kivy
Kivy 是一个开源的 Python 框架(2011年),用于快速开发应用,实现各种当前流行的用户界面,比如多点 触摸等等。 Kivy 可以运行于 Windows, Linux, MacOS, Android, iOS 等当前绝大部分主流桌面/移 动端操作系统。 Kivy 基于 Python,界面UI文件和程序文件相互分离的设计思路,设计简洁优雅,语法易学,适合新 人入门。 目前 Kivy 的官方文档还算很完善。
第一个应用
main.py:
from kivy.app import App
from kivy.uix.widget import Widget
from kivy.properties import (
NumericProperty, ReferenceListProperty, ObjectProperty
)
from kivy.vector import Vector
from kivy.clock import Clock
class PongPaddle(Widget):
score = NumericProperty(0)
def bounce_ball(self, ball):
if self.collide_widget(ball):
vx, vy = ball.velocity
offset = (ball.center_y - self.center_y) / (self.height / 2)
bounced = Vector(-1 * vx, vy)
vel = bounced * 1.1
ball.velocity = vel.x, vel.y + offset
class PongBall(Widget):
velocity_x = NumericProperty(0)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
def move(self):
self.pos = Vector(*self.velocity) + self.pos
class PongGame(Widget):
ball = ObjectProperty(None)
player1 = ObjectProperty(None)
player2 = ObjectProperty(None)
def serve_ball(self, vel=(4, 0)):
self.ball.center = self.center
self.ball.velocity = vel
def update(self, dt):
self.ball.move()
# bounce of paddles
self.player1.bounce_ball(self.ball)
self.player2.bounce_ball(self.ball)
# bounce ball off bottom or top
if (self.ball.y < self.y) or (self.ball.top > self.top):
self.ball.velocity_y *= -1
# went of to a side to score point?
if self.ball.x < self.x:
self.player2.score += 1
self.serve_ball(vel=(4, 0))
if self.ball.right > self.width:
self.player1.score += 1
self.serve_ball(vel=(-4, 0))
def on_touch_move(self, touch):
if touch.x < self.width / 3:
self.player1.center_y = touch.y
if touch.x > self.width - self.width / 3:
self.player2.center_y = touch.y
class PongApp(App):
def build(self):
game = PongGame()
game.serve_ball()
Clock.schedule_interval(game.update, 1.0 / 60.0)
return game
if __name__ == '__main__':
PongApp().run()
pong.kv:
#:kivy 1.0.9
<PongBall>:
size: 50, 50
canvas:
Ellipse:
pos: self.pos
size: self.size
<PongPaddle>:
size: 25, 200
canvas:
Rectangle:
pos: self.pos
size: self.size
<PongGame>:
ball: pong_ball
player1: player_left
player2: player_right
canvas:
Rectangle:
pos: self.center_x - 5, 0
size: 10, self.height
Label:
font_size: 70
center_x: root.width / 4
top: root.top - 50
text: str(root.player1.score)
Label:
font_size: 70
center_x: root.width * 3 / 4
top: root.top - 50
text: str(root.player2.score)
PongBall:
id: pong_ball
center: self.parent.center
PongPaddle:
id: player_left
x: root.x
center_y: root.center_y
PongPaddle:
id: player_right
x: root.width - self.width
center_y: root.center_y
打包apk文件
python+buildozer+kivy打包apk文件 - 简书
GitHub - kivy/python-for-android: Turn your Python application into an Android APK
beeware
用Python编写,无处不在运行。
BeeWare是一套工具和库的集合,每个工具和库都可以协同工作,帮助您编写跨平台本地GUI Python应用程序。它包括:
- Toga,一个跨平台的小部件工具包;
- Briefcase,一个将Python项目打包为可分发的工件并可发送给最终用户的工具;
- 库(如Rubicon ObjC)用于访问平台本地库;
- 预编译的Python版本,可用于官方Python安装程序不可用的平台。
您可以单独使用每个工具,也可以将它们全部用作一套工具。
完整的BeeWare套件还包括使用BeeWare自己的库编写的软件开发工具和应用程序。
BeeWare套件可用于macOS、Windows、Linux(使用GTK)、Android和iOS等移动平台,以及Web。我们的长期路线图中还支持其他平台(如机顶盒和手表)。
扫描二维码关注公众号,回复:
15437140 查看本文章
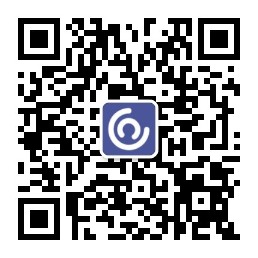
参考资料:
https://blog.csdn.net/ZNJIAYOUYA/article/details/126553693