【功能说明】
请开发一个类似微信的主页面框架,UI布局为上中下结构,包含4个tab页面;并且选择下方的不同功能可以在不同的界面之间切换。点击对应的图片之后要有相应的响应,且响应的是对应的界面。之前生成的界面会在下一个点击之后隐藏起来。
开发技术为:layout xml、控件、监听,fragment。
【实验步骤】
1、首先在【res -> Layout】文件夹下新建一个top.xml文件,并且将相应的图标复制到drawable中。在top.xml文件中拖一个linearLayout和一个textview控件。
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="65dp"
android:gravity="center"
android:background="#e6e6e6"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/WeChat"
android:layout_width="255dp"
android:layout_height="37dp"
android:gravity="center"
android:rotationX="0"
android:text="WeChat"
android:textColor="#000000"
android:textSize="30dp" />
</LinearLayout>
</LinearLayout>
2、同目录下新建一个bottom.xml文件:拖一个LinearLayout(horizontal)并在它之下拖四个LinearLayout(verticl),再在它们每个下面各拖一个imageButton和textview。在添加控件时,要注意拖动的位置,使控件处于正确的层次结构下。
添加LinearLayout时:
添加button时:
扫描二维码关注公众号,回复:
15284153 查看本文章
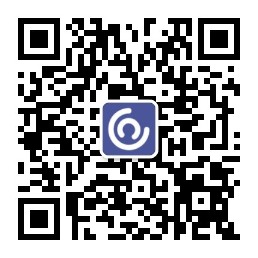
修改名称,方便辨别布局板块,注意不可以出现重名。添加好的四个LinearLayout如下:(有报错先不要慌,后续可能可以通过修改代码解决)
以第一个button为例,代码如下:(除内容和名称不同外,四个控件格式一致)
<LinearLayout
android:id="@+id/id_LinearLayout1"
android:layout_width="0dp"
android:layout_height="100dp"
android:layout_gravity="bottom"
android:layout_weight="1"
android:orientation="vertical">
<ImageButton
android:id="@+id/imageButton1"
android:layout_width="match_parent"
android:layout_height="78dp"
android:scaleType="fitCenter"
android:contentDescription="@string/app_name"
app:srcCompat="@drawable/button1_wechat" />
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="微信"
android:gravity="center"
android:textSize="15sp"
android:layout_gravity="bottom" />
</LinearLayout>
3、在activity_main.xml中运用Fragment控件将以上两个.xml文件组合在一起。
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<FrameLayout
android:id="@+id/id_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1">
<include layout="@layout/bottom" />
<include layout="@layout/top" />
</FrameLayout>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
4、在layout目录下继续新建xml文件,tab01.xml~tab04.xml,设置四个按钮对应的中间显示(本实验用文本内容简易替代)
以第一个tab页面为例:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="horizontal">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:textSize="25sp"
android:text="这是微信聊天页面" />
</LinearLayout>
5、在MainActivity.java文件中完成对底部控件的点击的监听和将监听到的底部点击事件,传递给fragment的事件控制。
新建四个fragment(blank)的java文件和一个mainactivity.java文件
MainActivity:
package com.example.myapplication.ui;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.ImageButton;
import androidx.appcompat.app.AppCompatActivity;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentTransaction;
import com.example.myapplication.R;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private final Fragment mTab01 = new weixinFragment();
private final Fragment mTab02 = new frdFragment();
private final Fragment mTab03 = new contactFragment();
private final Fragment mTab04 = new settingFragment();
//private LinearLayout mTabMessage;
//private LinearLayout mTabFriend;
//private LinearLayout mTabAddress;
//private LinearLayout mTabSetting;
private ImageButton mImgMessage;
private ImageButton mImgFriend;
private ImageButton mImgAddress;
private ImageButton mImgSetting;
private FragmentManager fm;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
initView();
initFragment();
initEvent();
setSelect(0);
}
private void initFragment(){
fm = getSupportFragmentManager();
FragmentTransaction transaction = fm.beginTransaction();
transaction.add(R.id.id_content,mTab01);
transaction.add(R.id.id_content,mTab02);
transaction.add(R.id.id_content,mTab03);
transaction.add(R.id.id_content,mTab04);
transaction.commit();
}
private void initView() {
mImgMessage = findViewById(R.id.imageButton1);
mImgFriend = findViewById(R.id.imageButton2);
mImgAddress = findViewById(R.id.imageButton3);
mImgSetting = findViewById(R.id.imageButton4);
}
private void initEvent(){
mImgMessage.setOnClickListener(this);
mImgFriend.setOnClickListener(this);
mImgAddress.setOnClickListener(this);
mImgSetting.setOnClickListener(this);
}
private void setSelect(int i){
FragmentTransaction transaction = fm.beginTransaction();
hideFragment(transaction);
switch (i) {
case 0:
transaction.show(mTab01);
mImgMessage.setImageResource(R.drawable.button1_wechat);
break;
case 1:
transaction.show(mTab02);
mImgFriend.setImageResource(R.drawable.button2_phone);
break;
case 2:
transaction.show(mTab03);
mImgAddress.setImageResource(R.drawable.button3_found);
break;
case 3:
transaction.show(mTab04);
mImgSetting.setImageResource(R.drawable.button4_mine);
break;
default:
break;
}
transaction.commit();
}
private void hideFragment(FragmentTransaction transaction) {
transaction.hide(mTab01);
transaction.hide(mTab02);
transaction.hide(mTab03);
transaction.hide(mTab04);
}
public void onClick(View v){
resetImg();
switch (v.getId()){
case R.id.imageButton1:
setSelect(0);
break;
case R.id.imageButton2:
setSelect(1);
break;
case R.id.imageButton3:
setSelect(2);
break;
case R.id.imageButton4:
setSelect(3);
break;
default:
break;
}
}
public void resetImg(){
mImgMessage.setImageResource(R.drawable.button1_wechat);
mImgFriend.setImageResource(R.drawable.button2_phone);
mImgAddress.setImageResource(R.drawable.button3_found);
mImgSetting.setImageResource(R.drawable.button4_mine);
}
}
【实验结果】
【实验过程中遇到的问题】
1、在添加下方四个按钮的布局时,一共有四个选择图标,首先先创建一个图像与文本组合,再进行复制操作,但是不能直接这样复制粘贴,会有重名无法修改。
2、文件名称不能出现中文,修改一下文件命名。