题目要求
支付宝AliPay和余额宝AliFund是一对好兄弟,他们来自同一个父类Account。
已知类Account是支付宝AliPay和余额宝AliFund的虚基类,包括两个protected成员数据:
long ID;//账号
string name;//用户名
支付宝AliPay是类Account的保护派生类,包括两个新增protected成员数据:
double amount;//支付宝账户中的金额
int out-limit;//支付宝转账上限,资金转出不得超出账户中金额也不能超出上限
//当转账要求超出上述限制时,自动转出最大允许金额
余额宝AliFund是类Account的保护派生类,包括三个新增protected成员数据:
double amount;//余额宝账户中的金额
double rate;//余额宝账户中资金年利率
int in-limit;//余额宝转账下限,资金转入余额宝时不得少于该下限,上不封顶
//当转入资金不足下限金额时,自动忽略该资金转入操作,资金退回
为了实现支付宝账户和余额宝账户间的资金收集和转账等功能,现以AliPay和AliFund为基类,创建一个淘宝账户类My_Ali,包括以下新增数据成员:
bool auto_collect_flag;//资金自动收集标志
int threshold;//资金自动收集阈值
/**/若资金自动收集标志为true,当用户支付宝账户金额超过资金自动收集阈值threshold时,超过且符合支付宝账户资金转出限制和余额宝资金转入下限的部分资金将自动转入余额宝中*/*/
增加合适的成员函数,可以实现支付宝账户和余额宝账户之间的资金转账和支付宝账户向余额宝账户的资金自动收集功能。
生成以上各类并编写主函数,根据输入的初始账户信息,建立用户对象,根据用户的账户操作信息,输出用户最后的资金信息。
测试输入包含一个测试用例:
第一行为初始账户信息,第一个数据是账号,第二个字符串是用户名,第三个数据是支付宝初始金额,第四个数据是支付宝转账上限,第五个数据是余额宝初始金额,第六个数据是余额宝资金年利率,第七个数据是余额宝转账下限,第八个数据是资金自动收集标志,字符Y代表设置资金自动收集,字符N代表不设置资金自动收集。若设置资金自动收集,第九个数据是资金自动收集阈值,否则,第九个数据不存在。
随后每一行代表用户资金账户的一次操作,第一个数字代表当前操作的账户类型,1代表支付宝账户,2代表余额宝账户,第二个数字代表具体操作,1代表账户资金增加,2代表账户金额减少,3代表支付宝和余额宝之间的资金转移,第三个数字代表操作金额。
最后输入0 0 0时结束。
注意:当设置资金自动收集为真时,支付宝账户资金每次增加时会自动触发资金自动收集。
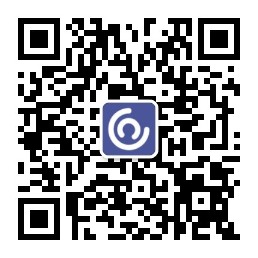
输入样例:
100001 Zhangsan 1000 30000 50000 0.047 5000 Y 3000
1 1 1500
1 2 500
1 3 500
1 1 7000
2 3 500
0 0 0
输出样例:
Account for Zhangsan
Total: 59000
AliPay: 3500
AliFund: 55500 with rate 4.7%
解题思路
-
定义账户类Account作为支付宝AliPay和余额宝AliFund的虚基类,并定义ID和name两个protected成员数据。
-
支付宝AliPay是保护派生类,包括新增amount和out-limit两个protected成员数据,还有add和sub两个方法实现了账户金额的增加和减少操作,其中sub方法自动处理账户金额不足或转账超限的状况。
-
余额宝AliFund也是保护派生类,包括新增amount, rate和in-limit三个protected成员数据,并定义add和sub方法实现了账户金额的增加和减少操作,其中add方法通过判断是否满足转账下限来决定是否添加账户金额。
-
My_Ali是以AliPay和AliFund为基类的公有派生类,也是最终的用户账户类,增加了auto_collect_flag和threshold两个数据成员,并且定义了payadd,fundadd,paysub,fundsub,paytofund,fundtopay和autodo方法,分别实现了支付宝账户向余额宝账户的资金转移,余额宝账户向支付宝账户的资金转移,支付宝账户的金额增加和减少,余额宝账户的金额增加和减少,还有自动收集资金的功能。
其中,paytofund方法通过调用AliPay类的sub方法和AliFund类的add方法实现支付宝资金向余额宝转移的操作,并且判断是否满足转账下限条件;fundtopay方法通过调用AliFund类的sub方法和AliPay类的add方法实现余额宝资金向支付宝转移的操作,并且在转移完成后调用autodo方法检测是否需要自动收集资金;autodo方法则是在支付宝账户金额超过阈值时自动将合适的资金转移到余额宝账户中。 -
主函数根据输入的账户信息建立用户对象,并依次处理用户操作,通过调用My_Ali类的相关方法来实现对支付宝账户和余额宝账户的操作,并最终输出账户信息。
代码
#include<iostream>
#include<iomanip>
using namespace std;
// 账户类,作为支付宝AliPay和余额宝AliFund的虚基类
class Account {
protected:
long ID; // 账号
string name; // 用户名
Account() {
cin >> ID >> name;
}
};
// 支付宝类,是Account类的保护派生类
class AliPay : virtual protected Account {
protected:
double amount; // 支付宝账户中的金额
int out_limit; // 支付宝单次转账上限,单次资金转出不得超出账户中金额也不能超出上限
// 当转账要求超出上述限制时,自动转出最大允许金额
// 支付宝账户金额增加操作
void add(double& pay);
// 支付宝账户金额减少操作
void sub(double& pay);
public:
AliPay() {
cin >> amount >> out_limit;
}
};
void AliPay::add(double& pay) {
amount += pay;
}
void AliPay::sub(double& pay) {
if (pay > amount)
pay = amount;
if (pay > out_limit)
pay = out_limit;
amount -= pay;
}
// 余额宝类,是Account类的保护派生类
class AliFund : virtual protected Account {
protected:
double amount; // 余额宝账户中的金额
double rate; // 余额宝账户中资金年利率
int in_limit; // 余额宝单次转账下限,单次资金转入余额宝时不得少于该下限,上不封顶
// 当转入资金不足下限金额时,自动忽略该资金转入操作,资金退回
// 余额宝账户金额增加操作
void add(double& pay);
// 余额宝账户金额减少操作
void sub(double& pay);
public:
AliFund() {
cin >> amount >> rate >> in_limit;
}
};
void AliFund::add(double& pay) {
if (pay >= in_limit)
amount += pay;
}
void AliFund::sub(double& pay) {
if (pay > amount)
pay = amount;
amount -= pay;
}
// 淘宝账户类,是以AliPay和AliFund为基类的公有派生类,实现了自动收集资金的功能
class My_Ali : public AliPay, public AliFund {
private:
bool auto_collect_flag; // 资金自动收集标志
int threshold; // 资金自动收集阈值
// 自动收集资金操作
void autodo();
public:
My_Ali() {
char t;
cin >> t;
if (t == 'Y') {
cin >> threshold;
auto_collect_flag = 1;
} else {
auto_collect_flag = 0;
}
}
// 支付宝账户金额增加操作
void payadd(double& pay) {
AliPay::add(pay);
autodo();
}
// 余额宝账户金额增加操作
void fundadd(double& pay) {
AliFund::add(pay);
}
// 支付宝账户金额减少操作
void paysub(double& pay) {
AliPay::sub(pay);
}
// 余额宝账户金额减少操作
void fundsub(double& pay) {
AliFund::sub(pay);
}
// 支付宝向余额宝转移资金操作
void paytofund(double& pay);
// 余额宝向支付宝转移资金操作
void fundtopay(double& pay);
// 输出账户信息操作
void out();
};
// My_Ali 类中的 paytofund 函数,将资金从支付宝账户转移至余额宝账户或者加回支付宝账户
void My_Ali::paytofund(double& pay) {
// 调用 AliPay 类中的 sub 函数扣除指定金额的资金
AliPay::sub(pay);
// 判断扣除的金额是否达到了余额宝转入下限
if (pay >= AliFund::in_limit) {
// 如果是,则调用 AliFund 类的 add 函数将资金转移到余额宝账户
AliFund::add(pay);
} else {
// 如果不是,则将该金额加回支付宝账户中,调用 AliPay 类的 add 函数
AliPay::add(pay);
}
}
// My_Ali 类中的 fundtopay 函数,将资金从余额宝账户转移至支付宝账户
void My_Ali::fundtopay(double& pay) {
// 调用 AliFund 类的 sub 函数扣除指定金额的资金
AliFund::sub(pay);
// 将剩余金额加回支付宝账户中,调用 AliPay 类的 add 函数
AliPay::add(pay);
// 自动收集超过阈值的资金
autodo();
}
// My_Ali 类中的 autodo 函数,实现自动收集资金的功能
void My_Ali::autodo() {
// 计算超出阈值的金额
double t = AliPay::amount - threshold;
// 判断是否开启了自动收集标志并且支付宝账户中的金额是否大于阈值
if (auto_collect_flag && AliPay::amount > threshold) {
// 如果是,则调用 paytofund 函数将超出阈值的资金转移至余额宝账户
paytofund(t);
}
}
// My_Ali 类中的 out 函数,将账户信息输出到屏幕上
void My_Ali::out() {
cout << "Account for " << name << endl << "Total: " << AliFund::amount + AliPay::amount << endl;
cout << "AliPay: " << AliPay::amount << endl << "AliFund: " << AliFund::amount << " with rate " << setiosflags(ios::fixed)
<< setprecision(1) << rate * 100 << "%" << endl;
}
// 主函数
int main() {
// 提示用户输入 ID 和姓名
cout << "Please input your ID and name: ";
// 创建 My_Ali 对象
My_Ali ali;
// 定义变量及对象
int m;
double pay;
char cmd;
// 提示用户输入指令
cout << "Please input command (pay/add/sub/collect/transfer/getout):" << endl;
// 循环读取用户输入的指令
while (cin >> cmd) {
switch (cmd) {
case 'p':
// 用户想要从支付宝账户中扣除资金
cout << "Please input the amount of money you want to pay: ";
cin >> pay;
ali.paysub(pay);
break;
case 'a':
// 用户想要往支付宝账户中添加资金
cout << "Please input the amount of money you want to add: ";
cin >> pay;
ali.payadd(pay);
break;
case 's':
// 用户想要从支付宝账户中减去资金
cout << "Please input the amount of money you want to subtract: ";
cin >> pay;
ali.paysub(pay);
break;
case 'c':
// 用户想要开启自动收集标志并设置阈值
cout << "Auto collect? (Y/N): ";
cin >> cmd;
if (cmd == 'Y') {
cout << "Please input the threshold value: ";
cin >> m;
}
break;
case 't':
// 用户想要将资金从支付宝账户转移到余额宝账户或从余额宝账户转移到支付宝账户
cout << "Transfer from (pay/fund) to (pay/fund): ";
cin >> cmd;
switch (cmd) {
case 'p':
// 将资金从支付宝账户转移到余额宝账户
cout << "Please input the amount of money you want to transfer from AliPay to AliFund: ";
cin >> pay;
ali.paytofund(pay);
break;
case 'f':
// 将资金从余额宝账户转移到支付宝账户
cout << "Please input the amount of money you want to transfer from AliFund to AliPay: ";
cin >> pay;
ali.fundtopay(pay);
break;
}
break;
case 'g':
// 用户想要获取账户总金额、支付宝账户金额、余额宝账户金额以及余额宝账户的年利率等信息
ali.out();
break;
default:
// 用户输入了无效指令
cout << "Invalid command!" << endl;
break;
}
// 提示用户输入下一个指令
cout << "Please input command (pay/add/sub/collect/transfer/getout):" << endl;
}
return 0;
}
总结
该题主要考察面向对象编程的基本概念和应用。
包括类的定义、成员变量和成员函数的访问控制、构造函数和析构函数的实现、类的继承和派生、虚函数的使用等。此外,还涉及到输入输出流的操作,以及简单的条件判断和循环控制语句的应用。
我是秋说,我们下次见。