Java---第三章
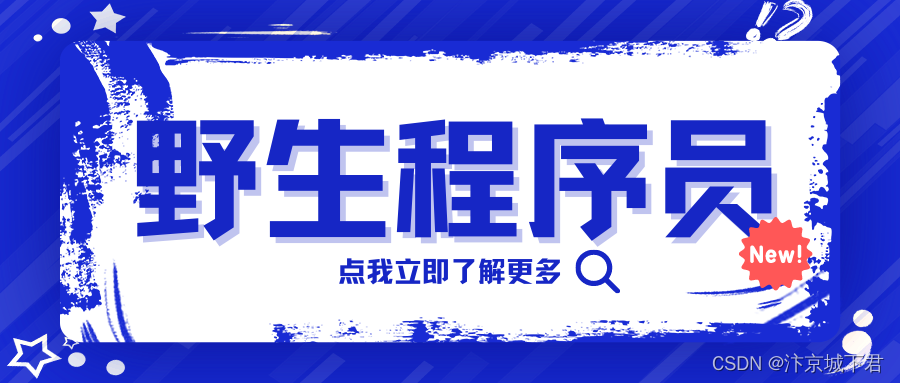
一 流程图
流程图概念:
流程图就是使用统一的标准图形来描述程序的过程
为什么要使用流程图?
流程图简单直观,可以很方便的为程序员提供编写代码的思路
图形规则:
示例:
二 选择结构
简单的if-else
格式:
if (条件)如果条件满足,则执行代码块
{
代码块
}
else 否则执行代码块2
{
代码块2
}
示例:
从控制台输入一个整数,若是偶数,就输出偶数,若是奇数,就输出奇数
代码实现:
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner myScanner = new Scanner(System.in);
int a = myScanner.nextInt();
if (a % 2 == 0)
{
System.out.println("偶数");
}
else
{
System.out.println("奇数");
}
}
}
利用三元运算符
System.out.println((a % 2 == 0) ? "偶数" : "奇数");
嵌套的if-else
例题1:
在半决赛中,若胜利了就进入决赛,若为男,就进入男子决赛,否则进入女子决赛。
若失败了就退出比赛
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入是否胜利:Y/N");
String win = sc.next();
if ("Y".equals(win)) {
System.out.println("请输入性别:");
String sex = sc.next();
if("男".equals(sex)) {
System.out.println("进入男子比赛");
} else {
System.out.println("进入女子比赛");
}
} else {
System.out.println("被淘汰");
}
}
}
判断字符串和变量是否相等:.equals()
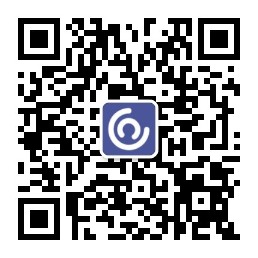
例题2:
从控制台输入一个整数,若该数<10,则该数乘3再加5,输出最后运算过的数是偶数1还是奇数1,否则直接输出该数是偶数2是奇数2
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int num = sc.nextInt();
if(num < 10){
int num1 = num * 3 +5;
if(num1 % 2 == 0){
System.out.println("偶数1");
}else{
System.out.println("奇数1");
}
}else {
if(num % 2 == 0){
System.out.println("偶数2");
}else{
System.out.println("奇数2");
}
}
}
}
多重if的选择语句(else-if)
例题1:
小明去买彩票,如果中了一等奖,可领取500W,如果中了二等奖,可领取300W,如果中了三等奖,可领取100W,否则没有奖励
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入中奖等级:");
int dt = sc.nextInt();
if(dt == 1){
System.out.println("一等奖!!!500W");
}else if(dt == 2){
System.out.println("二等奖!!!300W");
} else if (dt == 3) {
System.out.println("三等奖!!!100W");
}else {
System.out.println("没中奖!");
}
}
}
例题2:
从控制台输入该学生的分数,若分数在90到100,输出优秀;在80到90,输出良好;在60到80,输出中等;低于60的,输出差生。
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入该学生分数:");
int score = sc.nextInt();
if(score >= 90){
System.out.println("优秀");
}else if(score >= 80){
System.out.println("良好");
} else if (score >= 60) {
System.out.println("中等");
}else {
System.out.println("差生");
}
}
}
switch语句
switch表示开关的意思
支持的数据类型
byte
short
int
char
String
Enum(枚举)
String类型从JDK1.7开始才开始支持
案例1:
某公司在年终决定对研发部工作人员根据级别进行调薪,具体如下:
- 1级 +500
- 2级 +800
- 3级 +1200
- 4级 +2000
请从控制台输入该员工当前薪水和级别,输出调薪之后的薪水
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入该员工当前薪资:");
double sal = sc.nextDouble();
System.out.println("请输入该员工当前级别:");
int devel = sc.nextInt();
switch (devel){
case 1:
sal+= 500;
break;
case 2:
sal+= 800;
break;
case 3:
sal+= 1200;
break;
case 4:
sal+= 2000;
break;
}
System.out.println("调薪后的薪资为:" + sal);
}
}
案例2:
一年有12个月,4个季节。1,2,3为春季;4,5,6为夏季;7,8,9为秋季;10,11,12为冬季。
要求:从控制台输入月份,输出对应的季节
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入月份:");
int month = sc.nextInt();
switch (month){
case 1:
case 2:
case 3:
System.out.println("春季");
break;
case 4:
case 5:
case 6:
System.out.println("夏季");
break;
case 7:
case 8:
case 9:
System.out.println("秋季");
break;
case 10:
case 11:
case 12:
System.out.println("冬季");
break;
}
}
}
更巧妙的方法
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入月份:");
int month = sc.nextInt();
switch ((month-1)/3){
case 0:
System.out.println("春季");
break;
case 1:
System.out.println("夏季");
break;
case 2:
System.out.println("秋季");
break;
case 3:
System.out.println("冬季");
break;
}
}
}
二 输入验证
要求用户输入一个整数,但是用户输入一个字符串,该怎么办呢?
.hasNextInt()—>校验Scanner中是否存储着下一个整数,如果没有数据,让用户输入;用户输入后再判断是否为整数
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个整数:");
if(sc.hasNextInt()){
int a = sc.nextInt();
System.out.println(a);
}else{
System.out.println("别瞎输入");
}
}
}
运行举例:
请输入一个整数:
as
别瞎输入
三 程序调试
概念:
当程序出现问题时,我们希望程序可以暂停下来,然后我们去操作代码逐行执行,观察整个过程中,变量的变化是否是按照我们所设计的程序思维变化,从而找到问题并解决问题,这个过程称为调试
为什么要进行调试:
人无完人,考虑问题不可能面面俱到,尤其是在写复杂的程序时候,如果程序运行出了问题,定位问题就是关键,要定位就需要进行调试
如何进行调试:
- 在有效的代码上下断点
- DEBUG模式启动程序
- 程序启动后,通过操作程序使其进行逐行执行,观察其变量的值的变化,从而找出问题,并解决
四 循环结构
**循环:**同样的事情反复多次做
循环三要素:
- 定义循环变量并赋初值
- 循环条件
- 循环变量的更新
while循环
语法:
while(循环条件){
//循环操作
}
特点:
先判断,后执行。如果一开始条件就不满足,那么while循环可能一次也不执行
案例1:
打印0~9所有的数字
public class study {
public static void main(String[] args) {
int i = 0;
while(i < 10){
System.out.println(i);
i++;
}
}
}
案例2:
输出100~1000之内的水仙花数。水仙花数:比如153=1的三次方+5的三次方+3的三次方。
public class study {
public static void main(String[] args) {
int i = 100;
while(i <= 1000){
int a = i / 100;
int b = i % 100 / 10;
int c = i % 10;
if(a*a*a+b*b*b+c*c*c==i){
System.out.println(i);
}
i++;
}
}
}
do-while循环
语法:
do{
循环操作
}while(循环条件);
案例1:
从控制台录入学生的成绩并计算总成绩,输入0的时候退出
import java.util.Scanner;
public class study {
public static void main(String[] args) {
double score = 0;
double sum = 0;
do{
System.out.println("请输入学生成绩:");
Scanner sc = new Scanner(System.in);
score = sc.nextDouble();
sum += score;
}while(score != 0);
System.out.println("总成绩为:"+sum);
}
}
案例2:
从控制台输入一个数字,如果该数字不能被7整除,则重新输入,直到该数字能被7整除为止
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int i = 0;
do{
System.out.println("请输入一个整数:");
i = sc.nextInt();
}while(i%7!=0);
}
}
for循环
语法:
for(定义循环变量;循环条件;循环变量的更新){
循环操作
}
特点:
for循环是先判断,后执行;如果一开始就不符合条件,那么for循环可能一次都不执行;循环次数确定的情况下一般使用for循环;循环次数不确定的情况下,通常使用while或者do-while循环。
案例1:
求1~10的累加和
public class study {
public static void main(String[] args) {
int sum = 0;
for(int i = 1; i <= 10; i++){
sum+=i;
}
System.out.println("累加和为:"+sum);
}
}
i 的作用范围仅限于for循环结构
案例2:
求6的阶乘(6!)
public class study {
public static void main(String[] args) {
int sum = 1;
for(int i = 1; i <= 6; i++){
sum*=i;
}
System.out.println("6!:"+sum);
}
}
流程控制
break;
break只能应用于for循环,while循环,do-while循环和switch语句
作用:
- break应用在循环结构中,表示终止break所在的循环,执行循环结构下面的代码,通常与if语句配合使用
- break应用在switch语句中,表示终止在break所在的选择语句
案例1:(猜数字小游戏)
获取一个10以内的随机数,然后从控制台输入一个整数,如果输入的整数与随机数不相等,则重新输入;直到输入的整数与随机整数相等为止
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double ran = Math.random();
int number = (int)(ran*10);
int input;
do{
System.out.println("请输入一个整数:");
input = sc.nextInt();
if(input > number){
System.out.println("大了");
}else if(input < number){
System.out.println("小了");
}else{
System.out.println("猜对了");
break;
}
}while(true);
}
}
案例2:
判断一个数是否为素数。(素数:只能被1和自身整除)
二重循环(重点)
案例1:
输出九九乘法表
public class study {
public static void main(String[] args) {
for(int i = 1;i < 10; i++){
//打印九行
for(int j = 1;j <= i;j++){
//j<=i表示列数的最大值就是行号
//print表示在同一行打印,也就是不会换行
System.out.print(j+"×"+i+"="+(i*j)+"\t");
}
System.out.print("\n");
}
}
}
运行结果:
1×1=1
1×2=2 2×2=4
1×3=3 2×3=6 3×3=9
1×4=4 2×4=8 3×4=12 4×4=16
1×5=5 2×5=10 3×5=15 4×5=20 5×5=25
1×6=6 2×6=12 3×6=18 4×6=24 5×6=30 6×6=36
1×7=7 2×7=14 3×7=21 4×7=28 5×7=35 6×7=42 7×7=49
1×8=8 2×8=16 3×8=24 4×8=32 5×8=40 6×8=48 7×8=56 8×8=64
1×9=9 2×9=18 3×9=27 4×9=36 5×9=45 6×9=54 7×9=63 8×9=72 9×9=81
案例2:
打印:
☆☆☆☆☆☆☆☆☆☆
☆☆☆☆☆☆☆☆☆☆
☆☆☆☆☆☆☆☆☆☆
☆☆☆☆☆☆☆☆☆☆
public class study {
public static void main(String[] args) {
for(int i = 1;i <=4; i++){
for(int j = 1;j <=10;j++){
System.out.print("☆");
}
System.out.print("\n");
}
}
}
案例3:
打印:
××××××××××
×aaaaaaaa×
×aaaaaaaa×
××××××××××
public class study {
public static void main(String[] args) {
for(int i = 1;i <=4; i++){
for(int j = 1;j <=10;j++){
if(i==1 || j==1 || i==4 || j==10){
System.out.print("×");
}else{
System.out.print("a");
}
}
System.out.print("\n");
}
}
}
案例4:
打印三角形
对 5 分析:
- 三角形一共5行
- 三角形的第一行和三角形的行数一样
- 三角形的每一行的空白数=三角形的总行数-列数
- 三角形的每一行“x”的数量=(行号-1)*2+1
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入:");
int co = sc.nextInt();
for(int i = 1;i <= co;i++){
int space = co - i;
for(int j = 1;j <= space;j++){
System.out.print(" ");
}
int sp = (i - 1) * 2 + 1;
for(int m = 1;m <= sp;m++){
System.out.print("x");
}
System.out.println();
}
}
}
案例5:
打印倒三角形:
分析:
- 三角形一共4行
- 三角形的空白数=总行数-i
- 三角形的“x”数=(总行数-行号)*2+1
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入:");
int co = sc.nextInt();
for(int i = 1;i <= co;i++){
int space = i - 1;
for(int j = 1;j <= space;j++){
System.out.print(" ");
}
int sp = (co - i) * 2 + 1;
for(int m = 1;m <= sp;m++){
System.out.print("x");
}
System.out.println();
}
}
}
案例6:
打印菱形
分析:
- 总行数一定为奇数
- 上三角包括对称轴
- 上三角就是其上面的上三角讲解
- 下三角就是其上面的下三角讲解
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入(奇数):");
int all_co = sc.nextInt(); //总行数
//上三角
int top_co = all_co / 2 + 1;
for(int i = 1;i <= top_co;i++){
int space = top_co - i;
for(int j = 1;j <= space;j++){
System.out.print(" ");
}
int sp = (i - 1) * 2 + 1;
for(int m = 1;m <= sp;m++){
System.out.print("x");
}
System.out.println();
}
//下三角
int down_co = all_co - top_co;
for(int i = 1;i <= down_co;i++){
int space = i;
for(int j = 1;j <= space;j++){
System.out.print(" ");
}
int sp = (down_co - i) * 2 + 1;
for(int m = 1;m <= sp;m++){
System.out.print("x");
}
System.out.println();
}
}
}
案例7:
输出2~100之内的所有质数。(质数:只能被1和本身整除)
public class study {
public static void main(String[] args) {
for(int i = 2;i<=100;i++){
if(i==2) {
System.out.println(i+"是质数");
}else {
boolean isPrime = true;
for(int j = 2;j*j<i;j++){
if(i%j==0){
isPrime = false;
break;
}
}
if(isPrime) {
System.out.println(i + "是质数");
}
}
}
}
}
五 标签 label(标号)
- 语法结构
标号名称:循环结构
- 作用
给代码添加一个标记,方面以后的使用。通常用在循环结构中,与break语句配合使用
- 应用场景
import java.util.Scanner;
public class study {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
max_menu:while (true) {
System.out.println("=======================");
System.out.println("1.学生成绩管理");
System.out.println("2.学生选课管理");
System.out.println("3.退出系统");
System.out.println("=======================");
System.out.println("请选择菜单编号:");
int menuNo = sc.nextInt();
if (menuNo == 1) {
two_menu:while(true) {
System.out.println("----------------------");
System.out.println("1.添加成绩");
System.out.println("5.返回主菜单");
System.out.println("----------------------");
System.out.println("请选择编号:");
int menu2 = sc.nextInt();
switch (menu2){
case 1:
System.out.println("选择了添加成绩");
break;
case 5:
break two_menu;
}
}
}else if (menuNo == 2) {
} else {
System.out.println("退出咯");
break;
}
}
}
}
解释:
two_menu:while (true)
该while循环标号为max_menu
break two_menu;
跳出整个 two_menu对应的while循环