勤时当勉励 岁月不待人
C/C++ 游戏开发
如果你是从现在关注的老粉的话,你可能会有点疑惑“how old are you?”(怎么老是你?)
唉,没办法我也不想的,但是月末了参加新星计划和2023年博客之星的评选只能更新的勤快一点喽!废话不多说,咱们直接开始吧!
五子棋的实战
前言
今天带来的内容是五子棋项目的具体实现,其实咱们上回已经实现了三子棋,
链接如下:
【C语言】三子棋详解(包教包会的那种)
但是呢,上回咱们的三子棋其实还有非常多需要优化的地方,我们今天就来优化一下并把它改为五子棋。
一.上回三子棋的源码
- 上回讲过的东西就不再缀叙了,详情请看上方链接,这里就只把上回的源码贴出来方便大家更好的理解之后的内容。
- 以下为三子棋具体的每个文件中的源码哦,需要自取
1.game.h(头文件)
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
#define Col 3
#define Row 3
void InitBoard(char board[Row][Col], int row, int col);//初始化棋盘
void PrintBoard(char board[Row][Col], int row, int col);//打印棋盘
void PlayBoard(char board[Row][Col], int row, int col);//玩游戏
void ComputerBoard(char board[Row][Col], int row, int col);//电脑下
int IsFull(char board[Row][Col], int row, int col);//棋盘是否已满
int Is_Win(char board[Row][Col], int row, int col);//判断谁赢
2.game.c(定义函数的文件)
#include"game.h"
void InitBoard(char board[Row][Col], int row, int col)
{
int i = 0;
int j = 0;
for (i = 0; i < row; i++)
{
for (j =0; j < col; j++)
{
board[i][j] = ' ';
}
}
}
void PrintBoard(char board[Row][Col], int row, int col)
{
int i = 0;
//打印数据
for (i = 0; i < row; i++)
{
int j = 0;
for (j = 0; j < col; j++)
{
printf(" %c ", board[i][j]);
if (j < col - 1)
printf("|");
}
printf("\n");
if (i < row - 1)
{
int j = 0;
for (j = 0; j < col; j++)
{
printf("---");
if (j < col - 1)
printf("|");
}
printf("\n");
}
}
}
void PlayBoard(char board[Row][Col], int row, int col)
{
int x, y = 0;
while (1)
{
printf("玩家下棋>:\n");
printf("请输入要下的坐标,中间用空格隔开\n");
scanf("%d %d", &x, &y);
//判断输入坐标的合法性
if (x >= 1 && x <= row && y >= 1 && y <= col)
{
if (board[x - 1][y - 1] == ' ')
{
board[x - 1][y - 1] = '*';
break;
}
else
printf("坐标已被占用,请重新输入\n");
}
else
printf("坐标非法,请重新输入\n");
}
}
void ComputerBoard(char board[Row][Col], int row, int col)
{
int x = 0;
int y = 0;
printf("电脑下棋:>\n");
while(1)
{
x = rand() % row;//生成0-row-1的数
y = rand() % col;//生成0-col-1的数
if (board[x][y] == ' ')
{
board[x][y] = '#';
break;
}
}
}
int IsFull(char board[Row][Col], int row, int col)
{
int i ,j;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
if (board[i][j] == ' ')
return 0;
}
}
return 1;
}
int Is_Win(char board[Row][Col], int row, int col)
{
int x = 0;
int y = 0;
//竖着
if (board[x][0] == board[x][1] && board[x][1] == board[x][2] && board[x][0] !=' ')
{
return board[x][0];
}
//横着
if (board[0][y] == board[1][y] && board[1][y] == board[2][y] && board[0][y] != ' ')
{
return board[0][y];
}
//对角线
if (board[0][0] == board[1][1] && board[1][1] == board[2][2] && board[1][1] != ' ')
return board[1][1];
if (board[0][2] == board[1][1] && board[1][1] == board[2][0] && board[1][1] != ' ')
return board[1][1];
if (IsFull(board, row, col) == 1)
{
return 'Q';
}
return 'C';
}
3.test.c(主文件)
#include"game.h"
void menu()//打印菜单
{
printf("*****************************\n");
printf("*********** 1.play **********\n");
printf("*********** 0.exit **********\n");
printf("*****************************\n");
}
void game()
{
char board[Row][Col] = {
0 };
InitBoard(board, Row, Col);
PrintBoard(board,Row, Col);
char ret = 0;
while (1)
{
PlayBoard(board, Row, Col);
PrintBoard(board, Row, Col);
ret = Is_Win(board, Row, Col);
if (ret != 'C')
break;
ComputerBoard(board, Row, Col);
PrintBoard(board, Row, Col);
ret = Is_Win(board, Row, Col);
if (ret != 'C')
break;
}
if (ret == '*')
printf("玩家赢\n");
if (ret == '#')
printf("电脑赢\n");
if (ret == 'Q')
printf("平局\n");
}
int main()
{
srand((unsigned int)time(NULL));
int input = 0;
do {
menu();
printf("请选择:> ");
scanf("%d",&input);
switch (input)
{
case 1:
game();
break;
case 0:
printf("退出游戏\n");
break;
default:
printf("输入错误,请重新输入\n");
break;
}
}while(input);
return 0;
}
- 看看游戏效果
二.五子棋
1.棋盘大小
- 三子棋的棋盘大小是3*3的,对于五子棋来说显然不够,这下咱们之前定义的Row与Col就发挥了作用,当我们想改变棋盘大小时,只需要改变它俩的值即可!
#define Col 10
#define Row 10
扫描二维码关注公众号,回复:
15787304 查看本文章
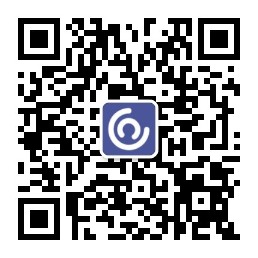
- 效果如图,如果你还想改的更大一点,当然也可以
- 15*15的棋盘
- 这一步完成
2.判断胜负条件
- 我们现在既然是五子棋,那么判断胜负的条件自然也要变化一下啦,我们先回顾一下三子棋中判断胜负的函数
int IsFull(char board[Row][Col], int row, int col)
{
int i ,j;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
if (board[i][j] == ' ')
return 0;
}
}
return 1;
}
int Is_Win(char board[Row][Col], int row, int col)
{
int x = 0;
int y = 0;
//竖着
if (board[x][0] == board[x][1] && board[x][1] == board[x][2] && board[x][0] !=' ')
{
return board[x][0];
}
//横着
if (board[0][y] == board[1][y] && board[1][y] == board[2][y] && board[0][y] != ' ')
{
return board[0][y];
}
//对角线
if (board[0][0] == board[1][1] && board[1][1] == board[2][2] && board[1][1] != ' ')
return board[1][1];
if (board[0][2] == board[1][1] && board[1][1] == board[2][0] && board[1][1] != ' ')
return board[1][1];
if (IsFull(board, row, col) == 1)
{
return 'Q';
}
return 'C';
}
- 首先我们的判定要从三子改为五子
- 其次,我们发现,上面的代码不具有灵活性,注意这里:
//竖着
if (board[x][0] == board[x][1] && board[x][1] == board[x][2] && board[x][0] !=' ')
{
return board[x][0];
}
//横着
if (board[0][y] == board[1][y] && board[1][y] == board[2][y] && board[0][y] != ' ')
{
return board[0][y];
}
//对角线
if (board[0][0] == board[1][1] && board[1][1] == board[2][2] && board[1][1] != ' ')
return board[1][1];
if (board[0][2] == board[1][1] && board[1][1] == board[2][0] && board[1][1] != ' ')
return board[1][1];
- 这中间我们发现它的判定方式是直接与棋盘挂钩的,只能在3*3的棋盘中使用,最明显的地方是对角线,上面代码的判定方式是直接判定(1,1)(2,2)(3,3)或者(1,3)(2,2)(3,1)中存放的元素是否相等,一旦我们把棋盘的大小改变,就无法正确判定输赢了。比如这样:
- 此时我们已经获胜了,但是程序却没有判定我们胜利
- 改进代码如下:
int Is_Win(char board[Row][Col], int row, int col)
{
int i = 0;
int j = 0;
//竖着
if (j < col - 4)//防止越界
if (board[i][j] == board[i][j + 1] && board[i][j] ==board[i][j + 2]&& board[i][j] == board[i][j + 3] && board[i][j]== board[i][j + 4])
return board[i][j];
//横着
if (i < row - 4)
if(board[i][j] == board[i][j + 1] && board[i][j] ==board[i][j + 2]&& board[i][j] == board[i][j + 3] && board[i][j]== board[i][j + 4])
return board[i][j];
//左对角线连成五子
if (i < row - 4 && j < col - 4)
if (board[i][j] ==board[i + 1][j + 1] && board[i][j] ==board[i + 2][j + 2]&& board[i][j] == board[i + 3][j + 3] && board[i][j] == board[i + 4][j + 4])
return board[i][j];
// 右对角线连成五子
if (i < row - 4 && j > 4)
if (board[i][j] == board[i + 1][j - 1] &&board[i][j] ==board[i + 2][j - 2]&& board[i][j] == board[i + 3][j - 3] && board[i][j] == board[i + 4][j - 4])
return board[i][j];
if (IsFull(board, row, col) == 1)
{
return 'Q';
}
return 'C';
}
- 把三子变成了五子,同时增加了代码的灵活性,方便我们以后玩六子棋等什么的。
3.试玩一下
- 游戏效果如图
- 虽然格子简陋一点,但是我们需要的功能完美实现!
4.五子棋的源码
- 下面把源码给大家分享一下,方便大家试玩以及修改自己在编写时可能出现的错误
game.h(五子棋头文件)
#define _CRT_SECURE_NO_WARNINGS 1
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
#define Col 10
#define Row 10
void InitBoard(char board[Row][Col], int row, int col);//初始化棋盘
void PrintBoard(char board[Row][Col], int row, int col);//打印棋盘
void PlayBoard(char board[Row][Col], int row, int col);//玩游戏
void ComputerBoard(char board[Row][Col], int row, int col);//电脑下
int IsFull(char board[Row][Col], int row, int col);//棋盘是否已满
int Is_Win(char board[Row][Col], int row, int col);//判断谁赢
test.c(测试五子棋的主函数文件)
#include"game.h"
void menu()//打印菜单
{
printf("*****************************\n");
printf("*********** 1.play **********\n");
printf("*********** 0.exit **********\n");
printf("*****************************\n");
}
void game()
{
char board[Row][Col] = {
0 };
InitBoard(board, Row, Col);
PrintBoard(board, Row, Col);
char ret = 0;
while (1)
{
PlayBoard(board, Row, Col);
PrintBoard(board, Row, Col);
ret = Is_Win(board, Row, Col);
if (ret != 'C')
break;
ComputerBoard(board, Row, Col);
PrintBoard(board, Row, Col);
ret = Is_Win(board, Row, Col);
if (ret != 'C')
break;
}
if (ret == '*')
printf("玩家赢\n");
if (ret == '#')
printf("电脑赢\n");
if (ret == 'Q')
printf("平局\n");
}
int main()
{
srand((unsigned int)time(NULL));
int input = 0;
do {
menu();
printf("请选择:> ");
scanf("%d", &input);
switch (input)
{
case 1:
game();
break;
case 0:
printf("退出游戏\n");
break;
default:
printf("输入错误,请重新输入\n");
break;
}
} while (input);
return 0;
}
game.c(五子棋函数的定义文件)
#include"game.h"
void InitBoard(char board[Row][Col], int row, int col)
{
int i = 0;
int j = 0;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
board[i][j] = ' ';
}
}
}
void PrintBoard(char board[Row][Col], int row, int col)
{
int i = 0;
//打印数据
for (i = 0; i < row; i++)
{
int j = 0;
for (j = 0; j < col; j++)
{
printf(" %c ", board[i][j]);
if (j < col - 1)
printf("|");
}
printf("\n");
if (i < row - 1)
{
int j = 0;
for (j = 0; j < col; j++)
{
printf("---");
if (j < col - 1)
printf("|");
}
printf("\n");
}
}
}
void PlayBoard(char board[Row][Col], int row, int col)
{
int x, y = 0;
while (1)
{
printf("玩家下棋>:\n");
printf("请输入要下的坐标,中间用空格隔开\n");
scanf("%d %d", &x, &y);
//判断输入坐标的合法性
if (x >= 1 && x <= row && y >= 1 && y <= col)
{
if (board[x - 1][y - 1] == ' ')
{
board[x - 1][y - 1] = '*';
break;
}
else
printf("坐标已被占用,请重新输入\n");
}
else
printf("坐标非法,请重新输入\n");
}
}
void ComputerBoard(char board[Row][Col], int row, int col)
{
int x = 0;
int y = 0;
printf("电脑下棋:>\n");
while (1)
{
x = rand() % row;//生成0-row-1的数
y = rand() % col;//生成0-col-1的数
if (board[x][y] == ' ')
{
board[x][y] = '#';
break;
}
}
}
int IsFull(char board[Row][Col], int row, int col)
{
int i, j;
for (i = 0; i < row; i++)
{
for (j = 0; j < col; j++)
{
if (board[i][j] == ' ')
return 0;
}
}
return 1;
}
int Is_Win(char board[Row][Col], int row, int col)
{
int i = 0;
int j = 0;
//竖着
if (j < col - 4)//防止越界
if (board[i][j] == board[i][j + 1] && board[i][j] ==board[i][j + 2]&& board[i][j] == board[i][j + 3] && board[i][j]== board[i][j + 4])
return board[i][j];
//横着
if (i < row - 4)
if(board[i][j] == board[i][j + 1] && board[i][j] ==board[i][j + 2]&& board[i][j] == board[i][j + 3] && board[i][j]== board[i][j + 4])
return board[i][j];
//左对角线连成五子
if (i < row - 4 && j < col - 4)
if (board[i][j] ==board[i + 1][j + 1] && board[i][j] ==board[i + 2][j + 2]&& board[i][j] == board[i + 3][j + 3] && board[i][j] == board[i + 4][j + 4])
return board[i][j];
// 右对角线连成五子
if (i < row - 4 && j > 4)
if (board[i][j] == board[i + 1][j - 1] &&board[i][j] ==board[i + 2][j - 2]&& board[i][j] == board[i + 3][j - 3] && board[i][j] == board[i + 4][j - 4])
return board[i][j];
if (IsFull(board, row, col) == 1)
{
return 'Q';
}
return 'C';
}
总结
- 结合咱们上回三子棋实现的详解,其实五子棋也没啥难的,如果你上次的三子棋没有自己独立的编写过,真的墙裂建议你试试五子棋的,毕竟代码只有自己亲手编写过了才能真正做到对它的理解。
- 以上就是今天的所有内容了,如果你有任何疑问欢迎在评论区指出或者私信我,我看到后会第一时间回复的哦!
新人博主创作不易,如果感觉文章内容对你有所帮助的话不妨三连一下这个新人博主再走呗。你们的支持就是我更新的动力!!!
(可莉请求你们三连支持一下博主!!!点击下方评论点赞收藏帮帮可莉吧)