以下是一个使用ffmpeg库解码视频文件并输出每个帧的示例代码:
```c++
include
include
include
ifdef __cplusplus
extern "C" {
endif
include <libavcodec/avcodec.h>
include <libavformat/avformat.h>
include <libswscale/swscale.h>
ifdef __cplusplus
}
endif
using namespace std;
int main(int argc, char* argv[]) { if (argc != 2) { cout << "Usage: " << argv[0] << " " << endl; return 1; }
// 注册所有的编解码器和格式
av_register_all();
// 打开输入文件
AVFormatContext* format_ctx = NULL;
if (avformat_open_input(&format_ctx, argv[1], NULL, NULL) != 0) {
cerr << "Failed to open input file " << argv[1] << endl;
return 1;
}
// 查找流信息
if (avformat_find_stream_info(format_ctx, NULL) < 0) {
cerr << "Failed to find stream information" << endl;
return 1;
}
// 打印视频信息
int video_stream_index = -1;
for (unsigned int i = 0; i < format_ctx->nb_streams; i++) {
if (format_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
cerr << "No video stream found in the input file" << endl;
return 1;
}
AVCodecParameters* codec_params = format_ctx->streams[video_stream_index]->codecpar;
cout << "Video codec: " << avcodec_get_name(codec_params->codec_id) << endl;
cout << "Resolution: " << codec_params->width << "x" << codec_params->height << endl;
cout << "Frame rate: " << av_q2d(format_ctx->streams[video_stream_index]->avg_frame_rate) << " fps" << endl;
// 查找视频解码器
AVCodec* codec = avcodec_find_decoder(codec_params->codec_id);
if (codec == NULL) {
cerr << "Unsupported codec: " << avcodec_get_name(codec_params->codec_id) << endl;
return 1;
}
// 打开解码器
AVCodecContext* codec_ctx = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codec_ctx, codec_params) < 0) {
cerr << "Failed to copy codec parameters to decoder context" << endl;
return 1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
cerr << "Failed to open codec" << endl;
return 1;
}
// 创建图像转换器
AVFrame* frame = av_frame_alloc();
AVFrame* frame_rgb = av_frame_alloc();
struct SwsContext* sws_ctx = sws_getContext(codec_ctx->width, codec_ctx->height, codec_ctx->pix_fmt,
codec_ctx->width, codec_ctx->height, AV_PIX_FMT_RGB24,
SWS_BILINEAR, NULL, NULL, NULL);
int num_bytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, codec_ctx->width, codec_ctx->height, 1);
uint8_t* buffer = (uint8_t*)av_malloc(num_bytes * sizeof(uint8_t));
av_image_fill_arrays(frame_rgb->data, frame_rgb->linesize, buffer, AV_PIX_FMT_RGB24,
codec_ctx->width, codec_ctx->height, 1);
// 解码视频并输出每一帧
AVPacket pkt;
int frame_count = 0;
while (av_read_frame(format_ctx, &pkt) >= 0) {
if (pkt.stream_index == video_stream_index) {
int ret = avcodec_send_packet(codec_ctx, &pkt);
if (ret < 0) {
cerr << "Failed to send packet to decoder" << endl;
return 1;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
cerr << "Failed to decode frame" << endl;
return 1;
}
// 转换图像格式
sws_scale(sws_ctx, frame->data, frame->linesize, 0, codec_ctx->height,
frame_rgb->data, frame_rgb->linesize);
// 输出帧信息
cout << "Frame " << frame_count++ << ": " << flush;
cout << "pts=" << frame->pts << ", " << flush;
cout << "dts=" << frame->pkt_dts << ", " << flush;
cout << "keyframe=" << boolalpha << (frame->key_frame != 0) << endl;
}
}
av_packet_unref(&pkt);
}
// 清理内存
av_free(buffer);
av_frame_free(&frame_rgb);
av_frame_free(&frame);
avcodec_free_context(&codec_ctx);
avformat_close_input(&format_ctx);
avformat_free_context(format_ctx);
return 0;
} ```
该程序首先打开输入视频文件,并查找视频流信息。然后它寻找适合于该视频编解码器的解码器,并使用该解码器打开视频流。
接下来,程序创建一个图像转换器,该转换器将每个解码帧从其原始格式(例如YUV)转换为RGB24格式。程序使用此图像转换器解码每个视频帧,并输出关于该帧的一些信息(如时间戳和关键帧标志)。
最后,程序清理所有分配的内存并关闭输入视频文件。
请注意,该程序仅限于演示目的,可能不适用于所有类型的视频文件和编解码器。它可以通过添加错误检查和处理程序等额外功能进行扩展。
扫描二维码关注公众号,回复:
14943134 查看本文章
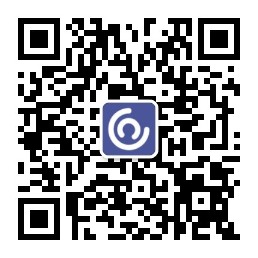
★文末名片可以免费领取音视频开发学习资料,内容包括(FFmpeg ,webRTC ,rtmp ,hls ,rtsp ,ffplay ,srs)以及音视频学习路线图等等。
见下方!↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓