今天实现一个简单的注册功能:首先提到注册,就会想到数据库连接,ajax异步传输数据校验,利用mvc架构进行分工。
首先,要能连接数据库 需要数据库jar包 -----设计专门的DBUtil类
1.用户名,密码及数据库名称 加载驱动 数据库的关闭
其次,要有相应的sql语句----设计一个专门的写sql的包
然后,有相应的方法执行sql语句
1.用dao层及daoImpl层实现方法,用service层进行数据校验及异常处理 servlet进行数据的获取和跳转
所以我采取如下的分层:
数据库连接:
static{
try {
Class.forName("com.mysql.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
//连接数据的url
private final static String URL="jdbc:mysql:///test";
private final static String USERNAME="root";
private final static String PASSWORD="root";
public static Connection getConn(){
Connection conn=null;
try {
conn=DriverManager.getConnection(URL, USERNAME, PASSWORD);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return conn;
}
public static void close(Connection conn){
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
注释:因为数据库驱动加载在整个驱动中,只需要被加载一次,所以放在static静态代码块里。
方法用static修饰,这样方便之后的调用过程中不需要创建对象就可以直接用类名.方法名直接调用
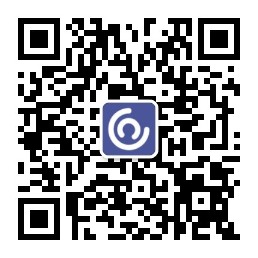
这里我只写了Connection的关闭,必要的时候可以把结果集和预处理的关闭一起写了
然后,需要有调用数据库类的方法,则先编写dao及其他的daoImpl,编写daoimpl时,我们要调用sql语句,进行插入功能,则需要对应的一个user实体类。
则代码如下:
1.dao:
public interface UserDao {
public void insertUser(User user);
}
2.user:
package com.hxl.entity;
public class User {
private String cn_user_name;
private String cn_user_password;
public User(String cn_user_name, String cn_user_password) {
super();
this.cn_user_name = cn_user_name;
this.cn_user_password = cn_user_password;
}
public User(){
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((cn_user_name == null) ? 0 : cn_user_name.hashCode());
result = prime * result + ((cn_user_password == null) ? 0 : cn_user_password.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
User other = (User) obj;
if (cn_user_name == null) {
if (other.cn_user_name != null)
return false;
} else if (!cn_user_name.equals(other.cn_user_name))
return false;
if (cn_user_password == null) {
if (other.cn_user_password != null)
return false;
} else if (!cn_user_password.equals(other.cn_user_password))
return false;
return true;
}
public String getCn_user_name() {
return cn_user_name;
}
public void setCn_user_name(String cn_user_name) {
this.cn_user_name = cn_user_name;
}
public String getCn_user_password() {
return cn_user_password;
}
public void setCn_user_password(String cn_user_password) {
this.cn_user_password = cn_user_password;
}
}
3.daoImpl:
package com.hxl.daoImpl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import com.hxl.dao.UserDao;
import com.hxl.entity.User;
import com.hxl.sql.SQLTEXT;
import com.hxl.util.DBUtil;
public class UserDaoImpl implements UserDao{
Connection conn;
public UserDaoImpl() {
this.conn=DBUtil.getConn();
}
@Override
public void insertUser(User user) {
try {
PreparedStatement pst=conn.prepareStatement(SQLTEXT.SQL_INSERT);
pst.setString(1, user.getCn_user_name());
pst.setString(2, user.getCn_user_password());
int count=pst.executeUpdate();
System.out.println(count);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
4.我们常常需要在servlet需要传递信息,所以我们干脆建一个Message实体类来装信息:
message:
package com.hxl.entity;
public class Message {
private int status;
private String message;
public Message(int status, String message) {
super();
this.status = status;
this.message = message;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
现在我们来写service及其实现类:
service:
public interface UserService {
public void inserUser(User user);
}
serviceImpl:
package com.hxl.serviceImpl;
import com.hxl.dao.UserDao;
import com.hxl.daoImpl.UserDaoImpl;
import com.hxl.entity.User;
import com.hxl.service.UserService;
public class UserServiceImpl implements UserService{
UserDao userDao;
public UserServiceImpl() {
this.userDao=new UserDaoImpl();
}
@Override
public void inserUser(User user) {
userDao.insertUser(user);
}
}
来到最后的servlet层
servlet:
package com.hxl.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.hxl.entity.Message;
import com.hxl.entity.User;
import com.hxl.service.UserService;
import com.hxl.serviceImpl.UserServiceImpl;
import net.sf.json.JSONObject;
/**
* Servlet implementation class RegisterServlet
*/
@WebServlet("/RegisterServlet")
public class RegisterServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public RegisterServlet() {
super();
// TODO Auto-generated constructor stub
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doPost(request,response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
PrintWriter pw=response.getWriter();
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
response.setContentType("text/html;charset=utf-8");
String cn_user_name=request.getParameter("cn_user_name");
String cn_user_password=request.getParameter("cn_user_password");
System.out.println(cn_user_name);
Message msg=new Message(0, "注册成功");
User user;
UserService userService;
JSONObject jsob=JSONObject.fromObject(msg);
if(msg.getStatus()==0){
user=new User(cn_user_name, cn_user_password);
userService=new UserServiceImpl();
userService.inserUser(user);
}
pw.println(jsob);
System.out.println(msg.getMessage());
}
}
里面包含一些测试的打印数据
下面我们来写js:
register.js
$(function(){$("#cn_user_name").blur(userNameCheck);
$("#cn_user_password1").blur(pw1);
$("#cn_user_password2").blur(pw2);
$("#ss").click(submit);
$("#cn_user_name").focus(function(){
$("#nameWarning").html("");
});
$("#cn_user_password1").focus(function(){
$("#pw1Warning").html("");
});
$("#cn_user_password2").focus(function(){
$("#pw2Warning").html("");
});
});
function userNameCheck(){
var cn_user_name=$("#cn_user_name").val();
if(cn_user_name==""){
$("#nameWarning").html("用户名为空");
}
}
function pw1(){
var cn_user_password1=$("#cn_user_password1").val();
var cn_user_password2=$("#cn_user_password2").val();
if(cn_user_password1==""){
$("#pw1Warning").html("密码不能为空");
}
}
function pw2(){
$("#pw1Warning").html("");
var cn_user_password2=$("#cn_user_password2").val();
var cn_user_password1=$("#cn_user_password1").val();
if(cn_user_password2==""){
$("#pw2Warning").html("密码不能为空");
}
if(cn_user_password2!=cn_user_password1){
$("#pw2Warning").html("俩次密码不一致");
}
}
function submit(){
var cn_user_name=$("#cn_user_name").val();
var cn_user_password=$("#cn_user_password1").val();
$.ajax({
url:"RegisterServlet",
method:"post",
dataType:"json",
data:{"cn_user_name":cn_user_name,
"cn_user_password":cn_user_password},
success:function(r){
alert("ok");
}
});
}
注意:我们这里用到$符号就必须要导入jquery.js
在servlet中我们把message对象转化为json对象,所以我们这需要导入一些包,,这些包可以在网上搜索下载
在主页上这样编辑:
<from>
<h1 style="text-align:center">注册用户</h1>
输入用户名:<input id="cn_user_name" name="cn_user_name" type="text"/><span id="nameWarning"></span><br>
输入密码:<input id="cn_user_password1" name="cn_user_password1" type="password"/><span id="pw1Warning"></span> <br>
重新输入密码:<input id="cn_user_password2" name="cn_user_password2" type="password"/><span id="pw2Warning"></span> <br>
<input type="submit" value="确认注册" id="ss"/>
<span id="success"></span>
</from>