-
1.构造和析构
- 构造函数与析构函数
- 默认构造函数
- People* p = new People();
- People(string name);
- People* p = new People("tra);
-
2.三五法则
- 1.需要析构函数的类也需要拷贝构造函数和拷贝赋值函数。
- 4.如果一个类有删除的或不可访问的析构函数,那么其默认和拷贝构造函数会被定义为删除的。
- 5.如果一个类有const或引用成员,则不能使用合成的拷贝赋值操作。
-
3.static
-
4.Task
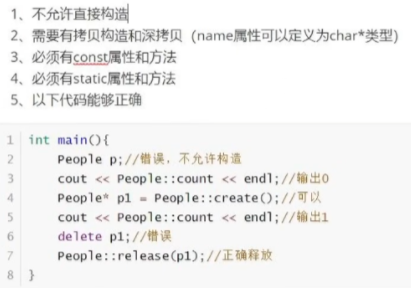
#include <iostream>
#include <string>
using namespace std;
class People
{
private:
string name;
int age;
float height;
float weight;
private:
//People() = delete;
//~People() = delete;
//People() {};
//~People() {};
People(): name("master"),age(0),height(0),weight(0) {
};
~People() {
};
public:
static int count;
static People* create() {
People::count++;
return new People();
}
static void release(People* p) {
delete p;
}
};
int People::count = 0;
int main() {
//People p; //错误,不允许构造
cout << People::count << endl; //输出0
People* p1 = People::create(); //可以
cout << People::count << endl; //输出1
//delete p1; //错误
People::release(p1); //正确释放
return 0;
}
-
5.mutable VS const
- const不能修改静态方法,可以修饰静态属性
- const方法: string& getName() const;
-
6.设计图书类
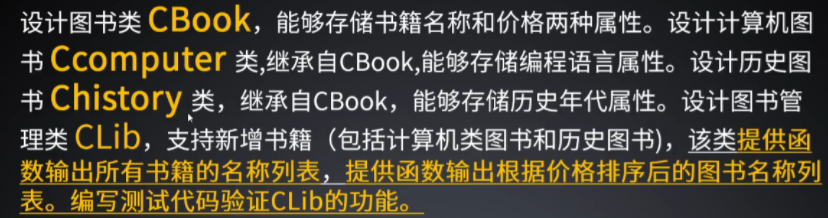
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
using namespace std;
class CBook{
private:
string _name;
int _price;
public:
CBook(const char* name, int price) : _name(name), _price(price) {
}
inline string name() {return _name;}
inline int price() {return _price;}
virtual string desc() = 0;
};
class CComputer: public CBook {
private:
string _desc;
public:
CComputer(const char* name, int price, const char* desc) : CBook(name, price), _desc(desc){
}
string desc(){return _desc;}
};
class CHistory : public CBook{
private:
string _date;
public:
CHistory(const char* name, int price, const char* date) : CBook(name, price), _date(date){
}
string desc(){return "日期:" + _date;}
};
class CLib{
private:
vector<CBook*> _books;
public:
void addBook(CBook* book) {
_books.push_back(book);
}
void listBooks() {
for (auto book: _books) {
cout << book->name() << " " << book->desc() << endl;
}
}
void listBooksOrdByPrice() {
sort(_books.begin(), _books.end(), [](CBook* a, CBook* b){
return a->price() > b->price();
});
for(auto book : _books) {
cout << book->name() << " " << book->price() << endl;
}
}
};
int main() {
CLib lib;
lib.addBook(new CComputer("C++", 55, "c++程序设计"));
lib.addBook(new CComputer("C语言", 66, "c程序设计"));
lib.addBook(new CComputer("Java", 140, "java程序设计"));
lib.addBook(new CComputer("历史", 40, "中国历史教程"));
lib.listBooks();
cout << "------------" << endl;
lib.listBooksOrdByPrice();
return 0;
}