努力了那么多年,回头一望,几乎全是漫长的挫折和煎熬。对于大多数人的一生来说,顺风顺水只是偶尔,挫折、不堪、焦虑和迷茫才是主旋律。我们登上并非我们所选择的舞台,演出并非我们所选择的剧本。继续加油吧!
目录
1、两数之和
题目链接:两数之和_牛客题霸_牛客网
思路:用hashmap存储,O(n)的时间复杂度。
Java版:
import java.util.*;
public class Solution {
/**
*
* @param numbers int整型一维数组
* @param target int整型
* @return int整型一维数组
*/
public int[] twoSum (int[] numbers, int target) {
// write code here
HashMap<Integer, Integer> map = new HashMap<>() ;
for(int i=0; i<numbers.length; i++){
if(map.containsKey(target - numbers[i])){
return new int [] {map.get(target-numbers[i])+1, i+1} ;
}
map.put(numbers[i], i) ;
}
return new int []{} ;
}
}
2、数组中出现次数超过一半的数字
题目链接:数组中出现次数超过一半的数字_牛客题霸_牛客网
思路:Map存储数字出现的次数,找到出现次数超出一半的。
Java版:
扫描二维码关注公众号,回复:
15435257 查看本文章
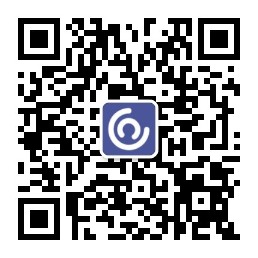
import java.util.* ;
public class Solution {
public int MoreThanHalfNum_Solution(int [] array) {
Map<Integer, Integer> map = new HashMap<>() ;
int i = 0 ;
for(; i<array.length; i++){
map.put(array[i],map.getOrDefault(array[i], 0)+1) ;
if(map.get(array[i]) > array.length / 2){
break ;
}
}
return array[i] ;
}
}
3、 数组中只出现一次的两个数字
思路:用hashmap存储,出现啊依次存入,出现2次删除。
Java版:
import java.util.*;
public class Solution {
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param array int整型一维数组
* @return int整型一维数组
*/
public int[] FindNumsAppearOnce (int[] array) {
// write code here
Map<Integer, Object> map = new HashMap<>() ;
for(int i=0; i<array.length; i++){
if(map.containsKey(array[i])){
map.remove(array[i], null) ;
}else{
map.put(array[i], null) ;
}
}
int [] ans = new int [map.size()] ;
int i = 0 ;
for(Integer res : map.keySet()){
ans[i++] = res ;
}
return ans ;
}
}
4、缺失的第一个正整数
题目链接:缺失的第一个正整数_牛客题霸_牛客网
思路:保证O(n)的时间复杂度,用map存储,然后从1开始判断是否存在map中。
Java版:
import java.util.*;
public class Solution {
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param nums int整型一维数组
* @return int整型
*/
public int minNumberDisappeared (int[] nums) {
// write code here
Map<Integer, Integer> map = new HashMap<>() ;
for(int i=0; i<nums.length; i++){
map.put(nums[i], 1);
}
int j = 1 ;
for(j=1; ; j++){
if(!map.containsKey(j)){
break ;
}
}
return j ;
}
}
5、三数之和
题目链接:三数之和_牛客题霸_牛客网
思路:暴力法,三层循环,时间复杂度O(n3).
Java版:
import java.util.*;
public class Solution {
public ArrayList<ArrayList<Integer>> threeSum(int[] num) {
ArrayList<ArrayList<Integer>> list = new ArrayList<>() ;
Arrays.sort(num) ;
for(int i=0; i<num.length; i++){
for(int j=i+1; j<num.length; j++){
for(int k=j+1; k<num.length; k++){
ArrayList<Integer> lst = new ArrayList<>() ;
lst.add(num[i]) ;
lst.add(num[j]) ;
lst.add(num[k]) ;
if(num[i] + num[j] + num[k] == 0 && !list.contains(lst)){
list.add(lst) ;
}
}
}
}
return list ;
}
}
思路2:双指针法,可以降低时间复杂度为O(n2),不过指针都有在右边,测试用例比较狗血。
import java.util.*;
public class Solution {
public ArrayList<ArrayList<Integer>> threeSum(int[] num) {
ArrayList<ArrayList<Integer>> list = new ArrayList<>() ;
Arrays.sort(num) ;
//使用双指针,固定中间的,左右两侧移动,可以降低时间复杂度
if(num.length < 3){
return list ;
}
for(int i=0; i<num.length; i++){
if(num[i] > 0){
return list ;
}
int left = i+1, right = num.length - 1 ;
while(left > i && i < right && left < right){
ArrayList<Integer> lst = new ArrayList<>() ;
if(num[left] + num[right] + num[i] == 0){
lst.add(num[i]) ;
lst.add(num[left]) ;
lst.add(num[right]) ;
if(!list.contains(lst)){
list.add(lst) ;
}
left ++ ;
right -- ;
}else if(num[left] + num[right] + num[i] > 0){
right -- ;
}else{
left ++ ;
}
}
}
return list ;
}
}