目录
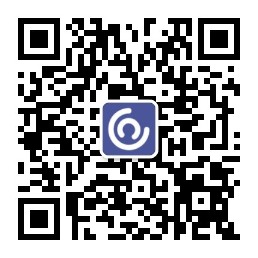
1. Qt模板库
1.1. Qstring字符串类
重载了“+”“+=”
QString str;
bool ok;
str="welcome";str+="to you";
str.append("welcome").append("to you");
str.sprintf("%s %s","welcome","to you");//str==welcome to you
str=QString("%1,hello %2").arg("zylg").arg("good morning");//str==zylg,hello good morning
str="zg";str.insert(1,"yl");//str==zylg
str.replace(0,4,"hello");//zylg -> hello
str=" hello world";str.trimmed();//去掉两端的空白字符
str.simplified();//去掉空白,单个空白代替
str.startsWith("zy",Qt::CaseInsensitive);str.endsWith("zy",Qt::CaseInsensitive);str.contains("zy",Qt::CaseInsensitive);//return bool
//><===compare字符串的比较
//字符串的转换
str="125"; long hex=str.toInt(&ok,16);//ok=true,hex=293
str.isNull();str.isEmpty();
str.fill('A', 2);
//切割字符串
QString csv = "forename,middlename,surname,phone";
QString path = "/usr/local/bin/myapp"; // First field is empty
QString::SectionFlag flag = QString::SectionSkipEmpty;
str = csv.section(',', 2, 2); // str == "surname"
str = path.section('/', 3, 4); // str == "bin/myapp"
str = path.section('/', 3, 3, flag); // str == "myapp"
/*else
* QString & QString::replace ( int position, int n, const QString & after )
* QString & QString::remove ( int position, int n )
* QString QString::mid ( int position, int n = -1 ) const
* /
ui->label2->setText(str.setNum(hex));
1.2. 容器类
QList:顺序存储
#include <QCoreApplication>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QList<QString> list;
list<<"aa"<<"bb"<<"cc"; // 插入数据
list.replace(2,"dd"); // 替换
list.append("ee"); // 末尾添加
list.prepend("dao"); // 表头添加
// 输出整个列表
for(int i=0;i<list.size();i++){
qDebug()<<list.at(i);
}
QString str=list.takeAt(2);// 删除,并获取到它
qDebug()<<"at(2) item is: "<<str;
list.insert(2,"dao");
list.swap(1,3); // 交换
qDebug()<<"now the list is:";
// 输出整个列表
for(int i=0;i<list.size();i++){
qDebug()<<list.at(i);
}
qDebug()<<"Containes dao?"<<list.contains("dao");// 是否包含dao
qDebug()<<"the dao count:"<<list.count("dao"); // 个数
qDebug()<<"the first dao index:"<<list.indexOf("dao");
qDebug()<<"the second dao index:"<<list.indexOf("dao",1); // 指定位置1开始
return a.exec();
}
1.3. QLinkList:链式存储
QVector:可以使用下标访问数据项
迭代器:(读写,读两种)
QList<QString> list;
list<<"你好"<<"我叫"<<"大家好";
QListIterator<QString> itera(list);//java风格读迭代器
//java风格
QMutableListIterator<QString> itera2(list);//java风格读写迭代器,,
/*<>::toBack()将迭代器移动到最后一个列表项后面
*<>::previous()移动到前一个
*<>::hasPrevious()检查前面是否还有元素等
* list的迭代器还有toFont(),findNext(),findprevious()
*/
for(itera2.toFront();itera2.hasNext();)
{
qDebug()<<itera2.next();
}
//STL风格
QList<QString>::Iterator itera1;//读写迭代器
for(int i=1;i<10;i++)
{
list.insert(list.end(),QString::number(i));
}
for(itera1=list.begin();itera1!=list.end();itera1++)
{
qDebug()<<*itera1;
}
1.4. 联合数据类型variant:
//QVariant联合体数据类型,.typename可得到类型
QVariant v(709);
QVariant w("zylg");
QMap<QString,QVariant> map;
map["int"]=520;
map["string"]="zylg";
map["color"]=QColor(255,0,0);
qDebug()<<v.toInt()<<w.toString();
qDebug()<<map["string"].toString()<<map["color"].value<QColor>();
1.5. 算法和正则表达式
qAbs() qMax() qRound() qSwap()
E?匹配0次或者多次
E+匹配1次或者多次
E*匹配0次或者多次
E[n]
E[n,m]匹配n次到m次
^字符串开头
$结尾
\b单词边界
\B非单词边界
1.6. 控件
Buttons:push batton 按钮
Tool button 工具按钮
Radio button 单选按钮
Check box 复选框
Command link button 命令链接按钮
Button box 按钮盒
Input widgets:
Combo box 组合框
Font combo box 字体组合框
Line edit 行编辑
Text edit 文本编辑
Plain text edit 纯文本编辑
Spin box 数字显示盒
Double spin box 双数字显示盒
Time edit 时间编辑
Date/time edit 时间/日期编辑
Dial 拨号
Horizontal scroll bar 横向滚动条
Vertical scroll bar 纵向滚动条
Key sequence edit 按键排序编辑
QDateTime类对应于Date/Time Edit组件,用来获取系统时间
QDateTime *dt=new QDateTime(QDateTime::currentDateTime());
qDebug()<<dt->date().toString();
qDebug()<<dt->time().toString();
QTimer定时器
QTimer *timer = new QTimer( myObject );
connect( timer, SIGNAL(timeout()), myObject, SLOT(timerDone()) );
timer->start( 2000, TRUE ); // 2秒单触发定时器
QTimer *t = new QTimer( myObject );
connect( t, SIGNAL(timeout()), SLOT(processOneThing()) );
t->start( 0, FALSE );
Display widgets:
Label标签
Text browser文本浏览器
Graphics view图形视图
Calendar日历
LCD number液晶数字
Progress bar进度条
Horizontal line水平线
Vertical line垂直线
Open GL widgets开放式图形库工具
QQuick widget嵌入QML工具
Qweb viewwen视图
QTextbrowser继承Qtextedit,仅仅是只读的,但是具有链接的作用,提供的曹有翻页
空间间隔组:horizontal spacer
vertical spacer
布局管理器:vertical layout
horizontal layout
grid
form
容器组:group box组框
scroll area滚动区域
tool box工具箱
tab widget标签小部件
stack widget堆叠部件
frame帧
widget标签小工具
mdiareaMDI区域
dock widget停靠窗体部件
qaxwidget封装flash的active部件
Qwidget是qt图形界面的基本生成块
项目视图组:
List view
Tree view
Table view
Column view
Qlabelview可以实现setmodel使用模型,可以和qsqltabelmodel绑定,但是不能和qtabelwidget一样可以使用复选框,这就是区别,视图和模型绑定时模型以new的形式建立
Example:
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent) :
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
init();
connect(ui->treeWidget,SIGNAL(itemChanged(QTreeWidgetItem*, int)),
this, SLOT(treeItemChanged(QTreeWidgetItem*, int)));
}
Widget::~Widget()
{
delete ui;
}
void Widget::init()
{
ui->treeWidget->clear();
//第一个分组
QTreeWidgetItem *group1 = new QTreeWidgetItem(ui->treeWidget);
group1->setText(0, "group1");
group1->setFlags(Qt::ItemIsUserCheckable|Qt::ItemIsEnabled|Qt::ItemIsSelectable);
group1->setCheckState(0, Qt::Unchecked);
QTreeWidgetItem *subItem11 = new QTreeWidgetItem(group1);
subItem11->setFlags(Qt::ItemIsUserCheckable|Qt::ItemIsEnabled|Qt:: ItemIsSelectable);
subItem11->setText(0, "subItem11");
subItem11->setCheckState(0, Qt::Unchecked);
QTreeWidgetItem *subItem12 = new QTreeWidgetItem(group1);
subItem12->setFlags(Qt::ItemIsUserCheckable|Qt::ItemIsEnabled|Qt:: ItemIsSelectable);
subItem12->setText(0, "subItem12");
subItem12->setCheckState(0, Qt::Unchecked);
}
void Widget::treeItemChanged(QTreeWidgetItem* item, int column)
{
QString itemText = item->text(0);
//选中时
if (Qt::Checked == item->checkState(0))
{
QTreeWidgetItem* parent = item->parent();
int count = item->childCount();
if (count > 0)
{
for (int i = 0; i < count; i++)
{
//子节点也选中
item->child(i)->setCheckState(0, Qt::Checked);
}
}
else
{
//是子节点
updateParentItem(item);
}
}
else if (Qt::Unchecked == item->checkState(0))
{
int count = item->childCount();
if (count > 0)
{
for (int i = 0; i < count; i++)
{
item->child(i)->setCheckState(0, Qt::Unchecked);
}
}
else
{
updateParentItem(item);
}
}
}
void Widget::updateParentItem(QTreeWidgetItem* item)
{
QTreeWidgetItem *parent = item->parent();
if (parent == NULL)
{
return;
}
//选中的子节点个数
int selectedCount = 0;
int childCount = parent->childCount();
for (int i = 0; i < childCount; i++)
{
QTreeWidgetItem *childItem = parent->child(i);
if (childItem->checkState(0) == Qt::Checked)
{
selectedCount++;
}
}
if (selectedCount <= 0)
{
//选中状态
parent->setCheckState(0, Qt::Unchecked);
}
else if (selectedCount > 0 && selectedCount < childCount)
{
//部分选中状态
parent->setCheckState(0, Qt::PartiallyChecked);
}
else if (selectedCount == childCount)
{
//未选中状态
parent->setCheckState(0, Qt::Checked);
}
}
1.7. 管理布局
1.splitter类,分割窗体,里面参数控制widget的排列,x轴还是y轴,在window头文件
QFont font("ZYSong18030",12);//set font
a.setFont(font);
//主窗口的分割
QSplitter *splitmain=new QSplitter(Qt::Horizontal,0);
QTextEdit *textLeft=new QTextEdit(QObject::tr("Left Widget"),splitmain);
textLeft->setAlignment(Qt::AlignCenter);// font align
//右部分窗口的分割
QSplitter *splitRight=new QSplitter(Qt::Vertical,splitmain);
splitRight->setOpaqueResize(false);
QTextEdit *textUp=new QTextEdit(QObject::tr("top widget"),splitRight);
textUp->setAlignment(Qt::AlignRight);
QTextEdit *textBottom =new QTextEdit(QObject::tr("Bottom Widget"),splitRight);
splitmain->setWindowTitle(QObject::tr("专业路过"));
splitmain->show();
停靠窗口:QDockWidget类,在window的头文件
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
setWindowTitle("Dockwidget的使用");
QTextEdit *textMain=new QTextEdit(QObject::tr("Main window"),this);
textMain->setAlignment(Qt::AlignCenter);
setCentralWidget(textMain);//to set become the center body of mainwindow
//dock 1
QDockWidget *dock1=new QDockWidget(tr("dockwindow1"),this);
dock1->setFeatures(QDockWidget::DockWidgetMovable);//can move
/*
* can select:
* DockwidgetClosable can close
* DockwidgetMovable can move
* DockwidgetFloatable can float
* AllDockWidgetFeatures can all
* NoDockWidgetFeatrues can`t all
*/
dock1->setAllowedAreas(Qt::LeftDockWidgetArea|Qt::RightDockWidgetArea);
/*
* LeftDockWidgetArea can stop in mainwindow left
* RightDockWidgetArea can stop in mainwindow right
* TopDockWidgetArea can stop in mainwindown top
* Buttom...
* All...
* no...
*/
addDockWidget(Qt::RightDockWidgetArea,dock1);
}
QStackedWidget类,堆栈窗体(一个窗体可放很多个dialog),在Dialog头文件时
list和stack在同一个主窗口
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QDialog>
#include<QListWidget>
#include<QStackedWidget>
#include<QLabel>
class MainWindow : public QDialog
{
Q_OBJECT
public:
MainWindow(QWidget *parent = 0);
~MainWindow();
private:
QListWidget *list;
QStackedWidget *stack;
QLabel *label1,*label2,*label3;
};
#endif // MAINWINDOW_H
#include "mainwindow.h"
#include<QHBoxLayout>
MainWindow::MainWindow(QWidget *parent)
: QDialog(parent)
{
setWindowTitle(tr("Stackwidget"));
list=new QListWidget(this);
list->insertItem(0,tr("window1"));
list->insertItem(1,tr("window2"));
list->insertItem(2,tr("window3"));
label1=new QLabel(tr("windowstest1"));
label2=new QLabel(tr("windowstest2"));
label3=new QLabel(tr("windowstest3"));
stack=new QStackedWidget(this);
stack->addWidget(label1);
stack->addWidget(label2);
stack->addWidget(label3);
QHBoxLayout *mainLayout=new QHBoxLayout(this);
mainLayout->setMargin(5);
mainLayout->setSpacing(5);
mainLayout->addWidget(list);
mainLayout->addWidget(stack,0,Qt::AlignCenter);
mainLayout->setStretchFactor(list,1);
mainLayout->setStretchFactor(stack,3);//valary >0即可
connect(list,SIGNAL(currentRowChanged(int)),stack,SLOT(setCurrentIndex(int)));
}
MainWindow::~MainWindow()
{
}
基本布局
#ifndef DIALOG_H
#define DIALOG_H
#include <QDialog>
#include<QLabel>
#include<QTextEdit>
#include<QLineEdit>
#include<QComboBox>
#include<QGridLayout>
class Dialog : public QDialog
{
Q_OBJECT
public:
Dialog(QWidget *parent = 0);
~Dialog();
//左侧
QLabel *UserNameLabel;
QLabel *NameLabel;
QLabel *SexLabel;
QLabel *DepartmentLabel;
QLabel *AgeLabel;
QLabel *OtherLabel;
QLineEdit *UserNameLineEdit;
QLineEdit *NameLineEdit;
QComboBox *SexComboBox;
QTextEdit *DepartmentTextEdit;
QLineEdit *AgeLineEdit;
QGridLayout *LeftLayout;
//右侧
QLabel *HeadLabel; //右上角部分
QLabel *HeadIconLabel;
QPushButton *UpdateHeadBtn;
QHBoxLayout *TopRightLayout;
QLabel *IntroductionLabel;
QTextEdit *IntroductionTextEdit;
QVBoxLayout *RightLayout;
//底部
QPushButton *OkBtn;
QPushButton *CancelBtn;
QHBoxLayout *ButtomLayout;
};
#endif // DIALOG_H
#include "dialog.h"
#include<QLabel>
#include<QLineEdit>
#include<QComboBox>
#include<QPushButton>
#include<QFrame>
#include<QGridLayout>
#include<QPixmap>
#include<QHBoxLayout>
Dialog::Dialog(QWidget *parent)
: QDialog(parent)
{
setWindowTitle(tr("UserInfo"));
/************** 左侧 ******************************/
UserNameLabel =new QLabel(tr("用户名:"));
UserNameLineEdit =new QLineEdit;
NameLabel =new QLabel(tr("姓名:"));
NameLineEdit =new QLineEdit;
SexLabel =new QLabel(tr("性别:"));
SexComboBox =new QComboBox;
SexComboBox->addItem(tr("女"));
SexComboBox->addItem(tr("男"));
DepartmentLabel =new QLabel(tr("部门:"));
DepartmentTextEdit =new QTextEdit;
AgeLabel =new QLabel(tr("年龄:"));
AgeLineEdit =new QLineEdit;
OtherLabel =new QLabel(tr("备注:"));
OtherLabel->setFrameStyle(QFrame::Panel|QFrame::Sunken);
/*
* 设置标签的风格,由形状和阴影组成,形状={noFrame,panel,box,HLine,VLine,WinPanel}
* 阴影={plain,raised,Sunken}
*/
LeftLayout =new QGridLayout();
LeftLayout->addWidget(UserNameLabel,0,0); //用户名
LeftLayout->addWidget(UserNameLineEdit,0,1);
LeftLayout->addWidget(NameLabel,1,0); //姓名
LeftLayout->addWidget(NameLineEdit,1,1);
LeftLayout->addWidget(SexLabel,2,0); //性别
LeftLayout->addWidget(SexComboBox,2,1);
LeftLayout->addWidget(DepartmentLabel,3,0); //部门
LeftLayout->addWidget(DepartmentTextEdit,3,1);
LeftLayout->addWidget(AgeLabel,4,0); //年龄
LeftLayout->addWidget(AgeLineEdit,4,1);
LeftLayout->addWidget(OtherLabel,5,0,1,2); //其他
LeftLayout->setColumnStretch(0,1);
LeftLayout->setColumnStretch(1,8);//设置行之间的比例
/*********右侧*********/
HeadLabel =new QLabel(tr("头像: ")); //右上角部分
HeadIconLabel =new QLabel;
QPixmap icon("312.png");
HeadIconLabel->setPixmap(icon);
HeadIconLabel->resize(icon.width(),icon.height());
UpdateHeadBtn =new QPushButton(tr("更新"));
TopRightLayout =new QHBoxLayout();
TopRightLayout->setSpacing(20);
TopRightLayout->addWidget(HeadLabel);
TopRightLayout->addWidget(HeadIconLabel);
TopRightLayout->addWidget(UpdateHeadBtn);
IntroductionLabel =new QLabel(tr("个人说明:")); //右下角部分
IntroductionTextEdit =new QTextEdit;
RightLayout =new QVBoxLayout();
RightLayout->setMargin(10);
RightLayout->addLayout(TopRightLayout);
RightLayout->addWidget(IntroductionLabel);
RightLayout->addWidget(IntroductionTextEdit);
/*--------------------- 底部 --------------------*/
OkBtn =new QPushButton(tr("确定"));
CancelBtn =new QPushButton(tr("取消"));
ButtomLayout =new QHBoxLayout();
ButtomLayout->addStretch();
ButtomLayout->addWidget(OkBtn);
ButtomLayout->addWidget(CancelBtn);
/*---------------------------------------------*/
QGridLayout *mainLayout =new QGridLayout(this);
mainLayout->setMargin(15);
mainLayout->setSpacing(10);
mainLayout->addLayout(LeftLayout,0,0);
mainLayout->addLayout(RightLayout,0,1);
mainLayout->addLayout(ButtomLayout,1,0,1,2);
mainLayout->setSizeConstraint(QLayout::SetFixedSize);
}
Dialog::~Dialog()
{
}
综合小例子:
程序说明:用baseinfo,contact,detai建立三个界面,然后用content去建立了stack,再在主窗口main.cpp里面加入list,处理了list 和stack即可
//baseinfo.h
#ifndef BASEINFO_H
#define BASEINFO_H
#include<QLabel>
#include<QLineEdit>
#include<QComboBox>
#include<QTextEdit>
#include<QGridLayout>
#include<QPushButton>
class BaseInfo: public QWidget
{
Q_OBJECT
public:
BaseInfo(QWidget *parent=0);
private:
//左侧//
QLabel *UserNameLabel;
QLabel *NameLabel;
QLabel *SexLabel;
QLabel *DepartmentLabel;
QLabel *AgeLabel;
QLabel *OtherLabel;
QLineEdit *UserNameLineEdit;
QLineEdit *NameLineEdit;
QComboBox *SexComboBox;
QTextEdit *DepartmentTextEdit;
QLineEdit *AgeLineEdit;
QGridLayout *LeftLayout;
//右侧//
QLabel *HeadLabel; //右上角部分
QLabel *HeadIconLabel;
QPushButton *UpdateHeadBtn;
QHBoxLayout *TopRightLayout;
QLabel *IntroductionLabel;
QTextEdit *IntroductionTextEdit;
QVBoxLayout *RightLayout;
};
#endif // BASEINFO_H
//baseinfo.cpp
#include "baseinfo.h"
BaseInfo::BaseInfo(QWidget *parent) :
QWidget(parent)
{
/**** 左侧 ****/
UserNameLabel =new QLabel(tr("用户名:"));
UserNameLineEdit =new QLineEdit;
NameLabel =new QLabel(tr("姓名:"));
NameLineEdit =new QLineEdit;
SexLabel =new QLabel(tr("性别:"));
SexComboBox =new QComboBox;
SexComboBox->addItem(tr("女"));
SexComboBox->addItem(tr("男"));
DepartmentLabel =new QLabel(tr("部门:"));
DepartmentTextEdit =new QTextEdit;
AgeLabel =new QLabel(tr("年龄:"));
AgeLineEdit =new QLineEdit;
OtherLabel =new QLabel(tr("备注:"));
OtherLabel->setFrameStyle(QFrame::Panel|QFrame::Sunken);
LeftLayout =new QGridLayout();
LeftLayout->addWidget(UserNameLabel,0,0);
LeftLayout->addWidget(UserNameLineEdit,0,1);
LeftLayout->addWidget(NameLabel,1,0);
LeftLayout->addWidget(NameLineEdit,1,1);
LeftLayout->addWidget(SexLabel,2,0);
LeftLayout->addWidget(SexComboBox,2,1);
LeftLayout->addWidget(DepartmentLabel,3,0);
LeftLayout->addWidget(DepartmentTextEdit,3,1);
LeftLayout->addWidget(AgeLabel,4,0);
LeftLayout->addWidget(AgeLineEdit,4,1);
LeftLayout->addWidget(OtherLabel,5,0,1,2);
LeftLayout->setColumnStretch(0,1);
LeftLayout->setColumnStretch(1,3);
/****右侧****/
HeadLabel =new QLabel(tr("头像: ")); //右上角部分
HeadIconLabel =new QLabel;
QPixmap icon("312.png");
HeadIconLabel->setPixmap(icon);
HeadIconLabel->resize(icon.width(),icon.height());
UpdateHeadBtn =new QPushButton(tr("更新"));
TopRightLayout =new QHBoxLayout();
TopRightLayout->setSpacing(20);
TopRightLayout->addWidget(HeadLabel);
TopRightLayout->addWidget(HeadIconLabel);
TopRightLayout->addWidget(UpdateHeadBtn);
IntroductionLabel =new QLabel(tr("个人说明:"));//右下角部分
IntroductionTextEdit =new QTextEdit;
RightLayout =new QVBoxLayout();
RightLayout->setMargin(10);
RightLayout->addLayout(TopRightLayout);
RightLayout->addWidget(IntroductionLabel);
RightLayout->addWidget(IntroductionTextEdit);
/*************************************/
QGridLayout *mainLayout =new QGridLayout(this);
mainLayout->setMargin(15);
mainLayout->setSpacing(10);
mainLayout->addLayout(LeftLayout,0,0);
mainLayout->addLayout(RightLayout,0,1);
mainLayout->setSizeConstraint(QLayout::SetFixedSize);
}
//contact.h
#ifndef CONTACT_H
#define CONTACT_H
#include <QLabel>
#include <QGridLayout>
#include <QLineEdit>
#include <QCheckBox>
class Contact : public QWidget
{
Q_OBJECT
public:
Contact(QWidget *parent=0);
private:
QLabel *EmailLabel;
QLineEdit *EmailLineEdit;
QLabel *AddrLabel;
QLineEdit *AddrLineEdit;
QLabel *CodeLabel;
QLineEdit *CodeLineEdit;
QLabel *MoviTelLabel;
QLineEdit *MoviTelLineEdit;
QCheckBox *MoviTelCheckBook;
QLabel *ProTelLabel;
QLineEdit *ProTelLineEdit;
QGridLayout *mainLayout;
};
#endif
//contact.cpp
#include "contact.h"
Contact::Contact(QWidget *parent) :
QWidget(parent)
{
EmailLabel =new QLabel(tr("电子邮件:"));
EmailLineEdit =new QLineEdit;
AddrLabel =new QLabel(tr("联系地址:"));
AddrLineEdit =new QLineEdit;
CodeLabel =new QLabel(tr("邮政编码:"));
CodeLineEdit =new QLineEdit;
MoviTelLabel =new QLabel(tr("移动电话:"));
MoviTelLineEdit =new QLineEdit;
MoviTelCheckBook =new QCheckBox(tr("接收留言"));
ProTelLabel =new QLabel(tr("办公电话:"));
ProTelLineEdit =new QLineEdit;
mainLayout =new QGridLayout(this);
mainLayout->setMargin(15);
mainLayout->setSpacing(10);
mainLayout->addWidget(EmailLabel,0,0);
mainLayout->addWidget(EmailLineEdit,0,1);
mainLayout->addWidget(AddrLabel,1,0);
mainLayout->addWidget(AddrLineEdit,1,1);
mainLayout->addWidget(CodeLabel,2,0);
mainLayout->addWidget(CodeLineEdit,2,1);
mainLayout->addWidget(MoviTelLabel,3,0);
mainLayout->addWidget(MoviTelLineEdit,3,1);
mainLayout->addWidget(MoviTelCheckBook,3,2);
mainLayout->addWidget(ProTelLabel,4,0);
mainLayout->addWidget(ProTelLineEdit,4,1);
mainLayout->setSizeConstraint(QLayout::SetFixedSize);
}
//detail.h
#ifndef DETAIL_H
#define DETAIL_H
#include <QLabel>
#include <QComboBox>
#include <QLineEdit>
#include <QTextEdit>
#include <QGridLayout>
class Detail : public QWidget
{
Q_OBJECT
public:
Detail(QWidget *parent=0);
private:
QLabel *NationalLabel;
QComboBox *NationalComboBox;
QLabel *ProvinceLabel;
QComboBox *ProvinceComboBox;
QLabel *CityLabel;
QLineEdit *CityLineEdit;
QLabel *IntroductLabel;
QTextEdit *IntroductTextEdit;
QGridLayout *mainLayout;
};
#endif // DETAIL_H
//detail.cpp
#include "detail.h"
Detail::Detail(QWidget *parent) :
QWidget(parent)
{
NationalLabel =new QLabel(tr("国家/地址:"));
NationalComboBox =new QComboBox;
NationalComboBox->insertItem(0,tr("中国"));
NationalComboBox->insertItem(1,tr("美国"));
NationalComboBox->insertItem(2,tr("英国"));
ProvinceLabel =new QLabel(tr("省份:"));
ProvinceComboBox =new QComboBox;
ProvinceComboBox->insertItem(0,tr("江苏省"));
ProvinceComboBox->insertItem(1,tr("山东省"));
ProvinceComboBox->insertItem(2,tr("浙江省"));
CityLabel =new QLabel(tr("城市:"));
CityLineEdit =new QLineEdit;
IntroductLabel =new QLabel(tr("个人说明:"));
IntroductTextEdit =new QTextEdit;
mainLayout =new QGridLayout(this);
mainLayout->setMargin(15);
mainLayout->setSpacing(10);
mainLayout->addWidget(NationalLabel,0,0);
mainLayout->addWidget(NationalComboBox,0,1);
mainLayout->addWidget(ProvinceLabel,1,0);
mainLayout->addWidget(ProvinceComboBox,1,1);
mainLayout->addWidget(CityLabel,2,0);
mainLayout->addWidget(CityLineEdit,2,1);
mainLayout->addWidget(IntroductLabel,3,0);
mainLayout->addWidget(IntroductTextEdit,3,1);
}
//content.h
#ifndef CONTENT_H
#define CONTENT_H
#include <QStackedWidget>
#include<QPushButton>
#include "baseinfo.h"
#include "contact.h"
#include "detail.h"
class Content : public QFrame
{
Q_OBJECT
public:
Content(QWidget *parent = 0);
QStackedWidget *stack;
QPushButton *AmendBtn;
QPushButton *CloseBtn;
BaseInfo *baseinfo;
Contact *contact;
Detail *detail;
~Content();
};
#endif // CONTENT_H
//content.cpp
#include "content.h"
Content::Content(QWidget *parent)
:QFrame(parent)
{
stack =new QStackedWidget(this);
stack->setFrameStyle(QFrame::Panel|QFrame::Raised);
baseinfo =new BaseInfo();
contact =new Contact();
detail =new Detail();
stack->addWidget(baseinfo);
stack->addWidget(contact);
stack->addWidget(detail);
AmendBtn =new QPushButton(tr("修改"));
CloseBtn =new QPushButton(tr("关闭"));
QHBoxLayout *BtnLayout =new QHBoxLayout;
BtnLayout->addStretch(1);
BtnLayout->addWidget(AmendBtn);
BtnLayout->addWidget(CloseBtn);
QVBoxLayout *RightLayout =new QVBoxLayout(this);
RightLayout->setMargin(10);
RightLayout->setSpacing(6);
RightLayout->addWidget(stack);
RightLayout->addLayout(BtnLayout);
}
Content::~Content()
{}
//main.cpp
#include "content.h"
#include <QApplication>
#include<QTextCodec>
#include<QSplitter>
#include<QListWidget>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QSplitter *splitterMain=new QSplitter(Qt::Horizontal,0);
splitterMain->setOpaqueResize(true);
QListWidget *list=new QListWidget(splitterMain);
list->insertItem(0,QObject::tr("基本信息"));
list->insertItem(1,QObject::tr("联系方式"));
list->insertItem(2,QObject::tr("详细资料"));
Content *content=new Content(splitterMain);
QObject::connect(list,SIGNAL(currentRowChanged(int)),content->stack,SLOT(setCurrentIndex(int)));
splitterMain->setWindowTitle(QObject::tr("修改用户资料"));
splitterMain->setMinimumSize(splitterMain->minimumSize());
splitterMain->setMaximumSize(splitterMain->maximumSize());
splitterMain->show();
return a.exec();
}
1.8. 基本对话框
文件,颜色,标准字体,标准输入,标准消息,还可以自定义对话框
文件:
QFileDialog::getExistingDirectory(NULL,"caption",".");获取文件夹名
QString s=QFileDialog::getOpenFileName(this,"标题","C://PerfLogs","(*.cpp *.c *.h);;(*.jpg)");
file_name = QFileDialog::getOpenFileNames(NULL,"标题",".","*.*");
fd.setViewMode(QFileDialog::Detail);//详细 fd.setViewMode(QFileDialog::List);//仅列表
设置历史目录setHistory
颜色:
#include "dialog.h"
Dialog::Dialog(QWidget *parent)
: QDialog(parent)
{
button=new QPushButton(tr("颜色选择"));
colorframe=new QFrame();
colorframe->setFrameShape(QFrame::Box);
colorframe->setAutoFillBackground(true);
layout=new QVBoxLayout(this);
layout->addWidget(button);
layout->addWidget(colorframe);
connect(button,SIGNAL(clicked()),this,SLOT(showcolor()));
}
void Dialog::showcolor()
{
QColor c=QColorDialog::getColor(Qt::blue);
if(c.isValid())colorframe->setPalette(c);
}
Dialog::~Dialog()
{
}
字体:有string list int double输入的窗口
#include "dialog.h"
Dialog::Dialog(QWidget *parent)
: QDialog(parent)
{
button=new QPushButton(tr("字体选择"));
textedit=new QTextEdit(tr("welcome you"));
layout=new QVBoxLayout(this);
layout->addWidget(button);
layout->addWidget(textedit);
connect(button,SIGNAL(clicked()),this,SLOT(showcolor()));
}
void Dialog::showcolor()
{
bool ok;
QFont f=QFontDialog::getFont(&ok);
if(ok)textedit->setFont(f);
}
Dialog::~Dialog()
{
}
输入框:
void Dialog::showcolor()
{
bool ok;
input=new QInputDialog(this);
input->show();
}
void Dialog::showcolor()
{
bool ok;
QString text=QInputDialog::getText(this,tr("标准字符串输入框"),tr("请输入姓名"),QLineEdit::Normal,button->text(),&ok);
textedit->setText(text);
}
void Dialog::showcolor()
{
bool ok;
QStringList str;
str<<tr("男")<<tr("女");
QString text=QInputDialog::getItem(this,tr("选择窗口"),tr("请选择"),str,0,false,&ok);
textedit->setText(text);
}
void Dialog::showcolor()
{
bool ok;
//初始数字0,0-100,step=0
int text=QInputDialog::getInt(this,tr("选择数字"),tr("请选择1-100"),0,0,100,1,&ok);
textedit->setText(QString("%1").arg(text));
}
Double型:
int text=QInputDialog::getDouble(this,tr("选择数字"),tr("请选择1-100"),0,0,100,1,&ok);
消息对话框:Question询问,information信息,warning警告,critica严重警告,about,custon自定义
void Dialog::showcolor()
{
button->setText("question messege box");
switch (QMessageBox::question(this,tr("询问消息框"),tr("程序象征性的问你一下"),QMessageBox::Ok|QMessageBox::Cancel
,QMessageBox::Ok))
{
case QMessageBox::Ok:textedit->setText("OK");
break;
default:
textedit->setText("CANAL");
break;
}
}
void Dialog::showcolor()
{
button->setText("information messege box");
QMessageBox::information(this,tr("信息提示"),tr("马上下雨了,快回家收衣服"));
}
void Dialog::showcolor()
{
button->setText("warning tion messege box");
QMessageBox::warning(this,tr("信息提示"),tr("马上下雨了,快回家收衣服"),QMessageBox::Save|QMessageBox::Discard|
QMessageBox::Cancel,QMessageBox::Save);
}
void Dialog::showcolor()
{
button->setText("critical messege box");
QMessageBox::critical(this,tr("信息提示"),tr("马上下雨了,快回家收衣服"));
}
void Dialog::showcolor()
{
button->setText("About messege box");
QMessageBox::about(this,tr("信息提示"),tr("马上下雨了,快回家收衣服"));
}
void Dialog::showcolor()
{
button->setText("自定义消息框");
QMessageBox custon;
custon.setWindowTitle("专业路过");
QPushButton *yesbt=custon.addButton(tr("确定"),QMessageBox::ActionRole);
QPushButton *nobt=custon.addButton(tr("取消"),QMessageBox::ActionRole);
QPushButton *canelbt=custon.addButton(tr("放弃"),QMessageBox::ActionRole);
// custon.setIconPixmap("*.jpg");
custon.exec();//开始显示窗口
if(custon.clickedButton()==yesbt)textedit->setText("我的名字叫做专业路过");
}
伸缩框
#include "drawer.h"
Drawer::Drawer(QWidget *parent,Qt::WindowFlags f)
{
setWindowTitle("My QQ");
toolBtn1_1 =new QToolButton;
toolBtn1_1->setText(tr("张三"));
toolBtn1_1->setIcon(QPixmap("G:/qt/MyQQ\\picture\\1.jpg"));
toolBtn1_1->setIconSize(QSize(70,100));
toolBtn1_1->setAutoRaise(true);//当鼠标离开时,按钮自动恢复弹起状态
toolBtn1_1->setToolButtonStyle(Qt::ToolButtonTextBesideIcon);
/*
* 设置按钮的属性
* Qt::ToolButtonIconOnly只显示图标
* Qt::ToolButtonTextOnly只显示文字
* Qt::ToolButtonTextBesideIcon文字显示在图片旁边
* Qt::ToolButtonTextUnderIcon文字显示在图片下面
* Qt::ToolButtonFollowStyle遵循style原则
*/
...
QGroupBox *groupBox1=new QGroupBox;
QVBoxLayout *layout1=new QVBoxLayout(groupBox1); //(groupBox1);
layout1->setMargin(10); //布局中各窗体的显示间距
layout1->setAlignment(Qt::AlignHCenter); //布局中各窗体的显示位置
layout1->addWidget(toolBtn1_1);
layout1->addWidget(toolBtn1_2);
layout1->addWidget(toolBtn1_3);
layout1->addWidget(toolBtn1_4);
layout1->addWidget(toolBtn1_5);
layout1->addStretch(); //插入一个占位符
...
this->addItem((QWidget*)groupBox1,tr("我的好友"));
this->addItem((QWidget*)groupBox2,tr("陌生人"));
this->addItem((QWidget*)groupBox3,tr("黑名单"));
this->resize(230,500);
}
进度条:
1.progressBar->setRange(0,num);
for(int i=1;i<num+1;i++)
{
progressBar->setValue(i);
}
2.QProgressDialog *progressDialog=new QProgressDialog(this);
QFont font("ZYSong18030",12);
progressDialog->setFont(font);
progressDialog->setWindowModality(Qt::WindowModal);//模态方式
progressDialog->setMinimumDuration(5);//等待时间
progressDialog->setWindowTitle(tr("Please Wait"));
progressDialog->setLabelText(tr("Copying..."));
progressDialog->setCancelButtonText(tr("Cancel"));
progressDialog->setRange(0,num);
for(int i=1;i<num+1;i++)
{
progressDialog->setValue(i);
if(progressDialog->wasCanceled())//检测取消按键是否激发
return;
}
调色板和电子钟palette;
void palette::fillColorList(QComboBox *comboBox)
{
QStringList colorList = QColor::colorNames();
QString color;
foreach(color,colorList)
{
QPixmap pix(QSize(70,20));
pix.fill(QColor(color));
comboBox->addItem(QIcon(pix),NULL);
comboBox->setIconSize(QSize(70,20));
comboBox->setSizeAdjustPolicy(QComboBox::AdjustToContents);//下拉框为符合内容的大小
}
}
void palette::ShowBase()
{
QStringList colorList = QColor::colorNames();
QColor color = QColor(colorList[baseComboBox->currentIndex()]);
QPalette p = contentFrame->palette();
p.setColor(QPalette::Base,color);
contentFrame->setAutoFillBackground(true);
contentFrame->setPalette(p);
}
p.setColor(QPalette::Window, color); p.setColor(QPalette::WindowText, color);
p.setColor(QPalette::ButtonText , color);
p.setColor(QPalette::Base , color);
QTime类:
#include "digtime.h"
#include<QMouseEvent>
#include<QDebug>
digtime::digtime(QWidget *parent):QLCDNumber(parent)
{
QPalette p=palette();
p.setColor(QPalette::Window,Qt::blue);//背景蓝色
setPalette(p);
setWindowFlags(Qt::FramelessWindowHint);//无边框
setDigitCount(8);//显示的个数为8
setWindowOpacity(0.5);//透明度0.5
QTimer *timer=new QTimer(this);
connect(timer,SIGNAL(timeout()),this,SLOT(showTime()));//定时器的
timer->start(1000);
showTime();
resize(250,60);
show1=true;
}
void digtime::showTime()
{
QTime time=QTime::currentTime();//get curren time
QString text=QString("%1:%2").arg(time.toString("hh:mm")).arg(time.toString("ss"));
if(show1)
{
text[2]=text[5]=':';
show1=false;
}
else
{
text[2]= text[5]=' ';
show1=true;
}
display(text);
}
void digtime::mousePressEvent(QMouseEvent *m)
{
if(m->button()==Qt::LeftButton)
{
point=m->globalPos()-frameGeometry().topLeft();
m->accept();
}
if(m->button()==Qt::RightButton)
{
close();
}
}
void digtime::mouseMoveEvent(QMouseEvent *m)
{
if(m->buttons()&Qt::LeftButton)//buttons()返回鼠标状态
{
move(m->globalPos()-point);
m->accept();
}
}
可扩展对话框:
QVBoxLayout *layout =new QVBoxLayout(this); //布局
layout->addWidget(baseWidget);
layout->addWidget(detailWidget);
layout->setSizeConstraint(QLayout::SetFixedSize);//固定位置
layout->setSpacing(10);
detailWidget =new QWidget;
detailWidget->hide();
void ExtensionDlg::showDetailInfo()
{
if(detailWidget->isHidden())
detailWidget->show();
else
detailWidget->hide();
}
不规则窗体:
QPixmap pix;
pix.load("16.png",0,Qt::AvoidDither|Qt::ThresholdDither|Qt::ThresholdAlphaDither);
resize(pix.size());
setMask(QBitmap(pix.mask()));//除了pix部分其他均为透明
void ExtensionDlg::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
painter.drawPixmap(0,0,QPixmap("16.png"));
}
程序启动画面:
先放一个窗体出来进行休眠,然后再到另一个窗体。
2. 主窗体
文本编辑器实例
1. 新建打开文件,打印文件(图像或者文本)
2. 图像的缩放旋转滤镜等
3. 字体编辑(字体,字号,粗体,颜色)
4. 排版,撤销,重做
//"打开"动作
openFileAction =new QAction(QIcon("open.png"),tr("打开"),this);
openFileAction->setShortcut(tr("Ctrl+O"));
openFileAction->setStatusTip(tr("打开一个文件"));
connect(openFileAction,SIGNAL(triggered()),this,SLOT(ShowOpenFile()));
void ImgProcessor::ShowOpenFile()
{
fileName =QFileDialog::getOpenFileName(this,"打开");
if(!fileName.isEmpty())
{
if(showWidget->text->document()->isEmpty())
{
loadFile(fileName);
}
else
{
ImgProcessor *newImgProcessor =new ImgProcessor;
newImgProcessor->show();
newImgProcessor->loadFile(fileName);
}
}
}
void ImgProcessor::loadFile(QString filename)
{
printf("file name:%s\n",filename.data());
QFile file(filename);
if(file.open(QIODevice::ReadOnly|QIODevice::Text))
{
QTextStream textStream(&file);
while(!textStream.atEnd())
{
showWidget->text->append(textStream.readLine());
printf("read line\n");
}
printf("end\n");
}
}
//"新建"动作
NewFileAction =new QAction(QIcon("new.png"),tr("新建"),this);
NewFileAction->setShortcut(tr("Ctrl+N"));
NewFileAction->setStatusTip(tr("新建一个文件"));
connect(NewFileAction,SIGNAL(triggered()),this,SLOT(ShowNewFile()));
void ImgProcessor::ShowNewFile()
{
ImgProcessor *newImgProcessor =new ImgProcessor;
newImgProcessor->show();
}
//"退出"动作
exitAction =new QAction(tr("退出"),this);
exitAction->setShortcut(tr("Ctrl+Q"));
exitAction->setStatusTip(tr("退出程序"));
connect(exitAction,SIGNAL(triggered()),this,SLOT(close()));
//"复制"动作
copyAction =new QAction(QIcon("copy.png"),tr("复制"),this);
copyAction->setShortcut(tr("Ctrl+C"));
copyAction->setStatusTip(tr("复制文件"));
connect(copyAction,SIGNAL(triggered()),showWidget->text,SLOT(copy()));
//"剪切"动作
cutAction =new QAction(QIcon("cut.png"),tr("剪切"),this);
cutAction->setShortcut(tr("Ctrl+X"));
cutAction->setStatusTip(tr("剪切文件"));
connect(cutAction,SIGNAL(triggered()),showWidget->text,SLOT(cut()));
//"粘贴"动作
pasteAction =new QAction(QIcon("paste.png"),tr("粘贴"),this);
pasteAction->setShortcut(tr("Ctrl+V"));
pasteAction->setStatusTip(tr("粘贴文件"));
connect(pasteAction,SIGNAL(triggered()),showWidget->text,SLOT(paste()));
//"打印文本"动作
PrintTextAction =new QAction(QIcon("printText.png"),tr("打印文本"), this);
PrintTextAction->setStatusTip(tr("打印一个文本"));
connect(PrintTextAction,SIGNAL(triggered()),this,SLOT(ShowPrintText()));
void ImgProcessor::ShowPrintText()
{
// QPrinter printer;
// QPrintDialog printDialog(&printer,this);
// if(printDialog.exec())
// {
// QTextDocument *doc =showWidget->text->document();
// doc->print(&printer);
// }
}
//"打印图像"动作
PrintImageAction =new QAction(QIcon("printImage.png"),tr("打印图像"), this);
PrintImageAction->setStatusTip(tr("打印一幅图像"));
connect(PrintImageAction,SIGNAL(triggered()),this,SLOT(ShowPrintImage()));
void ImgProcessor::ShowPrintImage()
{
// QPrinter printer;
// QPrintDialog printDialog(&printer,this);
// if(printDialog.exec())
// {
// QPainter painter(&printer);
// QRect rect =painter.viewport();
// QSize size = img.size();
// size.scale(rect.size(),Qt::KeepAspectRatio);
// painter.setViewport(rect.x(),rect.y(),size.width(),size.height());
// painter.setWindow(img.rect());
// painter.drawImage(0,0,img);
// }
}
//"放大"动作
zoomInAction =new QAction(QIcon("zoomin.png"),tr("放大"),this);
zoomInAction->setStatusTip(tr("放大一张图片"));
connect(zoomInAction,SIGNAL(triggered()),this,SLOT(ShowZoomIn()));
void ImgProcessor::ShowZoomIn()
{
if(img.isNull())
return;
QMatrix martix;
martix.scale(2,2);
img = img.transformed(martix);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//"缩小"动作
zoomOutAction =new QAction(QIcon("zoomout.png"),tr("缩小"),this);
zoomOutAction->setStatusTip(tr("缩小一张图片"));
connect(zoomOutAction,SIGNAL(triggered()),this,SLOT(ShowZoomOut()));
void ImgProcessor::ShowZoomOut()
{
if(img.isNull())
return;
QMatrix matrix;
matrix.scale(0.5,0.5);
img = img.transformed(matrix);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//实现图像旋转的动作(Action)
//旋转90°
rotate90Action =new QAction(QIcon("rotate90.png"),tr("旋转90°"),this);
rotate90Action->setStatusTip(tr("将一幅图旋转90°"));
connect(rotate90Action,SIGNAL(triggered()),this,SLOT(ShowRotate90()));
void ImgProcessor::ShowRotate90()
{
if(img.isNull())
return;
QMatrix matrix;
matrix.rotate(90);
img = img.transformed(matrix);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//旋转180°
rotate180Action =new QAction(QIcon("rotate180.png"),tr("旋转180°"), this);
rotate180Action->setStatusTip(tr("将一幅图旋转180°"));
connect(rotate180Action,SIGNAL(triggered()),this,SLOT(ShowRotate180()));
void ImgProcessor::ShowRotate180()
{
if(img.isNull())
return;
QMatrix matrix;
matrix.rotate(180);
img = img.transformed(matrix);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//旋转270°
rotate270Action =new QAction(QIcon("rotate270.png"),tr("旋转270°"), this);
rotate270Action->setStatusTip(tr("将一幅图旋转270°"));
connect(rotate270Action,SIGNAL(triggered()),this,SLOT(ShowRotate270()));
void ImgProcessor::ShowRotate270()
{
if(img.isNull())
return;
QMatrix matrix;
matrix.rotate(270);
img = img.transformed(matrix);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//实现图像镜像的动作(Action)
//纵向镜像
mirrorVerticalAction =new QAction(tr ("纵向镜像"),this);
mirrorVerticalAction->setStatusTip(tr("对一张图作纵向镜像"));
connect(mirrorVerticalAction,SIGNAL(triggered()),this,SLOT(ShowMirrorVertical()));
void ImgProcessor::ShowMirrorVertical()
{
if(img.isNull())
return;
img=img.mirrored(false,true);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//横向镜像
mirrorHorizontalAction =new QAction(tr("横向镜像"),this);
mirrorHorizontalAction->setStatusTip(tr("对一张图作横向镜像"));
connect(mirrorHorizontalAction,SIGNAL(triggered()),this,SLOT(ShowMirrorHorizontal()));
void ImgProcessor::ShowMirrorHorizontal()
{
if(img.isNull())
return;
img=img.mirrored(true,false);
showWidget->imageLabel->setPixmap(QPixmap::fromImage(img));
}
//排序:左对齐、右对齐、居中和两端对齐
actGrp =new QActionGroup(this);
leftAction =new QAction(QIcon("left.png"),"左对齐",actGrp);
leftAction->setCheckable(true);
rightAction =new QAction(QIcon("right.png"),"右对齐",actGrp);
rightAction->setCheckable(true);
centerAction =new QAction(QIcon("center.png"),"居中",actGrp);
centerAction->setCheckable(true);
justifyAction =new QAction(QIcon("justify.png"),"两端对齐",actGrp);
justifyAction->setCheckable(true);
connect(actGrp,SIGNAL(triggered(QAction*)),this,SLOT(ShowAlignment(QAction*)));
//实现撤销和重做的动作(Action)
//撤销和重做
undoAction =new QAction(QIcon("undo.png"),"撤销",this);
connect(undoAction,SIGNAL(triggered()),showWidget->text,SLOT(undo()));
redoAction =new QAction(QIcon("redo.png"),"重做",this);
connect(redoAction,SIGNAL(triggered()),showWidget->text,SLOT(redo()));
QTextCharFormat fmt;
fmt.setFontPointSize(spinValue.toFloat());
fmt.setFontWeight(boldBtn->isChecked()?QFont::Bold:QFont::Normal);
fmt.setFontItalic(italicBtn->isChecked());
fmt.setFontUnderline(underlineBtn->isChecked());
fmt.setForeground(color);
showWidget->text->mergeCurrentCharFormat(fmt);
3. 图形和图片
位置:
/*
* x() y() pos()获取窗体左上角的位置坐标
* frameGeometry()获取整个窗体左上角顶点,长,宽的值,Geometry()获取的是中央区域左上角的,
* width() height()获取中央区域的长宽的值
* size()获取中央区域的长宽的值
* rect()也是相对于中央区域,相当于Geometry(),但是返回Qrect()的左上角的值为(0,0)
*/
void geometry::updateLabel()
{
QString xStr; //获得x()函数的结果并显示
xValueLabel->setText(xStr.setNum(x()));
QString yStr; //获得y()函数的结果并显示
yValueLabel->setText(yStr.setNum(y()));
QString frameStr; //获得frameGeometry()函数的结果并显示
QString tempStr1,tempStr2,tempStr3,tempStr4;
frameStr = tempStr1.setNum(frameGeometry().x())+","+tempStr2.setNum(frameGeometry().y())+","+tempStr3.setNum(frameGeometry().width())+","+tempStr4.setNum(frameGeometry().height());
FrmValueLabel->setText(frameStr);
QString positionStr; //获得pos()函数的结果并显示
QString tempStr11,tempStr12;
positionStr =tempStr11.setNum(pos().x())+","+tempStr12.setNum(pos().y());
posValueLabel->setText(positionStr);
QString geoStr; //获得geometry()函数的结果并显示
QString tempStr21,tempStr22,tempStr23,tempStr24;
geoStr =tempStr21.setNum(geometry().x())+","+tempStr22.setNum(geometry().y())+","+tempStr23.setNum(geometry().width())+","+tempStr24.setNum(geometry().height());
geoValueLabel->setText(geoStr);
QString wStr,hStr; //获得width()、height()函数的结果并显示
widthValueLabel->setText(wStr.setNum(width()));
heightValueLabel->setText(hStr.setNum(height()));
QString rectStr; //获得rect()函数的结果并显示
QString tempStr31,tempStr32,tempStr33,tempStr34;
rectStr =tempStr31.setNum(rect().x())+","+tempStr32.setNum(rect().y())+","+tempStr33.setNum(/*rect().width()*/width())+","+tempStr34.setNum(height()/*rect().height()*/);
rectValueLabel->setText(rectStr);
QString sizeStr; //获得size()函数的结果并显示
QString tempStr41,tempStr42;
sizeStr =tempStr41.setNum(size().width())+","+tempStr42.setNum(size().height());
sizeValueLabel->setText(sizeStr);
}
(具体代码见https://blog.csdn.net/qq_33564134)
void PaintArea::paintEvent(QPaintEvent *)
{
QPainter p(this);
p.setPen(pen);
p.setBrush(brush);
QRect rect(50,100,300,200);//为矩形区域做准备
static const QPoint points[4]=//为多边点和多边点做准备
{
QPoint(150,100),
QPoint(300,150),
QPoint(350,250),
QPoint(100,300)
};
int startAngle =30*16;
int spanAngle =120*16;
QPainterPath path;
path.addRect(150,150,100,100);
path.moveTo(100,100);
path.cubicTo(300,100,200,200,300,300);
path.cubicTo(100,300,200,200,100,100);
path.setFillRule(fillRule);
switch(shape)
{
case Line: //直线
p.drawLine(rect.topLeft(),rect.bottomRight()); break;
case Rectangle: //长方形
p.drawRect(rect);break;
case RoundRect: //圆角方形
p.drawRoundRect(rect); break;
case Ellipse: //椭圆形
p.drawEllipse(rect); break;
case Polygon: //多边形
p.drawPolygon(points,4); break;
case Polyline: //多边线
p.drawPolyline(points,4); break;
case Points: //点
p.drawPoints(points,4); break;
case Arc: //弧
p.drawArc(rect,startAngle,spanAngle); break;
case Path: //路径
p.drawPath(path); break;
case Text: //文字
p.drawText(rect,Qt::AlignCenter,tr("Hello Qt!"));break;
case Pixmap: //图片
p.drawPixmap(150,150,QPixmap("butterfly.png")); break;
default: break;
}
}
基本绘画:
QPen:The QPen class defines how a QPainter should draw lines and outlines of shapes.
A pen has a style, width, color, cap style and join style
构造函数:QPen ( const QColor & cl, uint w, PenStyle(pen的stype) s, PenCapStyle(端点) c, PenJoinStyle j )等
void QPen::setWidth ( uint w )
void QPen::setStyle ( PenStyle s )
void QPen::setColor ( const QColor & c )
void QPen::setColor ( const QColor & c )
Qt::PenCapStylr
Qt:PenStype
自定义画笔:
QPen pen;
QVector<qreal> dashes;
qreal space = 4; dashes << 1 << space << 3 << space << 9 <<space << 27 <<space << 9 << space;
pen.setDashPattern(dashes);
Join stype连接样式:QT::PenJoinStype
Qbrush类:
The QBrush class defines the fill pattern of shapes drawn by a QPainter.
A brush has a style and a color. One of the brush styles is a custom pattern, which is defined by a QPixmap.
The brush style defines the fill pattern. The default brush style is NoBrush (depending on how you construct a brush). This style tells the painter to not fill shapes. The standard style for filling is SolidPattern.
The brush color defines the color of the fill pattern. The QColor documentation lists the predefined colors.
Use the QPen class for specifying line/outline styles.
Example:
QPainter painter;
QBrush brush( yellow ); // yellow solid pattern
painter.begin( &anyPaintDevice ); // paint something
painter.setBrush( brush ); // set the yellow brush
painter.setPen( NoPen ); // do not draw outline
painter.drawRect( 40,30, 200,100 ); // draw filled rectangle
painter.setBrush( NoBrush ); // do not fill
painter.setPen( black ); // set black pen, 0 pixel width
painter.drawRect( 10,10, 30,20 ); // draw rectangle outline
painter.end(); // painting done
See the setStyle() function for a complete list of brush styles.
Qpainter简单图形绘制
QPainter ( const QPaintDevice * pd, const QWidget * copyAttributes, bool unclipped = FALSE )
void SimpleExampleWidget::paintEvent()
{
QPainter paint( this );
paint.setPen( Qt::blue );
paint.drawText( rect(), AlignCenter, "The Text" );
}
最简单的绘制函数:drawPoint()、drawPoints()、drawLine()、drawRect()、drawWinFocusRect()、drawRoundRect()、drawEllipse()、drawArc()、drawPie()、drawChord()、drawLineSegments()、drawPolyline()、drawPolygon()、drawConvexPolygon()和drawCubicBezier()
绘制像素映射/图像的函数,名为drawPixmap()、drawImage()和drawTiledPixmap()
drawText()可以完成文本绘制
drawPicture()函数,用来使用这个绘制工具绘制整个QPicture的内容
setViewport()设置QPainter操作的矩形
isActive()指出绘制工具是否是激活的。begin()(和最常用的构造函数)使它激活。end()(和析构函数)释放它们。如果绘制工具是激活的,device()返回绘制工具在哪个绘制设备上绘制。
3.0.1. void QPainter::drawPicture ( int x, int y, const QPicture & pic )
在(x, y)重放图片pic。
当这个函数被调用时,如果(x, y) = (0, 0),它就和QPicture::play()一样了。
QPainterPath 类(绘图路径)提供了一个容器,用于绘图操作,可以创建和重用图形形状。QPainterPath 的主要优点在于:复杂的图形只需创建一次,然后只需调用 QPainter::drawPath() 函数即可绘制多次。QPainterPath 也提供了一些便利的函数来添加一个封闭的子路径 - addEllipse()、addPath()、 addRect()、addRegion() 和 addText()。addPolygon() 函数添加一个未封闭的子路径。事实上,这些函数都是 moveTo()、lineTo()、cubicTo() 操作的集合。
4. 渐变(Gradient)
· ConicalGradient,锥形渐变
· LinearGradient,线性渐变
· RadialGradient,径向渐变
1. //线性渐变
2. QLinearGradientlinearGradient(20,20,150,150);
3. //创建了一个QLinearGradient对象实例,参数为起点和终点坐标,可作为颜色渐变的方向
4. //painter.setPen(Qt::NoPen);
5. linearGradient.setColorAt(0.0,Qt::green);
6. linearGradient.setColorAt(0.2,Qt::white);
7. linearGradient.setColorAt(0.4,Qt::blue);
8. linearGradient.setColorAt(0.6,Qt::red);
9. linearGradient.setColorAt(1.0,Qt::yellow);
10. painter.setBrush(QBrush(linearGradient));
11. painter.drawEllipse(10,10,200,200);
12. //前面为左边,后面两个参数为横轴和纵轴,上面的四行分别设置渐变的颜色和路径比例
1. //辐射渐变
2. QRadialGradientradialGradient(310,110,100,310,110);
3. //创建了一个QRadialGradient对象实例,参数分别为中心坐标,半径长度和焦点坐标,如果需要对称那么中心坐标和焦点坐标要一致
4. radialGradient.setColorAt(0,Qt::green);
5. //radialGradient.setColorAt(0.2,Qt::white);
6. radialGradient.setColorAt(0.4,Qt::blue);
7. //radialGradient.setColorAt(0.6,Qt::red);
8. radialGradient.setColorAt(1.0,Qt::yellow);
9. painter.setBrush(QBrush(radialGradient));
10. painter.drawEllipse(210,10,200,200);//在相应的坐标画出来
11. //弧度渐变
12. QConicalGradientconicalGradient(510,110,0);
13. //创建了一个QConicalGradient对象实例,参数分别为中心坐标和初始角度
14. conicalGradient.setColorAt(0,Qt::green);
15. conicalGradient.setColorAt(0.2,Qt::white);
16. conicalGradient.setColorAt(0.4,Qt::blue);
17. conicalGradient.setColorAt(0.6,Qt::red);
18. conicalGradient.setColorAt(0.8,Qt::yellow);
19. conicalGradient.setColorAt(1.0,Qt::green);
通过响应鼠标进行绘图(鼠标事件进行重新定义)
void DrawWidget::mousePressEvent(QMouseEvent *e)
{
startPos = e->pos();
}
void DrawWidget::mouseMoveEvent(QMouseEvent *e)
{
QPainter *painter = new QPainter;
QPen pen;
pen.setStyle((Qt::PenStyle)style);
pen.setWidth(weight);
pen.setColor(color);
painter->begin(pix);
painter->setPen(pen);
painter->drawLine(startPos,e->pos());
painter->end();
startPos =e->pos();
update();
}
void DrawWidget::paintEvent(QPaintEvent *)
{
QPainter painter(this);
painter.drawPixmap(QPoint(0,0),*pix);
}
void DrawWidget::resizeEvent(QResizeEvent *event)
{
if(height()>pix->height()||width()>pix->width())
{
QPixmap *newPix = new QPixmap(size());
newPix->fill(Qt::white);
QPainter p(newPix);
p.drawPixmap(QPoint(0,0),*pix);
pix = newPix;
}
QWidget::resizeEvent(event);
}
void DrawWidget::clear()
{
QPixmap *clearPix =new QPixmap(size());
clearPix->fill(Qt::white);
pix = clearPix;
update();
}
Svg的显示:
#ifndef SVGWIDGET_H
#define SVGWIDGET_H
#include <QSvgWidget>
#include <QtSvg>
#include <QSvgWidget>
#include <QSvgRenderer>
class SvgWidget : public QSvgWidget
{
Q_OBJECT
public:
SvgWidget(QWidget *parent=0);
void wheelEvent(QWheelEvent *); //响应鼠标的滚轮事件,使SVG图片能通过鼠标的滚轮进行缩放
private:
QSvgRenderer *render; //用于图片显示尺寸的确定
};
#endif // SVGWIDGET_H
void SvgWidget::wheelEvent(QWheelEvent *e)
{
const double diff=0.1;
QSize size =render->defaultSize();
int width =size.width();
int height =size.height();
if(e->delta()>0)
{
width =int(this->width()+this->width()*diff);
height =int(this->height()+this->height()*diff);
}
else
{
width =int(this->width()-this->width()*diff);
height =int(this->height()-this->height()*diff);
}
resize(width,height);
}
Graphics view图形视图框架
三元素:
场景类,放置图元的容器,本身不可见,为图元提供操作接口,传递事件,管理状态,提供无变换的绘制功能,通过addItem()添加图元到场景中,items返回所有图元,itemat返回指定点最顶层的图元。
SetFocusItem(),setfocus(),render()
视图类:提供可视窗口来显示图元,matrix() maptoscene(),mapfromscene()
图元类:处理鼠标,键盘,拖曳,分组,碰撞检测
坐标问题,视图类以左上角为(0,0)其他两中心为(0,0)
变换的坐标函数:maptoscene mapfromscene maptoparent mapfromparent maptoitem mapfromitem
飞舞的蝴蝶:
#ifndef BUTTERFLY_H
#define BUTTERFLY_H
#include <QObject>
#include <QGraphicsItem>
#include <QPainter>
#include <QGraphicsScene>
#include <QGraphicsView>
class Butterfly : public QObject,public QGraphicsItem
{
Q_OBJECT
public:
explicit Butterfly(QObject *parent = 0);
~Butterfly();
void timerEvent(QTimerEvent *); //定时器实现翅膀振动
QRectF boundingRect() const; //图元区域限定范围,所有QGraphiItem都必须实现这个函数
protected:
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget);
private:
bool up;
QPixmap pix_up; //用于表示两幅蝴蝶的图片
QPixmap pix_down;
qreal angle; //相当于double或者float
};
#endif // BUTTERFLY_H
#include "butterfly.h"
#include<math.h>
const static double PI=3.1416;
Butterfly::Butterfly(QObject *parent) : QObject(parent)
{
up = true;
pix_up.load("up.png");
pix_down.load("down.png");
startTimer(100);
}
QRectF Butterfly::boundingRect() const
{
qreal adjust =2;
return QRectF(-pix_up.width()/2-adjust,-pix_up.height()/2-adjust,pix_up.width()+adjust*2,pix_up.height()+adjust*2);
}
void Butterfly::paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)
{
if(up)
{
painter->drawPixmap(boundingRect().topLeft(),pix_up);
up=!up;
}
else
{
painter->drawPixmap(boundingRect().topLeft(),pix_down);
up=!up;
}
}
void Butterfly::timerEvent(QTimerEvent *)
{
//边界控制
qreal edgex=scene()->sceneRect().right()+boundingRect().width()/2;
qreal edgetop=scene()->sceneRect().top()+boundingRect().height()/2;
qreal edgebottom=scene()->sceneRect().bottom()+boundingRect(). height()/2;
if(pos().x()>=edgex)
setPos(scene()->sceneRect().left(),pos().y());
if(pos().y()<=edgetop)
setPos(pos().x(),scene()->sceneRect().bottom());
if(pos().y()>=edgebottom)
setPos(pos().x(),scene()->sceneRect().top());
angle+=(qrand()%10)/20.0;
qreal dx=fabs(sin(angle*PI)*10.0);
qreal dy=(qrand()%20)-10.0;
setPos(mapToParent(dx,dy));
}
Butterfly::~Butterfly()
{
}
#include "mainwindow.h"
#include <QApplication>
#include "butterfly.h"
#include <QGraphicsScene>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QGraphicsScene *scene = new QGraphicsScene;
scene->setSceneRect(QRectF(-200,-200,400,400));
Butterfly *butterfly = new Butterfly;
butterfly->setPos(-100,0);
scene->addItem(butterfly);
QGraphicsView *view = new QGraphicsView;
view->setScene(scene);
view->resize(400,400);
view->show();
return a.exec();
}
地图浏览器:
#include "mapwidget.h"
#include <QSlider>
#include <QFile>
#include <QGridLayout>
#include <QTextStream>
#include <math.h>
/*
* 1、setCacheMode(CacheBackground)这个属性控制view的那一部分缓存中,QGraphicsView可以预存一些内容在QPixmap中,
* 然后被绘制到viewpoint上,这样做的目的是加速整体区域重绘的速度,例如:质地、倾斜度、和最初的混合背景可能重绘很缓慢,
* 尤其是在一个变形的view中,CacheBackground标志使能view的背景缓存,例如
* QGraphicsView view;
* view.setBackgroundBrush(QImage(":/images/backgroundtile.png"));
* view.setCacheMode(QGraphicsView::CacheBackground);
* 每次view转换后cache就无效了,然而,当滚动区域时候,只有部分无效默认的,没有使用cache
* 2、setTickInterval(int)来设置发射信号的间隔,一般都设置为1000ms,就是1s发射一次
* 3、setScaledContents(bool)这个属性保存标签是否按这个图片的比例填满所用的可用空间,默认false
*/
MapWidget::MapWidget()
{
readMap (); //读取地图信息
zoom = 50; //初始比例设置为50%
int width = map.width (); //获取当前地图的宽度
int height = map.height (); //获取当前地图的高度
QGraphicsScene *scene = new QGraphicsScene(this); //定义一个场景指针类
scene->setSceneRect (-width / 2, -height / 2, width, height); //设置显示的场景大小
setScene (scene);
setCacheMode (CacheBackground);
//用于地图缩放的滑动条
QSlider *slider = new QSlider;
slider->setOrientation (Qt::Vertical); //设置垂直显示
slider->setRange (1, 100);
slider->setTickInterval (10);
slider->setValue (50);
connect (slider, SIGNAL(valueChanged(int)), this, SLOT(slotZoom(int)));
QLabel *zoominLabel = new QLabel;
zoominLabel->setScaledContents (true);
zoominLabel->setPixmap (QPixmap("zoomin.png"));
QLabel *zoomoutLabel = new QLabel;
zoomoutLabel->setScaledContents (true);
zoomoutLabel->setPixmap (QPixmap("zoomout.png"));
//坐标显示
QLabel *label1 = new QLabel(tr("GraphicsView:"));
viewCoord = new QLabel;
QLabel *label2 = new QLabel(tr("GraphicsScene:"));
sceneCoord = new QLabel;
QLabel *label3 = new QLabel(tr("map:"));
mapCoord = new QLabel;
//坐标显示区布局
QGridLayout *gridLayout = new QGridLayout;
gridLayout->addWidget (label1, 0, 0);
gridLayout->addWidget (viewCoord, 0, 1);
gridLayout->addWidget (label2, 1, 0);
gridLayout->addWidget (sceneCoord, 1, 1);
gridLayout->addWidget (label3, 2, 0);
gridLayout->addWidget (mapCoord, 2, 1);
QFrame *coorFrame = new QFrame;
coorFrame->setLayout (gridLayout);
//缩放控制子布局(垂直设置布局)
QVBoxLayout *zoomLayout = new QVBoxLayout;
zoomLayout->addWidget (zoominLabel);
zoomLayout->addWidget (slider);
zoomLayout->addWidget (zoomoutLabel);
//坐标显示区域布局(垂直设置布局)
QVBoxLayout *coordLayout = new QVBoxLayout;
coordLayout->addWidget (coorFrame);
coordLayout->addStretch ();
//主布局(水平设置布局)
QHBoxLayout *mainLayout = new QHBoxLayout(this);
mainLayout->addLayout (zoomLayout);
mainLayout->addLayout (coordLayout);
mainLayout->addStretch ();
mainLayout->setMargin (15);
mainLayout->setSpacing (15);
setWindowTitle (tr("Map Widget"));
setMinimumSize (600, 400);
}
/*
* 读取地图信息
* 以只读方式打开文件
* 读取文件名字(mapName),x1、x2、y1、y2
* 加载图片
*/
void MapWidget::readMap ()
{
QString mapName;
QFile mapFile("maps.txt");
int ok = mapFile.open (QIODevice::ReadOnly);
if(ok)
{
QTextStream ts(&mapFile);
if(!ts.atEnd ())
{
ts >> mapName;
ts >> x1 >> x2 >> y1 >> y2;
}
}
map.load (mapName);
}
/*
* 从场景坐标到地图坐标的装换
*/
QPointF MapWidget::mapToMap (QPointF p)
{
QPointF latLon;
qreal w = sceneRect ().width ();
qreal h = sceneRect ().height ();
qreal lon = y1 - ((h / 2 + p.y ()) * abs(y1 - y2) / h);
qreal lat = x1 + ((w / 2 + p.x ()) * abs(x1 - x2) / w);
latLon.setX (lat);
latLon.setY (lon);
return latLon;
}
/*
* 检测value(slider改变得到的值),与当前value值得大小比较
* pow(x, y)表示x的y次方
* slider改变的值大于zoom值时,增加缩放比例
* slider改变的值小于zoom值时,减小缩放比例
* scale(s, s)将当前的视图装换为(s, s)
*/
void MapWidget::slotZoom (int value)
{
qreal s;
if(value > zoom)
{
s = pow(1.01, (value - zoom));
}
else
{
s = pow(1 / 1.01, (zoom - value));
}
scale(s, s);
zoom = value;
}
/*
* 绘制背景色
* 从左上方开始绘制
*/
void MapWidget::drawBackground (QPainter *painter, const QRectF &rect)
{
painter->drawPixmap (int(sceneRect ().left ()), int(sceneRect ().top ()), map);
}
/*
* 当鼠标按下移动时获取鼠标当前的位置信息(分别为x,y方向的值)
*/
void MapWidget::mouseMoveEvent (QMouseEvent *event)
{
//QGraphicsView坐标
QPoint viewPoint = event->pos ();
viewCoord->setText (QString::number (viewPoint.x ()) + " , " + QString::number (viewPoint.y ()));
//QGraphicsScene坐标
QPointF scenePoint = mapToScene (viewPoint);
sceneCoord->setText (QString::number (scenePoint.x ()) + " , " + QString::number (scenePoint.y ()));
//地图坐标经度纬度值
QPointF latLon = mapToMap (scenePoint);
mapCoord->setText (QString::number (latLon.x ()) + " , " + QString::number (latLon.y ()));
}
添加图元:
#ifndef PIXITEM_H
#define PIXITEM_H
#include <QGraphicsItem>
#include <QPixmap>
#include <QPainter>
class PixItem : public QGraphicsItem
{
public:
PixItem(QPixmap *pixmap);
QRectF boundingRect() const;
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget);
private:
QPixmap pix; //作为图元显示的图片
};
#endif // PIXITEM_H
#include "pixitem.h"
PixItem::PixItem(QPixmap *pixmap)
{
pix = *pixmap;
}
QRectF PixItem::boundingRect() const
{
return QRectF(-2-pix.width()/2,-2-pix.height()/2,pix.width()+4, pix.height()+4);
}
void PixItem::paint(QPainter *painter, const QStyleOptionGraphicsItem *option,QWidget *widget)
{
painter->drawPixmap(-pix.width()/2,-pix.height()/2,pix);
}
QGraphicsScene *scene = new QGraphicsScene;
scene->setSceneRect(-200,-200,400,400);
QPixmap *pixmap = new QPixmap("image.png");
pixItem = new PixItem(pixmap);
scene->addItem(pixItem);
pixItem->setPos(0,0);
view = new QGraphicsView;
view->setScene(scene);
view->setMinimumSize(400,400);
view->rotate(value-angle);
view->scale(s,s);
view->shear((value-shearValue)/10.0,0);
view->translate(value-translateValue,value-translateValue);
/*
*view的缩放和旋转zoomIn()=scale(1.5,1.5) zoomOut()=scale(1/1.5,1/1.5) rotateleft()=rotate(-90) rotateright()=rotate(90)
*item图元光标setCursor() 提示setToolTop()
*动画
/*在一定时间沿着一定轨迹
* QGraphicsEllipseItem sun=new QGraphicsEllipseItem;
*QTimeLine timeline=new QTimeLine(10000);
*QGraphicsItemAnimation *animation=new QGraphicsItemAnimation;
*animation->setItem(sun);
*animation->setTimeLine(timeline);
* for(int i=0;i<=180;i++)
* {
* x=200*cos(angle);
* y=200*sin(angle);
* animation->setPosAt(i/180,QPointF(x,y));
* angle+=i/180;
* }
*/
*图形效果:
*QGraphicsBlurEffect
该类用应产生模糊效果,主要函数setBlurRadius(qreal blurRadius),用于控制图形元素的模糊度,数值越大越模糊。使用该类例子如下
QGraphicsBlurEffect *e0 = new QGraphicsBlurEffect(this);
e0->setBlurRadius(0.2);
item[0]->setGraphicsEffect(e1);//item[0] 为QGraphicsItem指针
QGraphicsColorizeEffect
该类提供了使用另外一种颜色对当前图形的一种着色功能。主要函数是setColor(QColor)和setStrength (qreal strength),指定了着色和着色强度。使用该类例子如下
QGraphicsColorizeEffect *e1 = new QGraphicsColorizeEffect(this);
e1->setColor(QColor(0,0,192));
item[1]->setGraphicsEffect(e1);
QGraphicsDropShadowEffect
该类提供了图形元素的阴影效果,用于增加立体感。主要设置函数有3个,setColor()用于设定阴影的颜色,setBlurRadius()用于设定 阴影的模糊度,setOffset (qreal dx,qreal dy)用于设定在哪个方向产生阴影效果,如果dx为负数,则阴影在图形元素的左边。使用该类例子如下
QGraphicsDropShadowEffect *e2 = new QGraphicsDropShadowEffect(this);
e2->setOffset(8,8);
item[2]->setGraphicsEffect(e2);
QGraphicsOpacityEffect
该类用于图形元素的透明效果,主要函数是setOpacity(qreal opacity),用于设置透明度,参数值在0和1.0之间。也可以设置部分透明效果,需要调用的函数是setOpacityMask (QBrush mask)。使用该类例子如下
QGraphicsOpacityEffect *e3 = new QGraphicsOpacityEffect(this);
e3->setOpacity(0.7);
item[3]->setGraphicsEffect(e3);
*/
5. 模型视图结构
Mvc设计模式 model view delegate
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QDirModel model;
QTreeView tree;
QListView list;
QTableView table;
tree.setModel(&model);
list.setModel(&model);
table.setModel(&model);
tree.setSelectionMode(QAbstractItemView::MultiSelection);
list.setSelectionModel(tree.selectionModel());
table.setSelectionModel(tree.selectionModel());
QObject::connect(&tree,SIGNAL(doubleClicked(QModelIndex)),&list,SLOT(setRootIndex(QModelIndex)));
QObject::connect(&tree,SIGNAL(doubleClicked(QModelIndex)),&table,SLOT(setRootIndex(QModelIndex)));
QSplitter *splitter = new QSplitter;
splitter->addWidget(&tree);
splitter->addWidget(&list);
splitter->addWidget(&table);
splitter->setWindowTitle(QObject::tr("Model/View"));
splitter->show();
return a.exec();
}
/*
*gQAbstractItemView::SingleSelection;
*QAbstractItemView::NoSelection;
*QAbstractItemView::ContiguousSelection;
*QAbstractItemView::ExtendedSelection;
QAbstractItemView::MultiSelection;:
*/
Model:
QAbstractTableModel
QAbstractListModel
//此代码中四个函数为虚函数,必须重载,
virtual int rowCount(const QModelIndex &parent=QModelIndex()) const;
virtual int columnCount(const QModelIndex &parent=QModelIndex()) const;
QVariant data(const QModelIndex &index, int role) const;
QVariant headerData(int section, Qt::Orientation orientation, int role) const;
虚函数赋予了默认值
#include "modelex.h"
ModelEx::ModelEx(QObject *parent) :
QAbstractTableModel(parent)
{
armyMap[1]=tr("空军");
armyMap[2]=tr("海军");
armyMap[3]=tr("陆军");
armyMap[4]=tr("海军陆战队");
weaponTypeMap[1]=tr("轰炸机");
weaponTypeMap[2]=tr("战斗机");
weaponTypeMap[3]=tr("航空母舰");
weaponTypeMap[4]=tr("驱逐舰");
weaponTypeMap[5]=tr("直升机");
weaponTypeMap[6]=tr("坦克");
weaponTypeMap[7]=tr("两栖攻击舰");
weaponTypeMap[8]=tr("两栖战车");
populateModel();
}
void ModelEx::populateModel()
{
header<<tr("军种")<<tr("种类")<<tr("武器");
army<<1<<2<<3<<4<<2<<4<<3<<1;
weaponType<<1<<3<<5<<7<<4<<8<<6<<2;
weapon<<tr("B-2")<<tr("尼米兹级")<<tr("阿帕奇")<<tr("黄蜂级")<<tr("阿利伯克级")<<tr("AAAV")<<tr("M1A1")<<tr("F-22");
}
int ModelEx::columnCount(const QModelIndex &parent) const
{
return 3;
}
int ModelEx::rowCount(const QModelIndex &parent) const
{
return army.size();
}
QVariant ModelEx::data(const QModelIndex &index, int role) const
{
if(!index.isValid())
return QVariant();
if(role==Qt::DisplayRole)
{
switch(index.column())
{
case 0:
return armyMap[army[index.row()]];
break;
case 1:
return weaponTypeMap[weaponType[index.row()]];
break;
case 2:
return weapon[index.row()];
default:
return QVariant();
}
}
return QVariant();
}
QVariant ModelEx::headerData(int section, Qt::Orientation orientation, int role) const
{
if(role==Qt::DisplayRole&&orientation==Qt::Horizontal)
return header[section];
return QAbstractTableModel::headerData(section,orientation,role);
}
6. 文件及磁盘处理
#include "zfile.h"
#include <QApplication>
#include<QFile>
#include<QString>
#include<QDebug>
#include<QTextStream>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QFile file("/home/zz/temp");
/*
* QIODevice::ReadOnly QIODevice::WriteOnly QIODevice::ReadWrite open use mode or
* QFile::WriteOnly QFile::ReadOnly QFile::ReadWrite QFile::Truncate(to clear)
*/
if(file.open(QFile::ReadOnly))
{
QString str=file.readAll();
qDebug()<<str;
file.close();
}
QFile file2("/home/zz/test003");
if(file2.open(QFile::WriteOnly|QFile::Truncate))//append追加
{
QTextStream out(&file2);
/*
*Format control
* bin(oct dec hex) binary to write
* showbase to show Prefix as 0x 0 0b
* left right center
*/
out<<"Like a person who starts with Yan value, "
"is respectful of talent, unites with character, is good at being good, and finally has character.";
}
/*to write and read binary data*/
QFile file3("/home/zz/test004");
if(file3.open(QFile::WriteOnly|QFile::Truncate|QFile::ReadOnly))
{
QDataStream out(&file3);//Data serialization
out<<QString(QObject::tr("zylg"));
out<<(qint32)20;
file3.close();
}
if(file3.open(QFile::ReadOnly))
{
QDataStream in(&file3);
qint32 age;
QString name;
in>>name>>age;
qDebug()<<name<<age;
}
return a.exec();
}
6.1. 目录文件系统
Qdir:有绝对路径和相对路径之说,用isRelative()和isAbsolute()判断。
makeAbsolute()让相对变成绝对路径。
Path() absolutepath()可以返回路径
Setpath() cd() cdUp() mkdir() rename()改变目录名
Exists()判断目录是否存在
属性可以用isreadable() isabsolute() isrelative() isroot()查看
目录下的数目可以使用count()返回
删除文件remove() 删除目录rmdir()
entryList返回目录下所有条目组成的字符串链表
Ex:
#include <QCoreApplication>
#include <QStringList>
#include <QDir>
#include <QtDebug>
#include<QFile>
#include<QTextStream>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QDir dir("/home/zz");//absolute path dir("hello.txt")relative path
if(!dir.isRelative())qDebug()<<"The path is absolute Path\n";
/*
* dir.isAbsolute() dir.isRoot()
*/
qDebug()<<dir.path();//to show the path of dir
QFile file1("hello.txt");
QTextStream out(&file1);
if(file1.open(QFile::WriteOnly))out<<"we are chinese";
file1.close();
dir.setPath("hello.txt");
qDebug()<<dir.absolutePath();//to return the absolute path of hello.txt
dir.cd("/home/zz");
dir.cdUp();//cdUP==cd("../")
qDebug()<<dir.path();
dir.cd("/home/zz/");
dir.mkdir("test001");
dir.cd("test001");
dir.cd("../");
dir.rename("test001","test002");
dir.rmdir("test002"); //if have files,can`t to delete
/*to delete test002
* dir.cd("test002");
* foreach (QString name, dir.entryList(QDir::Files))
* {
* dir.remove(name);
* }
* dir.cd("../");
* dir.rmdir("test002");
*/
if(dir.exists("test002"))qDebug()<<"delete fail";
int i=1;
qDebug()<<dir.count()<<dir.current()<<dir.home();
/*
* QDirQStringReturn Value
*current()currentPath()The application's working directory
*home()homePath()The user's home directory
*root()rootPath()The root directory
*temp()tempPath()The system's temporary directory
*/
foreach(QString str , dir.entryList(QDir::Files|QDir::AllDirs))
{
qDebug()<<i<<str;++i;
}
dir.setFilter(QDir::Files | QDir::Hidden | QDir::NoSymLinks);
dir.setSorting(QDir::Size | QDir::Reversed);
QStringList list=dir.entryList();
for(int i=0;i<list.size();i++)qDebug()<<(QString)list.at(i);
return a.exec();
}
6.2. 监视文件和目录变化
QFileSystemWatcher fsWatcher;
fsWatcher.addPath(path);
connect(&fsWatcher,SIGNAL(directoryChanged(QString)),this,SLOT(directoryChanged(QString)));
7. 网络与通信
7.1. 获取本机信息
QString localHostName = QHostInfo::localHostName();
QHostInfo hostInfo = QHostInfo::fromName(localHostName);
QList<QHostAddress> listAddress = hostInfo.addresses();
QList<QNetworkInterface> list=QNetworkInterface::allInterfaces();
for(int i=0;i<list.count();i++)
{
QNetworkInterface interface=list.at(i);
detail=detail+tr("设备:")+interface.name()+"\n";
detail=detail+tr("硬件地址:")+interface.hardwareAddress()+"\n";
QList<QNetworkAddressEntry> entryList=interface.addressEntries();
for(int j=0;j<entryList.count();j++)
{
QNetworkAddressEntry entry=entryList.at(j);
detail=detail+"\t"+tr("IP 地址:")+entry.ip().toString()+"\n";
detail=detail+"\t"+tr("子网掩码:")+entry.netmask().toString()+"\n";
detail=detail+"\t"+tr("广播地址:")+entry.broadcast().toString()+"\n";
}
}
7.2. Udp数据传输
Server:
int port;
QUdpSocket *udpSocket;
port =5555;
udpSocket = new QUdpSocket(this);
QString msg = TextLineEdit->text();
udpSocket->writeDatagram(msg.toLatin1(),msg.length(),QHostAddress::Broadcast,port)
Client:
udpSocket->bind(port);
while(udpSocket->hasPendingDatagrams())
{
QByteArray datagram;
datagram.resize(udpSocket->pendingDatagramSize());
udpSocket->readDatagram(datagram.data(),datagram.size());
QString msg=datagram.data();
ReceiveTextEdit->insertPlainText(msg);
}
Tcp:
Qtcpserver:
QHostAddress::Null //空地址对象
QHostAddress::LocalHost //监听本地主机IPv4,相当于QHostAddress("127.0.0.1") QHostAddress::LocalHostIPv6 //监听本地主机IPv6,相当于QHostAddress("::1") QHostAddress::Broadcast //IPv4广播地址,相当于QHostAddress("255.255.255.255") QHostAddress::AnyIPv4 //监听当前网卡所有IPv4,相当于QHostAddress("0.0.0.0") QHostAddress::AnyIPv6 //监听当前网卡所有IPv6,相当于QHostAddress("::")
HostAddress::Any //监听当前网卡的所有IP
8. 事件处理
8.1. 鼠标事件
void mousePressEvent(QMouseEvent *e);
void mouseMoveEvent(QMouseEvent *e);
void mouseReleaseEvent(QMouseEvent *e);
void mouseDoubleClickEvent(QMouseEvent *e);
void MouseEvent::mousePressEvent(QMouseEvent *e)
{
QString str="("+QString::number(e->x())+","+QString::number(e->y())+")";
if(e->button()==Qt::LeftButton)
{
statusBar()->showMessage(tr("左键:")+str);
}
else if(e->button()==Qt::RightButton)
{
statusBar()->showMessage(tr("右键:")+str);
}
else if(e->button()==Qt::MidButton)
{
statusBar()->showMessage(tr("中键:")+str);
}
}
void MouseEvent::mouseMoveEvent(QMouseEvent *e)
{
MousePosLabel->setText("("+QString::number(e->x())+", "+QString::number(e->y())+")");
}
void MouseEvent::mouseReleaseEvent(QMouseEvent *e)
{
QString str="("+QString::number(e->x())+","+QString::number(e->y())+")";
statusBar()->showMessage(tr("释放在:")+str,3000);
}
void MouseEvent::mouseDoubleClickEvent(QMouseEvent *e){}
8.2. 键盘事件
void keyPressEvent(QKeyEvent *);
void paintEvent(QPaintEvent *);
void KeyEvent::keyPressEvent(QKeyEvent *event)
{
if(event->modifiers()==Qt::ControlModifier)
{
if(event->key()==Qt::Key_Left)
{
startX=(startX-1<0)?startX:startX-1;
}
if(event->key()==Qt::Key_Right)
{
startX=(startX+1+image.width()>width)?startX:startX+1;
}
if(event->key()==Qt::Key_Up)
{
startY=(startY-1<0)?startY:startY-1;
}
if(event->key()==Qt::Key_Down)
{
startY=(startY+1+image.height()>height)?startY:startY+1;
}
}
else
{
startX=startX-startX%step;
startY=startY-startY%step;
if(event->key()==Qt::Key_Left)
{
startX=(startX-step<0)?startX:startX-step;
}
if(event->key()==Qt::Key_Right)
{
startX=(startX+step+image.width()>width)?startX:startX+step;
}
if(event->key()==Qt::Key_Up)
{
startY=(startY-step<0)?startY:startY-step;
}
if(event->key()==Qt::Key_Down)
{
startY=(startY+step+image.height()>height)?startY:startY+step;
}
if(event->key()==Qt::Key_Home)
{
startX=0;
startY=0;
}
if(event->key()==Qt::Key_End)
{
startX=width-image.width();
startY=height-image.height();
}
}
drawPix();
update();
}
9. 多线程
9.1. 互斥量
现线程之间存在这同步和互斥两种关系,控制互斥的类有QMutex,QMutexLocker,QReadWriteLocker,QReadLocker,QWriteLocker,QSemaphore,QWaitCondition
class KK
{
public:
KK(int k=0):key(k){}
int key;
int addkey(){QMutex::lock();++key;QMutex::unlock();}
int value()const{return key;}
};
但是QMutexLocker会在局部变量结束只有释放被锁量
class KK
{
public:
KK(int k=0):key(k){}
int key;
int addkey(){QMutexLocker::locker(&key);++key;}
int value()const{return key;}
};
const int DataSize=1000;
const int BufferSize=80;
int buffer[BufferSize];
QSemaphore freeBytes(BufferSize);//表示有80个缓存单元可被利用
QSemaphore usedBytes(0);//一开始没有数据块可以进行读写
void Producer::run()
{
for(int i=0;i<DataSize;i++)
{
freeBytes.acquire();//获得一个数据块
buffer[i%BufferSize]=(i%BufferSize);
usedBytes.release();//一个数据块可以被使用(释放了一个资源)
}
}
void Consumer::run()
{
for(int i=0;i<DataSize;i++)//
{
usedBytes.acquire();/获取可以使用的数据块
fprintf(stderr,"%d",buffer[i%BufferSize]);
if(i%16==0&&i!=0)
fprintf(stderr,"\n");
freeBytes.release();
}
fprintf(stderr,"\n");
}
9.2. 线程等待与唤醒
#include <QCoreApplication>
#include <QWaitCondition>
#include <QMutex>
#include <QThread>
#include <stdio.h>
const int DataSize=1000;
const int BufferSize=80;
int buffer[BufferSize];
QWaitCondition bufferEmpty;
QWaitCondition bufferFull;
QMutex mutex;
int numUsedBytes=0;
int rIndex=0;
class Producer : public QThread
{
public:
Producer();
void run();
};
Producer::Producer()
{
}
void Producer::run()
{
for(int i=0;i<DataSize;i++)
{
mutex.lock();
if(numUsedBytes==BufferSize)
bufferEmpty.wait(&mutex);
buffer[i%BufferSize]=numUsedBytes;
++numUsedBytes;
bufferFull.wakeAll();
mutex.unlock();
}
}
class Consumer : public QThread
{
public:
Consumer();
void run();
};
Consumer::Consumer()
{
}
void Consumer::run()
{
forever
{
mutex.lock();
if(numUsedBytes==0)
bufferFull.wait(&mutex);
printf("%ul::[%d]=%d\n",currentThreadId(),rIndex,buffer[rIndex]);
rIndex=(++rIndex)%BufferSize;
--numUsedBytes;
bufferEmpty.wakeAll();//线程唤醒
mutex.unlock();
}
printf("\n");
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
Producer producer;
Consumer consumerA;
Consumer consumerB;
producer.start();
consumerA.start();
consumerB.start();
producer.wait();
consumerA.wait();
consumerB.wait();
return a.exec();
}
10. 数据库
#include <QApplication>
#include <QTextCodec>
#include <QSqlDatabase>
#include <QSqlQuery>
#include <QTime>
#include <QSqlError>
#include<QDebug>
#include <QSqlDriver>
#include <QSqlRecord>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QTextCodec::setCodecForLocale(QTextCodec::codecForLocale());
QSqlDatabase db =QSqlDatabase::addDatabase("QSQLITE");
db.setHostName("zylg"); //设置数据库主机名
db.setDatabaseName("student1.db"); //设置数据库名
db.setUserName("zz"); //设置数据库用户名
db.setPassword("123"); //设置数据库密码
db.open(); //打开连接
//创建数据库表
QSqlQuery query;
bool success=query.exec("create table info(id int primary key,name vchar)");
if(success)
qDebug()<<QObject::tr("Database table create success!\n");
else
qDebug()<<QObject::tr("Database table create failed!\n");
//查询
query.exec("select * from info");
QSqlRecord rec = query.record();
qDebug() << QObject::tr("info:" )<< rec.count();
//插入记录
QTime t;
t.start();
query.prepare("insert into info values(?,?)");
long records=100;
for(int i=0;i<records;i++)
{
query.bindValue(0,i);
query.bindValue(1,"四轮");
query.bindValue(2,"轿车");
query.bindValue(3,"富康");
query.bindValue(4,rand()%100);
query.bindValue(5,rand()%10000);
query.bindValue(6,rand()%300);
query.bindValue(7,rand()%200000);
query.bindValue(8,rand()%52);
query.bindValue(9,rand()%100);
success=query.exec();
if(!success)
{
QSqlError lastError=query.lastError();
qDebug()<<lastError.driverText()<<QString(QObject::tr("inserted failed"));
}
}
qDebug()<<QObject::tr("insert %1 counts recored,use time:%2 ms").arg(records).arg(t.elapsed());
//排序
t.restart();
success=query.exec("select * from info order by id desc");
if(success)
qDebug()<<QObject::tr("order %1 counts,use time:%2 ms").arg(records).arg(t.elapsed());
else
qDebug()<<QObject::tr("order failed!");
//更新记录
t.restart();
for(int i=0;i<records;i++)
{
query.clear();
query.prepare(QString("update info set name=? where id=%1").arg(i));
query.bindValue(1,"四轮");
query.bindValue(2,"轿车");
success=query.exec();
if(!success)
{
QSqlError lastError=query.lastError();
qDebug()<<lastError.driverText()<<QString(QObject::tr("update failed"));
}
}
qDebug()<<QObject::tr("uodate %1 counts,use time:%2 ms").arg(records).arg(t.elapsed());
//删除
t.restart();
query.exec("delete from automobil where id=1");
qDebug()<<QObject::tr("delete one record and use time:%1 ms").arg(t.elapsed());
return a.exec();
}
11. QML编程基础
11.1. 基础语法
Qml文挡构成:import部分和对象声明,每一个qml只有一个对象
对象和属性:“属性名:值”
对象标识符:id
属性别名:“property alias 别名:属性名”
注释:和c++一样
Qml可视元素:
Text image butoon 等
定位:
Row
{
X:25
Y:25
Spacing:30
Text{}
Text{}
}
Column
{
}
Grid
{
}
Flow
{
}
重复器:
Row
{
Repeater
{
model:20
x:25
y:25
Text{text:"nihao"}
}
spacing: 50
}
锚:
anchors.left: image1.left
11.2. 事件处理
有个mouseArea的东西 Onclick()
键盘事件:
Keys.***
输入控件与焦点:
Focus:true
QML动画
懒得写........................