掌握常用布局和基本控件的使用方法
掌握界面图像显示的使用方法
掌握SQLite数据库的创建和基本操作方法
通过线性布局和相对布局来搭建Activity界面,在MainActivity中编写逻辑代码,运行程序,输入两条联系人信息分别点击“添加”“查询”“修改”“删除”按钮。实现联系人信息的添加、查询、修改以及删除功能。操作成功后,通过弹出的消息内容显示对应操作。下图界面仅供参考,可根据功能另外设计界面。
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/bg">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="200dp"
android:id="@+id/ll1">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="姓名:"
android:layout_marginLeft="10dp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/et1"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/ll1"
android:id="@+id/ll2">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="电话:"
android:layout_marginLeft="10dp" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/et2"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/ll2"
android:layout_marginTop="10dp"
android:id="@+id/ll3">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#5522"
android:layout_weight="1"
android:layout_marginLeft="10dp"
android:text="添加"
android:textColor="#000"
android:id="@+id/addBtn"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#5522"
android:layout_weight="1"
android:layout_marginLeft="5dp"
android:text="查询"
android:textColor="#000"
android:id="@+id/selectBtn"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#5522"
android:layout_weight="1"
android:layout_marginLeft="5dp"
android:text="修改"
android:textColor="#000"
android:id="@+id/updateBtn"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#5522"
android:layout_weight="1"
android:layout_marginRight="10dp"
android:layout_marginLeft="5dp"
android:text="删除"
android:textColor="#000"
android:id="@+id/deleteBtn"/>
</LinearLayout>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/et3"
android:layout_below="@+id/ll3"
android:hint="请输入要查询或修改或删除的姓名:"
android:layout_marginLeft="10dp"
/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#5522"
android:layout_centerHorizontal="true"
android:layout_below="@+id/et3"
android:layout_marginTop="5dp"
android:textColor="#000"
android:text="条件查询"
android:id="@+id/conditional_query_btn"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/conditional_query_btn"
android:id="@+id/showInfo"
android:layout_marginLeft="10dp" />
</RelativeLayout>
我在原有界面上加了一个条件输入框,以便条件查询、修改和删除时通过用户姓名来执行操作。
MainActivity.java
package com.example.shiyan5;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Button add, select, update, delete, conditionalQueryBtn;
private EditText name, telNumber, conditionalQueryEt;
private TextView showInfo;
private SQLiteDatabase db;
private MyDbHelper myDbHelper;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initVeiw();
add.setOnClickListener(this);
select.setOnClickListener(this);
update.setOnClickListener(this);
delete.setOnClickListener(this);
conditionalQueryBtn.setOnClickListener(this);
conditionalQueryEt.setOnClickListener(this);
myDbHelper = new MyDbHelper(this, "MyDatabase.db", null, 669);
}
private void initVeiw() {
add = findViewById(R.id.addBtn);
select = findViewById(R.id.selectBtn);
update = findViewById(R.id.updateBtn);
delete = findViewById(R.id.deleteBtn);
name = findViewById(R.id.et1);
telNumber = findViewById(R.id.et2);
showInfo = findViewById(R.id.showInfo);
conditionalQueryEt = findViewById(R.id.et3);
conditionalQueryBtn = findViewById(R.id.conditional_query_btn);
}
@Override
public void onClick(View view) {
switch (view.getId()) {
case R.id.addBtn:
db = myDbHelper.getWritableDatabase();
String userName = name.getText().toString();
String telephoneNumber = telNumber.getText().toString();
//SQLiteDatabase的insert方法来添加
/* ContentValues contentValues = new ContentValues();
contentValues.put("userName", userName);
contentValues.put("telephoneNumber", telephoneNumber);
db.insert("user", null, contentValues);*///返回的是插入记录的索引号,也就是第多少条。
db.execSQL("insert into user(username,telephoneNumber) values(?,?)", new Object[]{userName, telephoneNumber});
db.close();
break;
case R.id.selectBtn:
db = myDbHelper.getReadableDatabase();
//Cursor: 结果集,有一个游标,游标会指向结果集中的某一条记录。
Cursor cursor = db.query("user", new String[]{"userName", "telephoneNumber"}, null, null, null, null, null, null);
showInfo.setText("查询结果:\n");
if (cursor.getCount()>0) {
Toast.makeText(this, "查询成功!!", Toast.LENGTH_SHORT).show();
while (cursor.moveToNext()) {
showInfo.append("姓名:" + cursor.getString(0) + "电话号码:" + cursor.getString(1) + "\n");
}
} else {
Toast.makeText(this, "一条数据都没有呢~~", Toast.LENGTH_SHORT).show();
}
cursor.close();
db.close();
break;
case R.id.conditional_query_btn:
db=myDbHelper.getReadableDatabase();
String conditionalQuery=conditionalQueryEt.getText().toString();
Cursor cursor1=db.query("user",new String[]{"userName", "telephoneNumber"},"userName=?",new String[]{conditionalQuery},null,null,null,null);
//Cursor cursor1 =db.rawQuery("select userName,telephoneNumber from user where userName=?",new String[]{conditionalQuery});
showInfo.setText("查询结果:\n");
if (cursor1.getCount()>0) {
Toast.makeText(this, "查询成功!!", Toast.LENGTH_SHORT).show();
while (cursor1.moveToNext()){
showInfo.append("姓名:" + cursor1.getString(0) + "电话号码:" + cursor1.getString(1) + "\n");
}
}else{
Toast.makeText(this, "没找到呢~~", Toast.LENGTH_SHORT).show();
}
cursor1.close();
db.close();
break;
case R.id.updateBtn:
db=myDbHelper.getWritableDatabase();
ContentValues contentValues1=new ContentValues();
String userName1 = name.getText().toString();
String telephoneNumber1= telNumber.getText().toString();
String conditionalQuery2=conditionalQueryEt.getText().toString();
contentValues1.put("userName", userName1);
contentValues1.put("telephoneNumber", telephoneNumber1);
int a=db.update("user",contentValues1,"userName=?",new String[]{conditionalQuery2});
if (a>0) {
Toast.makeText(this, "更改成功,共更改了"+a+"条数据", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(this, "更改失败", Toast.LENGTH_SHORT).show();
}
//db.execSQL("update user set userName=?,telephoneNumber=? where userName=?",new Object[]{userName1,telephoneNumber1,conditionalQuery2});
db.close();
break;
case R.id.deleteBtn:
db=myDbHelper.getWritableDatabase();
String conditionalQuery1=conditionalQueryEt.getText().toString();
//db.execSQL("delete from user where userName=?",new Object[]{conditionalQuery1});
int i=db.delete("user","userName=?",new String[]{conditionalQuery1});//返回删除的条数。
if(i>0)
{
Toast.makeText(this, "删除成功,共删除了"+i+"条数据", Toast.LENGTH_SHORT).show();
}else
{
Toast.makeText(this, "删除失败", Toast.LENGTH_SHORT).show();
}
db.close();
break;
}
}
class MyDbHelper extends SQLiteOpenHelper {
public MyDbHelper(@Nullable Context context, @Nullable String name, @Nullable SQLiteDatabase.CursorFactory factory, int version) {
super(context, name, factory, version);
}
@Override
public void onCreate(SQLiteDatabase sqLiteDatabase) {
sqLiteDatabase.execSQL("create table user(user_id integer primary key autoincrement,userName varchar(10),telephoneNumber varchar(20))");
}
@Override
public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) {
}
}
}
增删改查我写了两种方式,第一种是db.execSQL()直接用SQL语句进行操作,第二种使用 SQLiteDatabase中自带的增删改查的方法:db.insert() db.query() db.update() db.delete()。
哈哈
哈哈哈
扫描二维码关注公众号,回复:
14971613 查看本文章
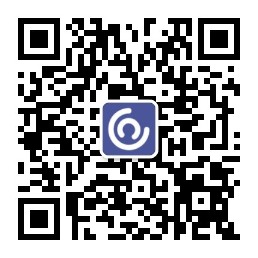
我对着潮汐推敲什么是以静制动
山林间迷雾
能不能当障眼法的内容
月转星移的轨迹跟临军布阵相同
风林火山是不是用兵之重
到最后必然是我运筹帷幄
你最后放弃抵抗
我仰望着夕阳
你低头黯然离场
听我说胜败是兵家之常
你不用放在心上
是因为我只适合