Vue中使用富文本ueditor解决图片上传问题
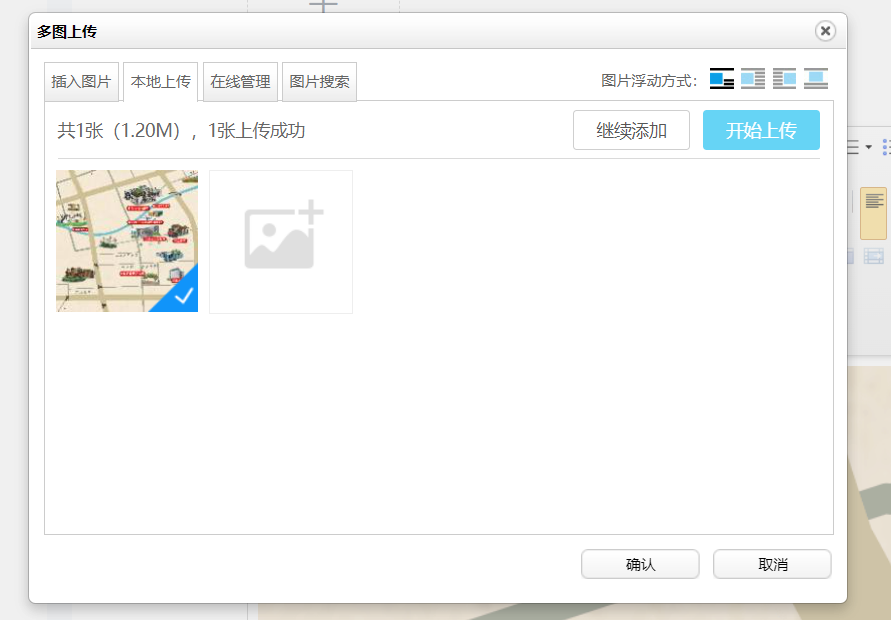
学习新内容可能少不了官方文档
目前使用的是 vue-ueditor-wrap 组件它对ueditor进行了二次封装,集成秀米等第三方插件也方便
https://hc199421.gitee.io/vue-ueditor-wrap/#/home
1. 安装组件
注意 vue-ueditor-wrap v3 仅支持 Vue 3
Vue2的话还是下载
npm i vue-ueditor-wrap
2. 下载 UEditor
https://cdn.zhenghaochuan.com/p/vue-ueditor-wrap/zip/utf8-php.zip
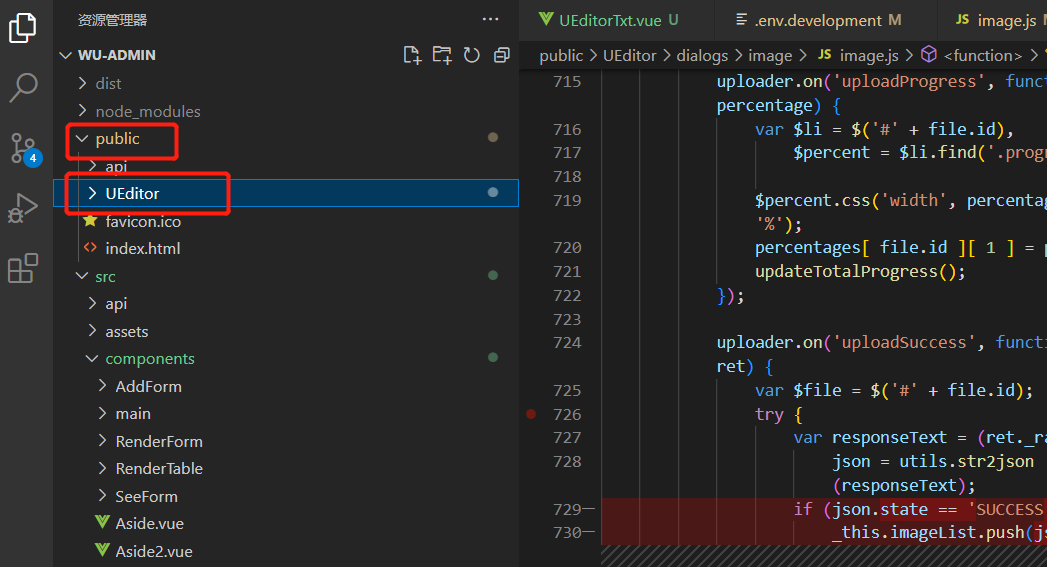
3. 注册组件
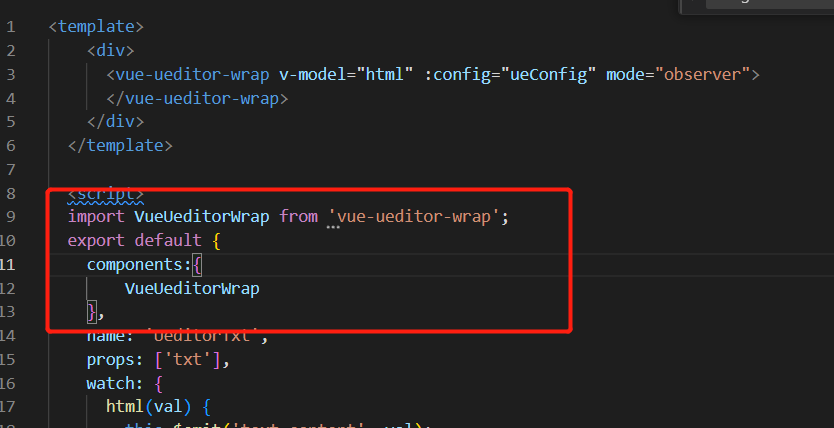
<template>
<div>
<vue-ueditor-wrap v-model="html" :config="ueConfig" mode="observer">
</vue-ueditor-wrap>
</div>
</template>
<script>
import VueUeditorWrap from 'vue-ueditor-wrap';
export default {
components:{
VueUeditorWrap
},
name: 'UeditorTxt',
props: ['txt'],
watch: {
html(val) {
this.$emit('text_content', val);
},
},
data() {
return {
html: '',
html_of: this.txt,
dialogVisible: false,
imageList: [],
ueConfig: {
UEDITOR_HOME_URL: '/UEditor/',
toolbars: [
[
'fullscreen',
'source',
'|',
'undo',
'redo',
'|',
'bold',
'italic',
'underline',
'fontborder',
'strikethrough',
'superscript',
'subscript',
'removeformat',
'formatmatch',
'autotypeset',
'blockquote',
'pasteplain',
'|',
'forecolor',
'backcolor',
'insertorderedlist',
'insertunorderedlist',
'selectall',
'cleardoc',
'|',
'rowspacingtop',
'rowspacingbottom',
'lineheight',
'|',
'customstyle',
'paragraph',
'fontfamily',
'fontsize',
//"|",
//"directionalityltr",
//"directionalityrtl",
//"indent",
'|',
'justifyleft',
'justifycenter',
'justifyright',
'justifyjustify',
'|',
//"touppercase",
//"tolowercase",
//"|",
'link',
'unlink',
//"anchor",
//"|",
'imagenone',
'imageleft',
'imageright',
'imagecenter',
'|',
'simpleupload',
'insertimage',
//"emotion",
//"scrawl",
//"insertvideo",
//"music",
//"attachment",
//"map",
//"gmap",
//"insertframe",
//"insertcode",
//"webapp",
'pagebreak',
//"template",
//"background",
'|',
'horizontal',
//"date",
//"time",
'spechars',
//"snapscreen",
//"wordimage",
'|',
'inserttable',
'deletetable',
'insertparagraphbeforetable',
'insertrow',
'deleterow',
'insertcol',
'deletecol',
'mergecells',
'mergeright',
'mergedown',
'splittocells',
'splittorows',
'splittocols',
//"charts",
//"|",
//"print",
//"preview",
//"searchreplace",
//"drafts",
//"help"
],
],
fontfamily: [
{
label: '',
name: 'songti',
val: '宋体,SimSun',
},
{
label: '仿宋',
name: 'fangsong',
val: '仿宋,FangSong',
},
{
label: '仿宋_GB2312',
name: 'fangsong',
val: '仿宋_GB2312,FangSong',
},
{
label: '',
name: 'kaiti',
val: '楷体,楷体_GB2312, SimKai',
},
{
label: '',
name: 'yahei',
val: '微软雅黑,Microsoft YaHei',
},
{
label: '',
name: 'heiti',
val: '黑体, SimHei',
},
{
label: '',
name: 'lishu',
val: '隶书, SimLi',
},
{
label: '',
name: 'andaleMono',
val: 'andale mono',
},
{
label: '',
name: 'arial',
val: 'arial, helvetica,sans-serif',
},
{
label: '',
name: 'arialBlack',
val: 'arial black,avant garde',
},
{
label: '',
name: 'comicSansMs',
val: 'comic sans ms',
},
{
label: '',
name: 'impact',
val: 'impact,chicago',
},
{
label: '',
name: 'timesNewRoman',
val: 'times new roman',
},
],
autoHeightEnabled: false, // 编辑器不自动被内容撑高
// 初始容器高度
initialFrameHeight: 300,
// 初始容器宽度
initialFrameWidth: '100%',
// 上传文件接口
serverUrl: "http://127.0.0.1:3300/uploadsimg" ,
imageActionName: 'uploadimage',
imageAllowFiles: ['.png', '.jpg', '.jpeg', '.gif', '.bmp'],
imageCompressBorder: 1600,
imageCompressEnable: true,
imageFieldName: 'file',
imageInsertAlign: 'none',
imageMaxSize: 20480000,
imagePathFormat: '/ueditor/image/{yyyy}{mm}{dd}/{time}{rand:6}',
imageUrlPrefix: '',
},
};
},
created() {
if (this.html_of != '') {
this.html = this.html_of;
}
},
methods: {
openModule(e) {
this.html = e;
},
},
};
</script>
<style scoped></style>
写个自定义组件
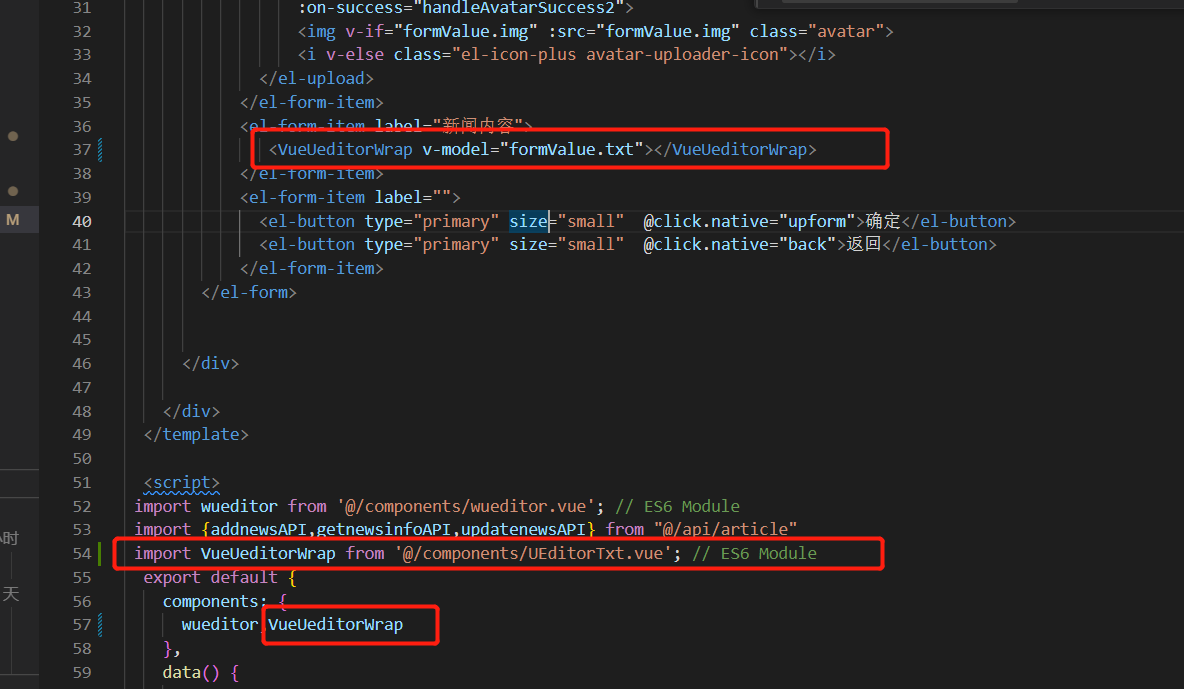
使用组件
因为后端已经有写好图片上传接口了,但是有时候需要的请求参数或者返回参数的内容以及请求头都是不符合的,此时就是需要我们前端去自定义了
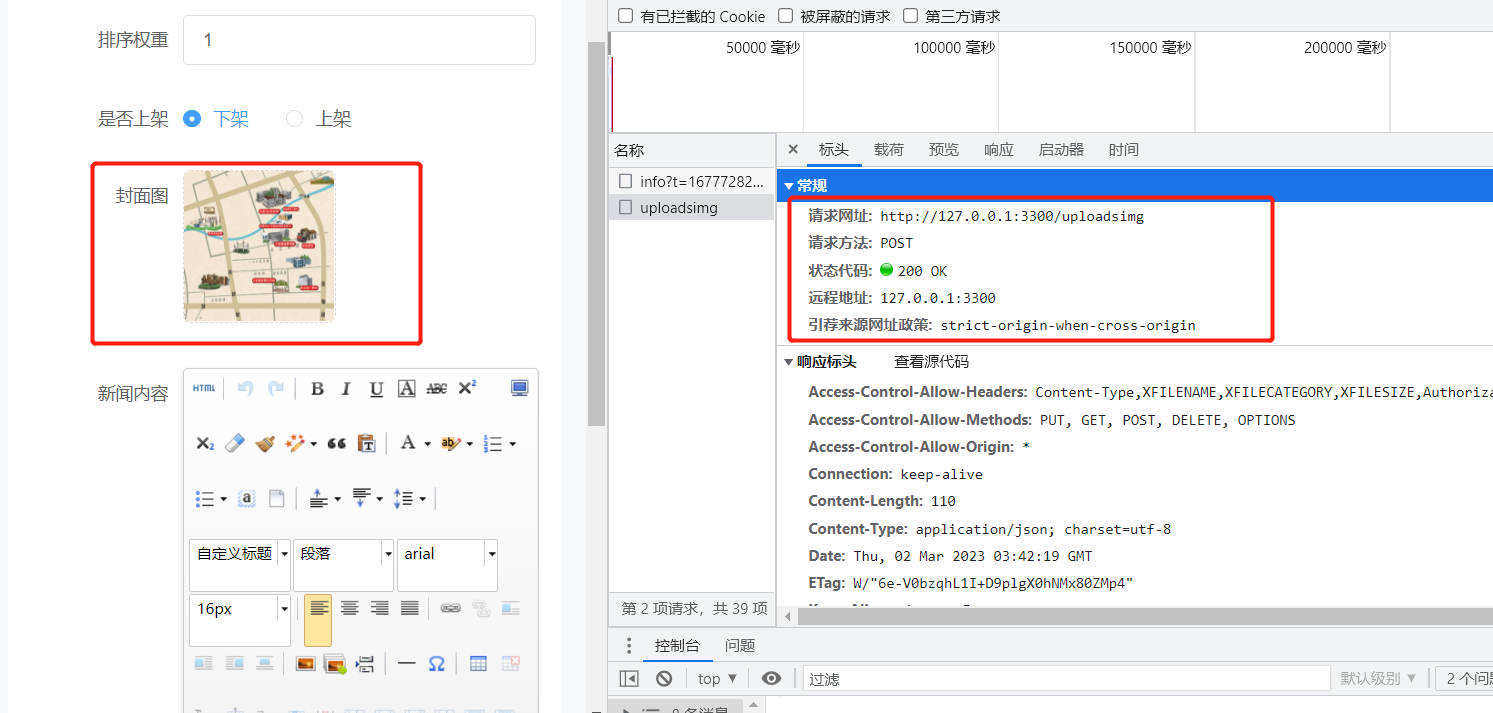


以上是我使用elementUI正常上传图片的请求及返回参数
要解决图片上传问题,
就得需要ueditor api地址为http://127.0.0.1:3300/uploadsimg而且为post请求
请求头要一致(不然浏览器容易报跨域问题)
请求参数为 file
返回的是图片绝对路径,插入的图片就需要直接拿data值
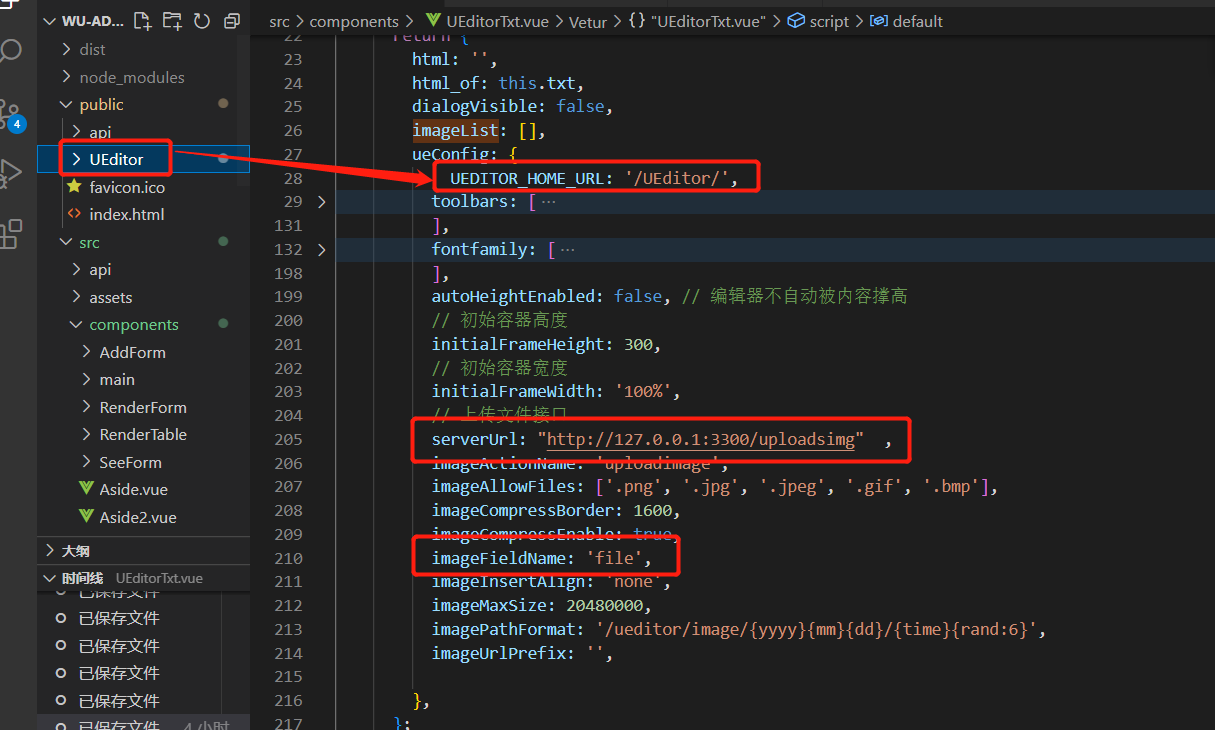
修改dialogs-》image.js文件
我直接把请求头给删了
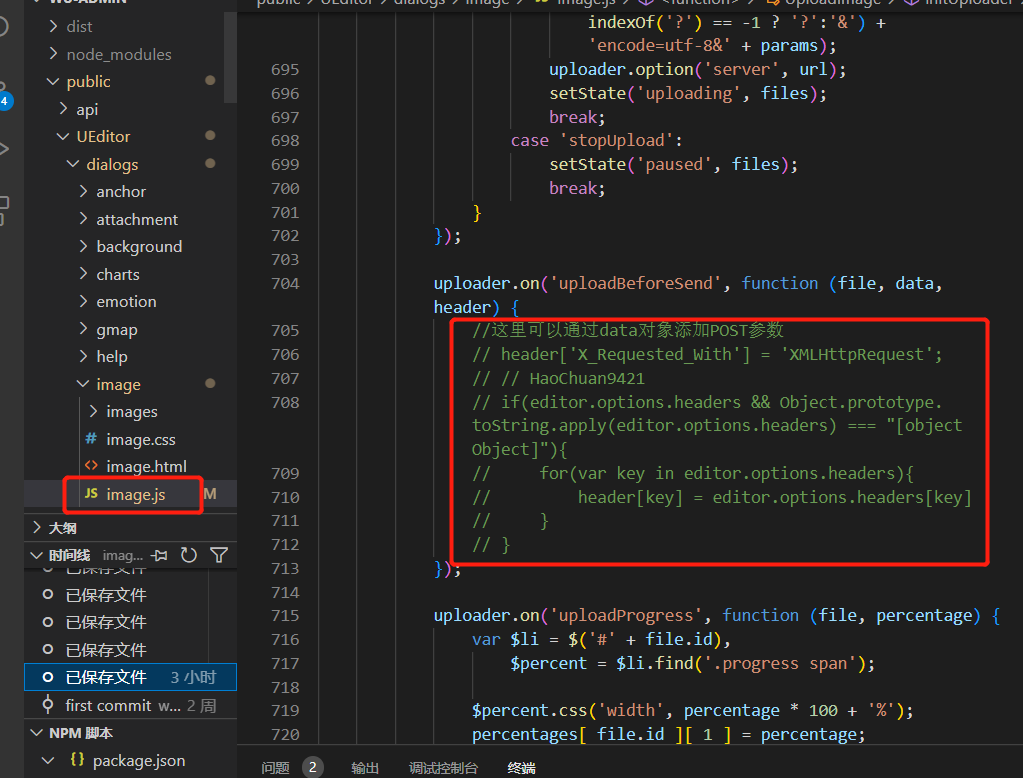
然后修改了下返回内容只要保证this.imageList数组内容有属性URL为图片地址就行了
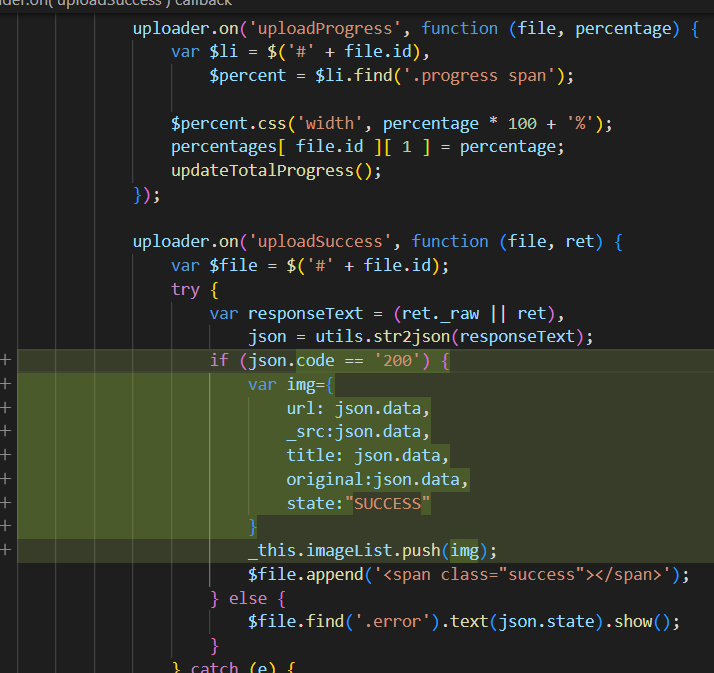
多选上传图片的话就没问题了
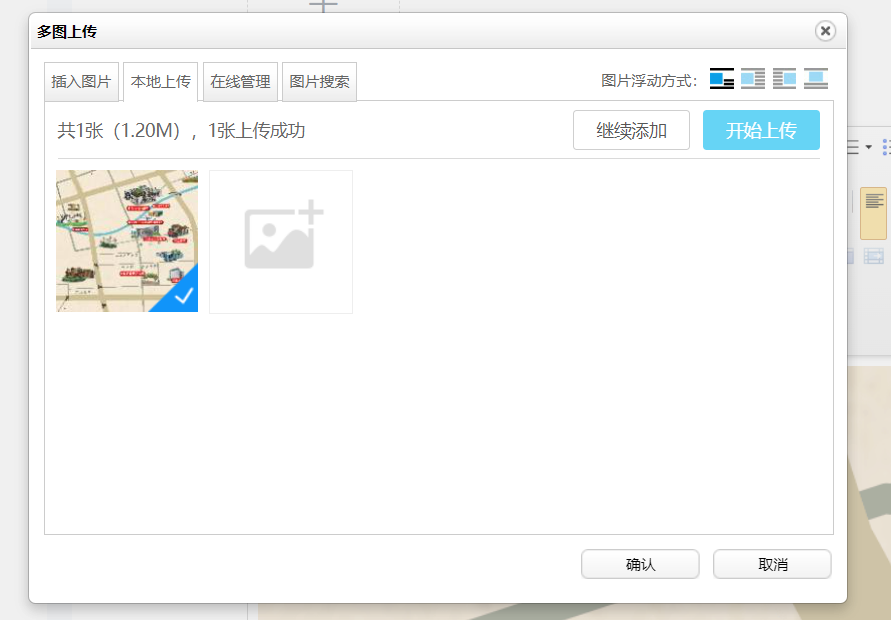