angChain版本:0.0.147 ;(没想到第二更LangChain已经更新到147了)
一、国内外大模型发布现状
图1 大模型时间线(2023-arxiv-A Survey of Large Language Models)
模型名称 | 企业/高校 | 发布时间 |
---|---|---|
ERNIE Bot(文心一言) | 百度 | 2023年3月 |
ChatGLM | 清华大学 | 2023年3月 |
通义千问 | 阿里 | 2023年4月 |
MOSS | 复旦大学 | 2023年4月 |
从图1中可以看出,大模型时代的起始最早可以追溯到2019年Google推出的T5大模型,直到ChatGPT在22年底推出,23年初开放测试后爆火。至此,真正进入大模型的高速发展时期。
2023年4月,OpenAI API进一步封锁国内用户的使用,身边挺多朋友的OpenAI账号被封。因此,我们的目光陆续转移到国内的大模型。国内的大语言模型主要包括了上表中的几个,其中清华大学发布的ChatGLM-6B是比较平民的大模型版本,在保证一定的效果的基础上也支持单卡部署,是很好的实验Baseline。
从ChatGLM Github[ChatGLM-6B, Github]
的介绍中可以知道,其硬件需求一张RTX3090就能满足。
量化等级 | 最低GPU现存(推理) | 最低GPU显存(高效参数微调) |
---|---|---|
FP16 | 13GB | 14GB |
二、LLMs接入OpenAI
虽然OpenAI API的使用已经越来越困难,但我还是循例写一下这部分的接入。
在OpenAI官网注册,获得对应的API key。
2.1 Python环境依赖
pip install langchain==0.0.147
pip install openai==0.27.4
2.2 使用LangChain调用GPTs
import os
from langchain.schema import HumanMessage
from langchain.chat_models import ChatOpenAI
# global environment
os.environ["OPENAI_API_KEY"] = "sk-*********************************"
# llm initialization
llm = ChatOpenAI(model_name="gpt-3.5-turbo", temperature=0)
while True:
human_input = input("(human): ")
human_input = [HumanMessage(content=human_input)]
ai_output = llm(human_input)
print(f"(ai): {ai_output.content}")
访问如果需要代理,可以通过openai包进行配置,代码如下所示:
import os
import openai
from langchain.schema import HumanMessage
from langchain.chat_models import ChatOpenAI
openai.proxy = {
"http": "{proxy_ip}:{port}"
}
# global environment
os.environ["OPENAI_API_KEY"] = "sk-*********************************"
# llm initialization
llm = ChatOpenAI(model_name="gpt-3.5-turbo", temperature=0)
while True:
human_input = input("(human): ")
human_input = [HumanMessage(content=human_input)]
ai_output = llm(human_input)
print(f"(ai): {ai_output.content}")
三、LLMs接入ChatGLM
ChatGLM是清华大学团队推出的平民大模型,使用RTX3090单卡即可部署,代码库开源,是目前大模型的最佳平替。
3.1 ChatGLM 本地部署
开发环境准备
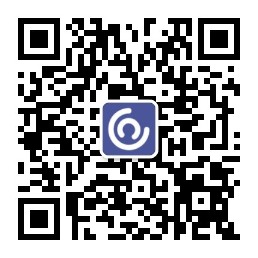
requirements.txt:
- 从ChatGLM代码库中复制它的requirements.txt下来;
- 参考:https://github.com/THUDM/ChatGLM-6B/blob/main/requirements.txt;
pip install -r requirements.txt
模型文件准备
方法1:直接从huggingface模型仓库拉取模型文件(需要先安装Git LFS,拉取速度很慢,不建议);
git clone https://huggingface.co/THUDM/chatglm-6b
方法2:从huggingface模型残酷拉取模型实现,然后从清华仓库下载模型参数文件,然后替换到chatglm-6b文件夹中;
GIT_LFS_SKIP_SMUDGE=1 git clone https://huggingface.co/THUDM/chatglm-6b
# 下载模型参数文件...
mv chatglm-6b/* THUDM/chatglm-6b/
方法3:点赞+收藏+关注,并评论,我会私信分享模型文件的百度云盘链接;
模型本地调用
一切准备就绪后,我们可以通过下列代码在本地测试ChatGLM;(记得根据实际情况修改使用的显卡参数:CUDA_VISIBLE_DEVICES)
import os
from transformers import AutoTokenizer, AutoModel
os.environ["CUDA_VISIBLE_DEVICES"] = "2"
tokenizer = AutoTokenizer.from_pretrained("THUDM/chatglm-6b", trust_remote_code=True)
model = AutoModel.from_pretrained("THUDM/chatglm-6b", trust_remote_code=True).half().cuda()
model = model.eval()
human_input = "你好"
response, history = model.chat(tokenizer, human_input, history=[])
print(f"Human: {human_input}")
print(f"AI: {response}")
运行后可以得到模型的下列输出:
Loading checkpoint shards: 100%|██████████████████████████████████████████████████████████████| 8/8 [00:07<00:00, 1.07it/s]
AI: 你好 !我是人工智能助手 ChatGLM-6B,很高兴见到你,欢迎问我任何问题。
模型服务调用(基于Flask)
模型服务代码如下
import os
import json
from flask import Flask
from flask import request
from transformers import AutoTokenizer, AutoModel
# system params
os.environ["CUDA_VISIBLE_DEVICES"] = "2"
tokenizer = AutoTokenizer.from_pretrained("THUDM/chatglm-6b", trust_remote_code=True)
model = AutoModel.from_pretrained("THUDM/chatglm-6b", trust_remote_code=True).half().cuda()
model.eval()
app = Flask(__name__)
@app.route("/", methods=["POST", "GET"])
def root():
"""root
"""
return "Welcome to chatglm model."
@app.route("/chat", methods=["POST"])
def chat():
"""chat
"""
data_seq = request.get_data()
data_dict = json.loads(data_seq)
human_input = data_dict["human_input"]
response, _ = model.chat(tokenizer, human_input, history=[])
result_dict = {
"response": response
}
result_seq = json.dumps(result_dict, ensure_ascii=False)
return result_seq
if __name__ == "__main__":
app.run(host="0.0.0.0", port=8595, debug=False)
服务启动后可以得到以下日志:
Loading checkpoint shards: 100%|█████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████| 8/8 [00:07<00:00, 1.03it/s]
* Serving Flask app 'chatglm_server'
* Debug mode: off
WARNING: This is a development server. Do not use it in a production deployment. Use a production WSGI server instead.
* Running on all addresses (0.0.0.0)
* Running on http://127.0.0.1:8595
* Running on http://10.158.99.12:8595
Press CTRL+C to quit
使用POST请求Flask服务可以得到以下返回
$ curl -d '{"human_input": "你好"}' http://127.0.0.1:8595/chat
{"response": "你好 !我是人工智能助手 ChatGLM-6B,很高兴见到你,欢迎问我任何问题。"}
3.2 使用LangChain调用ChatGLM
在2.1小节我们已经看到了LangChain直接调用OpenAI接口的示例,本小节我们来介绍一下我们如果有自己的大语言模型,该如何接入LangChain,以便后续跟LangChain的其他模块协同。
答案是:LLMs模块。
我们使用LLMs模块封装ChatGLM,请求我们的模型服务,主要重构两个函数:
- _call:模型调用的主要逻辑,输入用户字符串,输出模型生成的字符串;
- _identifying_params:返回模型的描述信息,通常返回一个字典,字典中包括模型的主要参数;
import time
import logging
import requests
from typing import Optional, List, Dict, Mapping, Any
import langchain
from langchain.llms.base import LLM
from langchain.cache import InMemoryCache
logging.basicConfig(level=logging.INFO)
# 启动llm的缓存
langchain.llm_cache = InMemoryCache()
class ChatGLM(LLM):
# 模型服务url
url = "http://127.0.0.1:8595/chat"
@property
def _llm_type(self) -> str:
return "chatglm"
def _construct_query(self, prompt: str) -> Dict:
"""构造请求体
"""
query = {
"human_input": prompt
}
return query
@classmethod
def _post(cls, url: str,
query: Dict) -> Any:
"""POST请求
"""
_headers = {"Content_Type": "application/json"}
with requests.session() as sess:
resp = sess.post(url,
json=query,
headers=_headers,
timeout=60)
return resp
def _call(self, prompt: str,
stop: Optional[List[str]] = None) -> str:
"""_call
"""
# construct query
query = self._construct_query(prompt=prompt)
# post
resp = self._post(url=self.url,
query=query)
if resp.status_code == 200:
resp_json = resp.json()
predictions = resp_json["response"]
return predictions
else:
return "请求模型"
@property
def _identifying_params(self) -> Mapping[str, Any]:
"""Get the identifying parameters.
"""
_param_dict = {
"url": self.url
}
return _param_dict
if __name__ == "__main__":
llm = ChatGLM()
while True:
human_input = input("Human: ")
begin_time = time.time() * 1000
# 请求模型
response = llm(human_input, stop=["you"])
end_time = time.time() * 1000
used_time = round(end_time - begin_time, 3)
logging.info(f"chatGLM process time: {used_time}ms")
print(f"ChatGLM: {response}")
使用LLM模块封装我们的模型接口的一个好处是有利于后续跟LangChain的其他模块协同,在这里给大家举一个LangChain Cache的例子,LangChain给LLM模块配置了Cache,如果同一个问题被第二次提问,模型可以快速给出答案。
启动Cache,需要在脚本中增加这两行代码即可:
from langchain.cache import InMemoryCache
# 启动llm的缓存
langchain.llm_cache = InMemoryCache()
启动Cache后,ChatGLM的访问效果如下所示,当第二次请求模块同一个问题时,模块处理用户问题所用的时间少了一个数量级:
Human: 你好
INFO:root:chatGLM process time: 993.685ms
ChatGLM: 你好 !我是人工智能助手 ChatGLM-6B,很高兴见到你,欢迎问我任何问题。
Human: 你好
INFO:root:chatGLM process time: 0.116ms
ChatGLM: 你好 !我是人工智能助手 ChatGLM-6B,很高兴见到你,欢迎问我任何问题。
Reference
- [ChatGLM-6B, Github] https://github.com/THUDM/ChatGLM-6B
- [Survey of LLM, arxiv, 2023] A Survey of Large Language Models
一起学AI,关注我 持续更新中