React中的路由react-router
URL的hash
- URL的hash就是锚点,本质上是改变window.location的href属性;
- 我们可以直接赋值location.hash来改变href,但是页面不发生刷新;
<body>
<div id="app">
<a href="#/home">首页</a>
<a href="#/about">关于</a>
<div class="router-view"></div>
</div>
</body>
<script>
const routerView = document.getElementsByClassName("router-view")[0];
//监听URL的改变
window.addEventListener("hashchange",()=>{
switch(location.hash){
case "#/home":
routerView.innerHTML = "首页";
break;
case "#/about":
routerView.innerHTML = "关于";
break;
default:
routerView.innerHTML = "";
}
})
</script>
HTML5的history
- history接口是HTML5新增的,它有六种模式改变URL而不刷新页面;
方法 | 解释 |
---|---|
history.back() | 在浏览器历史记录里前往上一页, 用户可点击浏览器左上角的返回(译者注:←)按钮模拟此方法. 等价于 history.go(-1) |
history.forward() | 在浏览器历史记录里前往下一页,用户可点击浏览器左上角的前进(译者注:→)按钮模拟此方法. 等价于 history.go(1) |
history.go() | 通过当前页面的相对位置从浏览器历史记录( 会话记录 )加载页面。比如:参数为-1的时候为上一页,参数为1的时候为下一页. |
history.pushState() | 按指定的名称和URL(如果提供该参数)将数据push进会话历史栈,数据被DOM进行不透明处理;你可以指定任何可以被序列化的javascript对象 |
history.replaceState() | 按指定的数据,名称和URL(如果提供该参数),更新历史栈上最新的入口。这个数据被DOM 进行了不透明处理。你可以指定任何可以被序列化的javascript对象。 |
<body>
<div>
<div id="app">
<a href="/home">首页</a>
<a href="/about">关于</a>
<div class="router-view"></div>
</div>
</div>
<script>
const routerView = document.getElementsByClassName("router-view")[0];
const aEL = document.getElementsByTagName("a");
for (let i = 0; i < aEL.length; i++) {
aEL[i].addEventListener("click", e => {
e.preventDefault();
const href = aEL[i].getAttribute("href");
history.pushState({
}, "", href);
urlChange();
})
}
// for(let el of aEL){
// el.addEventListener("click",e => {
// e.preventDefault();
// const href = el.getAttribute("href");
// history.pushState({},"",href);
// })
// }
//执行返回操作时,依然来到urlChange
window.addEventListener("popstate",urlChange);
function urlChange() {
switch (location.pathname) {
case "/home":
routerView.innerHTML = "首页";
break;
case "/about":
routerView.innerHTML = "关于";
break;
default:
routerView.innerHTML = "";
}
}
</script>
</body>
Router的基本使用
react-router最主要的API是给我们提供的一些组件:
-
BrowserRouter或HashRouter
1、Router中包含了对路径改变的监听,并且会将相应的路径传递给子组件;
2、BrowserRouter使用history模式;
3、HashRouter使用hash模式; -
Link和NavLink
1、 通常路径的跳转是使用Link组件,最终会被渲染成a元素;
2、 NavLink是在Link基础上增加了一些样式属性;
3、to属性:Link中最重要的属性,用于设置跳转到的路径; -
Route
1、Route用于路径的匹配;
2、path属性:用于设置匹配到的路径;
3、component属性:设置匹配到路径后,渲染的组件;
4、exact:精准匹配,只有精准匹配到完全一致的路径,才会渲染对应的组件; -
NavLink组件的使用
<NavLink>是<Link>的特定版本,会在匹配上当前的url的时候给已渲染的元素添加参数
- activeStyle(object):当元素被选中时,为此元素添加样式
- activeClassName(string):设置选中样式,默认值为active
- exact(boolean):是否精准匹配
1、注意:在组件添加exact属性,能够让路由精准匹配到url
2、若不添加exact属性的话,在"/about"的组件和"/profile"组件中也会渲染出"/"的组件
<NavLink exact to="/" activeStyle={
{
color:"blue"}}>首页</NavLink>
<NavLink to="/about" activeStyle={
{
color:"blue"}}>关于</NavLink>
<NavLink to="/profile" activeStyle={
{
color:"blue"}}>首页</NavLink>
Switch组件的使用
- 渲染第一个被location匹配到的并且作为子元素的或者
问题:在/about路径匹配到的同时,/:userid也被匹配到,并且最后一个NoMatch组件总是被匹配到,这该如何解决?
<Route exact path="/" exact component={
home} />
<Route path="/about" component={
about} />
<Route path="/profile" component={
profile} />
<Route path="/:id" component={
user} />
<Route path="/user" component={
noMatch} />
答:这个时候可以用Switch组件将所有的Route组件进行包裹。
<Switch>
<Route exact path="/" exact component={
home} />
<Route path="/about" component={
about} />
<Route path="/profile" component={
profile} />
<Route path="/:id" component={
user} />
<Route path="/user" component={
noMatch} />
</Switch>
Redirect组件的使用
- Redirect用于路由的重定向,它会执行跳转到对应的to路径中
import React, {
PureComponent } from 'react'
import {
Redirect } from 'react-router-dom';
export default class user extends PureComponent {
constructor(props){
super(props);
this.state ={
isLogin: true
}
}
render() {
return this.state.isLogin ? (
<div>
<h2>user</h2>
<h2>用户名:boge</h2>
</div>
) : <Redirect to="/login" />
}
}
手动实现路由跳转
比如,若想实现一个普通组件(button按钮)点击进行路由的跳转的时候,要满足两个条件:
- 普通组件必须包裹在Router组件之内;
- 普通组件使用withRouter高阶组件包裹;
src/App.js文件
import React, {
PureComponent } from 'react'
import {
withRouter} from 'react-router-dom'
class App extends PureComponent {
}
export default withRouter(App);
src/index.js文件
import React from 'react';
import ReactDOM from 'react-dom';
import {
BrowserRouter } from 'react-router-dom';
import App from './App';
ReactDOM.render(
<BrowserRouter> //这里要包裹一个Router组件才能使用withRouter高阶组件
<App />
</BrowserRouter>,
document.getElementById('root')
);
动态路由
传递参数有三种方式:
- 动态路由的方式;
- search传递参数;
- Link中to传入对象;
动态路由的概念–指的是路由中的路径不是固定的:
- 动态路由方式
1、 假如/detail的路径对应 一个组件Detail
2、若将路径中的Route匹配时写成/detail/:id,那么/detail/123,/detail/xyz都可以匹配到该Route并进行显示
<NavLink to="/detail/abc123">详情</NavLink>
<Route path="/detail/:id" component={
detail} />
- search传递参数
<NavLink to="/detail2?name=boge&age=20">详情2</NavLink>
- Link中to传入对象
<NavLink to={
{
pathname: "/detail2",
search:"?name=abc",
state: id
}}>详情3</NavLink>
react-router-config
若希望将所有的路由配置放到一个地方进行集中管理,这个时候可以使用react-router-config来完成。
- yarn add react-router-config
- 配置路由映射的关系数组
src/router/index.js文件
import home from '../pages/home';
import about,{
AboutHistory,AboutCulture,AboutContact,AboutJoin} from '../pages/about';
const routes = [
{
path:"/",
component:home,
exact:true
},
{
path:"/about",
component:about,
routes: [
{
path:"/about",
component: AboutHistory,
exact: true
},
{
path:"/about/culture",
component: AboutCulture,
},
{
path:"/about/contact",
component: AboutContact,
},
{
path:"/about/join",
component: AboutJoin,
}
]
}
]
export default routes;
- 使用renderRoutes函数完成配置
src/App.js文件
扫描二维码关注公众号,回复:
15593302 查看本文章
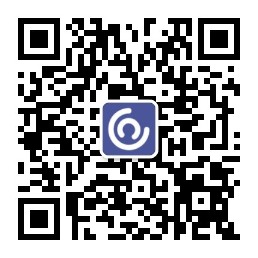
import React, {
PureComponent } from 'react';
import {
NavLink,withRouter} from 'react-router-dom';
import {
renderRoutes} from 'react-router-config';
import routes from './router';
App extends PureComponent {
render() {
const id = "abc"
return (
<div>
<NavLink exact to="/" activeClassName="link-active">首页</NavLink>
<NavLink to="/about" activeClassName="link-active">关于</NavLink>
<NavLink to="/profile" activeClassName="link-active">我的</NavLink>
<NavLink to="/user" activeClassName="link-active">用户</NavLink>
<NavLink to={
`/detail/${
id}`} activeClassName="link-active">详情</NavLink>
<NavLink to={
{
pathname: "/detail2",
search:"?name=abc",
state: id
}} activeClassName="link-active">详情3</NavLink>
<button onClick={
e => this.ToProduct()}>商品</button>
{
renderRoutes(routes)}
</div>
)
}
ToProduct(){
this.props.history.push("/product");
}
}
export default withRouter(App);