一、基本介绍
1. SVG 指可伸缩矢量图形 (Scalable Vector Graphics)
2. SVG 用于定义用于网络的基于矢量的图形
3. SVG 使用 XML 格式定义图形
4. SVG 图像在放大或改变尺寸的情况下其图形质量不会有损失
5. SVG 是万维网联盟的标准
- SVG 的优势,与其他图像格式相比(比如 JPEG 和 GIF),使用 SVG 的优势在于:
1. SVG 图像可通过文本编辑器来创建和修改
2. SVG 图像可被搜索、索引、脚本化或压缩
3. SVG 是可伸缩的
4. SVG 图像可在任何的分辨率下被高质量地打印
5. SVG 可在图像质量不下降的情况下被放大
属性 |
描述 |
xmlns |
定义 SVG 命名空间 |
version |
定义所使用的 SVG 版本 |
width |
设置此 SVG 文档的宽度 |
height |
设置此 SVG 文档的高度 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="200" width="200">
<polygon points="100,10 40,180 190,60 10,60 160,180"
style="fill:lime;stroke:purple;stroke-width:5;fill-rule:evenodd;" />
</svg>
</body>
<script>
</script>
</html>
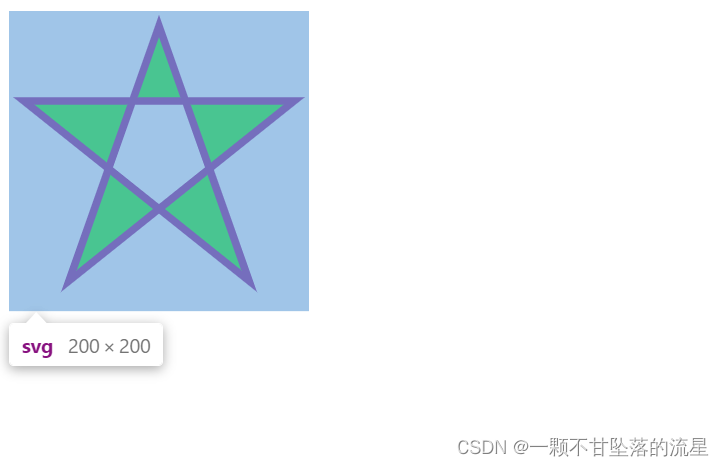
二、用法详解
属性 |
描述 |
fill |
定义图形的填充颜色 |
stroke-width |
定义图形边框的宽度 |
stroke |
定义图形边框的颜色 |
fill-opacity |
定义填充颜色透明度(合法的范围是:0 - 1) |
stroke-opacity |
定义笔触颜色的透明度(合法的范围是:0 - 1) |
opacity |
定义整个元素的透明值 |
2.1、矩形(rect)
<rect>
标签可用来创建矩形,以及矩形的变种。
属性 |
描述 |
width |
定义矩形的宽度 |
height |
定义矩形的高度 |
style |
定义 CSS 属性 |
x |
定义矩形的左侧位置(例如,x=“0” 定义矩形到浏览器窗口左侧的距离是 0px) |
y |
定义矩形的顶端位置(例如,y=“0” 定义矩形到浏览器窗口顶端的距离是 0px) |
rx 和 ry |
可使矩形产生圆角 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="200" width="200">
<rect x="20" y="20" width="100" height="100" rx="20" ry="20"/>
</svg>
</body>
<script>
</script>
</html>
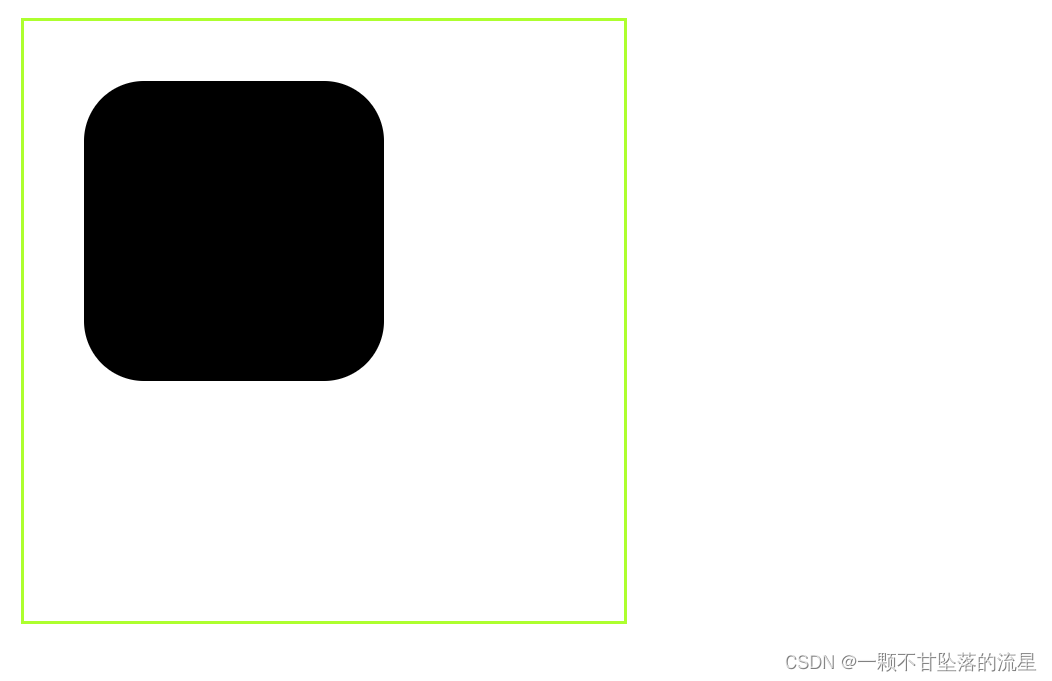
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="200" width="200">
<rect x="20" y="20" width="100" height="100" rx="20" ry="20"
style="fill:red; stroke:black; stroke-width:5; opacity:0.5"/>
</svg>
</body>
<script>
</script>
</html>
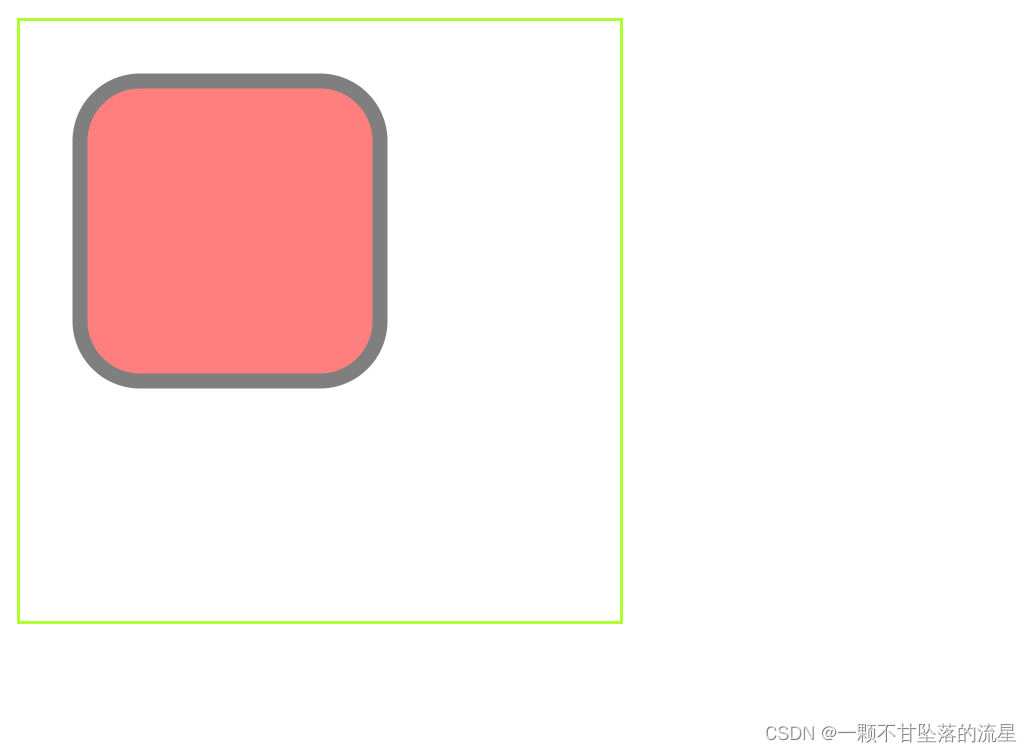
2.2、圆形(circle)
<circle>
标签可用来创建一个圆(如果省略 cx 和 cy,圆的中心会被设置为 (0, 0) )
属性 |
描述 |
r |
定义圆的半径 |
cx |
圆点的 x 坐标 |
cy |
圆点的 y 坐标 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<circle cx="100" cy="50" r="40"/>
</svg>
</body>
<script>
</script>
</html>
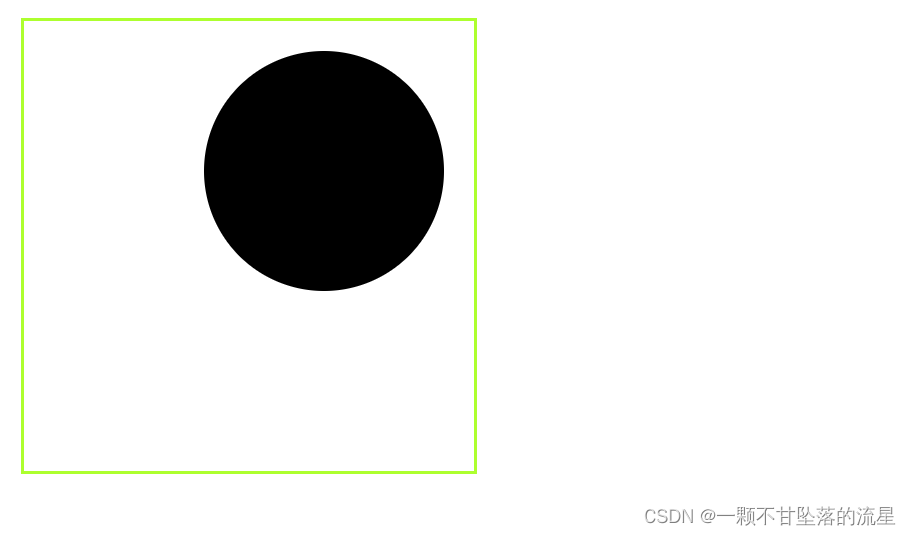
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<circle cx="100" cy="50" r="40" style="fill:red; stroke:black; stroke-width:5; opacity:0.5"/>
</svg>
</body>
<script>
</script>
</html>
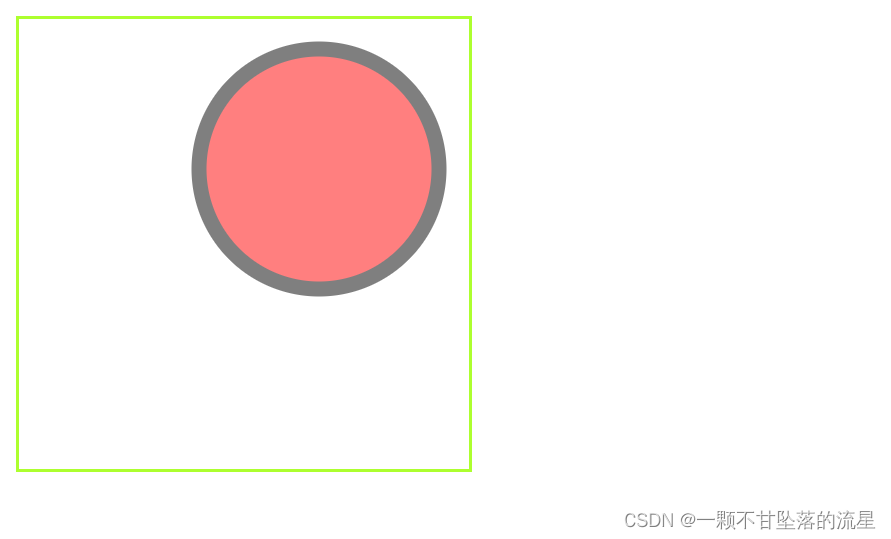
2.3、椭圆(ellipse)
属性 |
描述 |
rx |
定义水平半径 |
ry |
定义垂直半径 |
cx |
圆点的 x 坐标 |
cy |
圆点的 y 坐标 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<ellipse cx="75" cy="75" rx="60" ry="30"/>
</svg>
</body>
<script>
</script>
</html>
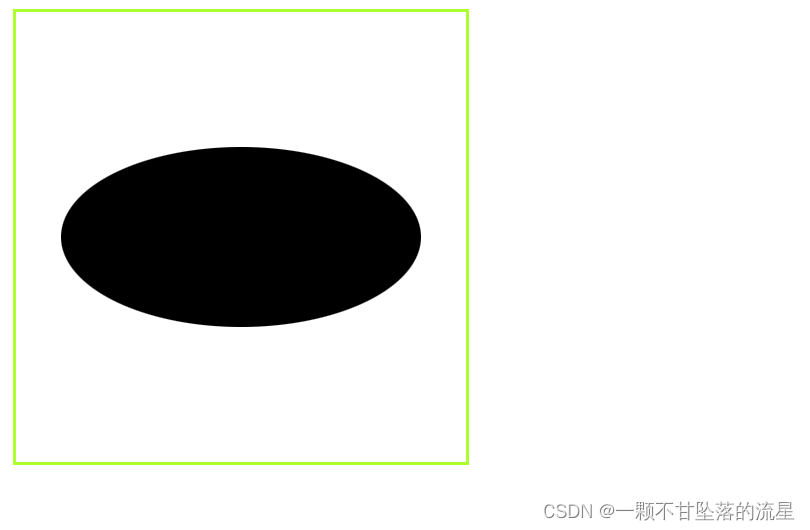
2.4、线条(line)
属性 |
描述 |
x1 |
在 x 轴定义线条的开始 |
y1 |
在 y 轴定义线条的开始 |
x2 |
在 x 轴定义线条的结束 |
y2 |
在 y 轴定义线条的结束 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<line x1="0" y1="10" x2="100" y2="150" style="stroke:red;"/>
</svg>
</body>
<script>
</script>
</html>
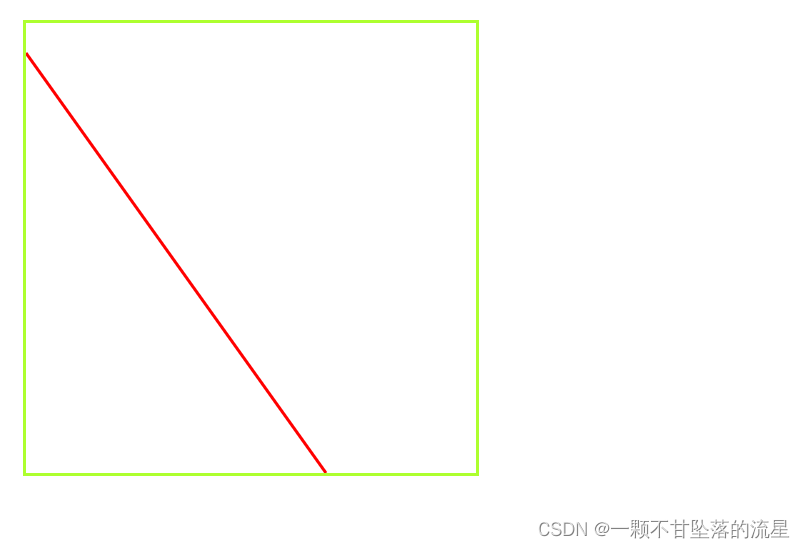
2.5、折线(polyline)
<polyline>
标签用来创建仅包含直线的形状。
属性 |
描述 |
points |
定义折线每个折点的 x 和 y 坐标 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<polyline points="0,0 0,20 20,20 20,40 40,40 40,60"/>
</svg>
</body>
<script>
</script>
</html>
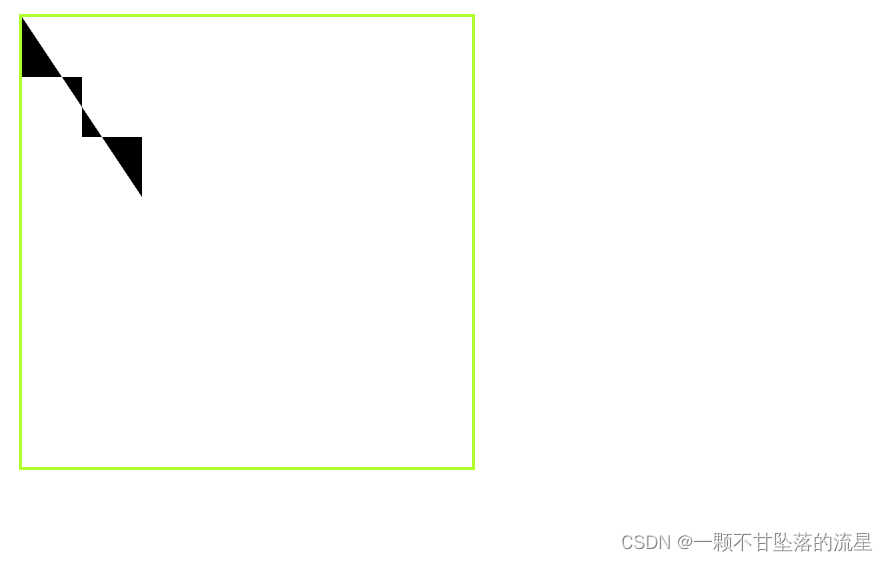
2.6、多边形(polygon)
<polygon>
标签用来创建含有不少于三个边的图形。
属性 |
描述 |
points |
定义多边形每个角的 x 和 y 坐标 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<polygon points="22,10 30,21 17,25"/>
</svg>
</body>
<script>
</script>
</html>
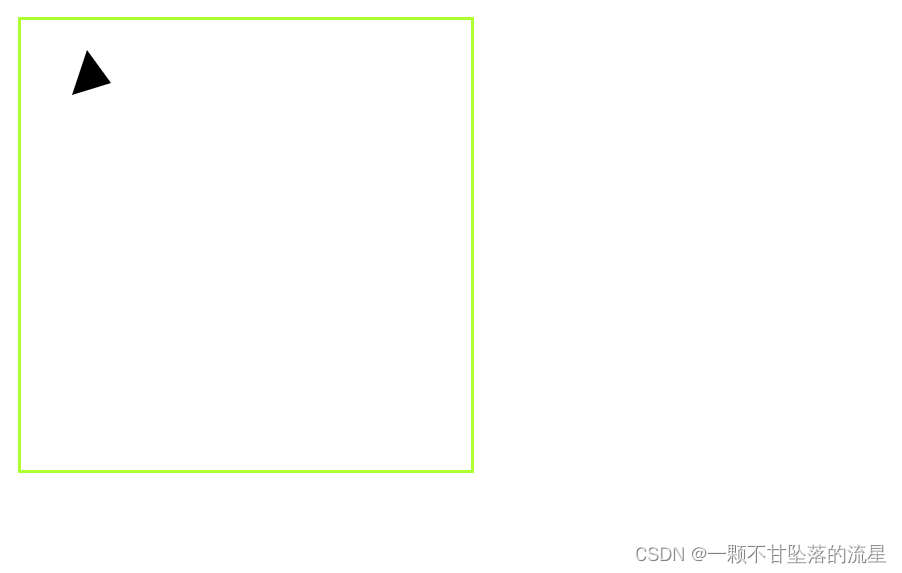
2.7、路径(path)
<path>
标签用来定义路径。
- 以下所有命令均允许小写字母。大写表示绝对定位,小写表示相对定位。
命令 |
描述 |
中文 |
M |
moveto |
移动 |
L |
lineto |
画线 |
H |
horizontal lineto |
横画线 |
V |
vertical lineto |
垂直画线 |
C |
curveto |
曲线 |
S |
smooth curveto |
光滑曲线 |
Q |
quadratic Belzier curve |
二次贝塞尔曲线 |
T |
smooth quadratic Belzier curveto |
二次贝塞尔光滑曲线 |
A |
elliptical Arc |
椭圆弧 |
Z |
closepath |
结束绘制路径 |
- 定义了一条路径,它开始于位置 25 15,到达位置 15 35,然后从那里开始到 35 35,最后在 25 15 关闭路径。
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="150" width="150">
<path d="M25 15 L15 35 L35 35 Z"/>
</svg>
</body>
<script>
</script>
</html>
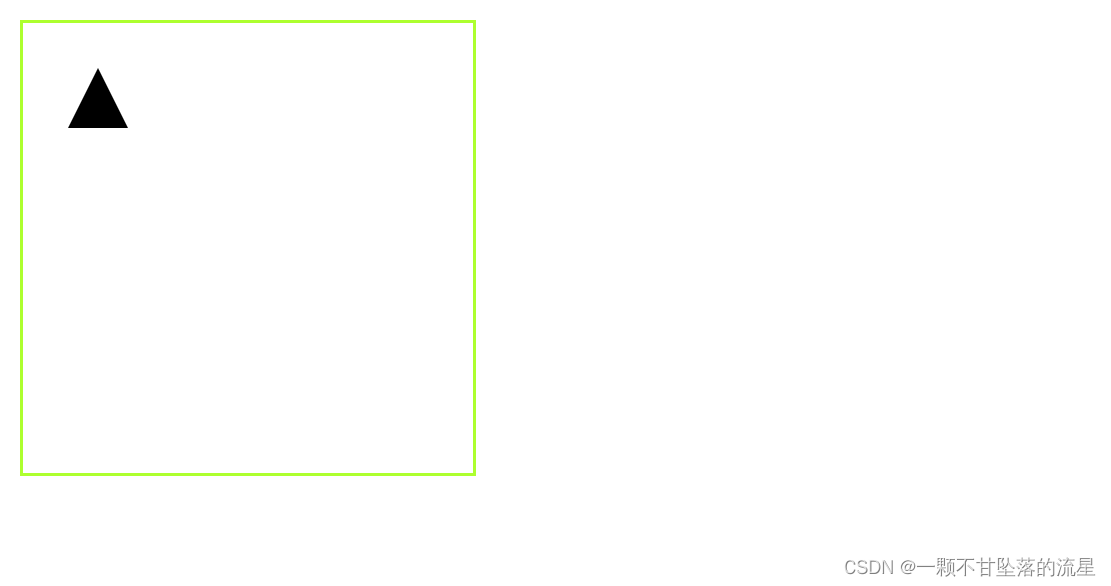
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
svg {
border: 1px greenyellow solid;
}
</style>
</head>
<body>
<svg xmlns="http://www.csnd.org/2000/svg" version="1.1" height="500" width="100%">
<path d="M153 334
C153 334 151 334 151 334
C151 339 153 344 156 344
C164 344 171 339 171 334
C171 322 164 314 156 314
C142 314 131 322 131 334
C131 350 142 364 156 364
C175 364 191 350 191 334
C191 311 175 294 156 294
C131 294 111 311 111 334
C111 361 131 384 156 384
C186 384 211 361 211 334
C211 300 186 274 156 274"/>
</svg>
</body>
<script>
</script>
</html>
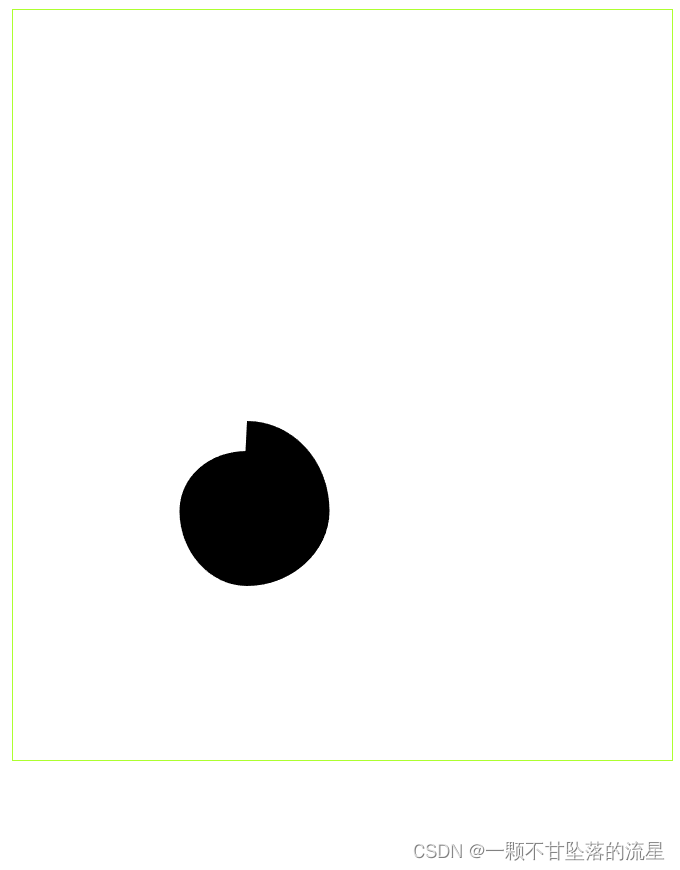