目录
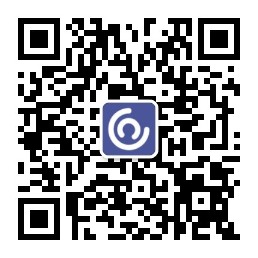
一、概述
反射指程序可以访问、检测和修改它本身状态或行为的一种能力。
程序集包含模块,而模块包含类型,类型又包含成员。反射则提供了封装程序集、模块和类型的对象。
您可以使用反射动态地创建类型的实例,将类型绑定到现有对象,或从现有对象中获取类型。然后,可以调用类型的方法或访问其字段和属性。
优点:
1、反射提高了程序的灵活性和扩展性。
2、降低耦合性,提高自适应能力。
3、它允许程序创建和控制任何类的对象,无需提前硬编码目标类。
缺点:
1、性能问题:使用反射基本上是一种解释操作,用于字段和方法接入时要远慢于直接代码。因此反射机制主要应用在对灵活性和拓展性要求很高的系统框架上,普通程序不建议使用。
2、使用反射会模糊程序内部逻辑;程序员希望在源代码中看到程序的逻辑,反射却绕过了源代码的技术,因而会带来维护的问题,反射代码比相应的直接代码更复杂。
反射(Reflection)有下列用途:
它允许在运行时查看特性(attribute)信息。
它允许审查集合中的各种类型,以及实例化这些类型。
它允许延迟绑定的方法和属性(property)。
它允许在运行时创建新类型,然后使用这些类型执行一些任务。
二、实例化类
要想使用反射来实例化一个类,可以使用 Activator.CreateInstance 方法,但实际上,C# 的反射性能要比 new 差很多,所以在一般情况下,不推荐使用反射,但是也不代表反射不能使用,在很多项目中,反射用到的频率也是很高的。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
object obj = Activator.CreateInstance(type);
MyClass myClass = (MyClass)obj;
Console.WriteLine("年龄:{0}", myClass.Age);
Console.ReadKey();
}
}
public class MyClass
{
public int Age { get; set; } = 12;
}
}
运行:
三、反射赋值
1.字段类型
可以使用 FieldInfo.SetValue 来给字段赋值
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
object obj = Activator.CreateInstance(type);
FieldInfo field = type.GetField("Age");
if (field != null)
field.SetValue(obj, 42);
MyClass myClass = (MyClass)obj;
Console.WriteLine("年龄:{0}", myClass.Age);
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
}
}
运行:
2.属性类型
根据上一节的代码可以看到,我把 Age 这个字段的属性去掉了,如果字段是一个属性, 调用 type.GetField("字段名") 方法返回的是 null。
下面是介绍如何给属性赋值:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
object obj = Activator.CreateInstance(type);
PropertyInfo property = type.GetProperty("UserName");
property.SetValue(obj, "李四");
MyClass myClass = (MyClass)obj;
Console.WriteLine("用户名:{0}", myClass.UserName);
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
public string UserName { get; set; } = "张三";
}
}
运行:
四、获取字段值
获取字段的值,只需要拿到当前类的实体对象就好了
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
object obj = Activator.CreateInstance(type);
MyClass myClass = (MyClass)obj;
Console.WriteLine("年龄:{0}", myClass.Age);
Console.WriteLine("用户名:{0}", myClass.UserName);
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
public string UserName { get; set; } = "张三";
}
}
运行:
五、获取字段名
1.字段类型
可以使用下面的方法来获取所有的字段名,但这种写法并不能获取属性的字段名
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
FieldInfo[] fields = type.GetFields(BindingFlags.Public | BindingFlags.Instance);
foreach (FieldInfo field in fields)
{
Console.WriteLine(field.Name);
}
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
public string UserName { get; set; } = "张三";
}
}
运行:
2.属性类型
代码大致差不多,区别在于,普通的字段使用 GetFields ,属性使用 GetProperties
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
PropertyInfo[] properties = type.GetProperties(BindingFlags.Public | BindingFlags.Instance);
foreach (PropertyInfo property in properties)
{
Console.WriteLine(property.Name);
}
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
public string UserName { get; set; } = "张三";
}
}
运行:
六、获取字段类型
1.字段类型
可以使用 FieldInfo.FieldType 来获取字段的类型
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
FieldInfo field = type.GetField("Age");
Type fieldType = field.FieldType;
Console.WriteLine(fieldType);
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
public string UserName { get; set; } = "张三";
}
}
运行:
2.属性类型
可以使用 PropertyInfo.GetProperty 方法来获取属性的类型
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
PropertyInfo property = type.GetProperty("UserName");
Type propertyType = property.PropertyType;
Console.WriteLine(propertyType);
Console.ReadKey();
}
}
public class MyClass
{
public int Age = 12;
public string UserName { get; set; } = "张三";
}
}
运行:
七、调用方法
可以使用 MethodInfo.Invoke 来调用方法
1.有参数
在这里,参数用的是一个数组,假设参数有两个,那么你在数组中也要加两个对应类型的值
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
object obj = Activator.CreateInstance(type);
MethodInfo method = type.GetMethod("Test");
object[] parameters = new object[] { "老王" };
string result = (string)method.Invoke(obj, parameters);
Console.WriteLine("返回值 {0}" , result);
Console.ReadKey();
}
}
public class MyClass
{
public string Test(string name)
{
return "你的名字:" + name;
}
}
}
运行:
2.无参数
假设方法无参数的时候,传值传空就好了
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace ReflectionTest
{
internal class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
object obj = Activator.CreateInstance(type);
MethodInfo method = type.GetMethod("SayHi");
method.Invoke(obj, null);
Console.ReadKey();
}
}
public class MyClass
{
public void SayHi()
{
Console.WriteLine("你好");
}
}
}
运行:
结束
如果这个帖子对你有所帮助,欢迎 关注 + 点赞 + 留言,谢谢!
end