在自定义 Unity 的Package插件的时候,我们需要编写一个Json文件:package.json 。 其中有个 version 字段,表示其版本,其值是一个字符串。一开始我以为这个version是随便写的,但其实并不是,其实是有固定格式的:
数字.数字.数字.-字符串+字符串
转化之后是Unity自带的结构体:SemVersion
//Unity规定的版本文件结构
internal struct SemVersion
{
public int Major { get; }
public int Minor { get; }
public int Patch { get; }
public string Prerelease { get; }
public string Build { get; }
}
用户定义的 version 字符串会按照如下规则进行拆分,依次为 Major、Minor、Patch、Prerelease、Build:
第一个 '.' 前面的字符串(必须是0~9)为 Major;
之后下一个 '.' 前面的字符串(必须是0~9)为 Minor;
之后下一个 '-' 前面的字符串(必须是0~9)为 Patch ;
之后下一个 '+' 前面的字符串为 Prerelease;
之后剩余的字符串为 Build 。
如果不满足上诉规则则会导致序列化失效,Unity可能不会识别你的 Pacakage 文件。
注意,虽然看起来是可以Build和Prerelease这种的,但是建议还是不要加,以为依然可能序列化出错。(和下面的代码没关系,下面的代码测试正常,但是Unity还是会报错)所以建议版本还是简单地写 数字.数字.数字 的模式。
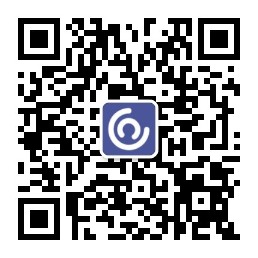
(我测试了 1.0.2+Something)的格式,不能转换成 SemVersion,但是是系统内置报错,这里找不到问题出在哪里。
下面提供一下Unity的反序列化代码,大家可以参考:
using System;
namespace UnityEditor.Scripting.ScriptCompilation
{
internal static class SemVersionParser
{
public static SemVersion Parse(string version, bool strict = false)
{
int cursor = 0;
int num = 0;
int minor = 0;
int patch = 0;
string prerelease = null;
string build = null;
try
{
num = int.Parse(VersionUtils.ConsumeVersionComponentFromString(version, ref cursor, (char x) => !char.IsDigit(x)));
if (cursor < version.Length && version[cursor] == '.')
{
cursor++;
minor = int.Parse(VersionUtils.ConsumeVersionComponentFromString(version, ref cursor, (char x) => !char.IsDigit(x)) ?? "0");
}
if (cursor < version.Length && version[cursor] == '.')
{
cursor++;
patch = int.Parse(VersionUtils.ConsumeVersionComponentFromString(version, ref cursor, (char x) => !char.IsDigit(x)) ?? "0");
}
if (cursor < version.Length && version[cursor] == '-')
{
cursor++;
prerelease = VersionUtils.ConsumeVersionComponentFromString(version, ref cursor, (char x) => x == '+');
}
if (cursor < version.Length && version[cursor] == '+')
{
cursor++;
build = VersionUtils.ConsumeVersionComponentFromString(version, ref cursor, (char x) => x == '\0');
}
}
catch (Exception)
{
throw new ArgumentException(version + " is not valid Semantic Version");
}
return new SemVersion(num, minor, patch, prerelease, build);
}
public static bool TryParse(string versionString, out SemVersion? result)
{
try
{
result = Parse(versionString);
return true;
}
catch (Exception)
{
result = null;
return false;
}
}
}
}
namespace UnityEditor.Scripting.ScriptCompilation
{
internal static class VersionUtils
{
public static string ConsumeVersionComponentFromString(string value, ref int cursor, Func<char, bool> isEnd)
{
int num = 0;
for (int i = cursor; i < value.Length && !isEnd(value[i]); i++)
{
num++;
}
int startIndex = cursor;
cursor += num;
return value.Substring(startIndex, num);
}
}
}