在我们日常使用 Vue 构建项目,开发工作中,不可避免的会遇上不同组件之间传值的需求,以下我会一一介绍几种传值的方式。
一、props 和 $emit 的用法:
// 父组件 v-bind绑定参数,v-on接收子组件事件反馈
<children :property="fatherProps" @sendVal="dataHandle"></children>
// 子组件 props 接收父组件参数,使用$emit 触发事件
props: ["property" ]
this.$emit("sendVal",params)
二、Event Bus(事件总线):
Event Bus 实际上是一个不具备 Dom
的Vue组件。首先我们创建一个event-bus.js
。
// event-bus.js
import Vue from 'vue'
export const EventBus = new Vue()
此外,若是我们将 EventBus 直接在 main.js
中注册,它就成了一个全局事件总线。
// main.js
Vue.prototype.$EventBus = new Vue()
执行上面的步骤了,我们就初始化了一个事件总线,下面我们如何使用它呢,很简单,若有A和B组件。
// 首先我们在A和B页面都引入event-bus.js
import { EventBus } from "/utils/event-bus.js"
// A 页面 使用event-bus $emit触发事件,传递参数(如果使用的是全局事件总线:则使用this.$EventBus,不需要上方引入event-bus.js操作)。
EventBus.$emit("sendMsg", "来自A页面的消息")
// B 使用event-bus $on 注册接收事件。
EventBus.$on("sendMsg",(msg)=>{
console.log(msg)
})
在进一步学习Vue我们知道,EventBus 主要是利用Vue 组件中 $emit
和 $on
触发、监听来传递参数,但是这个有个弊端:若 EventBus 使用在反复操作的组件上,$on
监听就会触发多次,且相关页面被刷新或者销毁,与之相关的 EventBus 就会被移除。
因此,我们可以在组件销毁前,使用 $off
去移除对应 $on
监听:
EventBus.$off("sendMsg",null)
// 或移除所有事件
EventBus.$off()
三、$refs:
在Vue中,官方并不建议直接对 Dom
对象进行操作,但是有些情况下仍然需要直接访问底层 DOM 元素。要实现这点,Vue官方给我们提供了一个特殊的 ref
attribute,它允许我们在一个特定的 DOM 元素或子组件实例被挂载后,获得对它的直接引用。
<input ref="inputRef" />
this.$refs.inputRef.focus()
若给子组件加上 ref
属性,则可以获取它的属性及方法等。
// children 子组件
data() {
return {
msg: "这是一个测试"
};
},
methods: {
logMsg(){
console.log("子组件的logMsg方法")
}
}
// 父组件中
<children ref="childrenRef" />
this.$refs.childrenRef.msg
this.$refs.childrenRef.logMsg()
四、Vuex:
关于Vuex这里就不再详细介绍,有需求的小伙伴参考 Vuex 开始。
五、$emit update 和 .sync 修饰符
最近学习到一个蛮实用的一个用法:$emit update
和 .sync
修饰符,具体用法如下。
//子组件
this.$emit("update:selectNode", params); // params,代表你传递的参数
// 父组件
<children :selectNode.sync="node" />
data() {
return {
node: {}
};
}
咋看一眼,好像没什么特殊之处,但是其实 $emit update
和 .sync
修饰符相当于帮你简化了定义 v-on
参数传递的工作。
扫描二维码关注公众号,回复:
15494303 查看本文章
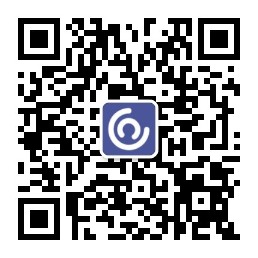
// 带 .sync 修饰符
<children :selectNode.sync="node" />
// 无 .sync 修饰符,等同于
<children :selectNode="node" @update:selectNode="node=$event" />