类:
- ProductTest,主方法操作
- ProductService,承接Test与Dao
- ProductDao,数据库连接方面
- Product,一个JavaBean类。
- JDBCUtils,工具类
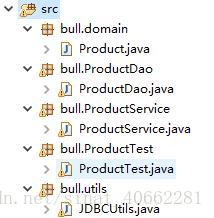
包
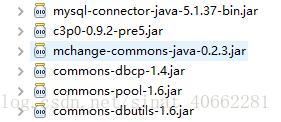
- 一个c3p0-config.xml文件。

数据库准备数据
create database webdb;
use webdb;
CREATE TABLE products(
pid int PRIMARY KEY auto_increment ,
pname VARCHAR(50),
price INT,
flag VARCHAR(2), #是否上架标记为:1表示上架、0表示下架
category_id VARCHAR(32)
);
#商品
INSERT INTO products(pname,price,flag,category_id) VALUES('联想',5000,'1','c001');
INSERT INTO products(pname,price,flag,category_id) VALUES('海尔',3000,'1','c001');
INSERT INTO products(pname,price,flag,category_id) VALUES('雷神',5000,'1','c001');
INSERT INTO products(pname,price,flag,category_id) VALUES('JACK JONES',800,'1','c002');
INSERT INTO products(pname,price,flag,category_id) VALUES('真维斯',200,'1','c002');
INSERT INTO products(pname,price,flag,category_id) VALUES('花花公子',440,'1','c002');
INSERT INTO products(pname,price,flag,category_id) VALUES('劲霸',2000,'1','c002');
INSERT INTO products(pname,price,flag,category_id) VALUES('香奈儿',800,'1','c003');
INSERT INTO products(pname,price,flag,category_id) VALUES('相宜本草',200,'1','c003');
Product
package bull.domain;
public class Product implements java.io.Serializable {
/**
*
*/
private static final long serialVersionUID = -6968409272670177143L;
private Integer pid;
private String pname;
private Integer price;
private String flag;
private String category_id;
public Product(Integer pid, String pname, Integer price, String flag,String category_id) {
this.pid = pid;
this.pname = pname;
this.price = price;
this.flag = flag;
this.category_id = category_id;
}
public Product() {
}
public Integer getPid() {
return pid;
}
public void setPid(Integer pid) {
this.pid = pid;
}
public String getPname() {
return pname;
}
public void setPname(String pname) {
this.pname = pname;
}
public Integer getPrice() {
return price;
}
public void setPrice(Integer price) {
this.price = price;
}
public String getFlag() {
return flag;
}
public void setFlag(String flag) {
this.flag = flag;
}
public String getCategory_id() {
return category_id;
}
public void setCategory_id(String category_id) {
this.category_id = category_id;
}
@Override
public String toString() {
return "Product [pid=" + pid + ", pname=" + pname + ", price=" + price
+ ", flag=" + flag + ", category_id=" + category_id + "]";
}
}
JDBCUtils
package bull.utils;
import java.sql.Connection;
import java.sql.SQLException;
import javax.sql.DataSource;
import com.mchange.v2.c3p0.ComboPooledDataSource;
public class JDBCUtils {
private static ComboPooledDataSource dataSource = new ComboPooledDataSource("bull");
private static ThreadLocal<Connection> local = new ThreadLocal<Connection>();
public static DataSource getDataSource() {
return dataSource;
}
public static Connection getConnection() {
Connection conn = local.get();
try {
if(conn == null) {
conn = dataSource.getConnection();
local.set(conn);
}
return conn;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
ProductTest
package bull.ProductTest;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import bull.ProductService.ProductService;
import bull.domain.Product;
public class ProductTest {
public static void main(String[] args) {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while(true) {
System.out.println("请输入操作命令:");
System.out.println("C:创建、U:修改、D:删除、DA:删除所有、I:通过id查询、FA:查询所有、Q:退出");
try {
String line = reader.readLine();
switch(line.toUpperCase()) {
case "C":
add(reader);
break;
case "U":
edit(reader);
break;
case "D":
delete(reader);
break;
case "DA":
deleteAll(reader);
break;
case "I":
findById(reader);
break;
case "FA":
findAll(reader);
break;
case "Q":
System.out.println("欢迎下次使用!");
System.exit(-1);
break;
default:
break;
}
} catch (Exception e) {
e.printStackTrace();
System.out.println("操作异常,请重新输入!");
}
}
}
private static void deleteAll(BufferedReader reader) {
try {
ProductService productService = new ProductService();
List<Integer> list = new ArrayList<Integer>();
while(true) {
System.out.println("请输入你要删除的商品编号(输入-1退出):");
String pidStr = reader.readLine();
Integer pid = Integer.parseInt(pidStr);
if(pid == -1) {
break;
}
Product product = productService.findById(pid);
if(product == null) {
System.out.println("商品不存在!");
}
else {
System.out.println("已选中要删除记录:" + product);
list.add(pid);
}
}
if(list.size() == 0) {
System.out.println("没有选择要删除的记录!");
return;
}
System.out.println("是否删除选中的" + list.size() + "条记录?Y/N");
String judge = reader.readLine();
if(judge.equalsIgnoreCase("y")) {
productService.deleteAll(list);
System.out.println("成功删除"+ list.size() + "条记录!");
}
else {
System.out.println("操作取消!");
}
} catch (Exception e) {
System.out.println("批量删除记录失败,请重新操作!");
}
}
private static void delete(BufferedReader reader) {
try {
System.out.println("请输入你要删除的商品编号:");
String pidStr = reader.readLine();
Integer pid = Integer.parseInt(pidStr);
ProductService productService = new ProductService();
Product product = productService.findById(pid);
if(product == null) {
System.out.println("此商品不存在,请重新输入!");
}
else {
System.out.println("此商品是:" + product);
System.out.println("是否删除?Y/N");
String judge = reader.readLine();
if(judge.equalsIgnoreCase("y")) {
productService.delete(pid);
System.out.println("删除成功!");
}
else {
System.out.println("操作取消!");
return;
}
}
} catch (Exception e) {
System.out.println("删除失败,请重新操作!");
}
}
private static void findAll(BufferedReader reader) {
try {
ProductService productService = new ProductService();
List<Map<String,Object>> list = productService.findAll();
for (Map<String,Object> map : list) {
System.out.println(map);
}
System.out.println("共有"+list.size()+"条记录!");
} catch (Exception e) {
System.out.println("查询所有异常,请重新输入!");
}
}
private static void findById(BufferedReader reader) {
try {
System.out.println("请输入你要查询的商品编号:");
String pidStr = reader.readLine();
Integer pid = Integer.parseInt(pidStr);
ProductService productService = new ProductService();
Product product = productService.findById(pid);
System.out.println("查询结果:" + product);
} catch (Exception e) {
e.printStackTrace();
System.out.println("查询失败,请重新输入!");
}
}
private static void edit(BufferedReader reader) {
try {
ProductService productService = new ProductService();
System.out.println("请输入你要修改的商品编号:");
String pidStr = reader.readLine();
int pid = Integer.parseInt(pidStr);
Product product = productService.findById(pid);
System.out.println("原商品是:"+product);
if(product == null) {
System.out.println("此商品不存在!");
return;
}
else {
System.out.println("请输入你要修改的商品名称");
String pname = reader.readLine();
System.out.println("请输入你要修改的商品价格:");
String priceStr = reader.readLine();
int price = Integer.parseInt(priceStr);
System.out.println("请输入你要修改的商品分类:");
String category_id = reader.readLine();
product.setPid(pid);
product.setPname(pname);
product.setPrice(price);
product.setCategory_id(category_id);
productService.edit(product);
System.out.println("修改成功!");
}
} catch (Exception e) {
System.out.println("修改异常,请重新输入!");
}
}
private static void add(BufferedReader reader) {
try {
ProductService productService = new ProductService();
System.out.println("请输入你要添加的商品名称:");
String name = reader.readLine();
System.out.println("请输入你要添加的商品价格:");
String priceStr = reader.readLine();
int price = Integer.parseInt(priceStr);
System.out.println("请输入你要添加的商品分类:");
String category_id = reader.readLine();
Product product = new Product(null,name,price,"1",category_id);
productService.add(product);
System.out.println("添加成功!");
} catch (Exception e) {
System.out.println("添加失败请重试!");
throw new RuntimeException(e);
}
}
}
ProductService
package bull.ProductService;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import org.apache.commons.dbutils.DbUtils;
import bull.ProductDao.ProductDao;
import bull.domain.Product;
import bull.utils.JDBCUtils;
public class ProductService {
private static final int Integer = 0;
private static final int List = 0;
private ProductDao productDao = new ProductDao();
public void add(Product product) throws SQLException {
productDao.add(product);
}
public Product findById(Integer pid) throws SQLException {
return productDao.findById(pid);
}
public void edit(Product product) throws SQLException {
productDao.edit(product);
}
public List findAll() throws SQLException {
return productDao.findAll();
}
public void delete(Integer pid) {
Connection conn = null;
try {
conn = JDBCUtils.getConnection();
conn.setAutoCommit(false);
productDao.delete(pid);
DbUtils.commitAndCloseQuietly(conn);
} catch (Exception e) {
DbUtils.rollbackAndCloseQuietly(conn);
throw new RuntimeException(e);
}
}
public void deleteAll(List<Integer> list) {
Connection conn = null;
try {
conn = JDBCUtils.getConnection();
conn.setAutoCommit(false);
for (Integer pid : list) {
productDao.deleteAll(pid);
}
DbUtils.commitAndCloseQuietly(conn);
} catch (Exception e) {
DbUtils.rollbackAndCloseQuietly(conn);
throw new RuntimeException(e);
}
}
}
ProductDao
package bull.ProductDao;
import java.sql.SQLException;
import java.util.List;
import java.util.Map;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.MapHandler;
import org.apache.commons.dbutils.handlers.MapListHandler;
import org.junit.Test;
import bull.domain.Product;
import bull.utils.JDBCUtils;
import bull05.DBSelectUtils.ProductJavaBean;
public class ProductDao {
public void add(Product product) throws SQLException {
QueryRunner queryRunner = new QueryRunner(JDBCUtils.getDataSource());
String sql = "insert into products(pname,price,flag,category_id) values (?,?,?,?);";
Object[] params = {product.getPname(),product.getPrice(),product.getFlag(),product.getCategory_id()};
queryRunner.update(sql, params);
}
public Product findById(Integer pid) throws SQLException {
QueryRunner queryRunner = new QueryRunner(JDBCUtils.getDataSource());
String sql = "select * from products where pid = ?;";
Object[] params = {pid};
return queryRunner.query(sql, new BeanHandler<Product>(Product.class), params);
}
public void edit(Product product) throws SQLException {
QueryRunner queryRunner = new QueryRunner(JDBCUtils.getDataSource());
String sql = "update products set pname = ?, price = ?,category_id = ? where pid = ?;";
Object[] params = {product.getPname(),product.getPrice(),product.getCategory_id(),product.getPid()};
queryRunner.update(sql, params);
}
public List findAll() throws SQLException {
QueryRunner queryRunner = new QueryRunner(JDBCUtils.getDataSource());
String sql = "select * from products;";
Object[] params = {};
List<Map<String,Object>> list = queryRunner.query(sql, new MapListHandler(), params);
return list;
}
public void delete(Integer pid) throws SQLException {
QueryRunner queryRunner = new QueryRunner();
String sql = "delete from products where pid = ?;";
Object[] params = {pid};
queryRunner.update(JDBCUtils.getConnection(), sql, params);
}
public void deleteAll(Integer pid) throws SQLException {
QueryRunner queryRunner = new QueryRunner();
String sql = "delete from products where pid = ?;";
Object[] params = {pid};
queryRunner.update(JDBCUtils.getConnection(), sql, params);
}
}