建立一个汽车Auto类,包括轮胎个数tyre,汽车颜色color,车身重量weigth、速度speed等成员变量。并通过不同的构造方法创建实例。至少要求:汽车能够加速accelerate(),减速slow(),停车stop()。创建测试类实例化Auto类的对象,调用加速、减速、停车等方法。
package com.kk;
class Auto {
public int tyre;
public double weight;
public String color;
public double speed;
public double accelerate(double up){
speed+=up;
return speed;
}
/*方法*/
public double slow(double down){
speed-=down;
return speed;
}
public double stop(){
speed=0;
return speed;
}
/*两种构造方法*/
public Auto(int tire,double weight,String colour,double speed) {
this.tyre =tire;
this.weight =weight;
this.color =colour;
this.speed =speed;
}
public Auto() {
}
}
public class Test {
public static void main(String[] args) {
Auto smallcar=new Auto();
smallcar.tyre=3;
smallcar.weight=150;
smallcar.color="深空灰";
smallcar.speed=100;
System.out.println("轮胎个数:"+smallcar.tyre+"\t车身重量:"+smallcar.weight+"\t汽车颜色:"+smallcar.color+"\t速度:"+smallcar.speed);
smallcar.slow(20);
System.out.println("汽车减速的速度:"+smallcar.speed);
smallcar.stop();
System.out.println("汽车停下的速度:"+smallcar.speed);
}
}
父类Employee 属性:name、sex , 带一个构造方法Employee(String n, char s) ,子类 Worker继承自Employee 属性:char category;//类别 boolean dressAllowance; //是否提供服装津贴 , 有一个构造方法 负责构造所有属性,还有一个自定义方法 isDressAll() 这个方法 负责通过判断dressAllowance的值输出 ,是否提供服装津贴。新建一个类测试类TestDemo ,在main方法中新建一个Worker对象,输出这个对象的所有属性 ,并调用isDressAll()方法得到津贴信息。
package com.kk;
class Employee {
private String name;
private String sex;
public Employee(String n, String s) {
this.name = n;
this.sex = s;
System.out.println("姓名:"+n+",性别:"+sex);
}
}
class Worker extends Exception{
private char category;
private boolean dressAllowance;
public Worker(char category, boolean dressAllowance) {
this.category = category;
this.dressAllowance = dressAllowance;
System.out.println("category:"+category+",dressAllowance:"+dressAllowance);
}
public boolean isDressAll(){
boolean a=dressAllowance;
System.out.println("是否津贴:"+a);
return a;
}
}
public class TestDemo {
public static void main(String[] args) {
Employee employee=new Employee("张三","男");
Worker worker=new Worker('是',true);
worker.isDressAll();
}
}
接受用户从键盘输入一个三位整数(如385),计算并输出各位数字之和
import java.util.Scanner;
public class Zuoye {
public static void main(String[] args) {
System.out.print("请输入一个三位数:");
Scanner add= new Scanner(System.in);
int num=add.nextInt();
int baiwei=num/100;
int shiwei=num/10%10;
int gewei=num%10;
System.out.print("各位数字之和:"+(baiwei+shiwei+gewei));
}
}
要求用户从键盘输入a,b和c的值,计算下列表达式的值。
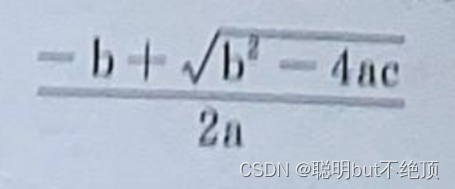
import java.util.Scanner;
public class Zuoye {
public static void main(String[] args) {
Scanner gs =new Scanner(System.in);
System.out.print("请输入a的值:");
double a=gs.nextDouble();
System.out.print("请输入b的值:");
double b=gs.nextDouble();
System.out.print("请输入c的值:");
double c=gs.nextDouble();
if ((b*b-4*a*c)>=0&&(2*a!=0)) {
System.out.println("表达式的值为:"+((-b)+Math.sqrt(b*b-4*a*c))/(2*a));
}
else {
System.out.println("输入的值有误,请重试");
}
}
}
打印出九九乘法表
public class Test4 {
public static void main(String[] args) {
int i=0;
for(i=1;i<=9;i++) {
int j=0;
for(j=1;j<=i;j++) {
System.out.print(j+"*"+i+"="+i*j+"\t");
}
System.out.println();
}
}
}
求出1-1000的所有完全数。完全数是指其所有因子(包括1但不包括该数本身)的和等于该数。例如,28=1+2+4+7+14,28就是一个完全数。
public class Text4 {
public static void main(String[] args) {
for (int i = 1; i <= 1000; ++i) {
int sum = 0;
for(int j = 1; j < i; ++j){
if(i % j == 0)
sum += j;
}
if(i == sum){
System.out.println(i);
}
}
}
}