1.基本概念
View的绘制是由measuer、layout、draw三个过程才能完整的绘制一个View,其中measure是测量View的宽、高,layout是为了确认View在父容器所在的位置,draw是负责在屏幕上将View绘制出来。View的绘制流程是从ViewRoot的performTraversals开始的,ViewRoot对应ViewRootImpl类,是连接WindowManager和DecorView的纽带,View的三大流程都是通过ViewRoot来完成的。
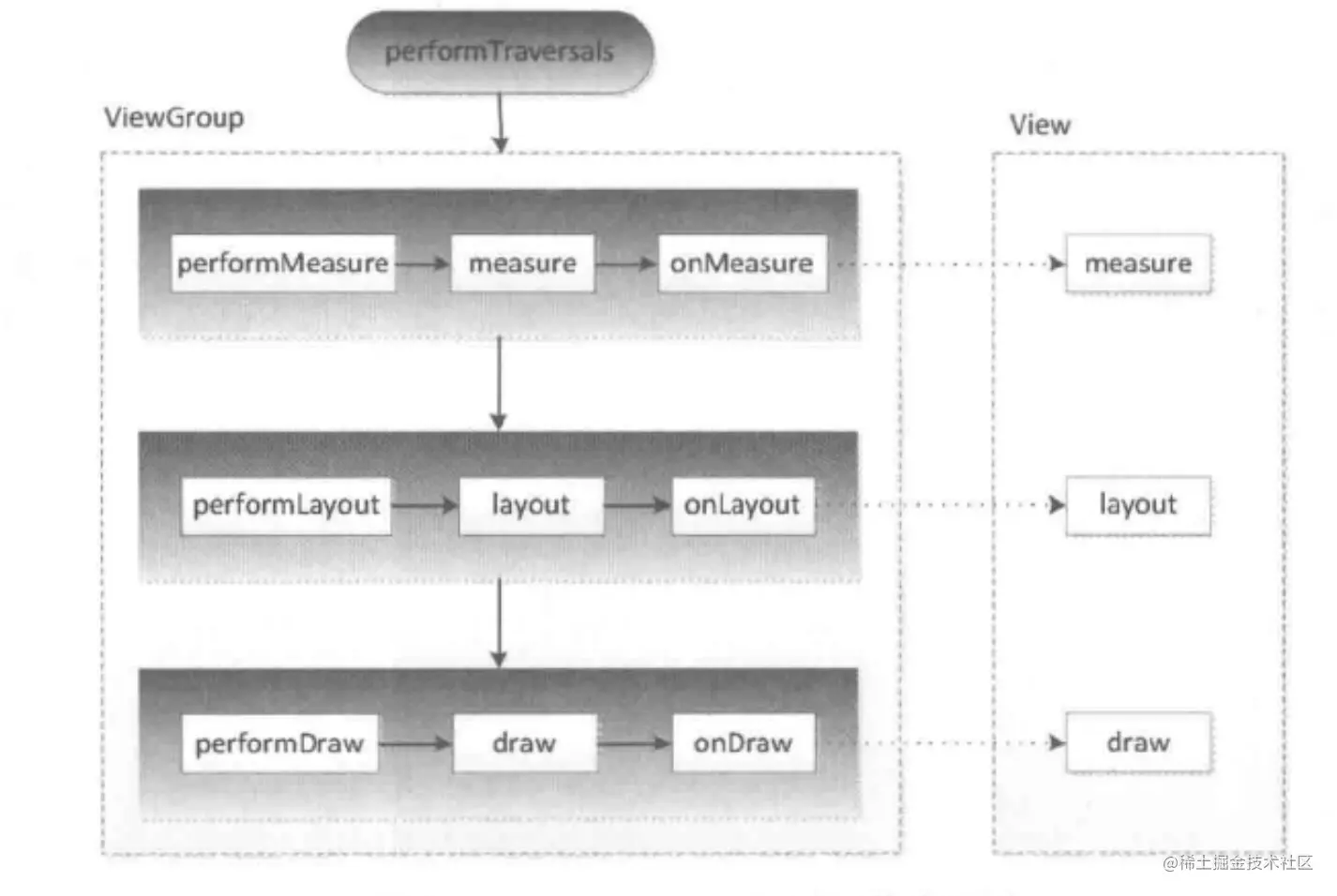
从图中可以得知,performTraversals会依次调用performMeasure,performLayout,performDraw这三个方法,这三个方法分别完成顶级View的measure,layout,draw这三大流程,来完成整个View的绘制,以performMeasure为例,performMeasure会调用measure,measure又会调用onMeasure,在onMeasure方法中则会对所有子View进行measure过程,这时measure的流程就从父容器传递给子元素了,这就完成了一次measure过程。子元素重复父容器的measure过程就完成了整个View树的遍历,那么performLayout和performDraw也是差不多的,但是performDraw有一点不同的是performDraw的传递过程是在draw方法中通过dispatchDraw来实现的不过没什么区别。
measure过程决定了View的宽/高,Measure完成以后,可以通过getMeasureWidth和getMeasureHeight获取测量后View的宽,高,并且在大多数情况下都等同于View的最终宽/高。Layout过程完成以后,可以知道View的四个顶点坐标和View的实际宽/高,通过getLeft、getTop、getRight、getBottom即可获得,宽/高可以通过getWidth,getHeight获得,并且是View的最终宽/高。Draw过程决定了View的显示,只有draw方法完成以后才可以将View的内容完全呈现出来。
2.理解MeasureSpec
这一段内容主要是加深对View测量过程的理解。MeasureSpec在决定了View的尺寸规格,但是会受到父容器的影响,因为父容器影响View的MeasureSpec的创建过程。在测量过程中,系统会将View的LayoutParams根据父容器所施加的规则转换成MeasureSpec,然后再根据这个MeasureSpec来测量View的宽/高。这里的宽/高是测量宽/高,不一定等于View的最终宽/高。
(1).MeasureSpec
MeasureSpec代表一个32位的int值,其中高2位代表SpecMode,低30位代表SpecSize,SpecMode代表测量模式,SpecSize代表在某种测量模式下的规格大小。SpecMode有三种模式:
UNSPECIFIED :父容器不对View有任何限制,有多大给多大,用于系统内部,表示一种测量状态。
AT_MOST :父容器已经指定了可用大小SpecSize,View的大小不能大于这个值,具体的值要看不同View的具体实现,对应LayoutParams的wrap_content。
EXACTLY:父容器已经检测出View的精确大小,这个时候View的最终值就是SpecSize所指定的值,对应LayoutParams的match_parent和具体的大小。
(2).MeasureSpec和layoutParams的对应关系
在View测量的时候,系统会将LayoutParams在父容器的约束下转换成MeasureSpec,然后再根据这个MeasureSpec来确定View在测量后的宽/高。需要注意的是,LayoutParams并不是唯一决定MeasureSpec的,需要由LayoutParams和父容器一起才能确定View的MeasureSpec,从而进一步确定View的宽/高。另外对于顶级View(DecorView)和普通View来说,MeasureSpec的转换过程略有不同。MeasureSpec一旦确定后,onMeasure就可以确定View的测量宽/高。
DecorView:其MeasureSpec是根据窗口尺寸和自身的LayoutParams来共同确定的;
普通View:其measureSpec是根据父容器的MeasureSpec和自身的LayoutParams来共同确定的。
①.DecorView的MeasureSpec是如何产生的呢?
对于DecorView来说,在ViewRootImpl中的measureHierarchy方法有如下一段代码,
childWidthMeasureSpec = getRootMeasureSpec(desiredWindowWidth, lp.width);childHeightMeasureSpec = getRootMeasureSpec(desiredWindowHeight, lp.height);
performMeasure(childWidthMeasureSpec, childHeightMeasureSpec);复制代码
再看一下getRootMeasureSpec的代码
/**
* Figures out the measure spec for the root view in a window based on it's
* layout params.
*
* @param windowSize
* The available width or height of the window
*
* @param rootDimension
* The layout params for one dimension (width or height) of the
* window.
*
* @return The measure spec to use to measure the root view.
*/privatestaticintgetRootMeasureSpec(int windowSize, int rootDimension) {
int measureSpec;
switch (rootDimension) {
case ViewGroup.LayoutParams.MATCH_PARENT:
// Window can't resize. Force root view to be windowSize.
measureSpec = MeasureSpec.makeMeasureSpec(windowSize, MeasureSpec.EXACTLY);
break;
case ViewGroup.LayoutParams.WRAP_CONTENT:
// Window can resize. Set max size for root view.
measureSpec = MeasureSpec.makeMeasureSpec(windowSize, MeasureSpec.AT_MOST);
break;
default:
// Window wants to be an exact size. Force root view to be that size.
measureSpec = MeasureSpec.makeMeasureSpec(rootDimension, MeasureSpec.EXACTLY);
break;
}
return measureSpec;
}
复制代码
从源码来看,DecorView的MeasureSpec的产生过程就明确了,主要遵守如下规则:
LayoutParams.MATCH_PARENT:精确模式,大小就是窗口大小;
LayoutParams.WRAP_CONTENT:最大模式,尺寸不限但是不能大于当前窗口
固定大小:精确模式,大小就是LayoutParams中所设置的大小。
②.普通View的MeasureSpec是如何产生的?
这里是指布局中的View,View的measure由ViewGroup传递而来,先看一下ViewGroup的measureChildWithMargins
/**
* Ask one of the children of this view to measure itself, taking into
* account both the MeasureSpec requirements for this view and its padding
* and margins. The child must have MarginLayoutParams The heavy lifting is
* done in getChildMeasureSpec.
*
* @param child The child to measure
* @param parentWidthMeasureSpec The width requirements for this view
* @param widthUsed Extra space that has been used up by the parent
* horizontally (possibly by other children of the parent)
* @param parentHeightMeasureSpec The height requirements for this view
* @param heightUsed Extra space that has been used up by the parent
* vertically (possibly by other children of the parent)
*/protectedvoidmeasureChildWithMargins(View child,
int parentWidthMeasureSpec, int widthUsed,
int parentHeightMeasureSpec, int heightUsed) {
finalMarginLayoutParamslp= (MarginLayoutParams) child.getLayoutParams();
finalintchildWidthMeasureSpec= getChildMeasureSpec(parentWidthMeasureSpec,
mPaddingLeft + mPaddingRight + lp.leftMargin + lp.rightMargin
+ widthUsed, lp.width);
finalintchildHeightMeasureSpec= getChildMeasureSpec(parentHeightMeasureSpec,
mPaddingTop + mPaddingBottom + lp.topMargin + lp.bottomMargin
+ heightUsed, lp.height);
child.measure(childWidthMeasureSpec, childHeightMeasureSpec);
}
复制代码
上述方法会对子元素进行measure,在调用子元素的measure方法之前会先通过getChildMeasureSpec方法得到子元素的MeasureSpec。从代码中可以知道,子元素的MeasureSpec的创建与父元素的MeasureSpec和子元素本身的LayoutParams有关,此外还和View的margin,padding有关,具体可以查看getChildMeasureSpec的源码
/**
* Does the hard part of measureChildren: figuring out the MeasureSpec to
* pass to a particular child. This method figures out the right MeasureSpec
* for one dimension (height or width) of one child view.
*
* The goal is to combine information from our MeasureSpec with the
* LayoutParams of the child to get the best possible results. For example,
* if the this view knows its size (because its MeasureSpec has a mode of
* EXACTLY), and the child has indicated in its LayoutParams that it wants
* to be the same size as the parent, the parent should ask the child to
* layout given an exact size.
*
* @param spec The requirements for this view
* @param padding The padding of this view for the current dimension and
* margins, if applicable
* @param childDimension How big the child wants to be in the current
* dimension
* @return a MeasureSpec integer for the child
*/publicstaticintgetChildMeasureSpec(int spec, int padding, int childDimension){
int specMode = MeasureSpec.getMode(spec);
int specSize = MeasureSpec.getSize(spec);
int size = Math.max(0, specSize - padding);
int resultSize = 0;
int resultMode = 0;
switch (specMode) {
// Parent has imposed an exact size on uscase MeasureSpec.EXACTLY:
if (childDimension >= 0) {
resultSize = childDimension;
resultMode = MeasureSpec.EXACTLY;
} elseif (childDimension == LayoutParams.MATCH_PARENT) {
// Child wants to be our size. So be it.
resultSize = size;
resultMode = MeasureSpec.EXACTLY;
} elseif (childDimension == LayoutParams.WRAP_CONTENT) {
// Child wants to determine its own size. It can't be// bigger than us.
resultSize = size;
resultMode = MeasureSpec.AT_MOST;
}
break;
// Parent has imposed a maximum size on uscase MeasureSpec.AT_MOST:
if (childDimension >= 0) {
// Child wants a specific size... so be it
resultSize = childDimension;
resultMode = MeasureSpec.EXACTLY;
} elseif (childDimension == LayoutParams.MATCH_PARENT) {
// Child wants to be our size, but our size is not fixed.// Constrain child to not be bigger than us.
resultSize = size;
resultMode = MeasureSpec.AT_MOST;
} elseif (childDimension == LayoutParams.WRAP_CONTENT) {
// Child wants to determine its own size. It can't be// bigger than us.
resultSize = size;
resultMode = MeasureSpec.AT_MOST;
}
break;
// Parent asked to see how big we want to becase MeasureSpec.UNSPECIFIED:
if (childDimension >= 0) {
// Child wants a specific size... let him have it
resultSize = childDimension;
resultMode = MeasureSpec.EXACTLY;
} elseif (childDimension == LayoutParams.MATCH_PARENT) {
// Child wants to be our size... find out how big it should// be
resultSize = View.sUseZeroUnspecifiedMeasureSpec ? 0 : size;
resultMode = MeasureSpec.UNSPECIFIED;
} elseif (childDimension == LayoutParams.WRAP_CONTENT) {
// Child wants to determine its own size.... find out how// big it should be
resultSize = View.sUseZeroUnspecifiedMeasureSpec ? 0 : size;
resultMode = MeasureSpec.UNSPECIFIED;
}
break;
}
//noinspection ResourceTypereturn MeasureSpec.makeMeasureSpec(resultSize, resultMode);
}
复制代码
上述代码主要作用是根据父容器的MeasureSpec同时结合View本身的LayoutParams来确定子元素的MeasureSpec,参数中的padding表示父容器已经占用的空间,因此子元素可用的空间大小为父容器减去padding。
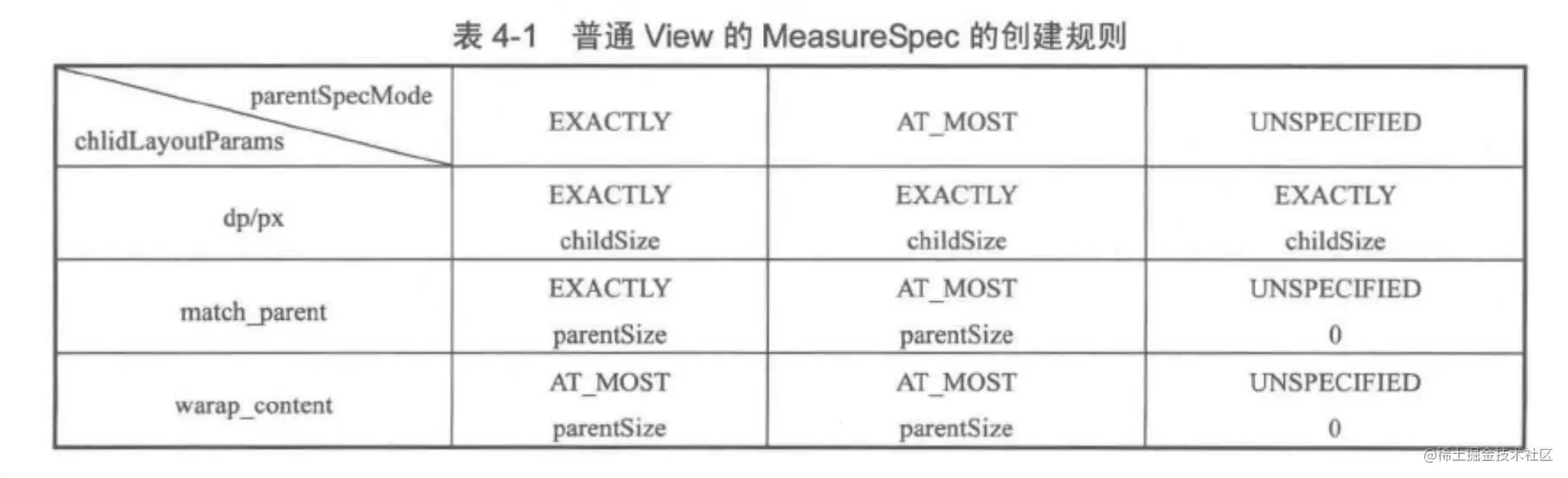
上面这张表是getChildMeasureSpec代码整理后的表示。通过上表可以得知:
当View采用固定宽/高时,不管父容器的MeasureSpec是什么,View的MeasureSpec都是精确模式,且其大小遵循LayoutParams中的大小;
当View采用match_parent时,父容器的MeasureSpec是精确模式时,View的MeasureSpec也是精确模式并且其大小是父容器的剩余空间;
当View采用match_parent时,父容器的MeasureSpec是最大模式时,View的MeasureSpec也是最大模式并且大小是父容器的剩余空间;
当View的宽高是wrap_content,父容器的MeasureSpec不管是精确模式还是最大模式,子View都是最大模式并且宽/高是父容器的剩余空间。