C++实现文本界面英语词典
C++实现文本界面的英语词典,能在Dev-C++运行。提供两种方案:一是简单仅查词功能;二是具有查词、添加、删除功能,具有选择菜单,值得一提的是,本程序对用户输入菜单选项序号做了检测,排除了无效输入造成的不响应情况。
一、简单仅查词功能
使用map容器实现电子词典,map容器
可以理解为:一种映射,一对一(例如x对y),可以通过x查询到唯一对应的y。
本文实现的功能
读取电子词典的文件,一对一压入map容器中(即英文对应中文解释),然后通过英文,获得中文含义,以达到电子词典的功能。
电子词典的文件Dictionary1.txt,每行一词,单词和解释之间用一个空格分割 (解释中间不能有空格),如
a art.一(个);每一(个)
abandon vt.丢弃;放弃,抛弃
ability n.能力;能耐,本领
able a.有能力的;出色的
baby n.婴儿;孩子气的人
back ad.在后;回原处;回
background n.背景,后景,经历
cabinet n.橱,柜;内阁
cable n.缆,索;电缆;电报
cafe n.咖啡馆;小餐厅
good a.好的;有本事的
zoo n.动物园
源码如下:
#include <iostream>
using namespace std;
#include <map>
#include <fstream>
int main()
{
map<string, string> wordDict;
fstream fs;
fs.open("Dictionary1.txt", ios::in);
char buf[1024] = { 0 };
char key[200] = { 0 }; //单词
char value[300] = { 0 }; //解释
while (fs.peek() != EOF)
{
fs.getline(buf, 1024); //getline从文件中读取一行数据
sscanf(buf, "%s %s", key, value); //实现拆分,"%s %s"表示用空格分割符 分别存入key, value
wordDict.insert(pair<string, string>(key, value));
}
cout <<"单词数量:"<< wordDict.size() << endl; //单词数量(行数)
string word;
while (1)
{
cout << "请输入要查询的单词:" ;
cin >> word;
int res = wordDict.count(word);
if (res == 0)
{
cout << "未查询到该单词!" << endl;
}
else
{
cout << "单词解释:" ;
cout << wordDict[word] << endl;
}
}
return 0;
}
关于sscanf函数可见
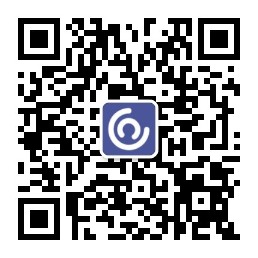
https://cplusplus.com/reference/cstdio/sscanf/
scanf, fscanf, sscanf, scanf_s, fscanf_s, sscanf_s - cppreference.com
运行效果:
二、具有查词、添加、删除功能
电子词典的文件Dictionary2.txt,其中第一行记录单词数量,其它行每行一词,单词和解释之间用一个空格分割 (解释中间不能有空格),如
12
a art.一(个);每一(个)
abandon vt.丢弃;放弃,抛弃
ability n.能力;能耐,本领
able a.有能力的;出色的
baby n.婴儿;孩子气的人
back ad.在后;回原处;回
background n.背景,后景,经历
cabinet n.橱,柜;内阁
cable n.缆,索;电缆;电报
cafe n.咖啡馆;小餐厅
good a.好的;有本事的
zoo n.动物园
源码如下
值得一提的是,本程序对用户输入菜单选项序号做了检测,排除了无效输入造成的不响应情况。
#include <windows.h>
#include <iostream>
#include <iomanip> //对齐输出用到
using namespace std;
int number; //全局变量单词总数
void menu(); //主界面
void translation_e(); //英译汉
void view(); //查看[列出]全部单词
void remove_(); //删除单词
void add(); //增加单词
void read(); //从文件中读取信息
void save() ; //保存单词到文件里函数
void back_t_e(); //英译汉 返回界面
void back_v(); //查看[列出]全部单词 返回界面
void back_r(); //删除单词 返回界面
void back_a(); //增加单词 返回界面
int CheckNum(int& n); //判断输入的字符串是否为正整数,若是,则合规返归其值 ,否则给出提示
struct words{
char english[30];
char chinese[60];
}word[100];
int main()
{
menu();
return 0;
}
void menu()//主界面
{
read();
int l;
cout<<"\t欢迎使用简易英汉词典\t\n";
cout <<"\t 1.单词查找 \t\n";
cout <<"\t 2.查看全部词库 \t\n";
cout <<"\t 3.删除单词 \t\n";
cout <<"\t 4.增加单词 \t\n";
cout <<"\t 5.退出 \n";
cout <<"=================================\n";
//处理输入非数字
int m;
while (true){
cout <<"请输入选择的编号: ";
l=CheckNum(m);
if(l>=1 && l<=5) //限制是合规的选项
{
break;
}
}
switch(l){
case 1:
system("cls");
translation_e();
break;
case 2:
system("cls");
view();
break;
case 3:
system("cls");
remove_();
break;
case 4:
system("cls");
add();
break;
case 5:
cout <<"感谢您的使用,已退出!\n";
exit(0);
}
}
//判断输入的字符串是否为正整数,若是,则合规返归其值 ,否则给出提示
int CheckNum(int& n)
{
int i;
string x; //用来接受输入
bool flag = false;
while (cin >> x) {
for (i = 0; i < x.size(); ++i) {
//判断是否为中文
if (x[i] & 0x80) {
cout << "\n输入错误,请重新输入正确的数字: ";
break;
}
//判断是否为字母或者其它字符
if (!isdigit(x[i])) {
cout << "\n输入错误,请重新输入正确的数字: ";
break;
}
}
if (i == x.size()) {
break; //如果字符串中所有字符都合法,则退出while循环
}
}
n = atoi(x.c_str()); //将仅含数字的string字符串转化为正整数
return n; //返回值
}
void translation_e() //英译汉
{
char eng[30]="\0";
int i;
cout <<"输入要查询的单词: ";
getchar();//去掉回车
cin >>eng;
for ( i =0;i<number;i++)
{
if (strcmp(eng,word[i].english)==0 )
{
cout <<"\n该单词含义: ";
cout <<word[i].chinese<<"\n";
break;
}
}
if (i == number)
{
cout <<"\n[错误]未找到该单词!\n";
}
back_t_e();
}
void back_t_e() //英译汉(查询单词)返回界面
{
int l;
cout <<"\n1.继续";
cout <<"\n2.返回";
cout <<"\n=========================";
cout <<"\n请输入要执行的操作: ";
//cin >>l;
//处理输入非数字
int m;
while (true){
cout <<"请输入选择的编号: ";
l=CheckNum(m);
if(l>=1 && l<=2) //限制是合规的选项
{
break;
}
}
switch(l){
case 1:
system("cls");
translation_e();
break;
case 2:
system("cls");
menu();
break;
}
}
void save() //保存单词到文件里函数
{
FILE* fp;
int i,ret;
fp=fopen("Dictionary2.txt", "w"); //单词文本文件
if (fp == NULL)
{
cout <<"\n[警告]文件打开失败\n";
}
fprintf(fp,"%d\n",number);
for (i = 0; i <number; i++)
{
ret=fprintf(fp,"%s %s\n",word[i].english,word[i].chinese);
}
if (ret<0)
{
cout <<"\n[错误]文件写入失败\n";
}
else
{
cout <<"\n[tip]文件写入成功\n";
}
fclose(fp); //关闭文件指针
}
void read()//从文件中读取信息
{
FILE *fp;
int i;
fp=fopen("Dictionary2.txt","r"); //单词文本文件
if(fp==NULL)
{
cout <<"[警告]文件打开失败\n";
}
else {
cout <<"[tip]文件读取成功\n";
fscanf(fp,"%d\n",&number);
for(i=0;i<number;i++)
{
fscanf(fp,"%s %s\n",word[i].english,word[i].chinese);
}
}
fclose(fp);
}
void add() //增加单词
{
int i = number, j, flag,m;
//cout <<"待增加的单词个数:";
//cin >>m;
//处理输入非数字
int n;
while (true){
cout <<"待增加的单词个数[限制一次1`20]: ";
m=CheckNum(n);
if(m>=1 && m<=20) //限制是合规的选项
{
break;
}
}
getchar();
if (m > 0){
do
{
flag = 1;
cout <<"\n请输入第"<< i + 1<<"个单词:\n";
while (flag)
{
flag = 0;
cout <<"英文:";
cin>>word[i].english;
for (j = 0; j < i; j++){
if (strcmp(word[i].english,word[j].english)==0){
cout <<"[错误]已存在该单词,请重新输入!\n";
flag = 1;
break;
}
}
}
getchar();
cout <<"中文[不能含有空格]: ";
cin >> word[i].chinese;
if (0 == flag){
i++;
}
} while (i<number + m);
number += m;
cout <<"[成功]单词添加完毕!!!";
save();
}
back_a();
}
void back_a() //增加单词 返回界面
{
int l;
cout <<"\n1.继续";
cout <<"\n2.返回";
cout <<"\n=========================";
cout <<"\n请输入要执行的操作: ";
//in >> l;
//处理输入非数字
int m;
while (true){
cout <<"请输入选择的编号: ";
l=CheckNum(m);
if(l>=0 && l<=2) //限制是合规的选项
{
break;
}
}
switch(l){
case 1:
system("cls");
add();
break;
case 2:
system("cls");
menu();
break;
}
}
void view() //查看[列出]全部单词
{
int i;
cout <<" 英文\t\t\t 中文\n";
cout <<" --------------------------------------------------------------------------\n";
for(i=0;i<number;i++)
{
cout <<left<<setw(30)<<word[i].english<<left<<setw(60)<<word[i].chinese<<"\n";
}
cout <<" --------------------------------------------------------------------------\n";
back_v() ;
}
void back_v() //查看[列出]全部单词 返回界面
{
int l;
cout <<"1.继续";
cout <<"\n2.返回";
cout <<"\n=========================";
cout <<"\n请输入要执行的操作: ";
//cin >>l;
//处理输入非数字
int m;
while (true){
cout <<"请输入选择的编号: ";
l=CheckNum(m);
if(l>=0 && l<=2) //限制是合规的选项
{
break;
}
}
switch(l){
case 1:
system("cls");
view();
break;
case 2:
system("cls");
menu();
break;
default:
cout <<"[输入错误]请重新输入有效数字!\n";
Sleep(3000);
back_v();
break;
}
}
void remove_() //删除单词
{
int tab=0; //标记
int a;
char eng1[30];
cout <<"请输入要删除之单词:";
//scanf("%s",eng1);
cin >>eng1;
int i, j;
for (i = 0;i<number; i++)
{
if (strcmp(word[i].english,eng1)==0)
{
cout <<"你将要删除该单词\n\n";
cout <<" --------------------------------------------------------------------------\n";
cout <<left<<setw(30)<<word[i].english<<left<<setw(604)<<word[i].chinese<<"\n";
cout <<" --------------------------------------------------------------------------\n";
cout <<"\n1.确认删除";
cout <<"\n2.取消删除";
cout <<"\n=========================";
cout <<"\n请输入:";
//cin >> a;
//处理输入非数字
int m;
while (true){
cout <<"请输入选择的编号: ";
a=CheckNum(m);
if(a>=0 && a<=2) //限制是合规的选项
{
break;
}
}
if (a == 1)
{
for(j=i;j<number-1;j++){
word[j]=word[j+1];
}
cout <<"\n[tip]删除成功!";
tab=1;
number--;
save();
break;
}
else
{
cout <<"\n[tip]已取消操作!";
tab=1;
break;
}
}
}
//if(i==number)
if(tab==0)
{
cout <<"\n[错误]未找到该单词!!!";
}
back_r() ;
}
void back_r() //删除单词 返回界面
{
int l;
cout <<"\n1.继续";
cout <<"\n2.返回";
cout <<"\n=========================";
cout <<"\n请输入要执行的操作: ";
//cin >> l;
//处理输入非数字
int m;
while (true){
cout <<"请输入选择的编号: ";
l=CheckNum(m);
if(l>=0 && l<=2) //限制是合规的选项
{
break;
}
}
switch(l){
case 1:
system("cls");
remove_();
break;
case 2:
system("cls");
menu();
break;
}
}
运行效果: