Collection
集合类体系结构
Collection集合概述和使用
Collection集合概述
- 是单例集合的顶层接口,它表示一组对象,这些对象也称为Collection的元素
- JDK不提供此接口的任何直接实现,它提供更具体的子接口(如Set和List)实现
创建Collection集合的对象
- 多态的方式
- 具体的实现类ArrayList
import java.util.ArrayList;
import java.util.Collection;
//创建Collection集合的对象
//多态的方式
// 具体的实现类ArrayList
public class CollectionDemo {
public static void main(String[] args) {
// 创建Collection集合的对象
Collection<String> c = new ArrayList<String>();
// 添加元素:boolean add(E e)
c.add("hello");
c.add("world");
c.add("java");
// 输出集合对象,重写了tostring方法
System.out.println(c);
}
运行结果:
Collection集合常用方法
方法名 | 说明 |
---|---|
boolean add(E e) | 添加元素 |
boolean remove(Object o) | 从集合中移除指定的元素 |
void clear() | 清空集合中的元素 |
boolean contains(Object o) | 判断集合中是否存在指定的元素 |
boolean isEmpty() | 判断集合是否为空 |
int size() | 集合的长度,也就是集合中元素的个数 |
import java.util.ArrayList;
import java.util.Collection;
//boolean add(E e) 添加元素
//boolean remove(Object o)从集合中移除指定的元素
//void clear()清空集合中的元素
//boolean contains(Object o)判断集合中是否存在指定的元素
//boolean isEmpty()判断集合是否为空
//int size()集合的长度,也就是集合中元素的个数
public class CollectionDemo {
public static void main(String[] args) {
// 创建Collection集合的对象
Collection<String> c = new ArrayList<String>();
// boolean add(E e) 添加元素
// 调用add 永远返回true
// System.out.println(c.add("hello"));
// System.out.println(c.add("world"));
// System.out.println(c.add("world"));
c.add("hello");
c.add("world");
c.add("java");
// boolean remove(Object o)从集合中移除指定的元素
// System.out.println(c.remove("world"));
// System.out.println(c.remove("javaee"));//返回false 因为集合中没有javaee
// void clear()清空集合中的所有元素
// c.clear();
// boolean contains(Object o)判断集合中是否存在指定的元素
// System.out.println(c.contains("world"));
// System.out.println(c.contains("javaee"));//false
// boolean isEmpty()判断集合是否为空
// System.out.println(c.isEmpty());
// int size()集合的长度,也就是集合中元素的个数
System.out.println(c.size());
// 输出集合对象
System.out.println(c);
}
}
运行结果:
Collection集合的遍历
Iterator:迭代器,集合的专用遍历方式
- Iterator iterator():返回此集合中元素的迭代器,同构集合的iterator()方式得到
- 迭代器是通过集合的iterator()方法得到的,所以我们说它是依赖于集合而存在的
Iterator中的常用方法
- E next():返回迭代中的下一个元素
- boolean hasNext():如果迭代具有更多元素,则返回true
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
//Iterator:迭代器,集合的专用遍历方式
// Iterator<E> iterator():返回此集合中元素的迭代器,同构集合的iterator()方式得到
// 迭代器是通过集合的iterator()方法得到的,所以我们说它是依赖于集合而存在的
//Iterator中的常用方法
// E next():返回迭代中的下一个元素
// boolean hasNext():如果迭代具有更多元素,则返回true
public class IteratorDemo {
public static void main(String[] args) {
// 创建集合对象
Collection<String> c = new ArrayList<String>();
// 添加元素
c.add("hello");
c.add("world");
c.add("java");
// Iterator<E> iterator():返回此集合中元素的迭代器,同构集合的iterator()方式得到
Iterator<String> it = c.iterator();
// E next():返回迭代中的下一个元素
// System.out.println(it.next());
// System.out.println(it.next());
// System.out.println(it.next());
// System.out.println(it.next());//NoSuchElementException:表示被请求的元素不存在
// boolean hasNext():如果迭代具有更多元素,则返回true
// if(it.hasNext()) {
// System.out.println(it.next());
// }
// 用while循环改进
while (it.hasNext()) {
String s = it.next();
System.out.println(s);
}
}
}
运行结果:
集合的使用步骤
Collection<String> c = new ArrayList<String>();
String s = "hello";
c.add(s);
c.add("world");
Iterator<String> it = c.iterator();
while (it.hasNext()) {
String ss = it.next();
System.out.println(ss);
}
- 创建集合对象
- 添加元素
2.1 创建元素
2.2 添加元素到集合 - 遍历集合
3.1 通过集合对象获取迭代器
3.2 通过迭代器对象的hasNext()方法判断是否还有元素
3.3 通过迭代器对象的next()方法获取下一个元素
案例:Collection集合存储学生对象并遍历
需求:创建一个存储学生对象的集合,存储3个学生对象,使用程序实现在控制台遍历该集合
//定义学生类
public class Student {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Student() {
super();
}
public Student(String name, int age) {
super();
this.name = name;
this.age = age;
}
}
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
//需求:创建一个存储学生对象的集合,存储3个学生对象,使用程序实现在控制台遍历该集合
public class CollectionDemo2 {
public static void main(String[] args) {
// 创建Collection集合对象
Collection<Student> c = new ArrayList<Student>();
// 创建学生对象
Student s1 = new Student("小白", 12);
Student s2 = new Student("小黑", 13);
Student s3 = new Student("小红", 11);
// 把学生添加到集合
c.add(s1);
c.add(s2);
c.add(s3);
// 遍历集合(迭代器方法)
Iterator<Student> it = c.iterator();
while (it.hasNext()) {
Student s = it.next();
System.out.println(s.getName() + "," + s.getAge());
}
}
}
运行结果:
扫描二维码关注公众号,回复:
15548469 查看本文章
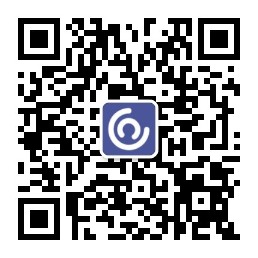