ToString(); else { val = 0 + ""; } } else if (valtype.Name == "DateTime") { if (!string.IsNullOrEmpty(val)) { if (DateTime.TryParse(val, out dt)) val = dt.ToString(); else { val = DateTime.Now + ""; } } } //为空且为时间类型-->设置成默认时间(有其它为空的类型 直接添加else if 就OK) if (string.IsNullOrEmpty(val) && valtype.Name == "DateTime") { property.SetValue(XTYPE, Convert.ChangeType(DateTime.MinValue, valtype), null); } else if (string.IsNullOrEmpty(val) && (valtype.Name == "Int32" || valtype.Name == "Int16" || valtype.Name == "Decimal" || valtype.Name == "Single" || valtype.Name == "Double" || valtype.Name == "Float")) { property.SetValue(XTYPE, Convert.ChangeType(0, valtype), null); } else { property.SetValue(XTYPE, Convert.ChangeType(val, valtype), null); } } } } #endregion }
}
3.如何使用呢
例如:aspx页面

<form class="stdform" runat="server"> <div id="field_tab_content" runat="server"></div> <div style="clear: both; text-align: center"> <asp:Button ID="btnSave" runat="server" Text=" 保 存 " OnClientClick="return GetFullLists()" OnClick="btnSave_Click" /> <asp:Button ID="btnCommit" runat="server" Text=" 提 交 " OnClientClick="return GetFullLists()" OnClick="btnCommit_Click" /> <asp:Button ID="btnReport" CssClass="submit radius2" runat="server" Text=" 上 报 " OnClientClick="return GetFullLists()" OnClick="btnReport_Click" /> <input type="reset" class="reset radius2" data-reveal-id="myModal" value="退回" runat="server" id="btnReSet" /> </div> <div id="myModal" class="reveal-modal"> <h2>请填写退回原因</h2> <asp:TextBox ID="txtReset" runat="server" TextMode="MultiLine" Height="100px"></asp:TextBox> <asp:Button ID="btnReject" CssClass="submit radius2" runat="server" Text=" 确定退回 " OnClientClick="return ResetValid()" OnClick="btnReject_Click" /> <a class="close-reveal-modal">×</a> </div> </form>
cs页面
public partial class ExpertCerditRecordEdit : CreateModel {
Type type; decimal id = 0;

protected void Page_Init(object Gsernder, EventArgs e) { //初始化类型 this.XTYPE = new S_ZEXPERTCERDITRECORD(); List<DictionaryEntry> industriesTypeLists = new List<DictionaryEntry>(); using (LsyjEntities data = new LsyjEntities()) { if (Request["id"] != null && Request["id"] != "0") { id = Convert.ToDecimal(Request["id"]); //通过id查询相应的数据信息 XTYPE = data.S_ZEXPERTCERDITRECORD.FirstOrDefault(p => p.RECORDID == id); //报送状态提示 if (((S_ZEXPERTCERDITRECORD)XTYPE).ISSENDOK == -1 || ((S_ZEXPERTCERDITRECORD)XTYPE).ISSENDOK == -2) Remark = ((S_ZEXPERTCERDITRECORD)XTYPE).REMARK; } else { #region 新增项目时设置初始值 //新增,自动赋值 //公共服务平台标识码 ((S_ZEXPERTCERDITRECORD)XTYPE).PUBSERVICEPLATCODE = ConfigurationManager.AppSettings["PubServicePlatCode"]; //默认交易平台标识码 ((S_ZEXPERTCERDITRECORD)XTYPE).PLATFORMCODE = ConfigurationManager.AppSettings["PlatformCode"]; ((S_ZEXPERTCERDITRECORD)XTYPE).ISDEL = 0; ((S_ZEXPERTCERDITRECORD)XTYPE).DATATIMESTAMP = DateTime.Now; #endregion } var query = from item in data.S_CREWARDPUNISHMENTTYPE select new { key = item.CODE + item.NAME, value = item.CODE.Trim() }; query.ToList().ForEach(p => industriesTypeLists.Add(new DictionaryEntry(p.key, p.value.Trim()))); } #region MyRegion //获取S_ZEXPERTCERDITRECORD类的类型 type = new S_ZEXPERTCERDITRECORD().GetType(); GetType gety = new GetType(); //EditPageControl下的属性 //FiledName 需要设置隐藏显示是否必填的名称,所有字母必须大写才有效 //CType 设置特殊的类型select, uedit, fileUpload 下拉列表,文本编辑器,文件上传 //如果是select类型则需要填写Value值 //isRequery 是否必填 SpecialControls.Add(new EditPageControl() { FiledName = "REWARDPUNISHMENTTYPE", CType = ContorlType.select, Value = industriesTypeLists }); //FiledInfo下的属性 //设置相应的IsRequired 设置为非必填(这里与正常逻辑有点区别)默认为必填IsRequired=false(必填);IsRequired=true(不必填) //VerificationStrType 设置需要验证的数据类型 省份证、时间类型、是否为空。。。。 //FiledName 需要设置隐藏显示是否必填的名称,所有字母必须大写才有效 //AllLine 设置占全行 //eqlen 设置必须填写多少位 //errormessage 错误提示 暂时没有使用到 //FiledDesc 文本框前的相应提示 //Index 设置排序 //IsHide 设置是否显示 //length 设置最大长度 现在是直接从数据库读取进行填写 仅限于NVARCHAR 和VARCHAR类型 //minlen 设置可填写的最小值 //Placeholder水印提示 //Readonly 设置只读 fileds.Add(new FiledInfo() { FiledName = "IDCARD", VerificationStrType = VerificationStr.card }); fileds.Add(new FiledInfo() { FiledName = "BIDSECTIONNAME", IsRequired = true }); fileds.Add(new FiledInfo() { FiledName = "BIDSECTIONCODE", IsRequired = true }); fileds.Add(new FiledInfo() { FiledName = "ENDDATE", IsRequired = true }); //通用方法 可设置ID隐藏 没有把握则不使用这个 gety.GetAll(type, SpecialControls, fileds); //如果是审核用户则将所有文本框下拉选择设置为只读 if ((int)userInfo.USERROLE == 2) { //设置只读 isReadonly = true; } //创建编辑表单 this.CreateData1(type); //将创建的表单显示添加到页面上 field_tab_content.Controls.Add(Cache[type.Name + "htmlDD"] as Control); #endregion }

protected void btnCommit_Click(object sender, EventArgs e) { //公用类进行页面数据获取 if (this.Save1(field_tab_content, type, XTYPE, "ok")) { //将XTYPE 转换为相应的类 S_ZEXPERTCERDITRECORD project = XTYPE as S_ZEXPERTCERDITRECORD; //数据规则和完整性检测 StringBuilder strError = new StringBuilder(); //if (project.INVESTPROJECTCODE.Length != 24) // strError.Append("投资项目统一代码字段应该为24位,不符合长度要求!\\n "); //if (project.INDUSTRIESTYPE == "0") // strError.Append("请选择项目行业分类!\\n "); if (strError.Length > 0) { Response.Write(string.Format("<script type='text/javascript'>alert('{0}');</script>", strError.ToString())); return; } try { using (LsyjEntities data = new LsyjEntities()) { //0:编辑;1,提交审核;-1,审核退回;2,报送成功;-2,报送异常 project.ISSENDOK = 1; data.Entry(project).State = System.Data.EntityState.Modified; //记录日志 DataLog.AddOperationLog(data, Request.UserHostAddress, (int)userInfo.ID, "数据ID" + project.RECORDID + "提交审核", OperationType.Commit, project.RECORDID, "S_ZEXPERTCERDITRECORD"); data.SaveChanges(); } var url = HttpContext.Current.Request.Url.AbsolutePath.ToString().Replace("Edit", "List").Replace("edit", "list"); Response.Write(string.Format("<script type='text/javascript'>alert('{0}'); window.location.href ='" + url + "';</script>", "提交成功")); } catch (Exception ex) { Common.WriteExceptionLog(ex); Response.Write("<script type='text/javascript'>alert('提交失败\\n'" + ex.Message + ");</script>"); } } }

protected void btnSave_Click(object sender, EventArgs e) { if (this.Save1(field_tab_content, type, XTYPE, "ok")) { S_ZEXPERTCERDITRECORD project = XTYPE as S_ZEXPERTCERDITRECORD; using (LsyjEntities data = new LsyjEntities()) { //if (project.ANNOUNCEMENTCODE.Length > 10) //{ // Response.Write(string.Format("<script type='text/javascript'>alert('{0}');</script>", "公示编号最长长度为10")); // return; //} if (project.RECORDID == 0) { project.RECORDID = GetSerialNO.GetNextSequence("SQ_S_ZEXPERTCERDITRECORD"); project.ISSENDOK = 0; project.ISDEL = 0; project.DATASOUSE = userInfo.TEMPNAME; data.S_ZEXPERTCERDITRECORD.Add(project); //记录日志 DataLog.AddOperationLog(data, Request.UserHostAddress, (int)userInfo.ID, "数据ID" + project.RECORDID + ",新增", OperationType.Add, project.RECORDID, "S_ZEXPERTCERDITRECORD"); } else { data.Entry(project).State = System.Data.EntityState.Modified; //记录日志 DataLog.AddOperationLog(data, Request.UserHostAddress, (int)userInfo.ID, "数据ID" + project.RECORDID + ",修改", OperationType.Edit, project.RECORDID, "S_ZEXPERTCERDITRECORD"); } data.Configuration.ValidateOnSaveEnabled = false; data.SaveChanges(); data.Configuration.ValidateOnSaveEnabled = false; } Response.Redirect(HttpContext.Current.Request.RawUrl.ToLower().Trim('/').Split('/')[1].Split('?')[0] + "?id=" + project.RECORDID); } //toubiaoSoapClient tou = new toubiaoSoapClient(); //var result = tou.AddBidder("6C4F3C6D-30BA-4079-B570-ACC7C6B0AE36", text.ToString()); }
}
来来来 见证一下我们经理的时间预估的能力--所有表,不仅仅只是新增修改列表这些基本的功能,还要像省上上报数据。字段辣么多表那么多用winform开发如果不想点其他的办法,就连页面粘贴复制粘贴复制我都不知道要多久才能完成这个项目
扫描二维码关注公众号,回复:
1604590 查看本文章
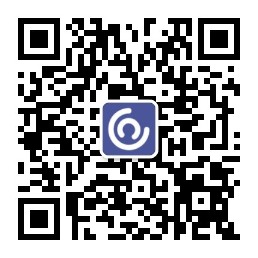