路由的定义
路由是根据不同的url地址展示不同的内容或页面
注: 一个针对react设计的路由解决方案,可以友好的帮助解决React components到URL之间的同步映射关系
路由安装
npm i react-router-dom@5
路由的使用
导入路由
import {
HashRouter as Router,Redirect,Route,Switch} from 'react-router-dom'
注:路由模式有HashRouter
和BrowserRouter
两种模式,其中BrowserRouter的路径没有#,样式好看,真正朝后端发请求,后端若没有对应的路径去处理路径,就会404
定义路由
<Route path="/films" component={
Films} />
其中path
是对应的路由路径,component
是与路由对应的组件
Switch的使用
<Switch>
<Route path="/films" component={
Films} />
<Route path="/cinemas" component={
Cinemas} />
<Route path="/center" component={
Center} />
</Switch>
Switch的功能与switch语句功能一样,对传入的路由进行匹配选择,当匹配到一个path后将不再继续进行匹配
Rediret的使用
对于匹配不到的路径,可以使用Redirect进行重定向,直接调转到某个指定页面
<Redirect from='/' to='/films' exact />
注意:Redirect的的使用要与Switch结合使用
,且在Switch中写在Route之后
嵌套路由
在父组件中定义路由
<Switch>
<Route path="/films/nowplaying" component={
nowplaying} />
<Route path="/films/coming" component={
coming}/>
<Route path="/films/comingsoon" component={
comingsoon} />
<Redirect from='/films' to='/films/nowplaying'/>
</Switch>
路由跳转模式
声明式导航
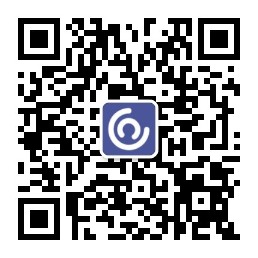
<NavLink to={
"/films"} activeClassName="active">电影</NavLink>
编程式导航
//方式一--通过父组件传的props参数
props.history.push ('/films')
//方式二--引入useHistory
import {
useHistory } from 'react-router-dom'
history.push(`/films`)
路由传参
路由传参共有三种模式–动态路由传参
,query传参
,state传参
动态路由传参
直接将传入的参数放置在路径中
history.push(`/detail/${
id}`)
子组件获取页面id
props.match.params.myid
query传参
将传入的参数赋值到query参数中,自动拼接
history.push({
pathname:"/detail",query:id})
子页面获取页面id
props.location.query
state传参
与query参数相似,将传入的参数赋值到state参数中,自动拼接
history.push({
pathname:"/detail",state:{
myid:id}})
子页面获取页面id
props.location.state.myid
路由拦截
对于某些页面的跳转需要用户满足一些条件,可以通过路由拦截阻止未满足的用户跳转到指定页面
<Route path='/center' render={
(props)=>{
return isAuth()? <Center {
...props}></Center>:<Redirect to='/login' />
}} />
反向代理
Reactke通过Proxy进行反向代理,解决跨域问题
https://facebook.github.io/create-react-app/docs/proxying-api-requests-in-development
安装包下载
npm install http-proxy-middleware --save
在src文件夹下建立
setuoProxy.js
文件
文件内容如下
const {
createProxyMiddleware } = require('http-proxy-middleware');
module.exports = function(app) {
app.use(
'/ajax', //转发请求--接口地址带有/ajax前缀的接口路径才会被转发给target
createProxyMiddleware({
target: 'https://i.maoyan.com', //target是api服务器访问的地址
changeOrigin: true,
})
);
};
注:可配置多个代理
css module
在React中每个组件的css样式会统一出现在主页面,为避免样式覆盖,css module默认局部样式
使用步骤:
-
css文件命名后缀改为
.module.css
-
在组件中引入并可命名为style
import style from './css/Film.module.css'
-
在对应的元素中使用该类名
<div className={ style.film}>
注意:类名可进行字符串拼接
<div className={
style.film +" aaa"}>
在css module文件中定义全局样式
:global(#kerwin){
color:red
}
欢迎各位评论指正,期待你们的支持✨✨✨